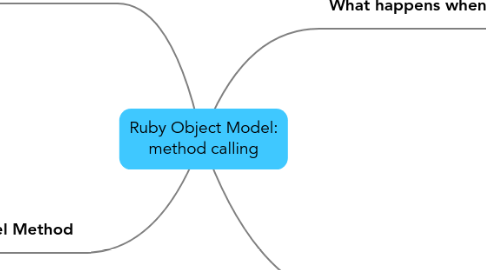
1. Method Execution
1.1. Every line of Ruby code is executed inside an object:
1.1.1. the current object
1.1.2. self
1.1.2.1. only one object at a time can take the role of self
1.1.2.2. when you call a method, the receiver takes the role of self, and from that moment on...
1.1.2.2.1. all instance variables are instance variables of self
1.1.2.2.2. all methods called without an explicit receiver are called on self
1.1.2.2.3. as soon as your code explicitly calls a method on some other object, that other object becomes self
1.1.2.3. in a class or module definition, the role of self is taken by the class or module
1.1.2.4. outside a class/module definition and outside a method, the role of self is taken by the top level context
1.1.2.4.1. main
1.1.2.4.2. it's the object you're in when you're at the tip level of the call stack
1.1.2.5. current object quiz
1.1.2.5.1. puts "current object is #{self}" class MyClass puts "current object is #{self}" def my_stunning_method puts "current object is #{self}" end end MyClass.new.my_stunning_method
1.1.2.5.2. see also http://rubylearning.com/satishtalim/ruby_self.html
2. spell: Kernel Method
2.1. class Object includes Kernel
2.1.1. so, Kernel gets into every object's ancestors chain
2.1.1.1. and you can call the Kernel methods from anywhere
2.1.1.1.1. e.g. puts
2.1.2. you can take advantage of this mechanism yourself
2.1.2.1. if you add a method to Kernel, this Kernel Method will be available to all objects
2.1.2.1.1. e.g. The RubyGems example
3. What happens when you call a method?
3.1. when you call a method, Ruby does two things
3.1.1. it finds the method
3.1.1.1. method lookup
3.1.2. it executes the method
3.1.2.1. with the receiver as self
4. Method Lookup
4.1. to find a method, Ruby goes into the receivers's class, and from there it climbs the ancestors chain until it finds the method
4.1.1. aka "one step to the right, than up" rule
4.1.2. Class#ancestors method asks a class for its ancestors chain
4.1.3. ancestors chain also include modules!
4.1.3.1. also known as "include classes"
4.1.3.2. When you include a module in a class, Ruby creates an anonymous class that wraps the module and inserts the anonymous class in the chain, just above the including class itself
4.1.3.3. exercise: a simple program to spot in which class/module a method is defined
4.1.3.3.1. require 'test/unit' class KernelTest < Test::Unit::TestCase def test_lookup_method assert_equal(Kernel, "a string instance".where_is(:send)) assert_equal(Kernel, "a string instance".where_is("instance_variable_get")) assert_equal(String, "a string instance".where_is("to_s")) assert_equal(Integer, 12.where_is("ceil")) assert_equal(Numeric, 12.where_is("nonzero?")) assert_nil("ciao".where_is("unexisting_method")) end end