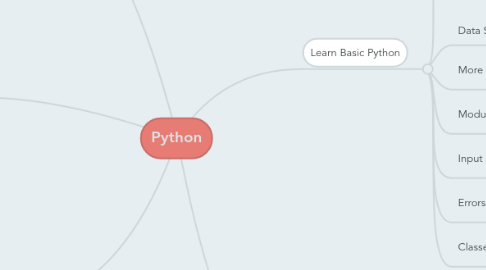
1. Education
2. Frameworks
2.1. Web Programming
2.1.1. Django
2.1.2. Flask
2.2. GUI Development
2.2.1. wxPython
3. Use
3.1. Web and Internet Development
3.2. Database Access
3.3. Desktop GUIs
3.4. Scientific & Numeric
3.5. Network Programming
3.6. Software & Game Development
4. Learn Basic Python
4.1. An Informal Introduction to Python
4.1.1. Using Python as a Calculator
4.1.1.1. Numbers
4.1.1.2. Strings
4.1.1.3. Lists
4.1.2. First Steps Towards Programming
4.2. Data Structures
4.2.1. More on Lists
4.2.1.1. Using Lists as Stacks
4.2.1.2. Using Lists as Queues
4.2.1.3. List Comprehensions
4.2.1.4. Nested List Comprehensions
4.2.2. The del statement
4.2.3. Tuples and Sequences
4.2.4. Sets
4.2.5. Dictionaries
4.2.6. Looping Techniques
4.2.7. More on Conditions
4.2.8. Comparing Sequences and Other Types
4.3. More Control Flow Tools
4.3.1. if Statements
4.3.2. for Statements
4.3.3. The range() Function
4.3.4. break and continue Statements, and else Clauses on Loops
4.3.5. pass Statements
4.3.6. Defining Functions
4.3.7. More on Defining Functions
4.3.7.1. Default Argument Values
4.3.7.2. Keyword Arguments
4.3.7.3. Arbitrary Argument Lists
4.3.7.4. Unpacking Argument Lists
4.3.7.5. Lambda Expressions
4.3.7.6. Documentation Strings
4.3.7.7. Function Annotations
4.3.8. Intermezzo: Coding Style
4.4. Modules
4.4.1. More on Modules
4.4.1.1. Executing modules as scripts
4.4.1.2. The Module Search Path
4.4.1.3. “Compiled” Python files
4.4.2. Standard Modules
4.4.3. The dir() Function
4.4.4. Packages
4.4.4.1. Importing * From a Package
4.4.4.2. Intra-package References
4.4.4.3. Packages in Multiple Directories
4.5. Input and Output
4.5.1. Fancier Output Formatting
4.5.1.1. Old string formatting
4.5.2. Reading and Writing Files
4.5.2.1. Methods of File Objects
4.5.2.2. Saving structured data with json
4.6. Errors and Exceptions
4.6.1. Syntax Errors
4.6.2. Exceptions
4.6.3. Handling Exceptions
4.6.4. Raising Exceptions
4.6.5. User-defined Exceptions
4.6.6. Defining Clean-up Actions
4.6.7. Predefined Clean-up Actions
4.7. Classes
4.7.1. A Word About Names and Objects
4.7.2. Python Scopes and Namespaces
4.7.2.1. Scopes and Namespaces Example
4.7.3. A First Look at Classes
4.7.3.1. Class Definition Syntax
4.7.3.2. Class Objects
4.7.3.3. Instance Objects
4.7.3.4. Method Objects
4.7.3.5. Class and Instance Variables
4.7.4. Random Remarks
4.7.5. Inheritance
4.7.5.1. Multiple Inheritance
4.7.6. Private Variables
4.7.7. Odds and Ends
4.7.8. Exceptions Are Classes Too
4.7.9. Iterators
4.7.10. Generators
4.7.11. Generator Expressions
5. Learn Python Library
5.1. Brief Tour of the Standard Library
5.1.1. Operating System Interface
5.1.2. File Wildcards
5.1.3. Command Line Arguments
5.1.4. Error Output Redirection and Program Termination
5.1.5. String Pattern Matching
5.1.6. Mathematics
5.1.7. Internet Access
5.1.8. Dates and Times
5.1.9. Data Compression
5.1.10. Performance Measurement
5.1.11. Quality Control
5.1.12. Batteries Included
5.2. Brief Tour of the Standard Library – Part II
5.2.1. Output Formatting
5.2.2. Templating
5.2.3. Working with Binary Data Record Layouts
5.2.4. Multi-threading
5.2.5. Logging
5.2.6. Weak References
5.2.7. Tools for Working with Lists
5.2.8. Decimal Floating Point Arithmetic