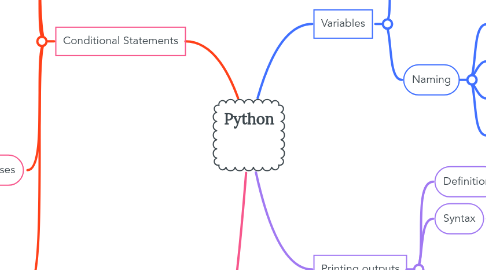
1. Conditional Statements
1.1. Definition
1.1.1. The if statement is how you perform decision-making. It allows for conditional execution of a statement or group of statements based on the value of an expression.
1.2. Syntax
1.2.1. if <expr>: <statement>
1.2.1.1. if keyword
1.2.1.2. <statement> is a valid Python statement, which must be indented
1.2.1.3. <expr> the condition is an expression evaluated in a Boolean context that has a TRUE or FALSE value.
1.2.1.3.1. Equals: a == b
1.2.1.3.2. Not Equals: a != b
1.2.1.3.3. Less than: a < b
1.2.1.3.4. Less than or equal to: a <= b
1.2.1.3.5. Greater than: a > b
1.2.1.3.6. Greater than or equal to: a >= b
1.2.1.4. colon : is required
1.2.1.5. indentation: (whitespace at the beginning of a line) to define scope in the code.
1.3. The else and elif Clauses
1.3.1. else
1.3.1.1. The else keyword catches anything which isn't caught by the preceding condition of (if).
1.3.2. elif
1.3.2.1. The elif keyword is pythons way of saying "if the previous conditions were not true, then try this condition".
1.4. Possible uses:
1.4.1. if only
1.4.2. if / else
1.4.3. if / elif / else
1.4.4. if / elif / elif
2. Getting inputs
2.1. Definition
2.1.1. The input( ) function allows user input.
2.2. Syntax
2.2.1. Variable = Type ( Input ("String, representing a default message before the input"))
2.3. Example
2.3.1. String input
2.3.1.1. x = input("What is your name?")
2.3.2. Integer input
2.3.2.1. y = int(input("How old are you?"))
2.3.3. Float input
2.3.3.1. z = float(input("What is your average?"))
3. Variables
3.1. Data Types
3.1.1. Integer
3.1.1.1. x = 2
3.1.2. Float
3.1.2.1. y = 4.3
3.1.3. String
3.1.3.1. z = "Computer"
3.1.3.1.1. declaring a variable with string data type should be inside quotations
3.2. Naming
3.2.1. A variable name must start with a letter or the underscore character.
3.2.1.1. a
3.2.2. A variable name cannot start with a number.
3.2.2.1. 3students
3.2.3. A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
3.2.3.1. name1 or name2
3.2.4. Variable names are case-sensitive
3.2.4.1. age, Age, or AGE are different variables
4. Printing outputs
4.1. Definition
4.1.1. The print( ) function prints the given object to the standard output device (screen) or to the text stream file.
4.2. Syntax
4.2.1. print( )
4.3. Example
4.3.1. printing text
4.3.1.1. print("Hello Everyone!"
4.3.2. printing number
4.3.2.1. print(55)
4.3.3. printing variables
4.3.3.1. x=2 print(x)
4.3.4. joining multiple of output in one line (one print command)
4.3.4.1. x= 4 print("The value of x", x)