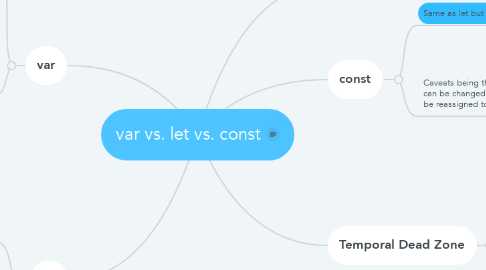
1. var
1.1. Can be redefined
1.1.1. Example:
1.1.1.1. var width = 100; var width = 300; // no errors
1.2. "Function scoped"
1.2.1. Adheres to function scoping
1.2.1.1. Example: function p(){ var i = 0; console.log(i); // 0 } console.log(i); // undefined for(int j = 0; j < 10; j++){ var insideForLoop = 9; } console.log(j); // 10 console.log(insideForLoop); // 9
1.2.2. Does NOT adhere to other types of scoping; like if/for/else; AKA other types of blocks or delimiters of { and }
2. Best practice
2.1. Use const all the time unless you need to change the value in a for loop or something
3. let
3.1. "Block scoped"
3.1.1. Carries scope in all block types
3.2. Can not be redefined
3.2.1. Example:
3.2.1.1. let width = 100; let width = 300; // error!
3.2.1.2. let width = 100; width = 300; width = 600; width = 123; // all good!
4. const
4.1. Same as let but value can't be changed
4.2. Caveats being that properties of objects can be changed but an object variable can't be reassigned to a totally different object
4.2.1. let name = 'Tyler'; const handle = 'tylermcginnis'; name = 'Tyler McGinnis'; // works handle = '@tylermcginnis'; // does not work
4.2.1.1. BUT
4.2.1.1.1. const person = { name: 'Kim Kardashian'; }; person.name = 'Kim Kardashian West'; // works person = {}; // does not work
4.2.1.1.2. Can't change the object it points to but CAN change the properties of the original object
5. Temporal Dead Zone
5.1. Example:
5.1.1. console.log(pizza); var pizza = 'pizza!'; // gives output of 'undefined' but doesn't break or error out
5.1.2. console.log(pizza); const pizza = 'pizza!'; // errors out
5.2. No hoisting for const and let unlike var
5.2.1. This is how I interpreted it but these words weren't said directly