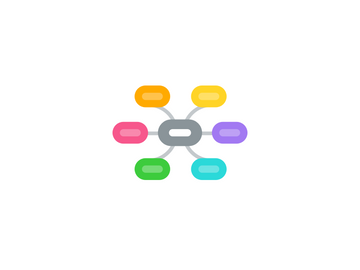
1. keep your iTerm window
1.1. large
1.1.1. option-command-left arrow with Spectacle
1.2. clean
1.2.1. use Command-R a lot to clear it
2. i.e. it's all inside Rails/gem code
3. Do this when the error message doesn't help
4. explore runtime context
4.1. pry
4.1.1. require 'pry' in your file
4.1.2. use binding.pry anywhere in your file
4.1.2.1. this opens up a console with current context
4.1.2.2. try typing in self to check
4.2. puts or p
4.2.1. why
4.2.1.1. increase your understanding
4.2.1.2. make a hypothesis and confirm or be surprised
4.2.2. how (style, optional)
4.2.2.1. "variable is #{variable}"
4.2.2.2. use puts instead of print for newline
4.2.2.3. use sleeps for infinite loops
4.2.3. where
4.2.3.1. infinite loops
4.2.3.1.1. once per loop
4.2.3.2. methods
4.2.3.2.1. very first line
4.2.3.3. runner code is okay
4.2.3.3.1. inside specs is GREAT
4.2.4. for objects
4.2.4.1. puts object.inspect
4.2.4.1.1. (or just require 'pp' and pp instead of puts)
5. RTFM: read the (error) message
5.1. read the entire message and try to understand it
5.2. try Googling it
5.3. try command-clicking in iTerm on the line of code where it happened, to open it up in SublimeText
6. follow your code
6.1. What code did you change to cause the error?
6.1.1. Try to reverse it
6.2. Start with the request you make
6.2.1. usually some route gets called by browser/spec
6.3. Then read your code, step-by-step
6.3.1. Controller
6.3.1.1. Any model methods
6.3.1.1.1. etc.
7. Google/StackOverflow it
7.1. what
7.1.1. specific error message you're getting
7.1.2. thing you're trying to do
7.2. how
7.2.1. read StackOverflow items first
7.2.2. skim, don't read everything
8. use Rubocop
8.1. add it to your Gemfile
8.2. run rubocop -D filepath
8.2.1. or rubocop -RD for Rails
8.3. especially helpful for mismatched do/end, (, {
9. use specs
9.1. write a spec for each sub-method
9.1.1. or just test something smaller
9.2. def method end
9.2.1. run one at a time
9.2.2. process them in order
9.2.2.1. sometimes troubleshooting a spec is easier than doing it in the console
9.2.2.1.1. you can print stuff, fresh DB, etc.
9.2.3. usually easiest to hardest
9.3. sometimes troubleshooting in localhost browser is easier than a spec
9.3.1. you can see it, use better_errors gem, etc.
10. big picture debugging
10.1. can you revert to a few commits back?
10.2. can another team member get same code to work?
10.2.1. yes
10.2.1.1. check if you're running the same code
10.2.1.2. maybe delete your repo/branch and clone again
11. solve a smaller problem
11.1. sanity check
11.1.1. when
11.1.1.1. if you're totally confused about what's going on
11.1.2. why
11.1.2.1. feel sane, in control again
11.1.3. what
11.1.3.1. predict something simple
11.1.3.2. check if it's true
11.1.4. examples
11.1.4.1. variable.class == String
11.1.4.1.1. maybe you're passing the wrong class?
11.1.4.2. editing view
11.1.4.2.1. change 'Edit' to 'XEdit'
11.1.4.3. esp. for styling issues
11.1.4.3.1. open localhost in fresh Incognito window
11.2. start a new file, cleaner, less complex
11.2.1. just to increase/confirm your understanding
11.2.2. if it works
11.2.2.1. increase complexity a bit
11.2.2.2. or just consider what's different
11.2.3. if it doesn't
11.2.3.1. find an even simpler problem
11.2.3.1.1. best idea since you know it
12. look at similar, working code
12.1. you wrote previously
12.1.1. build up a go-to library
12.2. cohort mates wrote
12.2.1. except for assessments
12.2.1.1. obviously
13. learn to explain
13.1. explain your problem to a 'rubber duck'
13.1.1. or camera
13.1.2. or cohort mate
13.2. make yourself think like a dumb computer
13.2.1. go through the code step by step
13.2.2. do exactly what it tells you to do
13.3. try it in real life
13.3.1. especially for iterating through objects
13.3.2. get a bunch of physical objects to mimic your objects