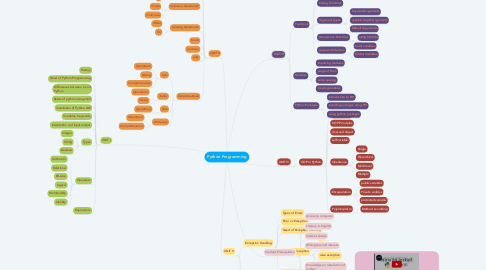
1. UNIT V
1.1. Exception Handling
1.1.1. Types of Errors
1.1.2. Error vs Exception
1.1.3. Need of Exception Handling
1.1.4. Handling Exception
1.1.4.1. try
1.1.4.2. except
1.1.4.3. raise exception
1.1.4.4. finally
1.1.5. user defined exception
1.2. Regular expressions
1.3. Turtle Graphics
2. UNIT I
2.1. History
2.2. Need of Python Programming
2.3. Differences between C and Python
2.4. Basics of python using shell
2.5. Installation of Python IDE
2.6. Variables, Keywords
2.7. Indentation and Input-output
2.8. Types
2.8.1. Integer
2.8.2. String
2.8.3. Boolean
2.9. Operators
2.9.1. Arithmetic
2.9.2. Relational
2.9.3. Bit-wise
2.9.4. Logical
2.9.5. Membership
2.9.6. Identity
2.10. Expressions
3. UNIT II
3.1. Control Flow
3.2. Order of Evaluations
3.3. Selection Statements
3.3.1. if
3.3.2. if else
3.3.3. if elif else
3.4. Looping Statements
3.4.1. While
3.4.2. for
3.5. break
3.6. continue
3.7. pass
3.8. Data Structures
3.8.1. Lists
3.8.1.1. Operations
3.8.1.2. Slicing
3.8.1.3. Comprehensions
3.8.2. Tuples
3.8.2.1. Operations
3.8.2.2. Slicing
3.8.3. Sets
3.8.3.1. Operations
3.8.4. Dictionary
3.8.4.1. Operations
3.8.4.2. Comprehensions
4. UNIT IV
4.1. OOP in Python
4.1.1. Need of OOP
4.1.2. OOP Principles
4.1.3. class and object
4.1.4. self variable
4.1.5. Inheritance
4.1.5.1. Single
4.1.5.2. Hierarchical
4.1.5.3. Multi level
4.1.5.4. Multiple
4.1.6. Encapsulation
4.1.6.1. public variables
4.1.6.2. Private varibles
4.1.6.3. protected variable
4.1.7. Polymorphism
4.1.7.1. Method overriding
5. UNIT III
5.1. Functions
5.1.1. Defining Functions
5.1.2. Calling Functions
5.1.3. Argument Types
5.1.3.1. Keyword Arguments
5.1.3.2. variable length Arguments
5.1.3.3. Default Arguments
5.1.4. Anonymous Functions
5.1.4.1. using lambda
5.1.5. scope and life time
5.1.5.1. Local variables
5.1.5.2. Global Variables
5.2. Modules
5.2.1. importing modules
5.2.2. usage of from
5.2.3. name spacing
5.2.4. creating modules
5.3. Python Packages
5.3.1. introduction to PIP
5.3.2. installing packages using PIP
5.3.3. using python packages
6. Student Prerequisites
6.1. Access to computer
6.2. Literacy in English
6.3. Internet Access
6.4. Willingness and interest
6.5. Knowledge on Installation of python
6.5.1. How to install Python on Windows 10 | Works with CMD!