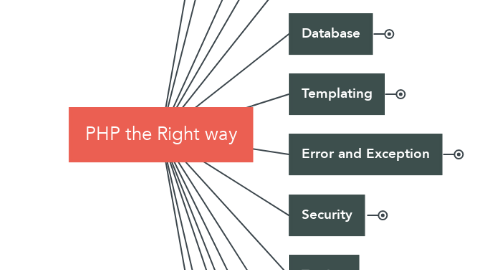
1. Code Style Guide
1.1. PSR-1
1.2. PSR_2
1.3. PSR_4
1.4. PEAR Coding Standards
1.5. Symfony Coding Standards
2. Language Highlights
2.1. Programming Paradigms
2.1.1. General
2.1.1.1. Solid object-oriented model in PHP 5.0 (2004)
2.1.1.2. Anonymous functions and namespaces in PHP 5.3 (2009)
2.1.1.3. Traits in PHP 5.4 (2012)
2.1.2. Object-oriented Programming
2.1.2.1. Including support for classes, abstract classes, interfaces, inheritance, constructors, cloning, exceptions, and more.
2.1.2.2. Read about Object-oriented PHP
2.1.2.3. Read about Traits
2.1.3. Functional Programming
2.1.3.1. A function can be assigned to a variable
2.1.3.2. Functions can be passed as arguments to other functions
2.1.3.3. Functions can return other functions
2.1.3.4. Anonymous functions
2.1.3.4.1. Support for closure
2.1.3.4.2. Present since PHP 5.3 (2009).
2.1.3.4.3. PHP 5.4 added the ability to bind closures to an object’s scope and also improved support for callables
2.1.4. Meta Programming
2.1.4.1. PHP supports various forms of meta-programming through mechanisms like the Reflection API and Magic Methods.
2.1.4.1.1. PHP: ReflectionClass
2.1.4.1.2. Magic Methods
2.1.4.2. Example: Use Meta Programing to generate method
2.2. Namespaces
2.2.1. are a way of encapsulating items
2.2.2. When both libraries are used in the same namespace, they collide and cause trouble => Namespaces solve this problem
2.2.3. Code style: PSR-4 (or PSR-0)
2.2.3.1. The latter requires PHP 5.3, so many PHP 5.2-only projects implement PSR-0.
2.2.3.2. In October 2014 the PHP-FIG deprecated the previous autoloading standard: PSR-0
2.2.3.3. For an autoloader standard for a new application or package -> PSR-4
2.2.4. Namespaces + autoloader
2.2.4.1. namespaces aid autoloader know what $PATH of class and auto require it.
2.2.4.2. Autoloader
2.2.4.2.1. relevant: Regular Expression 1
2.2.4.2.2. relevant: Regular Expression 2
2.2.4.2.3. Autoload with composer
2.3. Standard PHP Library
2.3.1. is a collection of interfaces and classes that are meant to solve common problems.
2.4. Command Line Interface
2.5. Xdebug
3. Dependency Management
3.1. Composer
3.1.1. Composer installs packages in separate project that mean only in project scope, NOT global packages.
3.2. PEAR
3.2.1. PEAR installs packages globally, which means after installing them once they are available to all projects on that server.
3.3. Handling PEAR dependencies with Composer
4. Coding Practices
4.1. The Basics
4.2. Date and Time
4.3. Design Patterns
4.4. Working with UTF-8
4.4.1. UTF-8 at the PHP level
4.4.2. UTF-8 at the Database level
4.4.3. UTF-8 at the browser level
4.5. Internationalization (i18n) and Localization (l10n)
4.6. Common ways to implement
4.7. Gettext
4.7.1. Installation
4.7.2. Structure
4.7.2.1. Types of files
4.7.2.2. Domains
4.7.2.3. Locale code
4.7.2.4. Directory structure
4.7.2.5. Plural forms
4.7.2.6. Sample implementation
4.7.2.7. Discussion on l10n keys
4.7.2.8. Everyday usage
4.7.2.9. .......
5. Dependency Injection
5.1. Basic Concept
5.1.1. Dependency injection is a software design pattern that allows the removal of hard-coded dependencies and makes it possible to change them, whether at run-time or compile-time
5.2. Complex Problem
5.2.1. Inversion of Control
5.2.1.1. Dependency Injection is a way to implement Inversion of Control
5.2.2. S.O.L.I.D
5.2.2.1. Single Responsibility Principle
5.2.2.1.1. Every class should only have responsibility over a single part of the functionality provided by the software.
5.2.2.2. Open/Closed Principle
5.2.2.2.1. We can very easily extend our code with support for something new without having to modify existing code (inheritance, traits, implements, extends,..)
5.2.2.3. Liskov Substitution Principle
5.2.2.3.1. “Child classes should never break the parent class’ type definitions.”
5.2.2.4. Interface Segregation Principle
5.2.2.4.1. Instead of having a single monolithic interface that all conforming classes need to implement, we should instead provide a set of smaller, concept-specific interfaces that a conforming class implements one or more of.
5.2.2.5. Dependency Inversion Principle
5.2.2.5.1. “Depend on Abstractions. Do not depend on concretions.”. Put simply, this means our dependencies should be interfaces/contracts or abstract classes rather than concrete implementations.
5.2.2.5.2. Classes communicate together through interfaces/abstract instead of implementation
5.3. Containers
5.3.1. aid init lower module
5.4. Further Reading
6. Database
6.1. MySQL Extension
6.1.1. { mysql } has superseded by { mysqli, PDO }
6.1.2. { mysql } officially removed in PHP 7.0.
6.2. PDO Extension
6.2.1. A database connection abstraction library
6.2.2. Built into PHP since 5.1.0
6.2.3. Provides a common interface to talk with many different databases.
6.3. Interacting with Databases
6.3.1. Use OOP to create a model - similar to MVC
6.4. Abstraction Layers
6.4.1. Some abstraction layers have been built using the PSR-0 or PSR-4 namespace standards
6.4.2. Open source
6.4.2.1. Aura SQL
6.4.2.2. Doctrine2 DBAL
6.4.2.3. Propel
6.4.2.4. Zend-db
7. Templating
7.1. Benefits
7.1.1. is clear separation they create between the presentation logic and the rest of your application.
7.1.2. Templates have the sole responsibility of displaying formatted content
7.1.3. Templates are not responsible for data lookup, persistence or other more complex tasks.
7.1.4. Improve the organization of presentation code
7.2. Plain PHP Templates
7.2.1. are simply templates that use native PHP code
7.2.2. can combine PHP code within other code, like HTML
7.3. Compiled Templates
7.4. Libraries
8. Error and Exception
8.1. Errors
8.1.1. Error Severity
8.1.1.1. E_ERROR
8.1.1.1.1. Errors are fatal run-time errors and are usually caused by faults in your code and need to be fixed as they’ll cause PHP to stop executing.
8.1.1.2. E_NOTICE
8.1.1.2.1. Notices are advisory messages caused by code that may or may not cause problems during the execution of the script, execution is not halted.
8.1.1.3. E_WARNING
8.1.1.3.1. Warnings are non-fatal errors, execution of the script will not be halted.
8.1.2. Changing PHP’s Error Reporting Behaviour
8.1.2.1. if you only want to see Errors and Warnings
8.1.2.1.1. <?php error_reporting(E_ERROR | E_WARNING);
8.1.3. Inline Error Suppression
8.1.3.1. You can also tell PHP to suppress specific errors with the Error Control Operator @
8.1.3.1.1. PHP handles expressions using an @ in a less performant way than expressions without an @
8.1.3.2. If there’s a way to avoid the error suppression operator, you should consider it
8.1.3.3. Earlier we mentioned there’s no way in a stock PHP system to turn off the error control operator. However, Xdebug has an xdebug.scream ini setting which will disable the error control operator. You can set this via your php.ini file with the following.
8.1.4. ErrorException
8.1.4.1. More information on this and details on how to use ErrorException with error handling can be found at ErrorException Class.
8.2. Exceptions
8.2.1. SPL Exceptions
8.2.1.1. create a specialized Exception type by sub-classing the generic Exception class
9. Security
9.1. Web Application Security
9.1.1. 1. Code-data separation
9.1.1.1. When data is executed as code, you get SQL Injection, Cross-Site Scripting, Local/Remote File Inclusion, etc.
9.1.1.2. When code is printed as data, you get information leaks (source code disclosure)
9.1.2. 2. Application logic
9.1.2.1. Missing authentication or authorization controls
9.1.2.2. Input validation.
9.1.3. 3. Operating environment
9.1.3.1. PHP versions
9.1.3.2. Third party libraries.
9.1.3.3. The operating system.
9.1.4. 4. Cryptography weaknesses
9.1.4.1. Weak random numbers
9.1.4.2. Chosen-ciphertext attacks
9.1.4.3. Side-channel information leaks
9.2. Password Hashing
9.2.1. Hashing is an irreversible, one-way function.
9.2.2. Hashing passwords with { password_hash }
9.2.2.1. In PHP 5.5
9.2.2.2. using BCrypt
9.3. Data Filtering
9.3.1. Cross-Site Scripting (XSS)
9.3.1.1. Hacker input HTML or javascipt into website
9.3.1.2. Removing HTML tags with the strip_tags()
9.3.2. Executed on the command line
9.3.2.1. Executed command’s arguments
9.3.2.2. Use the built-in escapeshellarg()
9.3.3. Load from the filesystem
9.3.3.1. This can be exploited by changing the filename to a file path
9.3.3.2. Remove "/", "../", null bytes, or other characters from the file path
9.3.4. Conclusion
9.3.4.1. Sanitization
9.3.4.2. Unserialization
9.3.4.3. Validation
9.4. Configuration Files
9.4.1. Store your configuration information where it cannot be accessed directly and pulled in via the file system.
9.4.2. If you store in the document root, .php -> not be output as plain text
9.4.3. Information in configuration files should be protected accordingly, either through encryption or group/user file system permissions
9.4.4. Ensure that you do not commit configuration files containing sensitive information e.g. passwords or API tokens to source control
9.5. Register Globals
9.5.1. As of PHP 5.4.0 -> remove register_globals
9.5.2. If the form include input name, email ->
9.5.2.1. isOFF: get value via $_POST['name'] và $_POST['email']
9.5.2.2. isON: PHP Default create two variable $name and $email -> Hacker easy get value
9.6. Error Reporting
9.6.1. It can also expose information about the structure of your application
9.6.2. Configure your server
9.6.2.1. Development
9.6.2.1.1. display_errors = On display_startup_errors = On error_reporting = -1 log_errors = On
9.6.2.2. Production
9.6.2.2.1. display_errors = Off display_startup_errors = Off error_reporting = E_ALL log_errors = On
10. Testing
10.1. Test Driven Development
10.1.1. Definition
10.1.1.1. Test-driven development (TDD) is a software development process that relies on the repetition of a very short development cycle
10.1.2. Unit Testing
10.1.2.1. By using Dependency Injection and building “mock” classes and stubs you can verify that dependencies are correctly used for even better test coverage
10.1.3. Integration Testing
10.1.3.1. Integration testing (“I&T”) is the phase in software testing in which individual software modules are combined and tested as a group.
10.1.3.2. It occurs after unit testing and before validation testing.
10.1.4. Functional Testing
10.1.4.1. Consists of using tools to create automated tests
10.1.5. Functional Testing Tools
10.1.5.1. Selenium
10.1.5.2. Mink
10.1.5.3. Codeception
10.1.5.3.1. is a full-stack testing framework that includes acceptance testing tools
10.1.5.4. Storyplayer
10.1.5.4.1. is a full-stack testing framework that includes support for creating and destroying test environments on demand
10.2. Behavior Driven Development
10.2.1. SpecBDD
10.2.1.1. Focuses on technical behavior of code
10.2.1.2. PHP framework: Behat
10.2.1.3. Write human-readable stories that describe the behavior of your application.
10.2.2. StoryBDD
10.2.2.1. Focuses on business or feature behaviors or interactions
10.2.2.2. PHP framework: PHPSpec
10.2.2.3. Write specifications that describe how your actual code should behave. Instead of testing a function or method, you are describing how that function or method should behave.
10.3. Complementary Testing Tools
10.3.1. Selenium
10.3.1.1. is a browser automation tool which can be integrated with PHPUnit
10.3.2. Mockery
10.3.2.1. is a Mock Object Framework which can be integrated with PHPUnit or PHPSpec
10.3.3. Prophecy
10.3.3.1. is a highly opinionated yet very powerful and flexible PHP object mocking framework. It’s integrated with PHPSpec and can be used with PHPUnit.
10.3.4. php-mock
10.3.4.1. is a library to help to mock PHP native functions.
10.3.5. Infection
10.3.5.1. is a PHP implementation of Mutation Testing to help to measure the effectiveness of your tests.
11. Sever and Deployment
11.1. Platform as a Service (PaaS)
11.1.1. PaaS provides the system and network architecture necessary to run PHP applications on the web.
11.2. Virtual or Dedicated Servers
11.2.1. nginx and PHP-FPM
11.2.1.1. Lightweight, high-performance web server
11.2.1.2. Uses less memory than Apache and can better handle more concurrent requests.
11.2.1.3. This is especially important on virtual servers that don’t have much memory to spare.
11.2.2. Apache and PHP
11.2.2.1. Apache uses more resources than nginx by default and cannot handle as many visitors at the same time.
11.2.2.2. If you are running Apache 2.4 or later, you can use mod_proxy_fcgi to get great performance that is easy to setup.
11.2.2.3. if you want to squeeze more performance and stability out of Apache
11.2.2.3.1. take advantage of the same FPM system as nginx
11.2.2.3.2. un the worker MPM or event MPM with mod_fastcgi or mod_fcgid
11.2.2.3.3. This configuration will be significantly more memory efficient and much faster but it is more work to set up
11.3. Shared Servers
11.3.1. Allow you and other developers to deploy websites to a single machine.
11.3.2. Upside
11.3.2.1. a cheap commodity
11.3.3. Downside
11.3.3.1. You never know what kind of a ruckus your neighboring tenants are going to create
11.3.3.2. loading down the server or opening up security holes
11.4. Building and Deploying your Application
11.4.1. Deployment Tools
11.4.1.1. Phing
11.4.1.2. Capistrano
11.4.1.3. Ansistrano
11.4.1.4. Rocketeer
11.4.1.5. Deployer
11.4.1.6. Magallanes
11.4.1.7. Further reading
11.4.1.7.1. Automate your project with Apache Ant
11.4.1.7.2. Deploying PHP Applications
11.4.2. Server Provisioning
11.4.2.1. Ansible
11.4.2.2. Puppet
11.4.2.3. Chef
11.4.2.4. Further reading
11.4.2.4.1. An Ansible Tutorial
11.4.2.4.2. Ansible for DevOps
11.4.2.4.3. Ansible for AWS
11.4.2.4.4. Three part blog series about deploying a LAMP application with Chef, Vagrant, and EC2
11.4.2.4.5. Chef Cookbook which installs and configures PHP and the PEAR package management system
11.4.2.4.6. Chef video tutorial series
11.4.3. Continuous Integration
11.4.3.1. is a software development practice where members of a team integrate their work frequently, usually each person integrates at least daily — leading to multiple integrations per day.
11.4.3.2. Many teams find that this approach leads to significantly reduced integration problems and allows a team to develop cohesive software more rapidly
11.4.3.3. Further reading
11.4.3.3.1. Continuous Integration with Jenkins
11.4.3.3.2. Continuous Integration with PHPCI
11.4.3.3.3. Continuous Integration with Teamcity
12. Virtualization
12.1. Vagrant
12.2. Docker
13. Catching
13.1. Opcode Cache
13.1.1. When a PHP file is executed, it must first be compiled into opcodes (machine language instructions for the CPU).
13.1.2. An opcode cache prevents redundant compilation by storing opcodes in memory and reusing them on successive calls
13.1.3. Since PHP 5.5 there is one built in - Zend OPcache
13.2. Object Caching
13.2.1. APCu
13.2.1.1. an excellent choice for object caching
13.2.1.2. it includes a simple API for adding your own data to its memory cache and is very easy to setup and use.
13.2.1.3. The one real limitation of APCu is that it is tied to the server it’s installed on.
13.2.1.4. Use it when?
13.2.1.4.1. Usually outperform memcached in terms of access speed
13.2.1.4.2. Easy to set up and use
13.2.2. Memcached
13.2.2.1. is installed as a separate service and can be accessed across the network
13.2.2.2. it’s not tied to the PHP processes
13.2.2.3. Use it when?
13.2.2.3.1. It's able to scale up faster and further
13.2.2.3.2. multiple servers running
13.2.2.3.3. the extra features that memcached offers
13.2.3. Should I use APCu or Mencached?
13.2.3.1. Note that when running PHP as a (Fast-)CGI application inside your webserver, every PHP process will have its own cache, i.e. APCu data is not shared between your worker processes. In these cases, you might want to consider using memcached instead, as it’s not tied to the PHP processes.
13.2.3.2. In a networked configuration APCu will usually outperform memcached in terms of access speed, but memcached will be able to scale up faster and further.
13.2.3.3. If you do not expect to have multiple servers running your application, or do not need the extra features that memcached offers then APCu is probably your best choice for object caching.
14. Documenting your Code
14.1. PHPDoc
14.1.1. Definition
14.1.1.1. PHPDoc is an informal standard for commenting PHP code. There are a lot of different tags available. The full list of tags and examples can be found at the PHPDoc manual.
14.1.2. Doc for class
14.1.2.1. @author
14.1.2.2. @link
14.1.3. Inside the class
14.1.3.1. @param
14.1.3.2. @return
14.1.3.3. @throws
15. Resource
15.1. From the Source
15.2. People to Follow
15.3. Mentoring
15.4. PHP PaaS Providers
15.5. Frameworks
15.5.1. Micro Frameworks
15.5.2. Full-Stack Frameworks
15.5.3. Component Frameworks