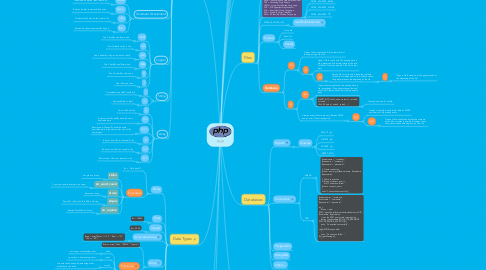
1. Operators
1.1. Arithmetic
1.1.1. +
1.1.1.1. Sum of $x and $y
1.1.2. -
1.1.2.1. Difference of $x and $y
1.1.3. *
1.1.3.1. Product of $x and $y
1.1.4. /
1.1.4.1. Quotient of $x and $y
1.1.5. %
1.1.5.1. Remainder of $x divided by $y
1.1.6. **
1.1.6.1. Result of raising $x to the $y'th power (Introduced in PHP 5.6)
1.2. Assignment
1.2.1. x = y
1.2.1.1. The left operand gets set to the value of the expression on the right
1.2.2. x += y
1.2.2.1. Addition
1.2.3. x -= y
1.2.3.1. Subtraction
1.2.4. x *= y
1.2.4.1. Multiplication
1.2.5. x /= y
1.2.5.1. Division
1.2.6. x %= y
1.2.6.1. Modulus
1.3. Comparison
1.3.1. ==
1.3.1.1. Returns true if $x is equal to $y
1.3.2. ===
1.3.2.1. Returns true if $x is equal to $y, and they are of the same type
1.3.3. !=
1.3.3.1. Returns true if $x is not equal to $y
1.3.4. <>
1.3.4.1. Returns true if $x is not equal to $y
1.3.5. !==
1.3.5.1. Returns true if $x is not equal to $y, or they are not of the same type
1.3.6. >
1.3.6.1. Returns true if $x is greater than $y
1.3.7. <
1.3.7.1. Returns true if $x is less than $y
1.3.8. >=
1.3.8.1. Returns true if $x is greater than or equal to $y
1.3.9. <=
1.3.9.1. Returns true if $x is less than or equal to $y
1.4. Increment / Decrement
1.4.1. ++$x
1.4.1.1. Increments $x by one, then returns $x
1.4.2. $x++
1.4.2.1. Returns $x, then increments $x by one
1.4.3. --$x
1.4.3.1. Decrements $x by one, then returns $x
1.4.4. $x--
1.4.4.1. Returns $x, then decrements $x by one
1.5. Logical
1.5.1. and
1.5.1.1. True if both $x and $y are true
1.5.2. or
1.5.2.1. True if either $x or $y is true
1.5.3. xor
1.5.3.1. True if either $x or $y is true, but not both
1.5.4. &&
1.5.4.1. True if both $x and $y are true
1.5.5. ||
1.5.5.1. True if either $x or $y is true
1.5.6. !
1.5.6.1. True if $x is not true
1.6. String
1.6.1. .
1.6.1.1. Concatenation of $txt1 and $txt2
1.6.2. .=
1.6.2.1. Appends $txt2 to $txt1
1.7. Array
1.7.1. +
1.7.1.1. Union of $x and $y
1.7.2. ==
1.7.2.1. Returns true if $x and $y have the same key/value pairs
1.7.3. ===
1.7.3.1. Returns true if $x and $y have the same key/value pairs in the same order and of the same types
1.7.4. !=
1.7.4.1. Returns true if $x is not equal to $y
1.7.5. <>
1.7.5.1. Returns true if $x is not equal to $y
1.7.6. !==
1.7.6.1. Returns true if $x is not identical to $y
2. Data Types
2.1. String
2.1.1. $x = "Hello world!";
2.1.2. Functions
2.1.2.1. strlen
2.1.2.1.1. Length of a String
2.1.2.2. str_word_count
2.1.2.2.1. Count the number of words in a string
2.1.2.3. strrev
2.1.2.3.1. Reverse a string
2.1.2.4. strpos
2.1.2.4.1. Search For a Specific Text Within a String
2.1.2.5. str_replace
2.1.2.5.1. Replace Text Within a String
2.2. Float
2.2.1. $x = 10.365;
2.3. Integer
2.3.1. $x = 5985;
2.4. Associative Array
2.4.1. $age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43");
2.5. Array
2.5.1. $cars = array("Volvo","BMW","Toyota");
2.5.2. Functions
2.5.2.1. sort()
2.5.2.1.1. sort arrays in ascending order
2.5.2.2. rsort()
2.5.2.2.1. sort arrays in descending order
2.5.2.3. arsort()
2.5.2.3.1. sort associative arrays in descending order, according to the value
2.5.2.4. array_keys
2.5.2.4.1. Return all the keys or a subset of the keys of an array
2.5.2.5. in_array
2.5.2.5.1. Checks if a value exists in an array
2.6. asort()
2.6.1. sort associative arrays in ascending order, according to the value
2.7. Boolean
2.7.1. $x = true;
2.8. Constants
2.8.1. define(name, value, case-insensitive)
2.8.1.1. define("GREETING", "Welcome to W3Schools.com!"); echo GREETING;
3. Loops
3.1. for
3.1.1. for (init counter; test counter; increment counter) { code to be executed; }
3.2. foreach
3.2.1. foreach ($array as $value) { code to be executed; }
3.3. NULL
3.3.1. $x = "Hello world!"; $x = null;
3.4. Resource
3.5. Object
3.5.1. class Car { function Car() { $this->model = "VW"; } } // create an object $herbie = new Car();
3.6. while
3.6.1. while (condition is true) { code to be executed; }
3.7. do...while
3.7.1. do { code to be executed; } while (condition is true);
4. Functions
4.1. User Defined Functions
4.1.1. function functionName() { code to be executed; }
4.2. Function Arguments
4.2.1. function familyName($fname, $year) { echo "$fname Refsnes. Born in $year <br>"; }
4.3. Function Default Argument Value
4.3.1. function setHeight($minheight = 50) { echo "The height is : $minheight <br>"; }
4.3.2. setHeight(350);
4.3.3. setHeight();
4.4. Returning Values
4.4.1. function sum($x, $y) { $z = $x + $y; return $z; }
5. SELECT column_name(s) FROM table_name
5.1. Filters data from table and returns it as array or object
6. UPDATE table_name SET column1=value, column2=value2,... WHERE some_column=some_value
6.1. Changes data for given record (updates)
7. DELETE FROM table_name WHERE some_column = some_value
7.1. Delete record(s) in table
8. fopen()
8.1. Open file
9. Forms
9.1. Handling
9.1.1. $_POST
9.1.1.1. echo $_POST["name"];
9.1.2. $_GET
9.1.2.1. echo $_GET["name"];
10. Files
10.1. Validation
10.2. AJAX = Asynchronous JavaScript and XML CSS = Cascading Style Sheets HTML = Hyper Text Markup Language PHP = PHP Hypertext Preprocessor SQL = Structured Query Language SVG = Scalable Vector Graphics XML = EXtensible Markup Language
10.2.1. FILTER_VALIDATE_EMAIL
10.2.2. FILTER_VALIDATE_PHONE
10.2.3. FILTER_VALIDATE
10.3. FILTER_VALIDATE_URL
10.3.1. readfile($fileName)
10.4. fclose()
10.4.1. Close file
10.4.2. Read file
10.4.3. fread()
10.5. fileModes
10.5.1. r+
10.5.1.1. Open a file for read/write. File pointer starts at the beginning of the file
10.5.1.2. a
10.5.1.2.1. Open a file for write only. The existing data in file is preserved. File pointer starts at the end of the file. Creates a new file if the file doesn't exist
10.5.1.2.2. w
10.5.2. x
10.5.2.1. a+
10.5.2.1.1. Open a file for read/write. The existing data in file is preserved. File pointer starts at the end of the file. Creates a new file if the file doesn't exist
10.5.2.1.2. INSERT INTO table_name (column1, column2, column3,...) VALUES (value1, value2, value3,...)
10.5.2.2. Creates a new file for write only. Returns FALSE and an error if file already exists
10.5.2.2.1. x+
11. Databases
11.1. MySQL
11.1.1. Queries
11.1.1.1. SELECT
11.1.1.2. DELETE
11.1.1.3. UPDATE
11.1.1.4. INSERT_INTO
11.2. Connection
11.2.1. MySQLi
11.2.1.1. $servername = "localhost"; $username = "username"; $password = "password"; // Create connection $conn = new mysqli($servername, $username, $password); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully";
11.2.2. PDO
11.2.2.1. $servername = "localhost"; $username = "username"; $password = "password"; try { $conn = new PDO("mysql:host=$servername;dbname=myDB", $username, $password); // set the PDO error mode to exception $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connected successfully"; } catch(PDOException $e) { echo "Connection failed: " . $e->getMessage(); }
11.3. PostgreSQL
11.4. MongoDB
11.5. others ...
12. Conditionals
12.1. if...else...elseif
12.1.1. if (condition) { code to be executed if condition is true; }
12.1.2. if (condition) { code to be executed if this condition is true; } elseif (condition) { code to be executed if this condition is true; } else { code to be executed if all conditions are false; }
12.2. switch
12.2.1. switch (n) { case label1: code to be executed if n=label1; break; case label2: code to be executed if n=label2; break; case label3: code to be executed if n=label3; break; ... default: code to be executed if n is different from all labels; }