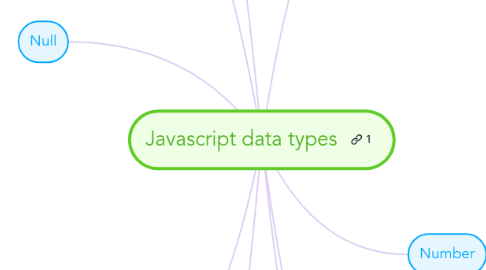
1. Array - Special type of Object
1.1. Not supported for named indexed ex: arr = new Array(); arr['name'] = "Abc"; arr['sex'] = "male"; ---> arr.length not support, ...
1.2. Avoid used to new Array(), should using []
1.3. valueOf() & toString is same : convert value of array to String
1.4. join( separator ) : join value or array to string with separator
1.5. pop() : remove the last element of array -> return "poped out"
1.6. push() : add new element at the end of array -> return new array
1.7. shift() : remove the first element of array
1.8. unshift() : add the new element at the first of array -> return new array length
1.9. delete(indexed) : delete element at position in array and set value is undefined
1.10. splice( positionAdded, totalElementReplace, [newElementAdded,...] )
1.11. sort() & reverse() : sort method cannot used to sort number array. sort( function(a,b){ return a - b; } ) : order number array - [b - a] will order with DESC
1.12. concat([array,...])
1.13. slice([position,...]) : slice element with position from array and return new array
2. Object everything in javascript is Object
3. Null
4. Date
4.1. Date() : string date time
4.2. new Date(year, month, day, hour, minute, second, milisecond) : Note - month count from 0 to 11
4.2.1. toString() : convert Date object to String
4.2.2. toUTCString()
4.2.3. toDateString()
4.2.4. set / getDate() : set / get day of month - from 0 to 31
4.2.5. set / getDay() : set / get day of week - from 0 to 6
4.2.6. set / getMonth() : set / get month - from 0 to 11
4.2.7. set / getFullYear() : set / get year xxxx Optional arguments : yyyy , mm, dd
4.2.8. set / getHour()
4.2.9. set / getMinute()
4.2.10. set / getSecond()
4.2.11. set / getTime() : return number time milisecond from 1/1/1970
4.3. Date.parse() : parse string to Date object new Date( Date.parse() )
4.4. Compare two Date with Date Object
5. Function
5.1. Function declarations
5.2. Function expression
6. Number
6.1. Avoid using new Number() to declare a variable.
6.2. Javascript not has type number( int, double, float, tinyint, ... )
6.3. Infinity
6.4. IsNaN() : is not a number???
6.5. toString() : convert number to string
6.6. toExponential() : convert number to exponential number
6.7. toFixed() : convert corectly number decimal
6.8. toPrecision() : convert number decimal with length
6.9. Operator + String variable ~ convert string to number
6.10. Math
6.10.1. round() : decimal number to nearly interger number
6.10.2. ceil() : up decimal number to nearly interger number
6.10.3. floor() : down decimal number to nearly interger number
7. String
7.1. Avoid using new String() to declare a variable.
7.2. charCodeAt() : return a unicode character
7.3. charAt() : return a value
7.4. Regular Expression
7.4.1. search()
7.4.1.1. return Position
7.4.2. replace()
7.4.2.1. return new String replaced
7.4.3. test()
7.4.3.1. return True / False
7.4.4. match()
7.4.4.1. return Array of string is match
7.4.5. exec()
7.4.5.1. return a string is found