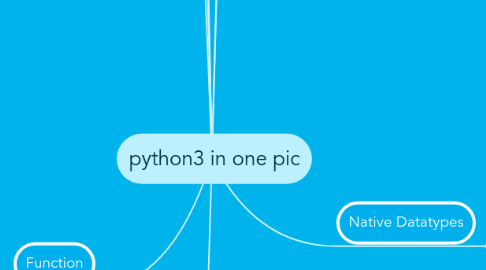
1. Standard Libraries
2. Pythonic
3. Module
3.1. Package
3.1.1. """ MyModule/ |--SubModuleOne/ |--__init__.py |--smo.py # smo.py def run(): print("Running MyModule.SubModuleOne.smo!") """ from MyModule.SubModule import smo smo.run() # Running MyModule.SubModuleOne.smo!
3.2. Search Path
3.2.1. 1. current directory 2. echo $PYTHONPATH 3. sys.path
3.3. Import
3.3.1. import os print(os.name) # posix from sys import version_info as PY_VERSION print("VERSON: {}.{}".format(PY_VERSION.major, PY_VERSION.minor)) # VERSON: 3.5 from math import * print(pi) # 3.141592653589793
4. Class (OOP)
4.1. Override
4.1.1. class Animal: """This is an Animal""" def __init__(self, can_fly = False): self.can_fly = can_fly def fly(self): if self.can_fly: print("I CAN fly!") else: print("I can not fly!") class Bird: """This is a Bird""" def fly(self): print("I'm flying high!") bird = Bird() bird.fly() # I'm flying high!
4.2. Inheritance
4.2.1. class Animal: """This is an Animal""" def __init__(self, can_fly = False): self.can_fly = can_fly def fly(self): if self.can_fly: print("I CAN fly!") else: print("I can not fly!") class Dog(Animal): """This is a Dog""" def bark(self): print("Woof!") d = Dog() d.fly() # I can not fly! d.bark() # Woof!
4.3. Instance
4.3.1. class Animal: pass class Human: pass a = Animal() h = Human() print(isinstance(a, Animal)) # True print(isinstance(h, Animal)) # False
4.4. __init__ & self
4.4.1. class Animal: """This is an Animal""" def __init__(self, can_fly = False): print("Calling __init__() when instantiation!") self.can_fly = can_fly def fly(self): if self.can_fly: print("I CAN fly!") else: print("I can not fly!") a = Animal() # Calling __init__() when instantiation! a.fly() # I can not fly! b = Animal(can_fly = True) # Calling __init__() when instantiation! b.fly() # I CAN fly!
4.5. Class
4.5.1. class Animal: """This is an Animal""" def fly(_): print("I can fly!") a = Animal() a.fly() # I can fly! print(a.__doc__) # This is an Animal
5. Function
5.1. Arguments
5.1.1. Decorator
5.1.1.1. def log(f): def wrapper(): print("Hey log~") f() print("Bye log~") return wrapper @log def fa(): print("This is fa!") # Equal to... def fb(): print("This is fb!") fb = log(fb) fa() print("*"*10) fb()
5.1.1.1.1. # Hey log~ # This is fa! # Bye log~ # ********** # Hey log~ # This is fb! # Bye log~
5.1.2. Lambda
5.1.2.1. pairs = [(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')] pairs.sort(key=lambda pair: pair[1]) print(pairs) "[(4, 'four'), (1, 'one'), (3, 'three'), (2, 'two')]" pairs.sort(key=lambda pair: pair[0]) print(pairs) "[(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')]"
5.1.3. arbitrary arguments
5.1.3.1. def f(*args, **kargs): print("args ", args) print("kargs ", kargs) print("FP: {} & Scripts: {}".format(kargs.get("fp"), "/".join(args))) f("Python", "Javascript", ms = "C++", fp = "Haskell") # args ('Python', ‘Javascript’) # kargs {'ms': 'C++', 'fp': 'Haskell'} # FP: Haskell and Scripts: Python/Javascript
5.1.3.2. def f(*args, con = " & "): print(isinstance(args, tuple)) print("Hello", con.join(args)) f("Python", "C", "C++", con = "/") # True # "Hello Python/C/C++"
5.1.4. keyword arguments
5.1.4.1. def f(v, l = "Python"): """return '$v, $l'""" return "{}, {}!".format(v, l) print(f("Hello")) # "Hello, Python!" print(f("Bye", "C/C++")) # "Bye, C/C++!"
5.1.5. default arguments
5.1.5.1. def f(name = "World"): """return 'Hello, $name'""" return "Hello, {}!".format(name) print(f()) # 'Hello, World!' print(f("Python")) # 'Hello, Python!'
5.2. Definition
5.2.1. def f(): """return 'Hello, World!'""" return "Hello, World!" print(f()) # "Hello, World!" print(f.__doc__) # "return 'Hello, World!'"
6. Flow Control
6.1. Comprehensions
6.1.1. Dict
6.1.1.1. ls = {s: len(s) for s in ["Python", "Javascript", "Golang"]} print(ls) # {'Python': 6, 'Javascript': 10, 'Golang': 6} sl = {v: k for k, v in ls.items()} print(sl) # {10: 'Javascript', 6: 'Golang'}
6.1.2. Set
6.1.2.1. s = {2 * x for x in range(10) if x ** 2 > 3} print(s) # {4, 6, 8, 10, 12, 14, 16, 18} pairs = set([(x, y) for x in range(2) for y in range(2)]) print(pairs) # {(0, 1), (1, 0), (0, 0), (1, 1)}
6.1.3. List
6.1.3.1. pairs = [(x, y) for x in range(2) for y in range(2)] print(pairs) # [(0, 0), (0, 1), (1, 0), (1, 1)]
6.1.3.2. s = [2 * x for x in range(10) if x ** 2 > 3] print(s) #[4, 6, 8, 10, 12, 14, 16, 18]
6.2. Loop
6.2.1. Iterators & Generators
6.2.1.1. def reverse(data): for index in range(len(data)-1, -1, -1): yield data[index] nohtyp = reverse("Python") print(nohtyp) # <generator object reverse at 0x1029539e8> for i in nohtyp: print(i) # n # o # h # t # y # P
6.2.1.2. python = iter("Python") print(python) # <str_iterator object at 0x10293f8d0> for i in python: print(i) # P # y # t # h # o # n
6.2.2. break / continue
6.2.2.1. for n in range(2, 10): if n % 2 == 0: print("Found an even number ", n) continue if n > 5: print("GT 5!") break
6.2.3. While
6.2.3.1. prod = 1 i = 1 while i < 10: prod = prod * i i += 1 print(prod)
6.2.4. For
6.2.4.1. for i in "Hello": print(i)
6.3. If
6.3.1. import sys if sys.version_info.major < 3: print("Version 2.X") elif sys.version_info.major > 3: print("Future") else: print("Version 3.X")
7. import this
7.1. """ The Zen of Python, by Tim Peters Beautiful is better than ugly. Explicit is better than implicit. Simple is better than complex. Complex is better than complicated. Flat is better than nested. Sparse is better than dense. Readability counts. Special cases aren't special enough to break the rules. Although practicality beats purity. Errors should never pass silently. Unless explicitly silenced. In the face of ambiguity, refuse the temptation to guess. There should be one-- and preferably only one --obvious way to do it. Although that way may not be obvious at first unless you're Dutch. Now is better than never. Although never is often better than *right* now. If the implementation is hard to explain, it's a bad idea. If the implementation is easy to explain, it may be a good idea. Namespaces are one honking great idea -- let's do more of those! """
8. Syntax Roles
8.1. Case-sensitive
8.1.1. a = 1 A = 2 print(a is not A) # True
8.2. Comments
8.2.1. # Comments will be ignored
8.3. Code blocks are defined by their indentation
8.3.1. Use 4 spaces per indentation level
9. Native Datatypes
9.1. Number
9.1.1. integer
9.1.1.1. a = 1 b = 0x10 # 16 print(type(a)) # <class 'int'>
9.1.2. float
9.1.2.1. c = 1.2 d = .5 # 0.5 g = .314e1 # 3.14 print(type(g)) # <class 'float'>
9.1.3. complex
9.1.3.1. e = 1+2j f = complex(1, 2) print(type(e)) # <class 'complex'> print(f == e) # True
9.1.4. Operators
9.1.4.1. + - * / % **
9.1.4.2. ## Operators: + - * / ** // % print(1 + 1) # 2 print(2 - 2) # 0 print(3 * 3) # 9 print(5 / 4) # 1.25 print(2 ** 10) # 1024 print(5 // 4) # 1 print(5 % 4) # 1
9.1.5. Casting
9.1.5.1. # Integer/String -> Float
9.1.5.1.1. print(float.__doc__)
9.1.5.1.2. print(float(3)) # 3.0 print(3 / 1) # 3.0 print(float("3.14")) # 3.14
9.1.5.2. # Float/String -> Integer
9.1.5.2.1. print(int.__doc__)
9.1.5.2.2. print(int(3.14)) # 3 print(int("3", base = 10)) # 3 print(int("1010", base = 2)) # 10 print(int("0b1010", base = 0)) # 10
9.2. String
9.2.1. s1 = ‘:dog:\n’ s2 = "Dogge's home" s3 = """ Hello, Dogge! """ print(type(s1)) # <class 'str'> print("%s, %s, %s" % (s1, s2, s3)) # :dog: # , Dogge's home, # Hello, # Dogge!
9.2.2. Length
9.2.2.1. print(len(s1)) # 2
9.2.3. Slicing
9.2.3.1. s = 'study and practice' print('{0}:{1}'.format(s[:5], s[-8:])) # study:practice
9.2.4. Operator
9.2.4.1. +
9.2.4.2. print("abc" + "." + "xyz") # "abc.xyz"
9.2.5. Casting
9.2.5.1. print(str.__doc__)
9.2.5.1.1. """ str(object='') -> str str(bytes_or_buffer[, encoding[, errors]]) -> str """
9.2.5.2. print(str(3.14)) # "3.14" print(str(3)) # "3" print(str([1,2,3])) # "[1,2,3]" print(str((1,2,3))) # "(1,2,3)" print(str({1,2,3})) # "{1,2,3}" print(str({'python': '*.py', 'javascript': '*.js'})) "{'python': '*.py', 'javascript': '*.js'}"
9.3. Byte
9.3.1. list of ascii character
9.3.2. # 0-255/x00-xff
9.3.2.1. byt = b'abc' print(type(byt)) # <class 'bytes'> print(byt[0] == 'a')# False print(byt[0] == 97) # True
9.3.3. Length
9.3.3.1. print(len(byt)) # 3
9.4. Boolean
9.4.1. True
9.4.2. False
9.4.3. print(type(True)) # <class 'bool'>
9.5. None
9.5.1. print(None is None) # True
9.5.2. print(type(None)) # <class 'NoneType'>
9.6. List
9.6.1. l = ['python', 3, 'in', 'one'] print(type(l)) # <class 'list'>
9.6.2. Length
9.6.2.1. print(len(l)) # 4
9.6.3. Slicing
9.6.3.1. print(l[0]) # 'python' print(l[-1]) # 'one' print(l[1:-1]) # [3, 'in']
9.6.4. Alter
9.6.4.1. l.append('pic') # None # l == ['python', 3, 'in', 'one', 'pic'] l.insert(2, '.4.1') # None # l == ['python', 3, '.4.1', 'in', 'one', 'pic'] l.extend(['!', '!']) # l == ['python', 3, '.4.1', 'in', 'one', 'pic', '!', '!'] print(l.pop()) # '!' # l == ['python', 3, '.4.1', 'in', 'one', 'pic', '!'] print(l.pop(2)) # '.4.1' # l == ['python', 3, 'in', 'one', 'pic', '!'] l.remove("in") # l == ['python', 3, 'one', 'pic', '!'] del l[2] # l == ['python', 3, 'pic', '!']
9.6.5. Index
9.6.5.1. print(l.index('pic')) # 2
9.7. Tuple
9.7.1. Immutable list
9.7.2. tp = (1, 2, 3, [4, 5]) print(type(tp)) # <class 'tuple'> ## Length print(len(tp)) # 4 print(tp[2]) # 3 tp[3][1] = 6 print(tp) # (1, 2, 3, [4, 6]) ## Single element tp = (1, ) # Not tp = (1)
9.7.3. assign multiple values
9.7.3.1. v = (3, 2, 'a') (c, b, a) = v print(a, b, c) # a 2 3
9.8. Set
9.8.1. st = {'s', 'e', 'T'} print(type(st)) # <class 'set'> ## Length print(len(st)) # 3 ## Empty st = set() print(len(st)) # 0 st = {} print(type(st)) # <class 'dict'>
9.8.2. Alter
9.8.2.1. st = set(['s', 'e', 'T']) st.add('t') # st == {'s', 'e', 't', 'T'} st.add('t') # st == {'s', 'e', 't', 'T'} st.update(['!', '!']) # st == {'s', 'e', 't', 'T', '!'} st.discard('t') # st == {'T', '!', 's', 'e'} # No Error st.remove('T') # st == {'s', 'e', '!'} # KeyError st.pop() # 's' # st == {'e'} st.clear() # st == set()
9.9. Dict
9.9.1. dic = {} print(type(dic)) # <class 'dict'> dic = {'k1': 'v1', 'k2': 'v2'} ## Length print(len(dic)) # 2
9.9.2. print(dic['k2']) # 'v2' print(dic.get('k1')) # 'v1' print(dic.get('k3', 'v0')) # 'v0' dic['k2'] = 'v3' print(dic) # {'k1': 'v1', 'k2': 'v3'} print('k2' in dic) # True print('v1' in dic) # False