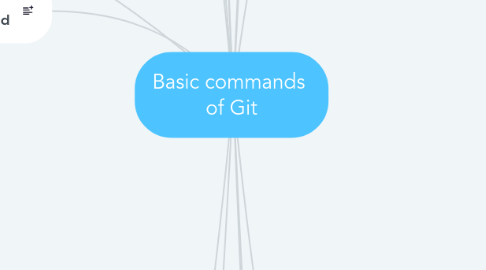
1. A Gist containing some good rules link
2. With graph (useful for branches)
3. Initializing a Project
3.1. git init
4. Reporting difference between WD,SA and R
4.1. git status
5. Viewing commits
5.1. Displaying all commits
5.1.1. git log
5.2. With a limit of commits
5.2.1. git log -[n]
5.3. Searching commits by date
5.3.1. git log --since="[yyyy-mm-dd]"
5.3.2. git log --until="[yyyy-mm-dd]"
5.3.3. git log --since="2 weeks ago" --until="3 days ago"
5.3.4. git log --since=2.weeks --until=3.days
5.4. Searching commits by author
5.4.1. git log --author = "[name]"
5.5. Searching commits by commit message
5.5.1. git log --grep = "[message]"
5.6. Getting a compressed list in one line
5.6.1. git log --oneline
5.6.2. git log --oneline -[n]
5.6.3. git log [SHA-value]..[SHA-value] --oneline
5.7. Give the logs that affect to a particular file
5.7.1. git log [SHA-value].. [file_name]
5.8. Showing a diff of what changed in every file
5.8.1. git log -p
5.9. Getting the statics about the changes in each file
5.9.1. git log --stat --summary
5.10. Getting the one-line log with full SHA
5.10.1. git log --format=oneline
5.11. Getting the log information in email format
5.11.1. git log --format=email
5.12. Getting the log in raw format
5.12.1. git log --format=raw
5.13. Try this format
5.13.1. git log --oneline --graph --all --decorate
5.14. Showing the full SHA, author, date, the commit message and the diff of a commit
5.14.1. git log --format=graph
5.14.2. git show [SHA-value]
5.14.3. git show [tree-ish] e.g. HEAD
6. Ignoring files to track or files you don't want to add
6.1. Project directory level
6.1.1. nano .gitignore
6.2. User directory level
6.2.1. Linux / Unix
6.2.1.1. nano /Users/[user_name_directory]/.gitignore_global
6.2.1.1.1. git config --global core.excludesfile ~/.gitignore_global
6.2.2. Windows
6.2.2.1. Use your preference text editor, create a file with .gitignore_global extension
6.2.2.1.1. git config --global core.excludesfile ~/.gitignore_global
6.3. What files to ignore?
6.3.1. A collection of useful .gitignore templates rules link
6.4. Ignoring tracked files
6.4.1. It's going to remove from the Staging Area, not from Repository and my Working Directory either
6.4.1.1. git rm --cached [file_name]
6.5. Tracking empty directories
6.5.1. touch [directory_path/directory_name_to_track]/.gitkeep
6.5.2. [directory_path/directory_name_to_track]> type nul > .gitkeep
7. Navigating the commit tree
7.1. What is a Tree-ish?
7.2. How Git can reference a commit?
7.2.1. full SHA-1 hash
7.2.2. short SHA-1 hash
7.2.2.1. Small Project
7.2.2.1.1. Current commit
7.2.2.1.2. Parent commit
7.2.2.1.3. Grand parent commit
7.2.2.1.4. Great grand parent commit
7.2.2.1.5. [n] parent commit
7.2.2.2. Medium project
7.2.2.3. Large project
7.2.3. HEAD Pointer
7.2.3.1. Current commit
7.2.3.1.1. git ls-tree HEAD
7.2.3.2. Parent commit
7.2.3.2.1. git ls-tree HEAD^
7.2.3.2.2. git ls-tree HEAD~1, git ls-tree HEAD~
7.2.3.3. Grand parent commit
7.2.3.3.1. git ls-tree HEAD~2
7.2.3.4. Great grand parent commit
7.2.3.4.1. git ls-tree HEAD~3
7.2.3.5. [n] parent commit
7.2.3.5.1. git ls-tree HEAD~[n]
7.2.4. Branch reference
7.2.4.1. Current commit
7.2.4.1.1. git ls-tree master
7.2.4.2. Parent commit
7.2.4.2.1. git ls-tree master^
7.2.4.2.2. git ls-tree master~1, git ls-tree master~
7.2.4.3. Grand parent commit
7.2.4.3.1. git ls-tree master~2
7.2.4.4. Great grand parent commit
7.2.4.4.1. git ls-tree master~3
7.2.4.5. [n] parent commit
7.2.4.5.1. git ls-tree master~[n]
7.2.5. Tag reference
8. Remote Repository
8.1. Listing all remote repositories
8.1.1. git remote
8.1.2. git remote -v
8.1.3. cat .git/config
8.1.4. ls -la .git/refs/remotes
8.1.4.1. ls -la .git/refs/remotes/[alias]
8.1.4.2. cat .git/refs/remotes/[alias]/master
8.1.5. git branch -r
8.1.6. git branch -a
8.2. Adding a remote
8.2.1. git remote add [alias_remote_repo] [URL]
8.3. Removing a remote
8.3.1. git remote rm [alias]
8.4. Creating a remote branch
8.4.1. git push -u [alias] [branch_name]
8.5. Creating non tracking remote branch
8.5.1. git push [alias] [branch_name]
8.6. Cloning from remote server repository
8.6.1. git clone [URL] <alias>
8.7. Viewing changes
8.7.1. git diff [alias]/[branch_name]..[branch_name]
8.8. Converting remote branch from non remote branch
8.8.1. git config branch.[non_tracking_branch_name].remote [alias]
8.8.2. git config branch.[non_tracking_branch_name].merge refs/heads/master
8.8.3. git branch --set-upstream [non-tracking_branch_name] [alias]/[non-tracking_branch_name]
8.9. Pushing changes to the remote repository
8.9.1. git push
8.10. Synchronizing remote repo to local repo
8.10.1. git fetch
8.11. Merging changes
8.11.1. git merge [alias]/[branch_name]
8.12. Synchronizing and merging in one step
8.12.1. git pull
8.13. Checking out remote branches
8.13.1. git branch [new_branch_name] [alias]/[remote_branch_name]
8.14. Deleting remote branch
8.14.1. git push [alias_remote_repo_name] :[remote_branch_name]
8.14.2. git push [alias_remote_repo_name] --delete [remote_branch_name]
9. Repository
9.1. Undoing multiple commits by moving the HEAD Pointer. Rewinding the HEAD pointer back in time, so that it records from there going forward.
9.1.1. git reset
9.1.1.1. The changes are still in the Staging Area and in the Working Directory
9.1.1.1.1. git reset --soft [SHA-value]
9.1.1.2. The changes are still only in the Working Directory
9.1.1.2.1. git reset --mixed [SHA-value]
9.1.1.3. The changes are not tracking and the Working Directory is clean
9.1.1.3.1. git reset --hard [SHA-value]
9.2. Making a new commit which undoes all the changes from an existing one
9.2.1. git revert [SHA-value]
9.3. Amending commits
9.3.1. git commit --amend -m “[message]”
9.4. Retrieving old version from the current branch
9.4.1. git checkout [SHA-value] -- [file_name]
9.5. Sending the changes to the Remote Repository
9.5.1. git push
10. Staging Area
10.1. Viewing the changes
10.1.1. git diff --staged [file_name]
10.1.2. git diff --staged --color-words [file_name]
10.2. Unstaging changes or sending back to the WD
10.2.1. git reset HEAD [file_name]
10.3. Sending the changes to the Repository
10.3.1. git commit -m "[message]"
11. Working Directory
11.1. Viewing the changes
11.1.1. git diff [file_name]
11.1.2. git diff --color-words [file_name]
11.1.3. Showing the difference between the directory at some point in time and my current working directory
11.1.3.1. git diff [SHA-value]
11.1.4. Getting a comparison between at the previous point in time and the working directory with a specific file.
11.1.4.1. git diff [SHA-value] [file_name]
11.1.5. Getting a comparison between two different points in time
11.1.5.1. git diff [SHA-value]..[SHA-value]
11.1.6. Getting a comparison between two different points in time with a specific file
11.1.6.1. git diff [SHA-value]..[SHA-value] [file_name]
11.1.7. Getting a comparison with a tree-ish
11.1.7.1. git diff [SHA-value]..[tree-ish]~[n]
11.1.8. Ignoring whether or not someone changed spaces
11.1.8.1. git diff --stat --summary [SHA-value]..[tree-ish]~[n]
11.1.8.2. git diff --ignore-space-change [criteria]
11.1.8.3. git diff -b [criteria]
11.1.9. Ignoring all space, every single change that could be made to space
11.1.9.1. git diff --ignore-all-space [criteria]
11.1.9.2. git diff -w [criteria]
11.1.10. Comparing with the latest commit
11.1.10.1. git diff --cached
11.2. Undoing changes from the current branch
11.2.1. git checkout -- [file_name]
11.3. Sending the changes to the Staging Area
11.3.1. git add [file_name]
11.3.2. git add .
11.4. Deleting file(s) and sending to the Staging Area
11.4.1. git rm [file_name]
11.5. Moving or renaming file(s) and sending to the Staging Area
11.5.1. git mv [file_name]
11.6. Sending the changes to the Repository (tracked files)
11.6.1. git commit -am "[message]"
11.7. Deleting untracked files
11.7.1. Testing run
11.7.1.1. git clean -n
11.7.2. Removing files
11.7.2.1. git clean -f
12. Branching
12.1. Viewing all the branches in our local repository
12.1.1. git branch
12.2. Creating a new branch
12.2.1. git branch [branch_name]
12.3. Where the HEAD pointer points to?
12.3.1. cat .git/HEAD
12.3.1.1. Result: ref: refs/heads/[current_branch_name]
12.3.1.1.1. Checking the heads directory
12.3.1.1.2. Take a look what's in the [current_branch_name] branch
12.4. Switching branches
12.4.1. git checkout [new_branch_name]
12.4.1.1. Examples for naming git branches
12.4.2. Creating a new branch and switch at the same time
12.4.2.1. git checkout -b [branch_name]
12.4.2.2. git checkout -b [branch_name] [alias_for_remote_repository]/[remote_branch_name]
12.5. Comparing branches
12.5.1. git diff [branch_name]..[branch_name]
12.5.2. git diff --color-words [branch_name]..[branch..name]
12.6. Renaming branches
12.6.1. git branch -m [old_branch_name] [new_branch_name]
12.7. Deleting branches
12.7.1. git branch -d [branch_name_to_delete]
12.7.2. git branch -D [branch_name_to_delete]
12.8. Configuring the command prompt to show the branch
12.8.1. Windows
12.8.1.1. Create a new file on NotePad and save it as .bash_profile: export PS1='Your-Name[\W]$(__git_ps1 "(%s)"): '
12.8.1.2. In order to run that file: source ~/.bash_profile
12.8.2. Unix
12.8.2.1. Download the .git-prompt.sh file from Command Line: curl -o ~/.git-prompt.sh \ https://raw.githubusercontent.com/git/git/master/contrib/completion/git-prompt.sh
12.8.2.2. Edit the .bash_profile and ADD the following code: if [ -f ~/.git-prompt.sh ]; then source ~/.git-prompt.sh export PS1='Your-Name[\W]$(__git_ps1 "(%s)"): ' fi
12.9. Showing all branches that are completely included into the checkout (current) branch that we are on
12.9.1. git branch --merged
12.10. Merging code
12.10.1. git merge [branch_name]
12.10.1.1. Fast-Forward
12.10.1.2. git merge --no-ff [branch_name]
12.10.1.3. git merge --ff-only [branch_name]
12.10.1.4. True merge or Non-Fast-Forward merge
12.10.2. Merging conflicts
12.10.2.1. Resolving merge conflicts
12.10.2.1.1. Abort the merge
12.10.2.1.2. Resolve the conflicts manually
12.10.2.1.3. Merge tool
13. Saving changes in the stash
13.1. git stash save "[message]"
13.2. Viewing stashed changes
13.2.1. git stash list
13.2.2. git stash show stash@{n}
13.2.3. git stash show -p stash@{n}
13.3. Retrieving stashed changes
13.3.1. git stash pop stash@{n}
13.3.2. git stash apply stash@{n}
13.4. Deleting stashed changes
13.4.1. git stash drop stash@{n}
13.4.2. git stash clear