objects
da Zoltán Parragi
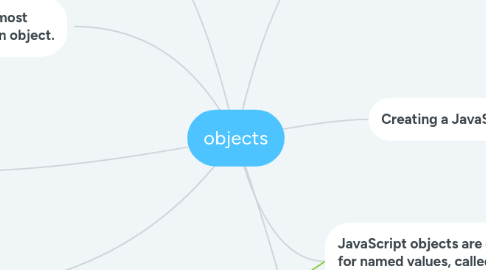
1. In JavaScript, objects are king. If you understand objects, you understand JavaScript.
1.1. In JavaScript, almost "everything" is an object.
2. In JavaScript, almost "everything" is an object.
2.1. All JavaScript values, except primitives, are objects.
2.2. primitives: string number boolean null undefined
2.3. Primitive values are immutable (they are hardcoded and therefore cannot be changed).
3. JavaScript variables can contain single values eg.: var person = "John Doe";
4. Objects are variables too. But objects can contain many values. name : value pairs eg.:var person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
4.1. A JavaScript object is a collection of named values
4.2. name = property, value = key
5. Object Methods
5.1. An object method is an object property containing a function definition. (actions)
6. JavaScript objects are containers for named values, called properties and methods.
7. Creating a JavaScript Object
7.1. Object Literal, the easiest way An object literal is a list of name:value pairs (like age:50) inside curly braces {}. eg. object with 4 properties: var person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
7.2. Using the JavaScript Keyword: new. There is no need to use new Object(). For simplicity, readability and execution speed, use the first one (the object literal method).
8. JavaScript Objects are Mutable
8.1. If person is an object, the following statement will not create a copy of person: var x = person; // This will not create a copy of person. The object x is not a copy of person. It is person. Both x and person are the same object. Any changes to x will also change person, because x and person are the same object.
8.1.1. eg.: var person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"} var x = person; x.age = 10; // This will change both x.age and person.age
8.1.2. JavaScript variables are not mutable. Only JavaScript objects.