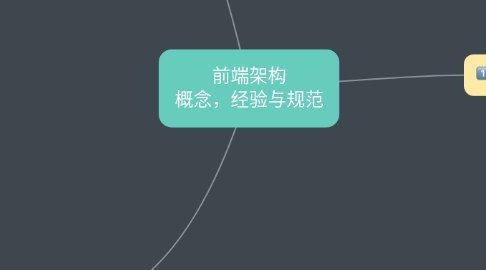
1. 规范
1.1. 目录结构
1.1.1. Motivation
1.2. 模块组织
1.3. 开发流程
1.3.1. React/Redux/Redux-saga
1.3.1.1. Write action/action creator
1.3.1.2. Write saga to dispatch/handle async operation
1.3.1.3. Write component reducer to return new state
1.3.1.4. Determine the shape of your state/data - root reducer
1.3.1.5. Write component that dispatches your action
1.3.1.6. Connect component to store to subscribe to changes
2. 经验
2.1. 最佳实践
2.1.1. React
2.1.1.1. Container Component VS Presentational Component
2.1.1.1.1. Presentational Component
2.1.1.1.2. Container Component
2.1.1.1.3. when to extract P-component ?
2.1.1.2. Single Component VS Many Components
2.1.1.2.1. extract component
2.1.2. Redux
2.1.2.1. mapDispatchToProps
2.1.2.1.1. Shorthand Notation
2.1.2.2. mapStateToProps
2.1.2.2.1. shorter ES6 property notation
2.1.2.3. override dispatch
2.1.2.3.1. benifit
2.1.2.3.2. caution
2.1.2.4. One Reducer One Concern
2.1.2.4.1. example
2.1.2.5. action creator extraction
2.1.2.5.1. why? because more than one component trigger the same action
2.1.2.5.2. don't underestimate make acton creator alone. because of team work. it make all team member know what kinds of actions the component can dispatch
2.1.2.5.3. action creator has behavior and need to be tested.
2.1.2.6. why initial state
2.1.2.6.1. get persisted state from local storage
2.1.2.6.2. do NOT put default state in initial state, leave it in the default value in reducer
2.1.2.7. state persistency
2.1.2.7.1. data should be persisted
2.1.2.7.2. ui state should NOT be persisted
2.1.2.8. middlewares
2.1.2.8.1. put custom behavior before action reaches reducers
2.1.2.9. thunk
2.1.2.9.1. why
2.1.3. React-Redux
2.1.3.1. connect
2.1.3.1.1. what it do?
2.1.3.1.2. it is a curried function
2.1.3.1.3. props to P-component
2.1.3.1.4. both 1st and 2nd argument could be set to null
2.1.4. ES6 in React/Redux
2.1.4.1. concise method notation
2.1.4.1.1. in mapDispatchToProps
2.1.4.2. object expression
2.1.4.2.1. in action creator
2.1.4.2.2. in mapStateToProps
2.1.4.2.3. in mapDispatchToProps
2.1.4.3. shorthand notation
2.1.4.3.1. in mapDispatchToProps
2.1.4.4. namespace import [ import * as XXs form 'XX' ]
2.1.4.4.1. state selector defined together with state reducer
2.1.4.5. object spread [ Not in ES 6 ]
2.1.4.5.1. use plugin
2.1.4.6. computed property syntax [ Not in ES 6 ]
2.1.4.6.1. use plugin
2.1.4.7. array spread
2.1.5. React-Router
2.1.5.1. router props as 'params' prop injected into component
2.1.5.2. A thought: router's filter params sometimes acts as single source of truth rather than state only. In other words both Redux and React-Router are source of truth.
2.1.5.3. withRouter [ Starting from V 3.0 ] working with Redux
2.1.5.3.1. Inject params of route into component as params prop
2.1.6. Common Practices/Conventions
2.1.6.1. colocate selectors with reducers
2.1.6.1.1. move the data selector into reducer where both determine the internal structure of data
2.1.6.1.2. selector share the same state in arguments as reducer
2.1.6.2. state as database
2.1.6.2.1. object with id get out of sync when defined in different array
2.1.6.3. define plugin
2.1.6.3.1. ensure some plugin in dev not included in product
2.1.6.4. colocate reducer creators with reducers or selectors
2.1.6.5. race conditions
2.1.6.5.1. check async action state before dispatch action
2.1.6.6. catch in Promise
2.1.6.6.1. do not catch at the end of promise but as second argument to each then
2.1.7. Redux-Saga
2.1.7.1. testing
2.1.7.1.1. the Generator will yield plain Objects containing instruction
2.1.7.1.2. test plain objects rather than instruction execution
2.1.7.2. effects
2.1.7.2.1. what ?
2.1.7.2.2. definition
2.1.7.3. investigate
2.1.7.3.1. difference between fork and yield multiple takeLatest effect
2.1.7.4. running task in parallel
2.1.7.4.1. yield an array of effects
2.2. 开发技巧
2.3. 技术坑
3. 概念
3.1. 编程基础
3.1.1. Functional Programming
3.1.1.1. Immutability
3.1.1.1.1. array
3.1.1.1.2. object
3.1.1.2. Pure Functions
3.1.1.3. Data Transformations
3.1.1.4. Higher Order Functions
3.1.1.5. Recursion
3.1.1.6. Currying
3.1.1.6.1. the mental model is that a function with serval arguments that are applied as they become available
3.1.2. ES6
3.1.2.1. Generator
3.1.2.1.1. A function can return multiple values
3.1.2.1.2. Iterator + Observer
3.1.2.1.3. async + await in ES7 = Generator + Promise
3.1.2.2. let & const
3.1.2.3. Importing Modules
3.1.2.4. Destructuring
3.1.2.5. Function Shorthand
3.1.2.6. Fat-Arrow Functions
3.1.2.7. Default Parameters
3.1.2.8. String Interpolation
3.1.2.9. Promise
3.1.3. Observable APIs
3.1.3.1. DOM Events
3.1.3.2. Webscokets
3.1.3.3. Server-sent Events
3.1.3.4. Node Streams
3.1.3.5. Service Workers
3.1.3.6. jQuery Events
3.1.3.7. XMLHttpRequest
3.1.3.8. setInterval/setTimeout
3.1.4. Isomorphic Application
3.1.4.1. benifits
3.1.4.1.1. Code Sharing
3.1.4.1.2. Abundance of frameworks
3.1.4.1.3. Performance and rendering speed
3.1.4.1.4. Progressive enhancement
3.1.4.1.5. Improved accessibility
3.1.4.1.6. SEO-friendly
3.2. 技术栈
3.2.1. Redux
3.2.1.1. State Tree
3.2.1.1.1. Read Only
3.2.1.1.2. Mutate State
3.2.1.1.3. Thinking in Redux
3.2.1.2. Action
3.2.1.2.1. Plain JS Object
3.2.1.2.2. Type Prop
3.2.1.3. Reducer
3.2.1.3.1. What is
3.2.1.3.2. Reducer Composition
3.2.1.4. Store
3.2.1.4.1. three jobs
3.2.1.4.2. impl from scratch
3.2.1.5. + React
3.2.1.5.1. How each component get update when state change ?