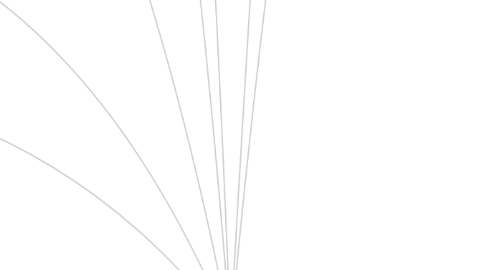
1. Few Other Links
1.1. Companies using C Programming Language
1.1.1. https://docs.google.com/spreadsheet/ccc?key=0AvPic5Th8MOmdFdHc05mRHQwQmFNazk0T0N1RDRmcUE&usp=sharing#gid=0
1.2. NPTEL Videos
1.2.1. http://nptel.ac.in/courses/106104074/
1.2.2. http://ekalavya.it.iitb.ac.in/eVideos/CS101/content/preview.html
1.3. NPTEL Slides
1.4. Virtal Lab
1.5. Spoken Tutorials
2. History of C
2.1. ALGOL
2.2. BCPL
2.3. B
2.4. Traditional C
2.5. ANSI C
2.6. ANSI/ ISO C
2.7. Dennis Retchie
3. mode
3.1. r - read only
3.2. w - write only
3.3. a - appending data
4. Files
4.1. Open
4.1.1. FILE *fp; fp=fopen("filename","mode");
4.2. Close
4.2.1. fclose(file_pointer);
4.3. Input
4.3.1. putc(c, fp1);
4.3.2. c=getc(fp2);
4.4. Access
4.4.1. position
4.4.1.1. n=ftell(fp);
4.4.2. reset position
4.4.2.1. rewind(fp);
4.4.3. moving
4.4.3.1. fseek(fp,offset,position)
4.5. Output
4.5.1. fprintf(fp,"control string",list);
4.5.2. fscanf(fp,"control string",list);
5. Structures / Unions
5.1. define a structure
5.1.1. struct name; { data type member1; datatype member2;..........};
5.2. initialization members
5.2.1. main(){ struct name{ datatype mem1; datatype mem2;.....}; struct name mem1={constant.....}; struct name mem2={constant......}; }
5.2.2. struct name{ datatype mem1; datatype mem2;.....};mem1={constants.......}; main(){ struct name mem2={constants.....};...............}
6. Pointers
6.1. declaring
6.1.1. datatype *pt_name
6.2. initialize
6.2.1. p=&quantity
6.3. accessing
6.3.1. int quantity *p,n quantity=179; p=&quantity; n=*p
7. Functions
7.1. declaration
7.1.1. type name (type var1.......)
7.2. calling
7.2.1. main() { name(var1........); }
7.2.2. Call By Value - actual value of parameters
7.2.3. Call By Reference - pointers
7.3. types
7.3.1. with no arguments no return type
7.3.2. with no arguments one return type
7.3.3. with arguments no return type
7.3.4. with arguments multiple return type
7.4. definition
7.4.1. syntax
7.4.1.1. type name(type var1........) { local declaration; executable part; return(var); }
7.5. Recursion
8. Strings
8.1. Concatenation
8.1.1. strcat(str1,str2); output is str1str2
8.2. Comparison
8.2.1. strcmp(str,str2); ASCII str1 - ASCII str2, +ve if str1<str2, -ve if str1 > str2
8.3. Copying
8.3.1. strcpy(str1,str2); outputs str1="str2";
8.4. Length
8.4.1. n=strlen(str);
8.5. Substr and many more string functions are available...
8.6. Built in function in ctype.h
8.6.1. isalpha(x)
8.6.2. isdigit(x)
8.6.3. isalphanum(x)
8.6.4. ispunct(x)
8.6.5. islower(x)
9. Arrays
9.1. Dimensions
9.1.1. One
9.1.1.1. Declaration
9.1.1.1.1. type var_name[size];
9.1.1.2. Initialization
9.1.1.2.1. type name[size]={list of values};
9.1.2. Two
9.1.2.1. Declaration
9.1.2.1.1. type name[row_size] [col_size];
9.1.2.2. Initialization
9.1.2.2.1. type name[row_size] [col_size]={{row list},{col list}};
9.1.3. Multi
9.1.3.1. Declaration
9.1.3.1.1. type name[s1][s2][s3]....[sn];
9.1.3.2. Initialization
9.1.3.2.1. type name[s1][s2][s3]...[s3]={{list s1},{list s2}, {list s3}, {list s4}.....{list sn}};
9.2. Character Array (String)
9.2.1. Declaring / Initialising
9.2.1.1. char name[size];
9.2.2. Reading
9.2.2.1. scanf("%s",name);
9.2.3. Writing
9.2.3.1. printf("%s",name);
10. Design on Paper
10.1. Ms. Divya Khanure
11. Datatypes
11.1. Built-In Type
11.1.1. Integer
11.1.1.1. Signed
11.1.1.1.1. int
11.1.1.1.2. short int
11.1.1.1.3. long int
11.1.1.2. Unsigned
11.1.1.2.1. long int
11.1.1.2.2. short int
11.1.1.2.3. int
11.1.2. Float
11.1.2.1. long double
11.1.2.1.1. 80bits
11.1.2.2. float
11.1.2.2.1. 32 bits
11.1.3. Character
11.1.3.1. Signed char
11.1.3.1.1. 8bits
11.1.3.2. Unsigned char
11.1.3.2.1. 8bits
11.1.3.3. Char
11.1.4. Double
11.1.4.1. double
11.1.4.1.1. 64bits
11.2. Derived Type
11.2.1. Array
11.2.2. Funtions
11.2.3. Pointer
11.2.4. Reference
11.3. User Defined Types
11.3.1. Structure
11.3.2. Union
11.3.3. Enumeration
12. C- Tokens
12.1. Special Symbols (Delimeters)
12.1.1. ;,!?#$
12.2. Constants & Variables
12.2.1. Numeric Constants
12.2.1.1. int
12.2.1.1.1. %d
12.2.1.2. real
12.2.1.2.1. float
12.2.1.2.2. exponential
12.2.2. Character Constants
12.2.2.1. Single
12.2.2.1.1. %c
12.2.2.2. String
12.2.2.2.1. %s
12.2.2.2.2. Backslash
12.3. Keywords
12.3.1. Basic Building Blocks
12.3.1.1. int
12.3.1.2. if
12.3.1.3. case
12.3.1.4. while
12.3.1.5. struct
12.3.1.6. auto
12.3.1.7. break
12.3.1.8. case
12.3.1.9. do
12.3.1.10. for
12.3.1.11. else
12.3.1.12. continue
12.3.1.13. goto
12.3.1.14. return
12.3.1.15. switch
12.3.1.16. union
12.3.1.17. void
12.3.1.18. float
12.3.1.19. char
12.3.1.20. long
12.3.1.21. int
12.3.1.22. short
12.3.1.23. double
12.3.1.24. sizeof
12.3.1.25. typedef
12.3.1.26. register
12.3.1.27. static
12.3.1.28. const
12.3.1.29. extern
12.3.1.30. define
12.4. Identifiers
12.4.1. Ex: amount, sum etc
12.5. Strings
12.5.1. Ex" :SDMCET", "123"
12.6. Operators
12.6.1. Arithmetic
12.6.1.1. +
12.6.1.2. -
12.6.1.3. /
12.6.1.4. %
12.6.1.5. *
12.6.2. Logical
12.6.2.1. ||
12.6.2.2. &&
12.6.2.3. !
12.6.3. Assignment
12.6.3.1. +=
12.6.3.2. -=
12.6.3.3. *=
12.6.3.4. /=
12.6.3.5. %=
12.6.4. Conditional
12.6.4.1. exp1 ? exp2 : exp3;
12.6.5. Special
12.6.5.1. sizeof
12.6.5.2. , [comma]
12.6.6. Increment / Decrement
12.6.6.1. post
12.6.6.1.1. ++
12.6.6.2. pre
12.6.6.2.1. --
12.6.7. Bitwise
12.6.7.1. &
12.6.7.2. /
12.6.7.3. <<
12.6.7.4. >>
12.6.7.5. ~
12.6.8. Relational
12.6.8.1. <
12.6.8.2. <=
12.6.8.3. >
12.6.8.4. >=
12.6.8.5. ==
12.6.8.6. !=
13. Expressions
13.1. Arithmetic
13.1.1. precedence
13.1.1.1. high
13.1.1.1.1. *
13.1.1.1.2. /
13.1.1.1.3. %
13.1.1.2. low
13.1.1.2.1. +
13.1.1.2.2. -
13.1.2. example
13.1.2.1. a*b-c ->a*b-c
13.1.2.2. 3*x*x-x ->3x^2-x
13.2. Conversions
13.2.1. automatic type
13.2.1.1. follows the hierarchy of size of the operands
13.2.2. casting a value
13.2.2.1. (type name)exp;
13.3. Mathematical Functions
13.3.1. #include<math.h> cc filename -lm
13.3.1.1. example
13.3.1.1.1. exp(x)
13.3.1.1.2. fabs(x)
13.3.1.1.3. sqrt(x)
14. Input/Output
14.1. Formatted
14.1.1. Input
14.1.1.1. scanf("control string", &arg1,&arg2..);
14.1.2. Output
14.1.2.1. printf("control string", arg1,arg2..);
14.2. Unformatted
14.2.1. putchar
14.2.1.1. putchar(variable_name);
14.2.2. getchar
15. Control Statements
15.1. if
15.1.1. simple if
15.1.1.1. if(test exp){ body;} statement -x;
15.1.2. if else
15.1.2.1. if(test exp){ true-block}; else {false-block}; statement-x
15.1.3. nested if else
15.1.3.1. if(test exp1) { if(test exp2){ statement1} else {statement2;} else {statement 3}; statement-x;
15.1.4. else if ladder
15.1.4.1. if(condition1) statement1; else if(condition2) statement2; else if.........else if(condition n) statement n; else default statement
15.2. swicth
15.2.1. switch(exp){ case value1:block1; break; case value2:block2; break; ..........default:block; break; } statement-x;
15.3. conditional
15.3.1. conditional exp? exp1:true exp2:false
15.4. goto
16. Loops
16.1. continue (skip)
16.2. for
16.2.1. for(initialize ; test exp ; increment) { body; }
16.3. while
16.3.1. while(test exp) { body; }
16.4. do.. while
16.4.1. do { body ; } while(test exp);