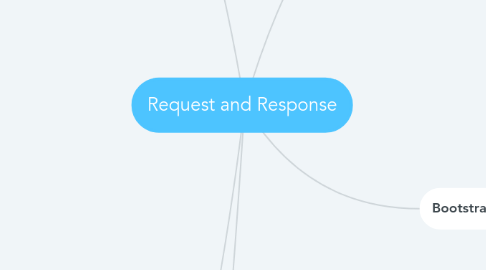
1. Request Object
1.1. extension from Symfony's HttpFoundation\Request
1.2. getting a Raw request: $request = Illuminate\Http\Request::capture()
1.3. Getting Laravel Request
1.3.1. type-hinting: ``` public function index(Request $request){ } ```
1.3.2. using global helper: $request = request()->all()
1.3.3. using app() global methods . app(Illuminate\Http\Request::class); . app('request');
1.4. Get Basic Information about a Request
1.4.1. Basic User input
1.4.1.1. all(): return array of all user-inputted
1.4.1.2. input(fieldname): returns value of single user-provided input field
1.4.1.3. only(fieldname, [array,of,field,names]): returns array of all user-input for the specified field names
1.4.1.4. except(fieldname, [array,of,field,names]): inverse of only
1.4.1.5. exist(fieldName): returns boolean whether fieldName exists in the input
1.4.1.6. has(fieldName): return boolean whether fieldName exists and has value
1.4.2. User and request state
1.4.2.1. method(): return method (GET, POST, PATCH, etc) used to access this data
1.4.2.2. path(): returns the path(without domain) to access this page
1.4.2.3. url(): return the URL(with domain) to access this page
1.4.2.4. is(): return boolean whether current path fuzzy-matches a provided string (eg: /a/b/c will be match by is('*b*)
1.4.2.5. ip(): return user's ip
1.4.2.6. header(): return array of headers, or passing header name as parameter
1.4.2.7. server(): return array of $_SERVER
1.4.2.8. secure(): return boolean whether this page is loaded with https
1.4.2.9. pjax(): returns a boolean whether this page is loaded with Pjax
1.4.2.10. wantsJson(): return boolean whether this request expects Json response (by Accept params in Header)
1.4.2.11. isJson(): return boolean whether this request content is Json (by checking request's header Content-Type
1.4.2.12. accepts(): return boolean whether this page request accepts a given content
1.4.3. Files
1.4.3.1. file(): return array of all uploaded files
1.4.3.2. hasFile(): returns a boolean whether a file was uploaded at the specified key
1.4.4. ParameterBag: associate arrays, get a particular item using $bag->get
1.5. Persistence
1.5.1. request can also interact with session
1.5.2. flash(): save request input to session
1.5.3. flush(): wipes all previously flash input
2. Response Object
2.1. extends Symfony/HttpFoundation/Response
2.2. Using and creating Response Objects in Controllers
2.2.1. Setting Headers
2.2.2. Adding cookies
2.3. Specialize Response Types
2.3.1. View Responses
2.3.2. Download Responses
2.3.3. File Responses
2.3.4. Json Responses
2.3.5. Redirect response
2.3.5.1. aren't commonly call on response() helper
2.3.5.2. redirect()->route: redirect to a route
2.3.5.3. redirect()->action(): redirect to an action
2.3.5.4. back()->withInput(): redirect back with Input
2.3.6. Custom Response Macros
2.3.6.1. register macro in AppServiceProvider
2.3.6.2. public function boot(){ Response::macro('myMacro', function($content){ return response(json_encode($content))->headers['Content-Type' => 'application/json']); }
3. Middleware
3.1. Definition: layer wrapping around applications
3.2. Middleware can inspect/decorate request.response
3.3. Sample code for decorating response
3.4. Binding Middleware
3.4.1. Global Middleware
3.4.1.1. binding in `app\Http\Kernel.php`
3.4.1.2. sample code:
3.4.2. Route Middleware
3.4.2.1. define routeMiddleware in `app\Http\Kernel`
3.4.2.2. sample code:
3.4.2.3. binding sample code:
3.4.3. Using Middleware groups
3.4.3.1. bundles of middleware that makes sense to be together in specific context
3.4.3.2. two default middleware group: web and api
3.4.3.3. the two middleware groups are mapped in routes/api.php file
3.5. Passing Parameter to Middleware
3.5.1. Parameter can be passing from routes to Middleware
3.5.2. split middleware name with parameters by `:`, and split between parameters by ','
3.5.3. sample code:
4. Laravel's request Lifecycle
4.1. Request coming to app via Middleware
4.2. Middleware decorate the request
4.3. Application resolve the request and makes response
4.4. Response is decorated (in reverse order comparing to request-decorated)
4.5. User receive response
5. Bootstrapping the Application
5.1. The flow of bootstrapping as above
5.2. kernel is core router of every Laravel Application
5.2.1. Web kernel
5.2.2. Console kernel (for console commands)
5.2.3. has `handle()` methods for taking in Illuminate Request and returning Illuminate Response
5.3. Service Provider
5.3.1. class that encapsulates logic that application need to run
5.3.2. ServiceProvider have two important methods: `boot` and `register`
5.3.2.1. register: bind classes and aliases to container
5.3.2.2. boot: binding event listener, defining routes
5.3.3. defer a service providers to stop bootstrapping unless the bindings is explicitly requested from container