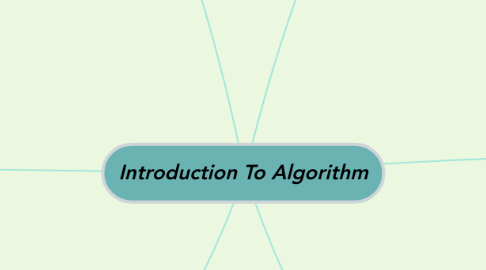
1. Mathematical Analysis
1.1. Recursive
1.1.1. Factorial
1.1.2. Tower of Hanoi
1.2. Non Recursive
1.2.1. Maximum Element
1.2.2. Distinct Element
1.2.3. Matrix Multiplication
2. Important Types
2.1. Searching
2.1.1. Linear Search
2.1.2. Binary search
2.2. Sorting
2.3. String Processing
2.4. Graph Problems
2.5. Combinatorial Problems
3. Fundamental Data Structure
3.1. Linear Data Structure
3.1.1. Array
3.1.2. Linked List
3.1.2.1. Singly Linked List
3.1.2.2. Doubly linked List
3.1.3. List
3.1.3.1. Stack
3.1.3.2. Queue
3.1.3.3. Priority Queue
3.2. Graphs
3.2.1. Directed
3.2.2. Undirected
3.2.3. Graph Representation
3.2.3.1. Adjacency Matrix
3.2.3.2. Adjacency Lists
3.2.3.3. Weighted Graph
3.3. Trees
3.3.1. Rooted Tree
3.3.1.1. Depth
3.3.1.2. Height
3.3.2. Ordered Tree
3.3.2.1. Binary Tree
3.3.2.2. Binary Search Tree
3.4. Sets and Dictionaries
4. Introduction
4.1. Algorithm
4.1.1. Input and Output
4.1.2. Definiteness
4.1.3. Finitenesss
4.1.4. Effectiveness
4.2. Algorithmic Specification
4.2.1. Simple English
4.2.2. Graphical Representation
4.2.3. Programming Languages
4.3. Analysis Frame Work
4.3.1. Measuring Input's Size
4.3.2. Units for Measuring Running Time
4.3.3. Order of Growth
5. Performance Analysis
5.1. Space Complexity
5.1.1. Fixed Part
5.1.2. Variable Part
5.2. Time Complexity
5.2.1. Method 1 (to introduce count variable)
5.2.2. Method 2 ( by building a step count table)