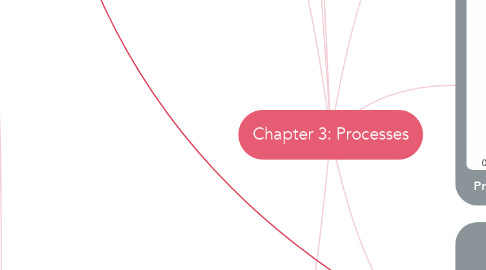
1. Process State
1.1. Process changes state as it executes
1.2. New
1.2.1. Process is being created
1.3. Ready
1.3.1. Waiting to be assigned to processor
1.4. Running
1.5. Waiting
1.6. Terminated
2. Process Scheduling
2.1. Goal: Maximize CPU usage
2.2. Process Scheduler
2.2.1. Short-term scheduler / CPU scheduler
2.2.1.1. selects which process should be executed next on CPU
2.2.1.2. Invoked frequently (milliseconds)
2.2.2. Long-term scheduler / Job Scheduler
2.2.2.1. selects which processes should be brought into ready queue
2.2.2.2. Invoked infrequently (seconds, minutes)
2.2.2.3. Controls degree of multiprogramming
2.3. Scheduling Queues
2.3.1. Job Queue
2.3.1.1. All processes in the system
2.3.2. Ready Queue
2.3.2.1. All processes in main memory waiting to execute
2.3.3. Device Queues
2.3.3.1. Processes waiting for a specific I/O device
2.3.4. Processes migrate among queues
2.4. Context Switch
2.4.1. When switching processes, system must save state of current process and load saved state of the new one
2.4.2. Switch time is pure overhead and should be minimized (don't overcomplicate PCBs!)
3. Operations on Processes
3.1. Process Creation
3.1.1. Parent process creates child processes, forming a tree
3.1.1.1. Resource sharing options
3.1.1.1.1. P & C share all resources
3.1.1.1.2. C share subset of P's resources
3.1.1.1.3. P & C share no resources
3.1.1.2. Execution options
3.1.1.2.1. P & C execute concurrently
3.1.1.2.2. P waits for C to terminate
3.1.2. Process identified and managed through PID
3.2. Process Termination
3.2.1. Returns status data to parent
3.2.2. Process asks for deletion with exit( ) syscall
3.2.3. Parent aborts children with abort( ) syscall
3.2.3.1. Children no longer needed
3.2.3.2. Child is exceeing allocated resources
3.2.3.3. Parent is terminating
3.2.4. Some OSes don't allow children with dead parents to exist :(
3.2.4.1. Cascading Termination
4. Interprocess Communication
4.1. Processes can be cooperating
4.2. Shared Memory
4.2.1. Area of memory is reserved for processes
4.2.2. Communication is under control of the user processes
4.2.3. Issue: synchronization!
4.3. Message Passing
4.3.1. OS provides mechanisms for processes to communicate and synchronize their actions
4.3.1.1. send(message)
4.3.1.2. receive(message)
4.3.2. Processes need to establish a Communication Link
4.3.2.1. Logical
4.3.2.1.1. Direct/Indirect
4.3.2.1.2. Synchronous/Asynchronous
4.3.2.1.3. Automatic/Explicit Buffering
4.4. Communication in Client-Server Systems
4.4.1. Sockets
4.4.1.1. one endpoint of a two-way communication link between two programs running on a network
4.4.1.2. Concatenation of IP and port
4.4.1.3. ports below 1024 are used for standard services
4.4.2. Remote Procedure Calls
4.4.2.1. abstracts procedure calls between processes over a network (?)
4.4.2.2. uses ports for service differentiation
4.4.2.3. Stubs
4.4.2.3.1. client-side stub locates server and marshalls parameters
4.4.2.3.2. server-side stub receives parameters and executed procedure
4.4.3. Pipes
4.4.3.1. Ordinary Pipes
4.4.3.1.1. cannot be accessed from outside the process that created it
4.4.3.1.2. Typically from parent to child
4.4.3.1.3. One way
4.4.3.2. Named Pipes
4.4.3.2.1. Can be accessed by other processes
4.4.3.2.2. Two way
5. Process
5.1. A program in execution, executed in sequential form
5.2. either
5.2.1. I/O bound process
5.2.1.1. spends more time doing I/O than computations
5.2.1.2. many short CPU bursts
5.2.2. CPU-bound process
5.2.2.1. Frequent computations
5.2.2.2. few long CPU bursts
6. Process in Memory
6.1. Stack
6.2. Heap
6.2.1. Memory dynamically allocated during runtime
6.3. Data Section
6.3.1. Global variables
6.4. Text Section
6.4.1. Contains Program code
6.5. PC and Registers
7. Process Control Block (PCB)
7.1. Stores information associated with each process
7.2. Process State (running, waiting...)
7.3. Process number (ID)
7.4. Program Counter
7.5. CPU Registers
7.5.1. content of all process-centric registers