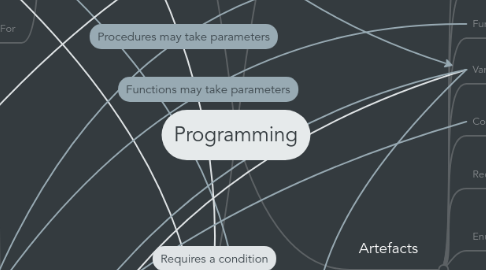
1. Concepts
1.1. Sequence
1.1.1. Programs are compiled and read in sequence (from top to bottom)
1.2. Reflection
1.3. Repetition
1.3.1. Programs need to be able repeat functions, statements and expressions.
1.4. Type
1.4.1. The values used in programs are set to a certain type.
1.5. Value
1.5.1. Values may be stored and used within programs.
2. Artefacts
2.1. Program
2.1.1. Initializes the program.
2.1.1.1. Is defined and accessible by a unique name known as an 'Identifier'
2.2. Procedure
2.2.1. A procedure is a sequence of statements and/or expressions which does not return a value.
2.3. Function
2.3.1. A function is a sequence of statements and/or expressions which returns a value.
2.4. Variable
2.4.1. A variable is an accessible and modifiable value which is stored by the program.
2.5. Constant
2.5.1. A constant is an accessible value which is stored by the program. Constants are not modifiable but are named with an identifier.
2.6. Record
2.6.1. A record is a structure of variables grouped under a single type.
2.7. Enumeration
2.7.1. A list of available options for a type which are assigned to a type.
2.7.2. These options are associated with a number.
2.8. Pointer
2.8.1. A pointer is a variable that unlike most variables does not store a value but the "address" of another variable in a program.
2.8.2. Similar expressions and arithmetic may be used on a pointer in order to update the stored variable.
2.8.3. Pointers are superior to variables in terms of:
2.8.3.1. Efficiency
2.8.3.1.1. As opposed to transferring a large variable through parameters, transferring a pointer has the same effect but with much less storage and processing required.
2.8.3.2. Dynamic
2.8.3.2.1. Pointers allow for dynamic operations on arrays
2.9. Array
2.9.1. An array is collection of variables.
2.9.1.1. The values in an array are referenced using an index starting at 0.
2.9.1.2. They may be dynamic in size or static depending on how they're declared and the language which is being used.
3. Action
3.1. Assignment
3.1.1. Assignment statements assign a value to a variable
3.2. Function Call
3.2.1. A statement used to run the code of a function.
3.2.2. Includes the necessary parameters for the function.
3.3. Procedure Call
3.3.1. Like a function call, a procedure call runs the code of a procedure.
3.3.2. Includes the necessary parameters for the procedure.
3.4. If
3.4.1. A type of statement which checks for a condition.
3.4.2. If true, the code within the block is run.
3.4.3. If false, the statement may :
3.4.3.1. Look for an else or an elseif statement to check for another condition
3.4.3.2. Jump the statement entirely.
3.5. Case
3.5.1. Checks a variable's value and runs code depending on which "case" has been presented.
3.6. Repeat
3.6.1. Repeats a series of statements until the condition presented is false.
3.7. While
3.7.1. Like a repeat loop, the while loop repeats a series of statements until the condition presented is false.
3.8. For
3.8.1. A for loop repeats a series of statements until a condition presented is false. This is achieved by iterating a variable through a range of values until the condition achieved is false.
4. Terminology
4.1. Statement
4.1.1. In computing terms, a statement is an instruction.
4.1.1.1. A program is a sequence of statements
4.2. Iteration
4.2.1. Iteration is the process of repeating a process / statements until a certain outcome is reached.
4.3. Expression
4.3.1. An expression is code that calculates and returns a value.
4.3.1.1. Is a type of statement
4.4. Identifier
4.4.1. An identifier is a unique name given to artefacts in a program.
4.5. Parameter
4.5.1. Parameters are variables and values which passed into functions and procedures which may be used in its statements.
4.5.2. Allows the functions and procedures to be more dynamic.
4.6. Global Variable
4.6.1. Global variables are variables declared within the entire scope of the program.
4.6.2. Are considered bad due to the fact :
4.6.2.1. The variables are less secure as they are on the most "public" level of the program
4.6.2.2. Variables in a global scope is inefficient as they may go unused considering the fact they aren't tied to any specific function or procedure.
4.6.2.3. Global variables break the natural structure of a program
4.6.3. Constants are a much better way of defining values on a global scope.
4.7. Local Variable
4.7.1. Local variables are variables declared within the scope of a procedure or a function.
4.7.2. They are only accessible within the method it was initialized in by default, other procedures/functions may use these variables through parameters.
4.8. Condition
4.8.1. A condition is an expression which returns a true or false value.
4.8.2. This is used in conjunction with various actions to change the structure and flow of a program.