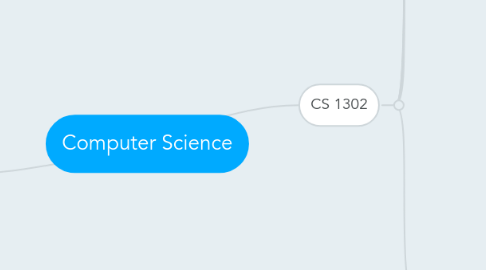
1. CS 1301
1.1. Chap 1
1.1.1. What are the various components of a computer (6 in total)
1.1.1.1. CPU
1.1.1.1.1. This is where all computation happens
1.1.1.2. Memory
1.1.1.2.1. Where temporary items are stored
1.1.1.3. Storage Device
1.1.1.3.1. Hard Drive - Is still there even if you shut down the computer
1.1.1.4. Communication Devices
1.1.1.4.1. Allows the computer to communicate with the outside world - USB
1.1.1.5. Input Devices
1.1.1.5.1. Allows information to be put in - Keyboard
1.1.1.6. Out Put Devices
1.1.1.6.1. Send out information - Monitor
1.1.2. What is Dot Pitch?
1.1.2.1. Dot Pitch is the space between each pixle
1.1.3. What are the 3 different languages
1.1.3.1. Machien Language
1.1.3.1.1. Written in Binary
1.1.3.2. Assembly Language
1.1.3.2.1. In short commands such as ADD,R1,R2
1.1.3.3. High level Language
1.1.3.3.1. English like language
1.1.4. What does a OS do?
1.1.4.1. Operating Systems manage all the computer's activities.
1.1.5. What makes Java special
1.1.5.1. It is machien indpendent and is in nearly every electronic device
1.1.6. What is Syntax and Semantic errors
1.1.6.1. Syntax errors - errors that break the laws of coding such as forgetting a semicolon at the end of a statment
1.1.6.2. Semantic errors - logical errors don't give the right result
1.1.7. What are the 3 main types of errors
1.1.7.1. Compile Errors - a mistake that breaks the code like forgetting a semicolon
1.1.7.2. Run Time Error - when you break the logic of a computer such as dividing by 0
1.1.7.3. Logical Error - wrong out put
1.1.8. What are the different types of JDK
1.1.8.1. J2SE
1.1.8.1.1. Java Standard Edition - client side program
1.1.8.2. J2EE
1.1.8.2.1. Java Enterprise Edition - server side program
1.1.8.3. J2ME
1.1.8.3.1. Java Micro Edition - handheld
1.2. Chap 2
1.2.1. What makes variables unique?
1.2.1.1. The program identifies an object by its name
1.2.2. What are the 8 primitive data type?
1.2.2.1. Byte - Memory space
1.2.2.2. Char - One Charater
1.2.2.3. Short - 16 bits Smallest no decimal
1.2.2.4. Int - 32 bits no decimal
1.2.2.5. Long - 64 bits Largest no decimal
1.2.2.6. Float - 32 bits decimal number
1.2.2.7. Double - 64 bits decimal number
1.2.2.8. Boolean - 16 bit yes or no
1.2.3. What is Widening conversions?
1.2.3.1. When you widen a conversion you go from a smaller data type to a larger one such as short to int
1.2.4. What is Narrowing conversion?
1.2.4.1. You narrow a conversion by going from a larger data type to a smaller one, often a loss of information will occur such as double to int.
1.2.5. What are the 3 main types of data type conversions?
1.2.5.1. Promotion (temporary conversion)
1.2.5.1.1. When a variable is temporarily converted for example multiplying a double with a int the int variable will be temporarily promoted to a double variable
1.2.5.2. Assignment conversion
1.2.5.2.1. When you assign one data type to another for example setting a double variable to the value of a int variable
1.2.5.3. Casting Conversion (explicit conversion)
1.2.5.3.1. When you tell Java explicitly to convert from one data type to another even if there is a loss of data
1.3. Chap 3
1.3.1. How does java read your programs
1.3.1.1. Java reads all programs from top to bottom 1 statment at a time.
1.3.2. What are the three main kinds of statements?
1.3.2.1. if
1.3.2.1.1. Allows one statment
1.3.2.1.2. if this is true do this
1.3.2.2. if-else
1.3.2.2.1. Allows two statments
1.3.2.2.2. if this is true do this else do this
1.3.2.3. switch
1.3.2.3.1. Allows multiple statments
1.3.2.3.2. after each case you need to put a break; in order for java to exit the statment
1.3.2.3.3. if this is true to this and this until you have reached the end of the statement or have reached a break; point
1.3.3. What are the different boolean operators?
1.3.3.1. ==
1.3.3.1.1. Equal too
1.3.3.2. !=
1.3.3.2.1. Not equal too
1.3.3.3. <
1.3.3.3.1. less than
1.3.3.4. >
1.3.3.4.1. greater than
1.3.3.5. <=
1.3.3.5.1. less than or equal too
1.3.3.6. >=
1.3.3.6.1. greater that or equal too
1.3.4. What are the different logical operators
1.3.4.1. !
1.3.4.1.1. Not
1.3.4.2. &&
1.3.4.2.1. And
1.3.4.3. | |
1.3.4.3.1. Or
1.3.4.4. ^
1.3.4.4.1. The two booleans should be different
1.4. Chap 4
1.5. Chap 5
1.6. Chap 6
1.6.1. What is a method?
1.6.1.1. A method is a chunk of code you can call on to do certain tasks over and over again.
1.6.2. What is a methods signature
1.6.2.1. A method's signature is its name and its paremeters.
1.6.3. What are the benefits of using a method?
1.6.3.1. It promotes information hiding so that it is harder to find loopholes in your code. Also help organizing and keeping the code is a readable fashion.
1.6.4. What makes void methods special?
1.6.4.1. They do not need to return any values
1.6.5. What is a runtime stack?
1.6.5.1. A runtime stack is how the computer thinks and what it stores in its temporary memory. It will execute the main method and if it comes across another method inside of the main it will create some space for that method and then clear the space when it is not needed.
1.6.6. What are overloaded methods?
1.6.6.1. These are methods that have two instances. Usually one with parameters and one without. One you run the method the computer will do its best to find out which method you wanted to invoke. But if it comes across a instances where it cannot decide which one you meant to run it will come back with an error.
1.6.7. What is an Ambiguous Invocation?
1.6.7.1. An ambiguous invocation is where the java cannot decide which version of an overloaded method you mean to run. For example if you say: methodName(1,2); and you have one version with integers and one version of the method as doubles the program will fail and come back saying that it did not know which version of the method you wanted it to run.
1.6.8. What is a Scope of a Variable?
1.6.8.1. The scope of a variable is how far is that variable known. For example when using a counter of i in a if statement that version of i only is their within that if statement. It cannot be referenced or re called at a point out side of that loop
1.7. Chap 7
1.7.1. What is an Array?
1.7.1.1. An array is a collection of data values that are grouped toghether
1.7.2. What are some special characteristics about an array?
1.7.2.1. A array can be changed through a method if run through.
1.7.2.2. A array is only a bunch of pointers that point to its specified data value.
1.7.3. What are some array short cut commands?
1.7.3.1. for (dataType value: arrayName){ System.out.print(value); }
1.7.4. How do you initialize an array?
1.7.4.1. datatype[] arrayName = new datatype [numberOfElements in the array]
1.7.5. What is an anonymous array?
1.7.5.1. An anonymous array is an array that does not have a name for example in this command : printArray(int[] {3,4,5,6});
1.7.6. What are the different ways the computer can search through an array?
1.7.6.1. There are two ways, linear and binary.
1.7.6.1.1. A LINEAR search method is by searching for through all data values in the array from first to last
1.7.6.1.2. A BINARY search method is dome by taking the first last and middle of the array, sees which one its is closest to and then crosses out the ones it cant be.
1.7.6.1.3. BINARY SEARCHES CAN ONLY BE DONE BY ARRAYS THAT ARE IN ORDER FROM LEAST TO GREATEST
1.8. Chap 8
1.8.1. What are multidimensional arrays?
1.8.1.1. Multidimensional arrays are arrays that have arrays in side of arrays.
1.8.2. How do you initialize a multidimensional array?
1.8.2.1. dataType[][] arrName = new dataType[1st dimension lentght][2nd dimension length];
1.8.3. How do you reference a certain element in an array?
1.8.3.1. arryName [place in 1st dimension][place in 1st dimension1]
1.8.4. What are ragged arrays?
1.8.4.1. 2 dimensional arrays that have different array lengths for each 2nd dimension.
1.9. Chap 9
1.9.1. What is an object?
1.9.1.1. An object is datatype that is not a primitive datatype for example String is not a datatype
1.9.2. What is unique about an object?
1.9.2.1. An object has an identity state and behaviors.
1.9.3. What is the state of an object?
1.9.3.1. The state of an object is the set of data fields.
1.9.4. What is a class?
1.9.4.1. It is a template for an object
1.9.5. What is the main method?
1.9.5.1. The main method is the part of the program that is executed.
1.9.6. Why do we use private variables?
1.9.6.1. We use private variables to hide information from the users and prevent them from changing them.
1.9.6.2. It also makes it harder for hackers to find loopholes in your program.
2. CS 1302
2.1. Ch 11
2.1.1. Inheritance
2.1.2. Polymorphism
2.1.2.1. Definition: A super type can reference a subtype
2.1.2.2. A super type can refer to a subtype
2.2. Ch 13
2.2.1. Abstract
2.2.1.1. Definition : An abstract class is a class that is declared abstract. Abstract class cannot be instantiated (cannot create a object from it). But they can have subclasses
2.2.1.1.1. An abstract method is a method that is defined but not implemented in the class. The subclasses must implement these abstract methods or call them selves abstract classes.
2.2.1.2. UML : Abstract is italizised
2.2.1.3. Abstract method : is a method that is declared without an implementation (without braces, and followed by a semicolon)
2.2.1.3.1. example : abstract void moveTo(double deltaX, double deltaY);
2.2.1.4. If a class has a Abstract method the class must be declared Abstract
2.2.1.5. * If the super class is abstract and has abstract methods, the subclass must implement all the abstract method from the super class
2.2.1.5.1. If the subclass does not implement all abstract methods from the superclass. The subclass must be abstract
2.2.1.6. Abstract can be used as a datatype
2.2.2. Interface
2.2.2.1. An interface is a class that contains only abstract methods and constant variables.
2.2.2.2. Interfaces cannot create instance of a object much like and abstract class
2.2.2.3. Interface can be used as a datatype
2.2.2.4. All data feilds and methods have a preset datatype
2.2.2.4.1. All data fields are public static final
2.2.2.4.2. All methods are public abstract
2.2.3. When to use a abstract vs interface class and how to use classes
2.2.3.1. Abstract
2.2.3.1.1. A "strong is a relationship"
2.2.3.2. Interface
2.2.3.2.1. A "weak relationship"
2.2.3.3. Each class should be well defined and should handle one object at a time.
2.2.3.3.1. For example when creating a list of people at a University, you may have a parent class of people and two subclasses, Student and Staff. These classes are well defined and hold only one object.
2.2.3.4. A class should have a well definied responsiblity
2.3. Ch 14
2.3.1. FX Basic Structure
2.3.1.1. Stage
2.3.1.1.1. Scene
2.3.1.1.2. This can be displayed as a UI
2.3.2. JavaFX
2.3.2.1. JavaFX had replaced AWT and Swing, because it was better to create Internet application
2.3.2.1.1. AWT(Abstract window tool kit)
2.3.3. GUI - Graphical User Interface
2.3.4. Binding Poperty
2.3.4.1. Allows a target object to be bound to a source object
2.3.4.1.1. If the source changed the target will change with it
2.4. Ch 15
2.4.1. Button
2.4.1.1. When clicked java recognizes different button
2.4.2. Event Object
2.4.2.1. This could be a button or something clicked on
2.4.2.2. Event Handler
2.4.2.2.1. These can handle events such as mouse clicks or a key being pressed and tells what java should do in certain situations
2.4.2.2.2. Action Event
2.5. Ch 16
2.5.1. Nodes
2.5.1.1. Labels
2.6. Ch 17
2.7. Ch 18
2.7.1. Recursion
2.7.1.1. Essentially methods that call themselves
2.7.1.2. Factorial is a good example from the power points of a method that calls itself
2.7.1.3. Recursions must have a base case or they will never stop
2.7.2. H trees
2.7.2.1. These kind of diagrams show complex pathways to a file
2.8. Ch19
2.8.1. Generics
2.8.1.1. A generic class is a a class that can take more than one data type. An example of this is ArrayLists<>
2.8.1.1.1. ArrayLists<> takes in multiple datatypes, although non of the datatypes are the primitive ones.
2.8.1.2. Some major pros for generics are that any kind of errors that would occur with a incompatible object would occur during the build instead of run time which makes it easier to find the error
2.9. Ch 20
2.9.1. Data Structures
2.9.1.1. Collection
2.9.1.1.1. List
2.9.1.1.2. Qeues
2.9.1.1.3. Stack
2.9.1.1.4. Priority Queue
2.9.1.1.5. Set
2.9.1.1.6. Iterators
2.9.1.2. Maps