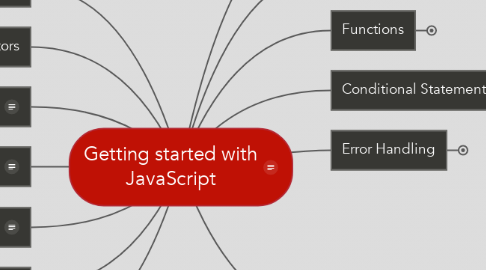
1. Data
1.1. Values
1.1.1. Value Types
1.1.1.1. object
1.1.1.2. Primitive
1.1.1.3. function
1.1.2. Primitive value (datum)
1.1.2.1. undefined
1.1.2.2. null
1.1.2.3. Boolean
1.1.2.4. number
1.1.2.4.1. Nan
1.1.2.4.2. Infinity
1.1.2.4.3. - Infinity
1.1.2.4.4. undefined
1.1.2.5. string
1.1.2.5.1. Can be delimited by either ' or "
1.1.2.5.2. Use backslash \ as escape char.
1.1.2.5.3. \uHHHH where HHHH is a 4-digit hex code.
1.1.2.5.4. Concatenate with +
1.1.3. JavaScript also defines the following built in objects
1.1.3.1. global
1.1.3.2. Object
1.1.3.3. Function
1.1.3.4. Array
1.1.3.4.1. constructing
1.1.3.4.2. Inserting into an existing array.
1.1.3.4.3. Properties
1.1.3.4.4. Methods
1.1.3.5. String
1.1.3.6. Boolean
1.1.3.7. Number
1.1.3.8. Math
1.1.3.9. Date
1.1.3.10. RegEx
1.1.3.11. JSON
1.1.3.12. Several variations of Error
2. Expression
3. Operators
3.1. Binary
3.1.1. Modulo % is a valid operator
3.1.2. Mathematical operators have normal precidence
3.2. Unary
3.2.1. typof
3.2.1.1. typeof "Hello" gives 'string'.
3.2.1.2. typeof 1.2 gives 'number'
3.2.1.3. typeof true gives 'Boolean'
3.3. Logical operators
3.3.1. ==
3.3.2. !=
3.3.3. >
3.3.4. <
3.3.5. !
3.3.6. &&
3.3.7. ||
3.4. Coalescing operators
3.5. Comparison
3.5.1. Equals (==)
3.5.2. Same (===)
4. Statements
5. Variables
5.1. Naming
5.2. Scope
5.2.1. global
5.2.1.1. If you do not use var then the variable will be created implicitly with global scope. THIS IS BAD.
5.2.2. local
6. Reserved Words / Keywords
7. Environment
7.1. In a web application, a new environment is created each time the web page is loaded .
7.2. In a Windows 8 program, an environment is created when the application starts.
8. Functions
8.1. Declaring
8.1.1. function Add(x, y) { ... }
8.1.2. var add = function(x, y) { ... }
8.2. Arguments
8.2.1. Extra arguments are discarded.
8.2.2. Missing arguments are set to 'undefined'
8.3. Browser built-in functions
8.3.1. alert
8.3.2. prompt
8.3.3. confirm
8.4. JavaScript built-in functions
8.4.1. Casting functions
8.4.1.1. Number(object)
8.4.1.2. String(object)
9. Conditional Statements
9.1. Boolean Functions
9.1.1. isNaN(object)
9.2. Flow control
9.2.1. if-else
9.2.2. switch-case
9.3. if (variable) will return true is the variable is not undefined. (NB: if it
9.4. null == undefined will evaluate as true.
9.5. Looping
9.5.1. while(expression) { ... }
9.5.2. for (int i = 0; i < max; i += increment) { ...}
9.5.3. do { ... } while (expression);
10. Error Handling
10.1. try { ... } catch(e) { /* Do something like alert(e.message) */ } finally { ... }
11. The <script> tag
11.1. type="text/javascript" is optional in HTML5
11.2. Attributes
11.2.1. async
11.2.2. defer
11.3. Wrap script code in an HTML comment
12. Accessing the DOM
12.1. NodeList
12.1.1. live NodeList
12.1.1.1. getElementByTagName(tagName)
12.1.1.2. getElemenntsByName(name)
12.1.1.3. getElementsByClass(class)
12.1.2. static NodeList
12.1.2.1. getElementById(id)
12.1.2.2. querySelector(cssSelector)
12.1.2.3. querySelectorAll(cssSelector)
12.2. Navigating the DOM
13. Events
13.1. Capturing and Bubbling
13.2. Subscribing to events
13.2.1. btn.addEventListener(eventNameAsString, callbackMethod, booleanOnCapture)
13.2.2. <a onclick="someMethod();">click</a>
13.2.3. btn.onclick = function(e) { ... };
13.3. Unsubscribing from events
13.3.1. btn.removeEventListener(eventNameAsString, callbackMethod, booleanOnCapture)
13.4. Cancelling propogation
13.4.1. function myEventHandler(e) { e.stopPropagation(); }
13.5. Preventing default operations
13.5.1. function myEventHandler(e) { e.preventDefault(); }
13.6. window Object
13.6.1. afterprint
13.6.2. beforeonload
13.6.3. beforeprint
13.6.4. blur
13.6.5. error
13.6.6. focus
13.6.7. haschange
13.6.8. load
13.6.9. message
13.6.10. offline
13.6.11. pagehide
13.6.12. pageshow
13.6.13. popstate
13.6.14. redo
13.6.15. resize
13.6.16. storage
13.6.17. undo
13.6.18. unload
13.7. Form
13.7.1. blur
13.7.2. change
13.7.3. contextmenu
13.7.4. focus
13.7.5. formchange
13.7.6. forminput
13.7.7. input
13.7.8. invalid
13.7.9. select
13.7.10. submit
13.8. Keyboard
13.8.1. keydown
13.8.2. keypress
13.8.3. keyup
13.9. Mouse
13.9.1. click
13.9.2. dblclick
13.9.3. drag
13.9.4. dragend
13.9.5. drapenter
13.9.6. drapleave
13.9.7. dragstart
13.9.8. drop
13.9.9. mousedown
13.9.10. mousemove
13.9.11. mouseout
13.9.12. mouseover
13.9.13. mouseup
13.9.14. mousewheel
13.9.15. scroll
13.10. Media
13.10.1. abort
13.10.2. canplay
13.10.3. canplaythrough
13.10.4. durationchange
13.10.5. emptied
13.10.6. ended
13.10.7. error
13.10.8. loadeddata
13.10.9. loadedmetadata
13.10.10. pause
13.10.11. play
13.10.12. playing
13.10.13. progress
13.10.14. ratechange
13.10.15. readystatechanged
13.10.16. seeked
13.10.17. seeking
13.10.18. stalled
13.10.19. suspend
13.10.20. timeupdate
13.10.21. waiting