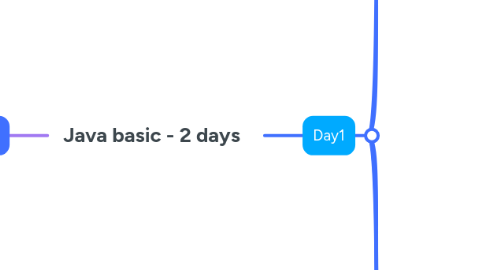
1. Day2
1.1. Four principle of OOP
1.1.1. Encapsulation
1.1.1.1. make sure that "sensitive" data is hidden from users
1.1.1.2. declare class variables/attributes as private
1.1.1.3. provide public get and set methods to access and update the value of a private variable
1.1.2. Abstraction
1.1.2.1. is the process of hiding certain details and showing only essential information to the user.
1.1.2.2. is a non-access modifier
1.1.2.3. access through "extend" keyword
1.1.3. Inheritance
1.1.3.1. An interface is a completely "abstract class" that is used to group related methods with empty bodies
1.1.3.2. access through "implements" keyword
1.1.3.3. Interface methods are by default abstract and public
1.1.3.4. Interface attributes are by default public, static and final
1.1.3.5. Finally, Java doesn't support multi inheritance, but can implement from interfaces
1.1.4. Polymorphism
1.1.4.1. Polymorphism uses those methods to perform different tasks
1.1.4.2. it occurs when we have many classes that are related to each other by inheritance.
1.2. Overloading
1.2.1. is used to increase the readability of the program
1.2.2. is performed within class
1.2.3. parameter must be different
1.2.4. is the example of compile time polymorphism
1.2.5. Return type can be same or different
1.3. Overriding
1.3.1. is used to provide the specific implementation of the method that is already provided by its super class
1.3.2. occurs in two classes that have IS-A (inheritance) relationship.
1.3.3. parameter must be same
1.3.4. is the example of run time polymorphism
1.3.5. Return type must be same or covariant
1.4. Parcelable
1.4.1. Parcelable thường được sử dụng để gửi dữ liệu (dạng Object) giữa các activity với nhau thông qua Bunble gửi cùng Intent
1.4.2. sử dụng đối tượng được cài đặt Parcelable sẽ chạy nhanh hơn đáng kể so với việc sử dụng Serializable
1.4.3. lớp này đuợc sử dụng rõ ràng về quá trình đọc ghi tuần tự thay vì sử dụng sự ánh xạ (reflection) để suy ra nó do đó mã đã được tối ưu hóa và tạo ra ít đối tuợng rác hơn cho mục đích này
2. Day1
2.1. Super and this
2.1.1. Super
2.1.1.1. is a reference variable which is used to refer immediate parent class object
2.1.1.2. Usage
2.1.1.2.1. used to refer immediate parent class instance variable
2.1.1.2.2. used to invoke immediate parent class method
2.1.1.2.3. super() can be used to invoke immediate parent class constructor
2.1.2. This
2.1.2.1. this is a reference variable that refers to the current object
2.1.2.2. Usage
2.1.2.2.1. used to refer current class instance variable
2.1.2.2.2. used to invoke current class method (implicitly)
2.1.2.2.3. this() can be used to invoke current class constructor
2.1.2.2.4. passed as an argument in the method call
2.1.2.2.5. passed as argument in the constructor call
2.1.2.2.6. used to return the current class instance from the method
2.2. Modifier
2.2.1. Access Modifier
2.2.1.1. controls the access level
2.2.1.2. for classes
2.2.1.2.1. public
2.2.1.2.2. default
2.2.1.3. for attributes, methods and constructors
2.2.1.3.1. public
2.2.1.3.2. private
2.2.1.3.3. protected
2.2.1.3.4. default
2.2.2. Non-AM
2.2.2.1. do not control access level, but provides other functionality
2.2.2.2. class
2.2.2.2.1. final
2.2.2.2.2. abstract
2.2.2.3. attributes and methods
2.2.2.3.1. final
2.2.2.3.2. abstract
2.2.2.3.3. static
2.2.2.3.4. transient
2.2.2.3.5. synchronized
2.2.2.3.6. volatile
2.3. Constructor
2.3.1. is a special method, that is used to initialize object
2.3.2. is called when the object of class is created
2.3.3. cant return type, such as: void
2.3.4. All classes have constructors, if you dont creat, Java will create one for you. But not able to set initial values for object attributes.
2.3.5. can also take parameters, which is used to initialize attributes
2.4. Class & Object
2.4.1. Class is a basic unit of Java
2.4.2. Object can be created from the class
2.4.3. You can acess another class or object in other file
2.5. final
2.5.1. cant change the value of variables: final int myNum = 15; same "val" of kotlin
2.6. Data type
2.6.1. byte (8 bit), short, int, long
2.6.2. char, String
2.6.3. float, double
2.6.4. boolean
2.7. Variables
2.7.1. String ("String")
2.7.2. int
2.7.3. boolean
2.7.4. float
2.7.5. char
2.8. Creating var
2.8.1. type nameVar = value;
2.9. local var
2.9.1. declare in the body of method
2.9.1.1. void method() { int local = 16; }
2.9.2. bonus
2.9.2.1. static var
2.9.2.1.1. declare in the class, outside the method, constructor, after "static" keyword
2.9.2.2. intance var
2.9.2.2.1. declare in the class, outside the method, constructor