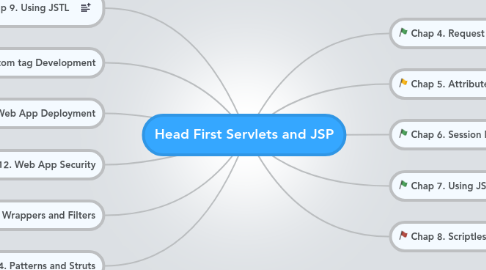
1. Chap 4. Request and Response
1.1. Container
1.1.1. Runs multiples threads to process multiple requests to a single servlet
1.1.2. Create request and response objects
1.2. Servlet's life
1.2.1. 1. Load class
1.2.2. 2. Instantiate servlet (constructor runs)
1.2.3. 3. init()
1.2.4. 4. Service()
1.2.5. 5. destroy()
1.3. Http Methods
1.3.1. GET
1.3.2. POST
1.3.3. HEAD
1.3.4. TRACE
1.3.5. PUT
1.3.6. DELETE
1.3.7. OPTIONS
1.3.8. CONNECT
1.4. Idempotent (GET, HEAD, PUT, DELETE, TRACE, OPTIONS)
1.5. Request
1.5.1. String param = request.getParameter("parameter"); request.getParameterValues("size")[0];
1.5.2. String client = request.getHeader("User-Agent"); request.getIntHeader("foo");
1.5.3. Cookie[] cookies = request.getCookies();
1.5.4. HttpSession session = request.getSession();
1.5.5. String method = request.getMethod();
1.5.6. ServletInputStream input = request.getInputSream();
1.6. Response
1.6.1. response.setContentType("application/jar");
1.6.2. PrintWriter writer = response.getWriter(); writer.println("some text and HTML");
1.6.3. ServletOutputStream out = response.getOutputStream(); out.write(aByteArray);
1.6.4. response.setHeader("foo", "bar"); response.setIntHeader("TheAnswer", 42);
1.6.5. response.addHeader("foo", "bar");
1.6.6. response.addCookie(cookie);
1.6.7. reponse.sendError(404);
1.6.8. response.sendRedirect("foo/some/pic.jpg");
1.6.9. RequestDispatcher view = request.getRequestDispatcher("foo.jsp"); view.forward(request,response);
1.7. javax.servlet.ServletRequest
1.7.1. Methods
1.7.1.1. getAttribute(String)
1.7.1.2. getContentLenght()
1.7.1.3. getinputStream()
1.7.1.4. getLocalPort()
1.7.1.5. getRemotePort()
1.7.1.6. getServerPort()
1.7.1.7. getParameter(String)
1.7.1.8. getParameterValues(String)
1.7.1.9. getParameterNames()
1.7.1.10. more..
1.7.2. father of
1.7.2.1. javax.servlet.http.HttpServletRequest
1.7.2.1.1. Methods
1.8. javax.servlet.ServletResponse
1.8.1. Methods
1.8.1.1. getBufferSize()
1.8.1.2. setContentType()
1.8.1.3. getOutputStream()
1.8.1.4. getWriter()
1.8.1.5. setContentLength()
1.8.1.6. more..
1.8.2. father of
1.8.2.1. javax.servlet.http.HttpServletResponse
1.8.2.1.1. Methods
2. Chap 5. Attributes and Listeners
2.1. Context init Parameters
2.1.1. <web-app> <context-param> <param-name>foo</.. <param-value>bar</...
2.1.2. getServletContext.getInintParam("foo");
2.1.3. Available to any servlet and JSP part of the web app
2.2. Servlet init Parameters
2.2.1. <servlet> <servlet-name> <init-param> <param-name>foo</.. <param-value>bar</...
2.2.2. getServletConfig.getInitParam("foo");
2.2.3. Available only to the servlet for which <init-param> was configured
2.3. ServletContext
2.3.1. getInitParameter(String) : String
2.3.2. getInitParameterNames() : String[]
2.3.3. getAttribute(String) : String
2.3.4. getAttributesName() : String[]
2.3.5. setAttributes(String, Object) : void
2.3.6. removeAttribute(String) : void
2.3.7. getRequestDispatcher(String) : RequestDispatcher
2.4. Listeners
2.4.1. <listener> <listener-class> com.some.foor.Listener </..
2.4.2. the AttributeEvent.getValue, holds the replaced or removed value
2.4.3. Types
2.4.3.1. javax.servlet.ServletContextListener : ServletContextEvent
2.4.3.1.1. contextInitialized
2.4.3.1.2. contextDestroyed
2.4.3.2. javax.servlet.ServletRequestListener : ServletRequestEvent
2.4.3.2.1. requestInitialized
2.4.3.2.2. requestDestroyed
2.4.3.3. javax.servlet.ServletContextAttributeListener : ServletContextAttributeEvent
2.4.3.3.1. attributeAdded
2.4.3.3.2. attributeRemoved
2.4.3.3.3. attributeReplaced
2.4.3.4. javax.servlet.ServletRequestAttributeListener : ServletRequestAttributeEvent
2.4.3.4.1. attributeAdded
2.4.3.4.2. attributeRemoved
2.4.3.4.3. attributeReplaced
2.4.3.5. javax.servlet.http.HttpSessionAttributeListener : HttpSessionBindingEvent
2.4.3.5.1. attributeAdded
2.4.3.5.2. attributeRemoved
2.4.3.5.3. attributeReplaced
2.4.3.6. javax.servlet.http.HttpSessionListener : HttpSessionEvent
2.4.3.6.1. sessionCreated
2.4.3.6.2. sessionDestroyed
2.4.3.7. javax.servlet.http.HttpSessionBindingListener : HttpSessionBindingEvent
2.4.3.7.1. valueBound
2.4.3.7.2. valueUnbound
2.4.3.8. javax.servlet.http.HttpSessionActivationListener : HttpSessionEvent
2.4.3.8.1. sessionDidActive
2.4.3.8.2. sessionWillPassivate
2.5. Attributes
2.5.1. Types
2.5.1.1. Application/context
2.5.1.2. Session
2.5.1.3. Request
2.5.2. setAttribute(String name, Object value)
2.5.3. getAtrribute(String) : Object
2.5.4. removeAttribute(String) : void
2.5.5. getAttributeNames() : Enumeration
2.6. Parameters
2.6.1. Types
2.6.1.1. Application/context init parameters
2.6.1.2. Request Paratemers
2.6.1.3. Servlet Iinit parameters
2.6.2. You CANNOT set Application and Servlet init parameters, they're set in the DD
2.6.3. getInitParameter(String) : String
2.7. RequestDispatcher
2.7.1. Methods
2.7.1.1. forward(ServletRequest, ServletResponse)
2.7.1.2. include(ServletRequest, ServletResponse)
2.7.2. RequestDispatcher view = request.getREquestDispatcher("result.jsp");
2.7.3. RequestDispatcher view = getServletContext().getRequestDispatcher("/result.jsp);
2.7.4. a flush often lead to an illegalStateException
2.7.5. getAttribute("javax.servlet.forward.query_string"); obtain the query string for the original access
2.7.6. if your servlet use an RD, it can never send its own response.
3. Chap 6. Session Managment
3.1. URL rewriting
3.1.1. Adds the session ID to the end of all URLs in the HTML that you write to the response
3.1.2. Is used to pass over the session ID when cookies are not supported but you need to explicitily encode all of the URLs you write
3.1.3. There's no way to get automatic URL rewriting with your static pages, so if you depend on sessions, you must use dynamically-generated pages
3.2. javax.servlet.http.HttpSession
3.2.1. getAttribute(String) : Object
3.2.2. getCreationTime() : long
3.2.3. getId() : String
3.2.4. getLastAcessedTime() : long
3.2.5. getMaxInactiveInterval(): int
3.2.6. getServletContext() : ServletContext
3.2.7. invalidate()
3.2.8. isNew(): boolean
3.2.9. removeAttribute(String)
3.2.10. setAttribute(String, Object)
3.2.11. setMaxInactiveInterval(int)
3.3. HttpServletRequsert
3.3.1. getSession()
3.3.2. getSession(boolean create new session if not exist)
3.4. Sessions
3.4.1. SSL has a built-in mechanism to define a session
3.4.2. URL rewriting
3.4.3. Cookies, the cookie name must be JSESSIONID
3.5. Ways a session die
3.5.1. Times out
3.5.1.1. <web-app> <session-config> <session-timeout>15</...
3.5.1.2. session.setMaxInactiveInterval(15*60);
3.5.2. invalidate()
3.5.3. App goes down
3.6. javax.servlet.http.Cookie
3.6.1. Cookie(String name, String value)
3.6.2. getDomain() : String
3.6.3. getMaxAge(): int
3.6.4. getName(): String
3.6.5. getPath() : String
3.6.6. getSecure(): boolean
3.6.7. getValue(): String
3.6.8. setDomain(String)
3.6.9. setMaxAge(int)
3.6.10. setPath(String)
3.6.11. SetValue(String)
3.7. Session Listeners
3.7.1. HttpSessionListener : HttpSessionEvent
3.7.1.1. sessionCreated
3.7.1.2. sessionDestroyed
3.7.2. HttpSessionActivationListener: HttpSessionEvent
3.7.2.1. sessionDidActive
3.7.2.2. sessionWillPassivate
3.7.3. HttpSessionBindingListener : HttpSessionBindingEvent
3.7.3.1. valueBound
3.7.3.2. valueUnbound
3.7.4. HttpSessionAttributeListener : HttpSessionBindingEvent
3.7.4.1. attributeAdded
3.7.4.2. attributeRemoved
3.7.4.3. attributeReplaced
4. Chap 7. Using JSP
4.1. Jsp
4.1.1. Import
4.1.1.1. <%@ page import="foo.*" %>
4.1.1.2. <%@ page import="foo.*,java.util.*" %>
4.1.2. Elements
4.1.2.1. <% %>
4.1.2.2. <%@ %>
4.1.2.3. <%= %>
4.1.2.4. <%! %>
4.1.3. Template text is just a fucking text =/
4.1.4. implicit objects
4.1.4.1. JspWriter : out
4.1.4.2. HttpServletRequest : request
4.1.4.3. HttpServletResponse : response
4.1.4.4. HttpSession: session
4.1.4.5. ServletContext : application
4.1.4.6. SerlvetConfig : config
4.1.4.7. Throwable : exception
4.1.4.8. PageContext : pageContext
4.1.4.9. Object : page
4.1.5. javax.servlet.jsp.JspPage
4.1.5.1. jspInit()
4.1.5.1.1. Access to
4.1.5.2. jspDestroy()
4.1.5.3. javax.servlet.jsp.HttpJspPage
4.1.5.3.1. _jspService()
4.1.6. Attributes
4.1.6.1. In a Servlet
4.1.6.1.1. Application: getServletContext().setAttribute("foo", obj);
4.1.6.1.2. Request: request.setAttribute("foo", obj);
4.1.6.1.3. Session: request.getSession().setAttribute("foo", obj);
4.1.6.1.4. Page: does not apply
4.1.6.2. In a JSP
4.1.6.2.1. Application : application.setAttribute("foo", obj)
4.1.6.2.2. Request: request.setAttribute("foo", obj)
4.1.6.2.3. Session : session.setAttribute("foo", obj)
4.1.6.2.4. Page: pageContext.setAttribute("foo", obj)
4.1.7. pageContext
4.1.7.1. Constants
4.1.7.1.1. APPLICATION_SCOPE
4.1.7.1.2. PAGE_SCOPE
4.1.7.1.3. REQUEST_SCOPE
4.1.7.1.4. SESSION_SCOPE
4.1.7.2. Methods to get any implicit object
4.1.7.2.1. getRequest()
4.1.7.2.2. getServletConfigs()
4.1.7.2.3. getServletContext()
4.1.7.2.4. getSession()
4.1.7.3. Inherited Methods from JspContext
4.1.7.3.1. getAttribute(String name)
4.1.7.3.2. getAttribute(String name, int scope)
4.1.7.3.3. getAttributeNameInScope(int scope)
4.1.7.3.4. findAttribute(String name)
4.1.8. Directives
4.1.8.1. page
4.1.8.1.1. Attributes
4.1.8.1.2. Attributes outside of the examn
4.1.8.2. taglib
4.1.8.3. include
4.1.9. Disable
4.1.9.1. scripting
4.1.9.1.1. <jsp-config> <jsp-property-group> <url-pattern>*.jsp <scriptin-invalid> true ....
4.1.9.2. EL
4.1.9.2.1. <jsp-config> <jsp-property-group> <url-patter>*.jsp.. <el-ignored> true
4.1.9.2.2. <% page isElIgnored="true">
4.1.10. Actions
4.1.10.1. Standar action : <jsp:include page="Foo.jsp"/>
4.1.10.2. Other action: <c:set var="rate" value="32" />
5. Chap 8. Scriptless JSP
5.1. EL
5.1.1. El expressions are always with curly braces, and prefixed with a dollar($) sign ${expression}
5.1.2. The first named variable in the expression is either an implicit object or an attribute in one of the four scopes (page, request, session or application)
5.1.3. The dot operator lets you access values by using a Map key or a bean property name. whatever comes to the right of the dot operator must follow normal Java naming rules
5.1.4. You can NEVER put anything to the right of the dot that wouldn't be legal as a Java identifire
5.1.5. The [] operator let you access arrays and lists
5.1.5.1. when retrive the entire list only the first element is displayed, initParam.list is the same as initParam.list[0]
5.1.6. If what's inside the brackets is not in quotes, the container evaluates it. if it's in quotes, and it's not an index into an array or list, the container sees it as the literal name of a property or key
5.1.7. All but one of the EL implicit objects are Maps (PageContext)
5.1.8. the implicit object request is represented by requestScope implicit object on EL
5.1.9. You can use TLD to call java methods
5.1.10. Alrays inside of [] should have "" if it's not a number
5.1.11. If the dot operator is used to access a bean property but the prperty doesn't exist, then a runtime exception is thrown
5.2. Bean-Related
5.2.1. <jsp:useBean>
5.2.1.1. Defines a variable that holds a reference to either an existing bean attribute or, if the bean doesn't already exist, create a new bean
5.2.1.2. MUST have an "id" attribute which declares the variable name
5.2.1.3. the "scope" attribute default is page
5.2.1.4. The "class" attribute is optional, and it declares the class type that will be used if a new bean is created. The type must be public, non-abstract and have no-args constructor
5.2.1.5. The "type" attribute must be a type to which the bean can be cast
5.2.1.6. if you have "type" attribute but not have a "class" the bean must already exist
5.2.1.7. This tag can have body, and anything in the body runs ONLY if a new bean is created
5.2.1.7.1. <jsp:setProperty>
5.3. Include
5.3.1. mechanisms
5.3.1.1. <%@ include FILE="header.html" %>
5.3.1.1.1. does the include at translation time, only once
5.3.1.2. <jsp:include PAGE="header.html" />
5.3.1.2.1. does the include at at runtime, every time!
5.3.1.2.2. can customize an included file by setting a request parameter using <jsp:param>
5.3.1.2.3. it's NOT possible to import the contents of any binary file into a JSP page
5.3.2. is position-sensitive directive
5.3.3. be sure to strip out the opening and closing tags.
5.3.4. Cannot change the response status code or set headers
5.4. Taglib declaration
5.4.1. <taglib> ... <function> <name>Some</.. <function-class>com.some.package.class</.. <function-signature>java.lang.String param (java.lang.String)</.. </... </...
5.5. <jsp:forward>
5.5.1. the response buffer is cleared first!, so anything written to the response before the forward will be thrown away
5.5.2. if you commit the response before the forwad (calling out.flush()), the forward won't happen, and the rest of the original page won't be processed
5.5.3. attribute Page
6. Chap 9. Using JSTL
6.1. Core Tags
6.1.1. <c:out />
6.1.1.1. Attributes
6.1.1.1.1. value
6.1.1.1.2. escapeXml attribute is optional, true as default
6.1.1.1.3. default attribute is optional, the body of the c:out tag also works as default attribute
6.1.2. <c:forEach>
6.1.2.1. Attributes
6.1.2.1.1. var
6.1.2.1.2. items
6.1.2.1.3. varSatus
6.1.2.2. can nest tags
6.1.3. <c:if>
6.1.3.1. Attributes
6.1.3.1.1. test
6.1.3.2. doesn't have an else
6.1.4. <c:choose>
6.1.4.1. <c:when>
6.1.4.1.1. test
6.1.4.2. <c:otherwise>
6.1.5. <c:set>
6.1.5.1. Variables
6.1.5.1.1. var
6.1.5.1.2. scope, default page
6.1.5.1.3. value, the body also works as value
6.1.5.2. Beans or Maps
6.1.5.2.1. target
6.1.5.2.2. property
6.1.5.2.3. value, the body also works as value
6.1.6. <c:remove>
6.1.6.1. attributes
6.1.6.1.1. var
6.1.6.1.2. scope, is optinoal if not exist remove from ALL
6.1.7. <c:import>
6.1.7.1. Attribute
6.1.7.1.1. url
6.1.7.1.2. value
6.1.7.1.3. var
6.1.7.2. add the contet from the value of url attribute to the current page, at request time (runtime) like jsp:include but it's more flexible
6.1.7.3. can reach outside of the web app
6.1.7.4. Body
6.1.7.4.1. <c:param>
6.1.8. <c:catch>
6.1.8.1. attirubte
6.1.8.1.1. var
6.1.9. <c:forTokens>
6.1.10. <c:url>
6.1.11. <c:redirect>
6.2. Errors
6.2.1. Manually
6.2.1.1. <%@ page isError="true">
6.2.1.1.1. ${pageContext.exception}
6.2.1.2. <%@ page errorPage="errorPage.jsp">
6.2.2. DD
6.2.2.1. <error-page> <exception-types>java.lang.Throwable</.. <location>/errorPage.jsp</... ...
6.2.2.2. <error-page> <exception-type>java.lang.ArithmeticException</... <location>/arimeticError.jsp</... ...
6.2.2.3. <error-page> <error-code>404</... <location>/notFoundError.jsp<... ....
6.3. TLD
6.3.1. declaration
6.3.1.1. <?xml ...> <taglib xmlns...> <tlib-verion>1.2</tlib-verion> //mandatory <short-name>Random</short-name>//mandatory <function> //to be called on EL <name>rollIt</name> <function-class>foo.DiceRoller</function-class> <function-signature>int rollDice()</function-signature> </function> <uri>randomThings</uri> //unique! <tag> <description>randome advice</description>//optional <name>advice</name>//Required <tag-class>foo.AdvisorTagHandler</tag-class>//required <body-contet>empty</body-contet>//required <attribute> <name>user</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> </tag> </taglib>
6.3.1.1.1. rtexprvalue, default is false
6.3.1.1.2. body-contet
6.3.1.1.3. uri
6.3.1.2. <taglib> <taglib-uri>randomThings</.. <taglib-location>/WEB-INF/tag.tld</.. </...
6.3.2. class
6.3.2.1. extends SimpleTagSupport
6.3.2.2. override void doTag()
6.3.2.3. all setters needed
6.3.3. usage
6.3.3.1. <%@ taglib prefix="calis" uri="randomThings" %> <mine:advice user="${username}">
6.3.4. The TLD file can be placed in any subdirectory of WEB-INF
7. Chap 10. Custom tag Development
7.1. Tag handlers
7.1.1. Classic
7.1.1.1. Extend TagSupport -> IterationTag -> Tag -> JspTag
7.1.1.2. Life
7.1.1.2.1. 1. Load class
7.1.1.2.2. 2. Instantiate class (no-args constructor runs)
7.1.1.2.3. 3. Call the SetPageContext(PageContext)
7.1.1.2.4. 4. if the tag is nested setParent(Tag)
7.1.1.2.5. 5. if the tag has attributes, call attributes setters
7.1.1.2.6. 6. doStartTag()
7.1.1.2.7. 7. if the tag is NOT declared to have an empty body, AND tag is NOT invoked with an empty body, AND the doStartTag() return EVAL_BODY_INCLUDE the body is evaluated
7.1.1.2.8. 8. if the body is evauated doAfterBody()
7.1.1.2.9. 9. doEndTag()
7.1.1.3. pageContext.getOutput().println
7.1.1.4. pageContext.implicitObjects
7.1.2. Classic BodyTag
7.1.2.1. extend BodyTagSupport (implement bodyTag -> iterationTag-> Tag-> JspTag )
7.1.2.2. Life
7.1.2.2.1. 1. Load class
7.1.2.2.2. 2. Instantiate class (no-args constructor runs)
7.1.2.2.3. 3. Call the SetPageContext(PageContext)
7.1.2.2.4. 4. if the tag is nested setParent(Tag)
7.1.2.2.5. 5. if the tag has attributes, call attributes setters
7.1.2.2.6. 6. doStartTag()
7.1.2.2.7. 7. setBodyContet() if Eval_body_buffered was returned
7.1.2.2.8. 8. doInitBody()
7.1.2.2.9. 9. if the tag is NOT declared to have an empty body, AND tag is NOT invoked with an empty body, AND the doStartTag() return EVAL_BODY_INCLUDE the body is evaluated
7.1.2.2.10. 10. if the body is evauated doAfterBody()
7.1.2.2.11. 11. doEndTag()
7.1.3. Simple
7.1.3.1. Extend SimpleTagSupport --> SimpleTag --> JspTag
7.1.3.2. To deploy it, must crate a TLD that describes the tag using the same <tag> element
7.1.3.3. to use it with body, make sure the TLD <tag> for this tag does not declare <body-contet>empty. then call getJspBody().invoke(null) to cause the body to be processed
7.1.3.4. Life
7.1.3.4.1. 1. Load class
7.1.3.4.2. 2. Instantiate class (no-args constructor runs)
7.1.3.4.3. 3. Call setJspContext(jspContext)
7.1.3.4.4. 4. If the tag is nested, call the setParent(JspTag)
7.1.3.4.5. 5. If the tag has attributes, call attribute setters
7.1.3.4.6. 6. if the tag is NOT decalred to have a <body-contet>empty.... and the tag has a body, call setJspBody(JspFragment)
7.1.3.4.7. 7. doTag()
7.1.3.5. can set attribute used by the body of the tag, calling getJspContext().setAttribute() followed by getJspBody().invoke()
7.1.3.6. You can iterate over the body invoking the body (getJspBody.invoke()) in a loop
7.1.3.7. the getJspBody() return a JspFragment
7.1.3.7.1. methods
7.1.3.8. Throw a SkipPageException if you want the current page to stop processing, if the page that invoked the ta was included from another page, the including page keeps going even though the included page stops processin from the moment the exception is thrown
7.1.3.9. getJspContext().getOutput().println
7.1.3.10. getJspContext().implicitobjects
7.2. Tag
7.2.1. Apply only to tags
7.2.1.1. tag
7.2.1.2. taglib
7.2.1.3. jsp:doBdoy
7.2.2. directives but page
7.2.3. NOT Scripting
7.2.4. jsp:invoke
7.3. DynamicAttributes Interface
7.3.1. method
7.3.1.1. setDynamicAttribute(String uri, String name, object, value)
7.3.2. <tag> <attribute>... <dynamic-attribute>true</dynamic-attribute> ...
7.3.3. <%@ tag body-contet="empty" dynamic-attribute="tagAttrs">
7.4. PageContext
7.4.1. extend from JspContext
7.4.1.1. mehtods
7.4.1.1.1. getAttributes(name)
7.4.1.1.2. getAttribute(name, int scope)
7.4.1.1.3. getAttributeNamesInScope(int scope)
7.4.1.1.4. findAttribute(name)
7.4.2. methods
7.4.2.1. getRequest()
7.4.2.2. getServletConfig()
7.4.2.3. getServletCnontext()
7.4.2.4. getSession()
7.4.3. Scopes
7.4.3.1. Application
7.4.3.2. Page
7.4.3.3. Request
7.4.3.4. Session
8. Chap 11. Web App Deployment
8.1. Directory Structure
8.1.1. Webapps
8.1.1.1. NameofTheApp
8.1.1.1.1. *.jsp *html
8.1.1.1.2. WEB-INF
8.1.2. War
8.1.2.1. Webapps
8.1.2.1.1. NameofTheApp
8.2. Mapping
8.2.1. <servlet> <servlet-name>Beer</servlet-name> <servlet-class>com.example.BeerSelect</servlet-class> </servlet> <servlet-mapping> <servlet-name>Beer</... <url-pattern>/Beer/SelectBeer.do</.. ...
8.2.2. Rules
8.2.2.1. one Match
8.2.2.1.1. 1. exact Match
8.2.2.1.2. 2. directory match
8.2.2.1.3. 3 extension match
8.2.2.2. multiples match
8.2.2.2.1. choose the longest mapp
8.3. Welcome file
8.3.1. <welcome-file-list> <welcome-file>index.html</... <welcome-file>default.jsp</.. </...
8.4. initialization
8.4.1. <servlet> <servlet-name>... <servlet-class>... <load-on-startup> number </.. ...
8.5. JSP Document (XML-based dcument)
8.5.1. Directives (except taglib)
8.5.1.1. <%@ page import="java.util.*" %>
8.5.1.2. <jsp:directive.page import="java.util.*"/>
8.5.2. Declaration
8.5.2.1. <%! int y=3; %>
8.5.2.2. <jsp:declaration> int y = 3; </jsp:declaration>
8.5.3. Scriptlet
8.5.3.1. <%list.add("foo"); %>
8.5.3.2. <jsp:scriptlet> list.add("foo"); </jsp:scriptlet>
8.5.4. text
8.5.4.1. Simple text
8.5.4.2. <jsp:text> Simple text </jsp:text>
8.5.5. scripting expression
8.5.5.1. <%= it.next() %>
8.5.5.2. <jsp:expression> it.next() </jsp:expression>
8.6. EJB
8.6.1. local
8.6.1.1. <ejb-local-ref> <ejb-ref-name>ejb/Customer</.. <ejb-ref-type>Entity</.. <local-home>org.some.CustomerHome</... <local>org.some.Customer</... ......
8.6.2. remote
8.6.2.1. <ejb-ref> <ejb-ref-name>ejb</... <ejb-ref-type>Entitiy</... <home>org.some.CustomerHome</.. <remote>org.some.Customer</.. ...
8.7. env-entry
8.7.1. <env-entry> <env-entry-name>rates/discountRate</.... <env-entry-type>java.lang.Integer</.. <env-entry-value>10</... ....
8.8. Mime
8.8.1. <mime-mapping> <extension>mpg</... <mime-type>video/mpeg</... .......
9. Chap 12. Web App Security
9.1. Realm: place where authentication information is stored
9.2. Authorization
9.2.1. Step 1. Defining roles
9.2.1.1. tomcat-user.xml
9.2.1.1.1. <tomcat-users> <role rolename="Admin"/> <role rolename="Member"/> <role rolename="Guest"/> <user username="Guti" password="secret" roles="Admin, Member, Guest"/> <user username="Ted" password="Tedsecret" roles="Guest"/> ...........
9.2.1.2. DD
9.2.1.2.1. <security-role><role-name>Admin</role-name></security-role> <security-role><role-name>Member</role-name></security-role> <security-role><role-name>Guest</role-name></security-role>
9.2.1.2.2. <login-config> <auth-method>BASIC</... </...
9.2.2. Sept 2. Defining resource/method constrains
9.2.2.1. DD
9.2.2.1.1. <web-app> .... <web-resource-collection>
9.2.2.1.2. <web-resource-collection>
9.2.2.1.3. <web-resource-name>UpdateRecipes</..
9.2.2.1.4. <url-pattern>/Beer/AddRecipe/*</... <url-pattern>/Beer/ReviewRecipe/*</...
9.2.2.1.5. <http-method>GET</... <http-method>POST</....
9.2.2.1.6. </web-resource-collection>
9.2.2.1.7. <auth-constraint> <role-name>Admin</.. <role-name>Member</.. </....
9.2.2.1.8. <user-data-constraint> <transport-guarantee>CONFIDENTIAL</... </...
9.2.2.1.9. </security-constraint> </web-app>
9.3. Multiples <security-constraint>
9.3.1. 1. when combinig individual role names, all of the roles names listed will be allowed
9.3.2. 2. A rle name of "*" combines with anything else to allow acces to everybody
9.3.3. 3. An empty <auth-constraint> tag combines with anything else to allow access to nobody, empty tag is always final word, nobody allowed
9.3.4. 4. if one <securty-constraint> has no <auth-constraint>, combines with anything else to allow access to everybody
9.4. Authentication types
9.4.1. BASIC
9.4.2. DIGEST
9.4.3. CLIENT-CERT
9.4.4. FORM
9.4.4.1. <login-config> <auth-method>FORM</.. <form-login-config> <form-login-page>/loginPage.html</.. <form-error-page>/loginError.html</.. </.... </....
9.4.4.2. action= j_security_check j_username j_password
9.5. HttpServletRequest
9.5.1. isUserInRole("roleName")
9.5.2. getRemoteUser
9.5.3. getUserPrincipal()
10. Chap 13. Wrappers and Filters
10.1. javax.servlet.Filter
10.1.1. Methods
10.1.1.1. init(FilterConfig config)
10.1.1.2. doFilter(ServletRequest, ServletResponse, FilterChain)
10.1.1.3. destroy()
10.1.2. Filters have no idea who's going to call them or who's next in line
10.2. Declaring (DD)
10.2.1. <filter> <filter-name>BeerRequest</.. <filter-class>com.example.SomeFilter</.. <init-param> <param-name>logFile</.. <param-value>Log.txt</... </init... ...
10.2.2. <filter-mapping> <filter-name>BeerRequest</.. <url-pattern>*.do</... ...
10.2.3. <filter-mapping> <filter-name>BeerRequest</.. <servlet-name>AdviceServlet</... ...
10.2.4. <dispatcher>
10.2.4.1. REQUEST, default
10.2.4.2. INCLUDE
10.2.4.3. FORWARD
10.2.4.4. ERROR
10.3. Decorator/Wrapper
10.3.1. wraps one kind of an object with an "enhanced" implementations (add new capabilities)
10.3.2. not necessary needs to override methods
10.4. Order rule
10.4.1. 1. All matching Url patterns
10.4.2. 2. Servlet-name
11. Chap 14. Patterns and Struts
11.1. Business Delegate
11.1.1. Features
11.1.1.1. Acts as a proxy, implementing the remote service's interface
11.1.1.2. initiates communications with a remote service
11.1.1.3. handles communication details and exceptions
11.1.1.4. receives request from a controller component
11.1.1.5. translates the request and forwards it to the business services
11.1.1.6. translate the response and returns it to the controller component
11.1.1.7. by handling the details of remote component lookup and communications, allows controllers to be more cohesive
11.1.2. Principales
11.1.2.1. based on
11.1.2.1.1. hiding complexity
11.1.2.1.2. coding to interfaces
11.1.2.1.3. loose copuling
11.1.2.1.4. separation of concerns
11.1.2.2. minimizes the impact on the web tier when changes occur on the buisiness tier
11.1.2.3. Reduces coupling between tiers
11.1.2.4. Adds a layer to the app, which incrases complexity
11.1.2.5. Methods calls to the Buisness Delegate should be a coarse-grained to reduce network traffic
11.2. Service Locator
11.2.1. Features
11.2.1.1. Obtains InitialContext objects
11.2.1.2. Performs registry lookups
11.2.1.3. Handles communication details and exceptions
11.2.1.4. Can improve perfomance by caching previously obtained references
11.2.1.5. Works with a variety of registries such as: JNDI, RMI, UDDI, and COSS naming
11.2.2. Principales
11.2.2.1. based on
11.2.2.1.1. hiding complexity
11.2.2.1.2. separation of concerns
11.2.2.2. minimize the impact on the web tier when remote components change locations or containers
11.2.2.3. Reduce coupling between tiers
11.3. Transfer Object
11.3.1. functions
11.3.1.1. provides a local representation of a remote entity
11.3.1.2. minimize network traffic
11.3.1.3. can follow java bean conventions so that it can be easily accessed by other objects
11.3.1.4. implemented as a serializable objects so that it can move across the network
11.3.1.5. typically easily accessible by view components
11.3.2. principales
11.3.2.1. based on reducing network traffic
11.3.2.2. minimize the performance impact on the web tier when remote components data is accessed with fine-grained calls
11.3.2.3. Reduces coupling between tiers
11.3.2.4. a drawback is that components accessing the Transfer Object can receive out-of-data data, because transfer object's data is really representing sate that's stored somewhere elese.
11.3.2.5. Making updatable transfer objects concurency-safe is typcally complex
11.4. Intercepting Filter
11.4.1. functions
11.4.1.1. can intercept and/or modify request before they reach the servlet
11.4.1.2. can intercept and/or modify the response before they are returned to the client
11.4.1.3. Filters are deployed declaratively using the DD
11.4.1.4. Filters are modular so that they can be exceuted in chains
11.4.1.5. Filters have lifecycles managed by the container
11.4.1.6. Filter must implemente Container callback methods
11.4.2. principales
11.4.2.1. based on
11.4.2.1.1. cohesion
11.4.2.1.2. lose coupling
11.4.2.1.3. increasing declarative control
11.4.2.2. delcarative control allow filters to be easily implemented on either a temporary or permanent basis
11.4.2.3. declarative control allows the sequence of invocation to be easily updated
11.5. Model View, Controller
11.5.1. features
11.5.1.1. views can change independtly from controllres and model
11.5.1.2. model components hide interal details, from the view and controllers
11.5.1.3. if the model adheres to a stric contract,then these components can be reused in other app
11.5.1.4. separation fo model code from controller code allows for easier migration to using remote buisness components
11.5.2. principales
11.5.2.1. based on
11.5.2.1.1. separation of concerns
11.5.2.1.2. loose coupling
11.5.2.2. increase cohesion in inividual components
11.5.2.3. increase the overall complexity of the application
11.5.2.4. minimize the impact of changes in other tiers of the application
11.6. Fron controller
11.6.1. features
11.6.1.1. centralize a web app's initial request handling tasks in a single component
11.6.1.2. using the fron controller with other patterns can provide loose coupling by making presentation tier dispatching declarative
11.6.1.3. a drawback of front controller is that is' very barebons compared to strus
11.6.2. principales
11.6.2.1. based on
11.6.2.1.1. hiding complexity
11.6.2.1.2. separation of concerns
11.6.2.1.3. loose coupling
11.6.2.2. increases cohesin in application controller components
11.6.2.3. decreases the overall complexity of the application
11.6.2.4. increases the maintainability of the inferastructure code