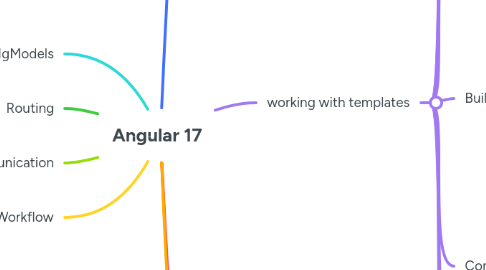
1. NgModels
2. Routing
3. Server Communication
4. Workflow
5. fundamentals
5.1. npm install -g @angular/cli
5.2. ng new my-angular-project
5.2.1. cd my-angular-project
5.2.1.1. ng serve
6. working with templates
6.1. Accessibility
6.1.1. people with disabilities
6.2. Animations
6.3. Attribute, class, and style bindings
6.3.1. <a [href]="product.url">View Product</a>
6.3.2. <button [class.disabled]="!isActive">Buy Now</button>
6.3.3. <div [style.background-color]="selectedColor">This is a box!</div>
6.4. Attribute directives
6.4.1. ngStyle: Dynamically sets styles on elements based on data.
6.4.2. ngClass: Adds or removes CSS classes based on conditions.
6.4.3. ngModel: Two-way data binding for forms.
6.5. Binding syntax
6.5.1. <input type="text" [(ngModel)]="productName">
6.6. Built-in template functions
6.6.1. *ngIf
6.6.2. *ngFor
6.6.3. json
6.6.4. slice
6.6.5. uppercase, lowercase: Changing text case.
6.6.6. date: Formatting dates.
6.7. Content projection
6.7.1. create parent components that act as containers for child components.
6.7.1.1. <ng-content></ng-content>
6.8. Interpolation
6.8.1. Allows for dynamic content based on your component's data.
6.8.1.1. {{ product.price }}
6.9. Template expression operators
6.9.1. 1. Pipe Operator ( | )
6.9.1.1. <span>Price: {{ product.price | currency:'USD' }}</span>
6.9.2. 2. Safe Navigation Operator ( ? )
6.9.2.1. <span *ngIf="user">Welcome, {{ user?.name }}</span>
6.9.3. 3. Non-Null Assertion Operator ( ! )
6.9.3.1. <img [src]="imageUrl!">
6.10. Template reference variables
6.10.1. <input type="text" id="username" #usernameInput>
6.10.1.1. submitForm() { const username = this.usernameInput.nativeElement.value; }
6.11. <ngcontainer>
6.11.1. <ng-container> is like a hidden section in your Angular template. It holds projected content but doesn't become a visible element in the DOM.
6.11.1.1. <ng-container *ngIf="!items.length"> <span>No items to display!</span> </ng-container>
6.12. Pipes
6.12.1. Pipes transform data before it's shown to the customer (user).