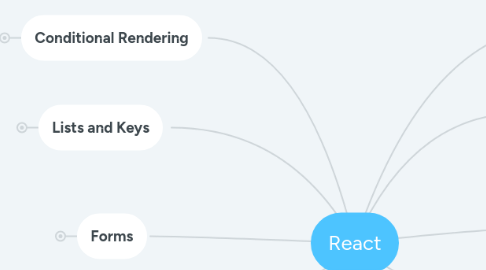
1. Conditional Rendering
1.1. Element Variables
1.2. Inline If with Logical && Operator
1.3. Inline If-Else with Conditional Operator
1.4. Preventing Component from Rendering
2. Lists and Keys
2.1. Rendering Multiple Components
2.2. Basic List Component
2.3. Keys
2.4. Extracting Components with Keys
2.5. Keys Must Only Be Unique Among Siblings
3. Forms
3.1. Controlled Components
3.2. Uncontrolled Components
4. Lifting State Up
5. Composition vs Inheritance
6. Thinking In React
6.1. Step 1: Break The UI Into A Component Hierarchy
6.1.1. single responsibility principle
6.2. Step 2: Build A Static Version in React
6.3. Step 3: Identify The Minimal (but complete) Representation Of UI State
6.3.1. DRY: Don’t Repeat Yourself
6.3.2. Is it passed in from a parent via props? If so, it probably isn’t state.
6.3.3. Does it remain unchanged over time? If so, it probably isn’t state.
6.3.4. Can you compute it based on any other state or props in your component? If so, it isn’t state.
6.4. Identify Where Your State Should Live
6.4.1. Identify every component that renders something based on that state.
6.4.2. Find a common owner component (a single component above all the components that need the state in the hierarchy).
6.4.3. Either the common owner or another component higher up in the hierarchy should own the state.
6.4.4. If you can’t find a component where it makes sense to own the state, create a new component solely for holding the state and add it somewhere in the hierarchy above the common owner component.
7. JSX
7.1. React element
7.1.1. Plain Object
7.1.2. Immutable Object
7.2. Describe UI what see on screen
8. Render Element
8.1. Rendering an Element into the DOM
8.1.1. ReactDOM.render(<elements/>, rootDoom)
8.2. Updating the Rendered Element
8.2.1. React Only Updates What’s Necessary
9. Component
9.1. Independent, reusable pieces, and think about each piece in isolation
9.2. Function and Class Components
9.3. Rendering a Component
9.4. Composing Components
9.5. Extracting Components
9.6. Props are Read-Only
10. Props
10.1. Immutable
10.2. Pass data from top to down
10.2.1. way one data flow
10.3. re-render whenever new props
11. State and Lifecycle
11.1. private and fully controlled by the component
11.2. Mounting, updating, unmounting
11.3. re-render whenever state changes
11.4. Using State Correctly
11.4.1. Do Not Modify State Directly
11.4.2. State Updates May Be Asynchronous
11.4.3. State Updates are Merged
11.4.4. The Data Flows Down