Pythonic Programming
저자: Thomas Heinrichsdobler
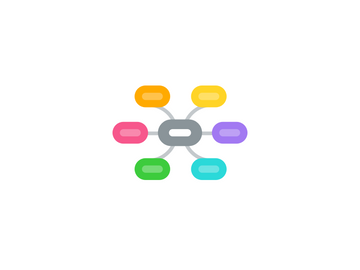
1. Links
1.1. Code Like a Pythonista
1.2. Idioms and Anti-Idioms in Python
1.3. Python Idioms and Efficiency Suggestions
2. Syntax
2.1. use parenthesis for long-line wrapping
2.2. use r"C:\temp.txt" for raw strings
2.2.1. Backslashes not interpreted as escapes
2.2.2. ideal for Windows file paths
2.3. use "".join(list) to join a list of strings
2.3.1. add spaces or commas as needed
2.3.1.1. " ".join(list)
2.3.1.2. ", ".join(list)
2.3.2. use generator functions
2.3.2.1. "".join(fn(i) for i in items)
2.4. use collections.defaultdict() to create dictionaries with default factory functions
2.5. use locals() for named string formatting
2.6. Use a list comprehension when a computed list is the desired end result.
2.6.1. [n ** 2 for n in range(10) if n % 2]
2.6.2. all values calculated at once
2.6.3. greedy evaluation
2.7. Use a generator expression when the computed list is just an intermediate step.
2.7.1. values calculated as needed
2.8. use lists instead of concatenating strings using the "+=" operator.
2.8.1. huge memory saver
2.8.2. huge speed improvement
2.8.3. can be joined before output