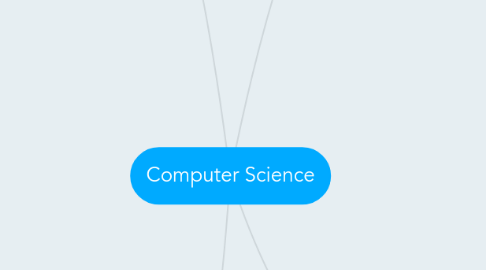
1. Programming I
1.1. Procedural
1.1.1. Primitive types
1.1.1.1. Int
1.1.1.2. char
1.1.1.3. float
1.1.1.4. boolean
1.1.2. Conditionals
1.1.3. Iteration
1.1.4. Functions
1.1.4.1. Parameters
1.1.4.2. Return value
1.1.4.3. ref vs value
1.1.4.4. Method Accessors
1.1.4.5. Recursion
1.1.5. Scope
1.2. OOP
1.2.1. Classes
1.2.2. Objects
1.2.3. Functions
1.2.4. Member Variables
1.2.4.1. Accessors
1.2.5. Inheritance
1.2.6. polymorphism
1.2.7. Encapsulation
1.2.8. Constants
1.3. Searching and Sorting
1.3.1. Bubble
1.3.2. Selection
1.3.3. Insertion
1.3.4. MergeSort
1.3.5. BucketSort
1.3.6. Big O
1.4. Data structures I
1.4.1. Lists/Arrays
1.4.2. Multi-dimensional Arrays
1.5. Testing/Debugging
1.6. projects
1.6.1. typing tutorial
1.6.2. tamagachi
2. Computer Fundamentals
2.1. Google Suite
2.2. Introduction to Computing
2.3. Binary Conversions
2.3.1. Decimal2bin
2.3.2. bin2Decimal
2.3.3. How all computers use binary
2.3.4. binary addition/subtraction
2.4. Intro to Algorithms
2.4.1. understanding p vs np
2.4.2. understanding big o
2.4.3. sorting and searching
2.4.4. A look at some famous algorithms
2.4.4.1. traveling salesman
2.4.4.2. knapsack
2.5. Computer Hardware
2.6. Projects
2.6.1. goodbad_badgood
2.6.2. product mockup
2.6.3. write a letter to a vet
3. Computer Science II
3.1. Data structures
3.1.1. Lists/Arrays
3.1.2. Linked Lists/Trees
3.1.3. Strings
3.1.4. stack/queue
3.1.5. heap
3.2. Databases
3.2.1. CRUD SQL
3.2.2. normlization v denormalization
3.2.3. NoSQL
3.3. Classical algorithms
3.3.1. operations on data structures
3.3.2. sorting
3.3.3. searching
3.4. Design Patterns
3.4.1. Proxy
3.4.2. Singleton
3.4.3. Observer
3.4.4. Factory
3.4.5. MVC
3.5. APIs
3.5.1. Write a chatbot into google classrooms- each person does a text based game
3.6. Advanced algorithms
3.6.1. TSP
3.6.2. Edit distance
3.6.3. Hashing functions
3.6.4. Compression
4. Computer Fundamentals II
4.1. Intro to HTML
4.2. Intro to CSS
4.3. Responsive design using bootstrap
4.4. Understanding Source control and git
4.5. Ionic
4.6. Projects
4.6.1. Build a site about you
4.6.2. Build a site for a non-profit
4.6.3. Build a site for a product
4.6.4. Model a modern existing site of your choosing
4.6.5. Build an Ecommerce site
4.6.6. Use Javascript to make a meme creator
4.6.7. Use Javascript to access your camera and lay over an image for pokemon go