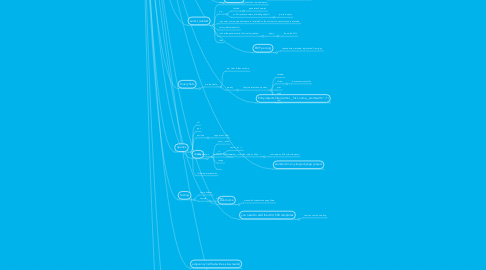
1. uses HTTP properly
2. Entry.objects.filter(author__first_name__startswith="J")
3. adjacency list (fast writes, slow reads)
4. stand-alne
4.1. manual documentation page there
5. select_related
5.1. django abstracts the db but is sensitive to performance
5.2. e.g.
5.2.1. Untitled
5.2.1.1. generates 2 queries
5.2.2. e = Entry.objects.select_related().get(pk=1)
5.2.2.1. pre-join query
5.3. in general, your page performance is correlated to the number of queries that gets executed
5.4. select_related(depth=2)
5.5. look at the generated sql from select_related
5.5.1. .query
5.5.1.1. shows the SQL
5.6. filter
6. EXIF parsing
6.1. reads photo metadata (eg location) from jpg's
7. base models
8. standard in any large django project
9. you need to add the 404, 500 templates
9.1. test can't work on debug
10. are immutable
10.1. qs1 = ..
10.2. qs2 = qs1.filter(...)
10.2.1. doesn't change qs1
11. select_related('field1', 'field2')
12. celery
12.1. Task queue for Python
13. you can limit the select_related to save memory taken by select_related
13.1. which fields
13.2. to what depth
14. About
14.1. "Django is a high-level Python web framework that encourages rapid development ddd clean pragmatic design"
14.2. dddd
14.3. Came from newspaper environment - very short dead-lines
14.3.1. Boss usually came back from lunch & requested stuff by EOD
14.4. Favour agile iterative methodology
14.5. To balance "clean" & "pragmatic"- Django guides you how to do things the right way
15. Install
15.1. quick way
15.1.1. easy_install django
15.1.2. http://bit.ly/dsetup
15.1.2.1. distributed setup
15.2. best way
15.2.1. virtualenv
16. models
16.1. inspectdb
16.1.1. mgmt command to start with existing db
16.2. always add related_name
17. admin
17.1. syntax for customization
17.1.1. Untitled
17.2. Original motivation
17.2.1. Allow content editors to start entering data while developers develop the application
17.3. list_editable
18. templates
18.1. filters
18.1.1. can be piped
18.2. tags
18.2.1. length
18.2.2. regroup
18.3. inheritance
18.3.1. extends must be 1st line
18.3.2. the more blocks the better
18.3.3. if you're duplicating code, you're missing a block
18.3.4. block.super
19. queries
19.1. all
19.2. get
19.3. exclude
19.3.1. opposite of filter
19.4. field lookups
19.4.1. exact, iexact
19.4.2. in
19.4.2.1. name__in=(...)
19.4.2.2. author__in=Author.objects.filter(...)
19.4.2.2.1. will create an SQL with sub-query
19.4.3. range
19.4.4. ...
19.5. following relationships
20. Query Sets
20.1. are chainable
20.1.1. e.g., filter & then exclude
20.1.2. are lazy
20.1.2.1. they're evaluated only when
20.1.2.1.1. iterated
20.1.2.1.2. slicing
20.1.2.1.3. print
20.1.2.1.4. len()
20.1.2.1.5. list()
21. eco-system
21.1. djangopackages
21.2. selected apps
21.2.1. django-extensions
21.2.1.1. many manage.py extensions
21.2.1.1.1. graph_models, passwd, runserver_plus, show_urls, shell_plus
21.2.1.2. custom fields
21.2.2. django-treebeard
21.2.3. Supports Whoosh & Solr & recently also ...
21.2.3.1. Whoosh is python
21.2.4. django-photologue
21.2.4.1. on-the-fly photo resizing & effects
21.2.4.2. enhanced image field & base classes
21.2.4.3. Bulk image upload
21.2.4.4. Photos, galleries
21.2.4.5. tree-like data structures
21.2.4.5.1. materialized path
21.2.4.5.2. nested sets (slow writes, fast reads)
21.2.5. easy-thumbnails
21.2.6. django-registration
21.2.7. django-taggit
21.2.7.1. just add to the model
21.2.7.1.1. tags = TaggableManager()
21.2.8. haystack
21.2.8.1. full-text indexing & search
21.2.8.2. Automatic update of indexed models
21.2.8.2.1. Automatic 2-way sync
21.2.8.3. Query via strings or QuerySet-like interface
21.2.8.4. Support for advanced back-end operations
21.2.9. piston
21.2.9.1. RESTful API framework
21.2.9.2. either
21.2.9.2.1. model-linked
21.2.9.3. pluggable auth
21.2.9.4. Validation, throttling, streaming
21.2.9.5. there's a utility that updates the fixtures when you're changing data
21.2.10. south
21.2.10.1. migration tool
21.2.10.2. that's the 1st thing Jacob installs in a new projects
21.3. bigger apps
21.3.1. pinax
21.3.1.1. social apps
21.3.2. satchmo
21.3.2.1. ecommerce apps
22. sample app
22.1. metric
22.1.1. app for recording stats
22.1.2. invoked in views & save method of models
22.2. tasks
22.2.1. uses decorator
22.3. api
22.3.1. /api/v1
22.3.2. using piston
22.4. iterative test-driven cycles
22.4.1. rapid iterations
22.4.1.1. whats the smallest thing we can build in a day
22.4.1.2. then whats the next thing we can add
22.4.1.3. & so on
22.4.2. feedback as soon as possible
22.4.3. at any given moment, this works
23. testing
23.1. dump fixtures
23.2. sample
23.2.1. Untitled