React.js Learning Roadmap
作者:Code King
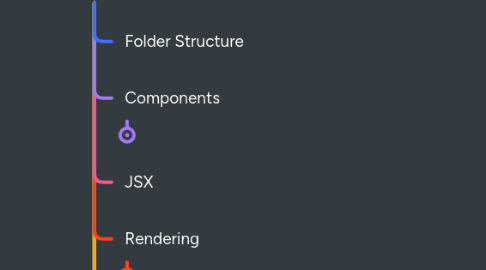
1. Folder Structure
2. Components
2.1. Class Components
2.2. Functional Components
3. JSX
4. Rendering
4.1. Conditional Rendering
5. Props
6. State
7. Hooks
7.1. useState
7.2. useEffect
8. Handling Event
9. Lists and Keys
10. Components Lifecycle
10.1. Mounting
10.2. Updating
10.3. Unmounting
11. Router
11.1. React Router
12. Context
13. High Order Components
14. Refs
15. Render Props
16. Portals
17. Hooks
17.1. useContext
17.2. useCallback
17.3. useMemo
17.4. useRef
17.5. useReducer
17.6. Custom Hook
17.7. Advance Hooks
17.7.1. useDebugValue
17.7.2. useDeferredValue
17.7.3. useImperativeHandle
17.7.4. useId
17.7.5. useLayoutEffect
17.7.6. useInsertionEffect
17.7.7. useTransition
17.7.8. useSyncExternalStore
18. Error Boundaries
19. Https Requests
19.1. fetch
19.2. GET
19.3. PUT
19.4. POST
19.5. DELETE
19.6. axios
20. Styling
20.1. CSS
20.2. Material UI
20.3. Chakra UI
20.4. Bootstrap
20.5. Tailwind
21. Forms
21.1. Components
21.1.1. Uncontrolled components
21.1.2. Controlled components
21.2. Forms
21.2.1. React Form
21.2.2. Formik