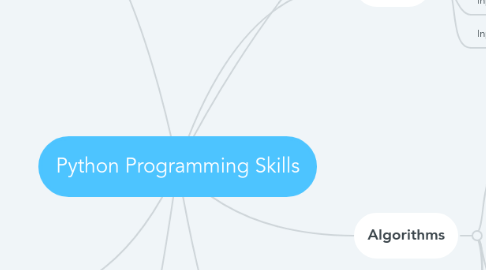
1. GUI
1.1. Draw shapes
1.2. Make a screen
1.3. Place images
1.4. Take input from keyboard
1.4.1. Key presses as events
1.4.2. Text input
1.5. Take input from mouse
1.5.1. Button Presses
1.5.1.1. Establishing which button
1.5.2. Movement
1.5.3. Hover
2. Error Trapping
2.1. Try Except
3. Conditions
3.1. Branching
3.1.1. If statement basic
3.1.2. If else
3.1.3. Multiway
3.1.4. Nested if
3.2. Branching in loops
3.3. Loops
3.3.1. While loop
3.3.1.1. infinite loops
3.3.1.2. Counter conditions
3.3.1.3. text comparision
3.3.2. For loop
3.3.2.1. Loops for number of times
3.3.2.2. Loop through string
3.3.2.3. Loop through other iterators
3.3.3. Nested loops
3.3.3.1. Traverse grid
3.3.3.2. Basic sorts
3.4. Comparisions
3.4.1. Relational Operators
3.4.2. AND, OR, NOT
3.4.3. Combinations of AND, OR, NOT
4. Red Bubbles are extensions
5. STD IO
5.1. Printing to screen
5.1.1. Printing from list
5.2. Output to file
5.3. Input from file
5.4. Input from keyboard
6. Data
6.1. STD Data Types
6.1.1. Converting between data types
6.1.1.1. string to integer
6.1.1.2. string to list
6.1.1.2.1. string to list of integers
6.1.1.3. integer to string
6.1.2. Strings
6.1.2.1. String manipulation
6.1.2.1.1. Concatenation
6.1.2.1.2. Slicing
6.1.2.1.3. Case conversion
6.1.2.1.4. Length
6.1.2.1.5. Replace
6.1.3. Integers
6.1.4. Floats
6.1.5. Boolean
6.2. Complex Data Types
6.2.1. List
6.2.1.1. list comprehensions
6.2.2. Array
6.2.3. Dictionary
6.2.3.1. Building from STD input
6.2.3.2. Reading from a file
6.2.4. Class
6.2.4.1. Array of records
6.2.5. Tree
6.3. Creating Variables
6.3.1. Declaring
6.3.2. Overwriting
6.3.3. Comparisons
7. Algorithms
7.1. Searches
7.1.1. Linear Search
7.1.1.1. Find Minimum
7.1.1.2. Find Maximum
7.1.2. Binary Search
7.1.3. DFS
7.1.4. BFS
7.2. Dynamic Programming
7.3. Complexity
7.4. Functional Programming
7.5. Recursion
8. Functions
8.1. defining
8.2. arguments/parameters
8.3. returning
8.3.1. capturing return values