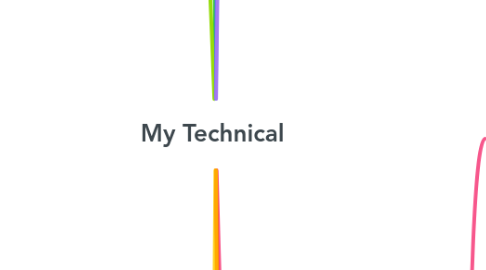
1. NodeJS
2. React Native
2.1. Introduction
2.1.1. What is React Native?
2.1.1.1. React Native is a popular open-source framework developed by Facebook for building mobile applications using JavaScript and React. It enables developers to build native mobile apps for iOS and Android platforms using a single codebase, which significantly speeds up development without compromising on the performance and usability of the apps. With React Native, you write components with JSX, a syntax that combines JavaScript and XML. These components can map to native UI elements like views, text, images, and more.
2.1.2. Why React Native?
2.1.2.1. React Native is a widely popular framework for building native mobile applications using JavaScript and React
2.1.2.2. Code Reusability
2.1.2.3. Familiar React Concepts
2.1.2.4. Native Performance
2.1.2.5. Vast Ecosystem
2.1.2.6. Hot Reloading
2.2. Enviroment Setup
2.2.1. Expo
2.2.1.1. Expo is a framework and a platform that allows you to develop, build, and deploy React Native applications easily and quickly. It simplifies the development process and provides a set of useful tools and services, including its own CLI (Command Line Interface), a managed workflow, and an SDK (Software Development Kit) with pre-built modules for common features.
2.2.1.2. create-expo-app
2.2.1.2.1. is a command line tool that generates a React Native project that works out of the box with Expo. It is the easiest way to get started building a new React Native application.
2.2.1.3. Expo Snack
2.2.1.3.1. Expo Snack is an online playground and development environment for creating and testing React Native projects. With Snack, you can easily edit and preview your code changes directly in your browser or on a mobile device using the Expo Go app. It offers a fast, easy, and convenient way to develop, test, and share your projects without needing to set up a local development environment.
2.2.1.4. Expo Tradeoffs
2.2.1.4.1. Expo is a powerful tool that simplifies the React Native development process, but it has some limitations. Here’s a summary of the tradeoffs you may face when using Expo.
2.2.1.4.2. Limited native modules
2.2.1.4.3. Performance
2.2.1.4.4. App size
2.2.1.4.5. Updates and upgrades
2.2.1.4.6. Ejecting complications
2.2.1.4.7. Limited customizability
2.2.2. React Native CLI
2.2.2.1. Metro Bundler
2.2.2.1.1. Metro Bundler is the default bundler for React Native applications. It’s a JavaScript module bundler that takes all your application code and dependencies, and bundles them together into a single JavaScript file or multiple files (based on platform).
2.2.2.2. React Native CLI is the official command-line interface for building native mobile apps using React Native. This method requires you to manually set up the native development environment and tools needed for iOS and Android app development.
2.3. Development Workflow
2.3.1. Running on Device
2.3.1.1. open -a Simulator
2.3.1.2. Open emulator
2.3.1.2.1. - emulator -list-avds - emulator -avd Pixel_2_API_30
2.3.1.3. npx pod-install ios Nvm list Nvm install Nvm use
2.3.1.4. Reset metro bundler cache
2.3.1.4.1. npx react-native start --reset-cache
2.3.1.5. Remove Android assets cache
2.3.1.5.1. cd android && ./gradlew clean
2.3.2. Debugging
2.3.2.1. In-App Developer Meny
2.3.2.1.1. Android: Cmd + M or Ctrl + M
2.3.2.1.2. iOS: Cmd + D or Ctrl + D
2.3.2.2. Enabling Fast Refresh
2.3.2.3. DevTools
2.3.2.4. LogBox
2.3.2.4.1. LogBox is a new feature added to React Native to improve how logs are displayed and managed in your development environment. It provides better visualization and organization of logs, warnings, and errors, making it easier for developers to address issues in their code.
2.3.2.4.2. Better Error Formatting
2.3.2.4.3. Improved Warnings
2.3.2.4.4. Component Stacks
2.3.2.4.5. Customizable
2.3.2.5. Sourcemaps
2.4. Core Components
2.4.1. Text
2.4.2. TextInput
2.4.3. Button
2.4.4. Image
2.4.5. ImageBackground
2.4.6. Switch
2.4.7. StatusBar
2.4.8. Actiivity Indicator
2.4.9. Modal
2.4.10. Pressable
2.4.11. View
2.4.12. Listings
2.4.12.1. Scroll View
2.4.12.2. ListViews
2.4.12.2.1. FlatList
2.4.12.2.2. SectionList
2.4.12.3. VirtualizedList
2.4.12.4. Refresh Control
2.4.13. Platform Specific Code
2.4.13.1. const styles = StyleSheet.create({ container: { ...Platform.select({ web: { // Web-specific styles }, native: { // Native-specific styles }, }), }, text: { ...Platform.select({ web: { // Web-specific styles }, native: { // Native-specific styles }, }), }, });
2.4.13.2. if (Platform.OS === 'ios') { console.log('Running on iOS'); } else if (Platform.OS === 'android') { console.log('Running on Android'); }
2.4.14. Styling
2.4.14.1. Stylesheets
2.4.14.2. Styled component
2.4.15. Networking
2.4.15.1. Fetch
2.4.15.2. Websockets
2.4.16. Interactions
2.4.16.1. Touchables
2.4.16.1.1. TouchableOpacity
2.4.16.1.2. TouchableHighlight
2.4.16.1.3. TouchableWithoutFeedback
2.4.16.1.4. TouchableNativeFeedback
2.4.16.1.5. TouchableScale
2.4.16.2. Gesture Responder System
2.4.16.2.1. PanResponder
2.4.16.3. Scrolling and Swiping
2.4.16.3.1. ScrollView
2.4.16.3.2. Swipeable
2.4.16.4. Screen Navigation
2.4.16.4.1. React Native Navigation
2.4.16.4.2. React Navigation
2.4.16.5. Animations
2.4.16.5.1. Animated
2.4.16.5.2. LayoutAnimation
2.5. Push Notifications
2.5.1. Firebase Cloud Messaging (FCM)
2.5.2. Apple Push Notification Service (APNs)
2.5.3. OneSignal
2.6. Deep Linking
2.6.1. Deep linking is a technique used in mobile applications that allows you to open a specific screen, content, or functionality within the application using a URL or a custom URL scheme
2.6.2. Universal Links
2.6.2.1. Universal Links (iOS) / App Links (Android): These are HTTPS URLs that allow the user to navigate to a specific screen when the app is installed and fallback to a specified website when the app is not installed.
2.6.3. Custom URL Schemes
2.6.3.1. Unique URLs, like myapp://my-screen, that can open the app directly to a specific screen when clicked.
2.6.4. Handling Deep Links
2.6.4.1. Linking
2.7. Security
2.7.1. Secure Storage
2.7.1.1. Store sensitive data, such as authentication tokens, encryption keys, or user credentials, securely using a storage solution that comes with built-in encryption mechanisms.
2.7.1.1.1. react-native-keychain
2.7.1.1.2. react-native-encrypted-storage
2.7.2. Secure Communication
2.7.2.1. https
2.7.3. Minimize Permissions
2.7.3.1. react-native-permissions
2.7.4. Validate User Input
2.7.4.1. Yup
2.7.5. Keep Dependencies Up to Date
2.7.5.1. npm update npm audit
2.7.6. Authentication
2.7.6.1. JWT
2.7.6.2. OAuth
2.8. Storage
2.8.1. react-native-async-storage
2.8.2. Expo Secure Store
2.8.3. Expo File System
2.8.4. Expo SQLite
2.8.5. Realm
2.8.6. Firebase Realtime Database
2.9. Testing
2.9.1. Jest
2.9.2. Component Tests
2.9.2.1. React Test Renderer
2.9.2.2. React Native Testing Library
2.9.3. E2E Testing
2.9.3.1. Detox
2.9.3.1.1. Detox is an end-to-end testing framework for React Native applications. It enables you to run tests on an actual device or in a simulator/emulator environment. The goal of Detox is to maintain a high level of confidence in your application’s behavior while allowing for quick test runs and easy debugging
2.9.3.2. Appium
2.9.3.2.1. Appium is an open-source test automation framework for mobile devices, targeting native, hybrid, or mobile-web apps for iOS, Android, and Windows platforms. Appium works with multiple programming languages, including JavaScript, Ruby, Python, Java, and C#. Appium uses the WebDriver protocol, which allows you to write tests that can interact with your app through a series of commands. The WebDriver protocol interprets these commands into actions that are then performed on the app.
2.10. Performance
2.10.1. Understand Frame Rates
2.10.1.1. Frame rates represent the number of frames (or images) displayed per second in an animation or video
2.10.1.2. frame rates can be categorized into two types
2.10.1.2.1. Static Frame Rate
2.10.1.2.2. Adaptive Frame Rate
2.10.2. Common Problem Sources
2.10.2.1. Console Logs
2.10.2.1.1. Excessive console logs can lead to decreased performance in React Native, especially in debug mode. In order to avoid this, keep the usage of console logging to a minimum, and clean up unnecessary logs before releasing the app.
2.10.2.2. Images
2.10.2.2.1. Heavy and unoptimized images can lead to performance issues in React Native. To avoid this, use the following techniques:
2.10.2.3. Inline Functions and Styles
2.10.2.3.1. Using inline functions and styles within components can lead to unnecessary re-rendering and performance issues. Instead, define functions and styles outside of the component render method.
2.10.2.4. PureComponent and React.memo
2.10.2.4.1. can lead to performance issues in case they’re updating frequently and causing unnecessary re-renders. Make sure to only use them when appropriate to avoid performance bottlenecks.
2.10.2.5. ListView and FlatList
2.10.2.5.1. When working with large lists in React Native, using ListView instead of FlatList can cause performance issues. Replace ListView with the more performant FlatList or SectionList components.
2.10.2.6. JavaScript Thread Blockage
2.10.2.6.1. Blocking the JavaScript thread with expensive synchronous computations can lead to poor performance. Make sure to handle heavy computations asynchronously or offload them to native modules.
2.10.3. Speeding up Builds
2.10.3.1. https://reactnative.dev/docs/build-speed
2.10.4. Optimizing FlatList Config
2.10.4.1. Set windowSize
2.10.4.1.1. a prop that determines the number of pages rendered above and below the current window. By default, this value is 21.
2.10.4.2. Set removeClippedSubviews
2.10.4.2.1. Enable the removeClippedSubviews prop to unmount components that are off the screen.
2.10.4.3. Set maxToRenderPerBatch
2.10.4.3.1. helps to control the number of items to be rendered per batch. By default, this is set to 10
2.10.4.4. Set initialNumToRender
2.10.4.4.1. initialNumToRender is used to set the number of items to be rendered initially. Setting this value to a reasonable number can help in avoiding blank screens on load.
2.10.4.5. Set getItemLayout
2.10.4.5.1. Using the getItemLayout prop allows you to specify the exact dimensions of each item. This prevents the need for measuring them dynamically, resulting in improved performance
2.10.4.6. Avoid anonymous function on renderItem
2.10.4.6.1. For functional components, move the renderItem function outside of the returned JSX. Also, ensure that it is wrapped in a useCallback hook to prevent it from being recreated each render.
2.10.4.7. https://reactnative.dev/docs/optimizing-flatlist-configuration
2.10.5. RAM Bundles + Inline Requires
2.10.5.1. https://reactnative.dev/docs/ram-bundles-inline-requires
2.10.6. Profiling
2.10.6.1. https://reactnative.dev/docs/profiling
2.11. Using Native Modules
2.11.1. For iOS
2.11.1.1. https://reactnative.dev/docs/native-modules-ios
2.11.2. For Android
2.11.2.1. https://reactnative.dev/docs/native-modules-android
2.12. Publishing Apps
2.12.1. Apple App Store
2.12.2. Google Play Store
2.12.3. Appcenter
2.12.3.1. Install appcenter cli
2.12.3.1.1. Npm install -g appcenter-cli
2.12.3.2. Open autocomplete
2.12.3.2.1. appcenter setup-autocomplete
2.12.3.3. appcenter codepush release-react -a icn-holdings/Flock-iOS -d Production
3. NextJS
3.1. What is NextJS
3.1.1. A framework for building fast & search engine friendly applications
3.1.2. Static Site Generation
3.1.2.1. Render one time and serve them whenever they are needed
3.1.2.1.1. make applicatiom super fast
3.1.3. Server side rendering
3.1.3.1. render components on the server and send content to client
3.1.3.1.1. search-engine friendly
3.1.3.1.2. fast
3.1.3.1.3. make application faster and more search
3.2. Routing and Navigation
3.2.1. create in app folders
3.2.2. page.tsx is index file
3.2.3. using Link to navigate screen for download each page when navigate, don't download all page in the first time
3.2.3.1. 2 request
3.2.3.1.1. for downloading this page
3.2.3.1.2. dont reloading font, css file and a bunch of js files
3.3. Client and Server components
3.3.1. Client side Rendering
3.3.1.1. Large bundles (we have to bundle all our components and send them to the client for rendering => application grows) => the large the bundle the more memory we need on the client to load all these components so this approach is resource heavy
3.3.1.2. Client Components allows you to write interactive UI that can be rendered on the client at request time
3.3.1.3. Resource intensive
3.3.1.4. No SEO
3.3.1.5. Less secure
3.3.1.6. when use
3.3.1.6.1. when we use event listeners (eq: onClick, onChange) in our components when we use hooks like useState(), useReducer(), useEffect(), or other custom hooks that are depending on the state or lifecycle hooks when we use browser-only APIs (eq: window, document) or hooks that use these APIs when you want to use localStorage in your components some very particular cases for data fetching
3.3.2. Server side rendering
3.3.2.1. Smaller bundles
3.3.2.2. Resource efficient
3.3.2.3. SEO
3.3.2.4. More secure
3.3.3. Server component cannot
3.3.3.1. Listen to browser events
3.3.3.2. Access browser APIs
3.3.3.3. Maintain state
3.3.3.4. Use effects
3.3.4. Best practive
3.3.4.1. Use default to server component and use client components only when we absolutely need them
3.4. Data Fetching
3.4.1. Client
3.4.1.1. useState + useEffect and call to BE to ge the data and put to state
3.4.1.2. React Query
3.4.1.3. Time and bundles would get larger because we have to ship more and more components because all the rendering is done on the client, not visible to SEO, and less secure, extra roundtrip to server
3.4.2. Server
3.4.2.1. Resolve all prolems when fetching by Client side
3.4.2.2. has Caching
3.4.2.2.1. storing data somewhere that is faster to access
3.4.2.2.2. Next JS build in data cache
3.5. Static and Dynamic Rendering
3.5.1. Static Renderig
3.5.1.1. Render at build time
4. What is Javascript
4.1. JavaScript is a high-level object-oriented, multi-paradigm programming language
4.1.1. Programming language
4.1.1.1. instruct computer to do things
4.1.1.2. Object-oriented
4.1.1.2.1. Based on objects, for storing most kinds of data
4.1.1.3. Multi-paradigm
4.1.1.3.1. We can use different styles of programming
4.2. Building web applications
4.3. There is nothing you can’t do with JavaScript
4.3.1. Dynamic effects and web applications in the browser
4.3.1.1. ReactJS
4.3.1.2. Angular
4.3.1.3. Vue.js
4.3.2. Web applications on web servers
4.3.2.1. NodeJS
4.3.3. Native mobile applications
4.3.3.1. React Native
4.3.3.2. Ionic
4.3.4. Native desktop applications
5. Basics
5.1. Part 1
5.1.1. Boolean Logic
5.1.1.1. AND
5.1.1.2. OR
5.1.1.3. NOT
5.1.2. Template Literal
5.1.3. History of Javascript
5.1.3.1. ES2015( ES & 6 )
5.1.3.1.1. The buggest update to the language
5.1.3.2. has been annually updating ever since
5.1.3.2.1. principles
5.1.4. Type Coercion
5.1.4.1. the plus operator(+) will always convert into a string
5.1.4.2. Boolean
5.1.4.2.1. Falsey Value
5.1.4.2.2. Values that are not False, yet will become false when we try to convert a Boolean
5.1.5. Type Conversion
5.1.6. Conditionals
5.1.6.1. If / Else Statements
5.1.6.2. Switch Statements
5.1.6.3. Conditional (Ternary) Operator Ternary operator/conditional operator
5.1.6.3.1. the conditional operator allows us to write something similar to an if/else statement but all in one line
5.1.6.3.2. an operator creates a value (value) therefore is an expression (expression)
5.1.6.3.3. the conditional operator is also called the ternary operator because it has three parts. condition, if part, else part
5.1.7. Equality Operator
5.1.7.1. ===
5.1.7.2. ==
5.2. Part 2
5.2.1. strict mode
5.2.1.1. forbids us from doing certain things
5.2.1.2. creates visible errors in the console which would have otherwise failed silently
5.2.2. Function
5.2.2.1. a piece of code you can use over and over again
5.2.2.2. can also receive and return data
5.2.2.2.1. like a machine
5.2.2.3. like a variable but for whole chunks of code
5.2.2.3.1. a variable holds a value
5.2.2.3.2. a func holds one or more lines of complete code
5.2.2.4. the process of using the func is called
5.2.2.4.1. calling the func
5.2.2.4.2. running the func
5.2.2.4.3. invoking the func
5.2.2.5. parameters
5.2.2.5.1. variables that are specific only to this function
5.2.2.5.2. values of the parameters are called argurements
5.2.2.6. types of functions
5.2.2.6.1. function declaration
5.2.2.6.2. function expressions
5.2.2.6.3. arrow function
5.2.2.6.4. three different wats of writing functions, but they all work in a similar way, receive input data, transform data, and output data
5.2.3. Data Structure
5.2.3.1. Arrays
5.2.3.1.1. add elements
5.2.3.1.2. remove elements
5.2.3.1.3. indexOf
5.2.3.1.4. includes method
5.2.3.1.5. use for order data
5.2.3.2. Objects
5.2.3.2.1. in objects we define key-value-pairs
5.2.3.2.2. creating an object using the curly brackets is called the object literal syntax because we are literally writing down the entire object content
5.2.3.2.3. in objects, the order of the elements do not matter when we want to retrieve them
5.2.3.2.4. use for unstructured data
5.2.3.2.5. Object method
5.2.4. Loop
5.2.4.1. for loop
5.2.4.1.1. automates repetitive tasks
5.2.4.1.2. has three parts
5.2.4.2. while loop
5.2.4.2.1. can only specify the confition
5.2.4.2.2. conclusion: the while loop does not have to depend on the counter variable
5.2.4.2.3. use when you do not know how many iterations you will have beforehand
5.3. How to think like a developer
5.3.1. understanding the problem
5.3.1.1. breaking into sub-problems
5.4. JavaScript in the browser: DOM and Events Fundamentals
5.4.1. DOM
5.4.1.1. Document Object Model
5.4.1.2. Structured representation of HTML documents.
5.4.1.3. Allows JavaScript to access html elements and styles to manipulate them
5.4.1.4. in short: the DOM is basically a connection point for html documents and JavaScript code.
5.4.1.5. How it works
5.4.1.5.1. The DOM is created as soon as the html loads and is stored in a tree structure
5.4.1.5.2. The DOM is not part of JavaScript Language, yet it works because DOM and DOM Methods are part of WEB APIs
5.4.2. Handing click events
5.4.2.1. addEventListener method
5.4.2.1.1. argument number one: type of event
5.4.2.1.2. argument number two: event handler
5.4.2.1.3. manipulating css styles
6. ReactJS
6.1. Basic knowledge
6.1.1. What is React
6.1.1.1. Development by Facebook
6.1.1.2. SPA
6.1.1.3. Javascript library
6.1.1.4. Create web UI A JavaScript library for building User Interfaces
6.1.1.4.1. UI can be classified into two types.
6.1.1.4.2. What is a component?
6.1.1.4.3. First of all: The structure of the web is a tree structure of components.
6.1.1.4.4. React apps run in the browser
6.1.2. Features of React
6.1.2.1. Virtual DOM
6.1.2.1.1. What is DOM?
6.1.2.1.2. DOM managed with React
6.1.2.1.3. - It's a lightweight copy of the actual DOM, - Instead of updating the entire DOM every time there is a change, the virtual DOM is updated first and then compared to the actual DOM to determine what changes need to be made.
6.1.2.2. JSX
6.1.2.2.1. In other words, you can write like HTML in JavaScript.
6.1.2.3. Difference drawing
6.1.2.3.1. It rewrites only the parts that have changed.
6.1.3. Basic knowledge of React
6.2. Strengths of React What is JSX?
6.2.1. What is JSX ?
6.2.1.1. A language for easily writing HTML in JavaScript (JavaScript extension language)
6.2.1.2. Industry standard in React
6.2.1.2.1. Developed by Facebook
6.2.1.2.2. Most of React's official documentation is written in JSX.
6.2.1.3. why use it
6.2.1.3.1. readability
6.2.1.4. Not JavaScript
6.2.1.4.1. Browsers cannot understand JSX syntax
6.2.2. Basic grammar of JSX
6.2.2.1. React package installation required
6.2.2.2. Almost the same description as Html (class is className)
6.2.2.3. You can embed variables and functions within {}
6.2.2.3.1. Inside {} becomes the world of JavaScript
6.2.2.4. All variable names are in camel case.
6.2.2.5. Close empty elements
6.2.3. Explanation of JSX, which is essential for React
6.3. Environment construction
6.3.1. create-react-app
6.3.1.1. What create-react-app requires
6.3.1.1.1. node 8.10
6.3.1.1.2. npm 5.6
6.3.1.1.3. Install homebrew and nodebrew for installation
6.3.1.2. What is create-react-app?
6.3.1.2.1. A tool that allows you to build a react development environment super easily
6.3.1.2.2. Why create-react-app?
6.3.1.3. Configuring the environment for create-react-app
6.3.1.3.1. src
6.3.1.3.2. public
6.3.1.3.3. build
6.3.1.4. Other environment construction tools
6.3.1.4.1. Next.js
6.3.1.4.2. Gatsby
6.3.1.5. Command collection
6.3.1.5.1. npm run build
6.3.1.5.2. npm run start
6.3.1.5.3. npm run eject
6.3.2. vite
6.4. Component basics (data passing and reuse)
6.4.1. Why use components?
6.4.1.1. to reuse
6.4.1.2. to divide and rule
6.4.1.2.1. Each component is loosely coupled
6.4.1.3. To make it resistant to change
6.4.2. Component type
6.4.2.1. Functional Component
6.4.2.1.1. Components defined functionally
6.4.2.1.2. Features
6.4.2.1.3. simple
6.4.2.2. Class component
6.4.2.2.1. Component defined by class
6.4.2.2.2. Features
6.4.2.2.3. Notice
6.4.3. Pass data with props
6.4.3.1. parent component
6.4.3.1.1. Let's use props
6.4.3.2. Data types that can be passed
6.4.3.2.1. Described within {}
6.4.3.2.2. You can pass anything such as strings, numbers, boolean values, arrays, objects, variables, etc.
6.4.3.2.3. Strings can be written without {}
6.4.3.3. Make the most of your components!
6.5. Component state (state, acquisition and modification)
6.6. What is Babel and Webpack?
6.7. How to make a React router
7. Git
7.1. eval `ssh-agent -s`
7.1.1. ssh-add ~/.ssh/khanhlong1315_rsa
8. Redux
8.1. Operating Principle
8.1.1. State of application store in a one Store
8.1.2. Only one way to change state is create a action
8.1.3. Changes are made with pure functions
8.2. Middleware
8.2.1. Define
8.2.1.1. Class is center of Reducers and Dispath Action. Used to process Async action(API Request)
8.2.2. Library
8.2.2.1. Redux-thunk
8.2.2.1.1. allows you to write action creators that return a function instead of an action. The thunk can be used to delay the dispatch of an action, or to dispatch only if a certain condition is met. The inner function receives the store methods dispatch and getState as parameters.
8.2.2.2. Redux-saga
8.2.2.2.1. One of the most significant advantages of Redux Sagas over other middleware is their handling of side effects natively. As we know, side effects are the operations that affect our application's state outside the current scope of a function.
8.2.2.3. Redux toolkit
8.2.2.3.1. Useful
8.2.2.3.2. Three issues underpin the creation of RTK:
8.2.2.3.3. Component
8.2.2.3.4. Immutable
8.2.3. Operation Position
8.2.3.1. Before Reducer recive Action
8.2.3.2. after Action was dispatch
8.2.4. Component
8.2.4.1. Store
8.2.4.1.1. a store is a state container. This is store state and action was to dispatch in here
8.2.4.2. Action
8.2.4.2.1. is action descible what data you want change
8.2.4.3. Reducer
8.2.4.3.1. is action handler. call action to change state follow dispatch func
8.3. Flux
8.3.1. Architecture
8.3.1.1. for building SPA. Its suggest to split the application into the following parts:
8.3.1.1.1. Stores
8.3.1.1.2. Dispatcher
8.3.1.1.3. Views
8.3.1.1.4. Action/Action Creators