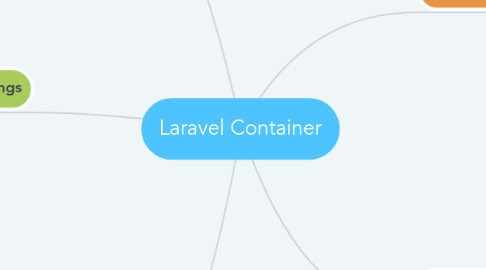
1. Injection
1.1. Constructor Injection
1.1.1. app() also resolving: controllers, middleware, jobs, event listener
1.1.2. if we want a new Logger, just put it in __constructor, and container will typehint it
1.2. Method Injection
1.2.1. Laravel don't just read constructor signature, It also read `method` 's signature
1.2.2. we can call methods directly by app() helper
1.2.3. sample code
2. Bindings
2.1. Closure Bindings
2.1.1. place: bindings via ServiceProvider's register methods
2.1.2. Sample Code
2.2. Singleton, Aliases and Instances Bindings
2.2.1. singleton: using singleton pattern to cache the result
2.2.2. we can alias Class instance to easy remember
2.2.3. sample code
2.3. Concrete Instance Binding
2.3.1. we can bind interface with concrete class
2.3.2. we can typehints interface instead of Class in Constructor
2.4. ContextualBindings
2.4.1. resolve interface depending on context
2.4.2. sample code
3. Facades
3.1. Definition: proxied static call to non static resources
3.2. Benefit: . simple access to core pieces of Laravel . available in global namespace (\Log is alias of \Illuminate\Support\Facades\Log)
3.3. sample code
3.4. How Facade works
3.4.1. facade has the `getFacadeAccessor` defines the key that Laravel should use to lookup this facade backing instances.
3.4.2. Every call to Log facade will be proxied to class call.
3.4.3. We can create our own facade by extending the `Illuminate\Support\Facades\Facade` and adding: getFacadeAccessor() method
4. Dependency Injection
4.1. Dependency Injection 101
4.1.1. as a name rather instantiated up (in constructor/ method), it will be injected from outside
4.1.2. Depends on constructor or method we have Constructor Injection and Method Injection
4.1.3. Benefit: we inject class from outside, so we are free to change what we are injecting
4.2. Dependency Injection in Laravel
4.2.1. Benefit: constructor injection
4.2.2. sample code
5. Container
5.1. Definition: . Application Container . IoC (Inversion of Control) Container . Service Container . DI (Dependency Injection) Container
5.2. app() global helper, receive any string as Fully Qualified Class Name (FQCN), then we have instance of that class
5.3. Benefit: app() creates instance of class and returned it for us
5.4. Thoughts: app() just return the instance, they don't even know about parameters (should set manually)
5.5. Typehints in PHP
5.5.1. means putting the name of class or interface in front of variables `Logger $logger` : this is typehints