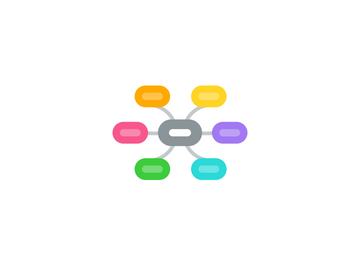
1. Q3. In each of the scenarios given below, name the design pattern that would be the most appropriate to implement.
1.1. a) You are designing an application with a Graphical User Interface (GUI).
1.1.1. You want to implement a menu structure, where each menu element is either (1) an action that the user can perform, or (2) a submenu containing other menu elements.
1.1.1.1. We have a hierarchical structure where we need to treat the branch and leaf nodes uniformly.
1.1.1.2. Composite Design Pattern
1.2. b) Your application needs a logger class to maintain a history of some debugging information (e.g., memory usage).
1.2.1. Your logger class will be used by many other objects within your application, but you want to ensure that they are all sharing one instance of your logger class. In other words, you want to avoid the situation where multiple logger objects are (accidentally or deliberately) instantiated.
1.2.1.1. We need to ensure that only one instance of a class is created and provide a global access point to the object.
1.2.1.2. The Singleton Design Pattern
1.3. c) You an are designing a fast food ordering system that receives orders from customers through a smartphone app.
1.3.1. Your ordering system will queue up orders as they are received, and should make it easy to perform various generic operations on the orders (e.g., repeat an order, cancel an order).
1.3.1.1. We'd like to encapsulate requests in objects so that we can queue them and modify the requests' state.
1.3.1.2. Command Design Pattern
1.4. d) You are working on a backup utility that supports several algorithms for compressing the user’s backup files.
1.4.1. You want to make it easy to extend your utility to support new compression algorithms.
1.4.1.1. i.e., adding a new compression algorithm should be as simple as defining a new class, with minimal or no changes to the existing code.
1.4.1.1.1. Strategy Design Pattern
1.5. e) You are designing a web application that supports several layouts (e.g., smartphone, tablet, and desktop layouts).
1.5.1. You want to define a class so that when the user clicks on a link to view a page, the web application will invoke a method in your class to generate a template page based on the user’s current layout.
1.5.2. Your web application can then insert the content into the template page and send it to the user’s device.
1.6. f) You are writing a GUI application based on a Model-View-Controller architecture.
1.6.1. You need to implement a pattern that allows the View to be automatically notified (so that it can update itself) whenever the Model changes.
1.6.1.1. Observer Design Pattern
2. Q1. b) Suppose you have just cloned your Assignment 2 repo and you want to start working on fixing Bug 42. Below, write the necessary Git command(s) that you need to perform before starting working on the bug fix.
2.1. Read about the feature branch workflow.
3. Q1. a) What is the difference between the int and Integer data types in Java.
3.1. Java has a two-fold type system consisting of primitives such as int, boolean and reference types such as Integer, Boolean.
3.1.1. Every primitive type corresponds to a reference type.
3.2. More about Java Primitives vs Objects
4. Q4. a) Write a regular expression that matches strings representing integers from “1970” to “2018”, inclusive.
4.1. ^(19[7-9]\d|200\d|201[0-8])$
4.1.1. 19[7-9]\d
4.1.1.1. Match 19, then 7 to 9, then any digit.
4.1.2. 200\d
4.1.2.1. OR Match 200 and then any digit.
4.1.3. 201[0-8]
4.1.3.1. OR Match 201, then 0 to 8.
4.2. Use Regex101 to further understand why this works.
5. Q6
5.1. Q6. a) Which design pattern should be used to implement Fibonacci?
5.1.1. Over here we need to provide a way to access its elements without exposing its internal structure.
5.1.2. Iterator Design Pattern
5.2. b) Write your code below. You are allowed to create a new class in addition to Fibonacci if necessary.
5.2.1. Hint
5.2.2. Have the Fibonnaci class implement ierable.
5.2.3. Create a FibonacciIterator class that allows the Fibonacci classd to be iterable.