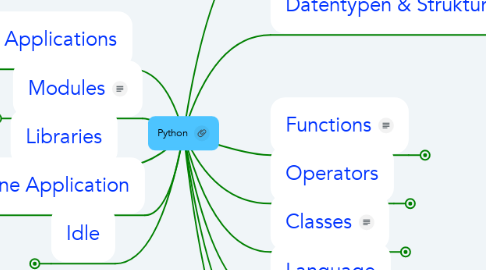
1. Python Applications
1.1. Sugar
1.2. Scripts
2. Modules
2.1. os Operating System
2.2. shutil File and Directory Management
2.3. sys
2.4. Install a package
3. Libraries
3.1. PyQt
3.2. TKinter
3.2.1. Root Widget
3.2.2. Tkinter Classes
3.2.3. Widget Configuration Interface
3.2.4. Events
3.3. wxPython
3.3.1. wx.Frame
3.3.2. wxWindow
3.3.3. Elemente
3.3.3.1. self.CreateStatusBar()
3.3.3.2. Common Dialogs
3.3.3.2.1. OnOpen
3.3.3.2.2. wx.FileDialog
3.3.3.3. Within a Frame
3.3.3.3.1. wx.TextCtrl
3.3.3.3.2. wxStaticText
3.3.3.3.3. wxButton
3.3.3.3.4. wx.MenuBar()
3.3.3.3.5. wx.Menu()
3.3.3.3.6. wxStatusBar
3.3.3.3.7. wxToolBar
3.3.3.3.8. wxPanel
3.3.4. Layout
3.3.4.1. manually position the elemnts by specifying x,y coordinates in the parent window.
3.3.4.2. wxLayoutConstraints
3.3.4.3. Layout Anchors
3.3.4.4. wxSizer subclasses
3.3.4.4.1. wxBoxSizer
3.3.4.4.2. wxGridSizer
3.3.4.4.3. wxFlexGridSizer
3.3.4.5. wxGlade
3.3.5. Callbacks
3.3.6. Dokumentation
3.3.6.1. Tutorial
3.3.7. SPE
3.4. matplotlib
3.5. NumPy
3.6. Requests
3.7. SQLAlchemy
4. create a Standalone Application
4.1. py2exe
5. Idle
5.1. Interpreter Fenster
5.2. Programmier Fenster
5.2.1. Run->Run-Module (F5)
5.3. import <module> <module>.main()
6. Control Structures
6.1. loops
6.1.1. for
6.1.1.1. advanced Looping
6.1.1.1.1. iteritems()
6.1.1.1.2. enumerate()
6.1.1.1.3. zip - loop over n seq
6.1.1.1.4. reversed()
6.1.1.1.5. sorted()
6.1.2. while
6.1.3. break, continue
6.1.4. >>range(10) [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
6.2. branches
6.2.1. if
6.2.2. there is no switch statement
7. Datentypen & Strukturen
7.1. List [1,2,3]
7.1.1. a.index(e)
7.1.2. a.remove(e)
7.1.3. del a[2:4]
7.1.4. a.insert(index, e)
7.1.5. a.reverse()
7.1.6. a.sort()
7.1.7. a.count(e)
7.2. Tupel (1,2,3)
7.3. Set
7.4. Dictionary {"key1" : "val1", "key2" : "val2"}
7.5. Typ Conversion
7.5.1. hex(x)
7.5.2. int(x)
7.5.3. ord(x)
7.5.4. chr(x)
7.5.5. unichr(x)
7.5.6. oct(x)
8. Functions
8.1. Default Values
9. Operators
9.1. Boolean Operations
9.2. Math
10. Classes
10.1. Inheritance
10.1.1. super()
10.2. Konstruktor
10.3. Metaklassen
10.3.1. Registrieren einer Klasse in einer Liste
10.4. Class.Method(par1)
10.5. Dynamic Class Structure
11. Language
11.1. Documentation Strings
11.2. print()
11.3. Regular Expressions
11.4. Trickkiste
11.4.1. if __name__ == "__main__":
12. Directories
12.1. os.path.walk(rootdir, f, arg)
13. Files
13.1. Textfiles
13.1.1. read linewise
13.2. Binary Files
13.2.1. Read binary Data