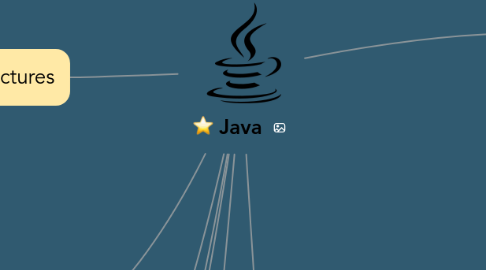
1. features
1.1. Simple
1.2. Dynamic
1.3. Interpreted
1.4. Portable
1.5. High Performance
1.6. Multi Threading
1.7. Platform Independent
1.8. Robust
1.9. Secured
1.10. Distributed
1.11. Object Oriented
1.12. Architectural Neutral
2. Collection
2.1. Map, List & Set
2.2. Equans-Hashcode
2.3. Legacy-Synchronized Classes
3. JVM
3.1. Stack vs Heap Memory
3.2. Class loaders
3.3. JRE, JVM, JDK
4. OPP
4.1. Classes
4.1.1. Lambda Expressions
4.1.2. Generics
4.1.3. Enum
4.1.4. Anonymous
4.1.5. Nested
4.1.6. Static
4.1.7. Final
4.1.8. Package
4.2. Objects
4.2.1. Common methods
4.2.1.1. Equals
4.2.1.2. HashCode
4.2.1.3. toString
4.2.1.4. notify
4.2.1.5. wait
4.2.1.6. notifyAll
4.3. Encapsulation
4.3.1. Access modifiers
4.3.1.1. public
4.3.1.2. privat
4.3.1.3. protected
4.3.1.4. package
4.4. Polimorphism
4.4.1. Overloading
4.4.2. Overriding
4.4.3. Runtime
4.4.4. Static
4.4.5. Casting
4.4.5.1. Downcasting
4.4.5.2. Upcasting
4.5. Inheritance
4.5.1. Interfaces
4.5.2. Abstract Classes
5. Exception
5.1. Checked Vs Unchecked Exceptions
5.2. Exception handling best practices
5.3. try, catch, finally, throw & throws
6. Language Basics
6.1. Arrays
6.2. Primitive types
6.3. Key words
6.4. Operators
6.4.1. Unary
6.4.2. Equality
6.4.3. Conditional
6.4.4. Bitwise
6.4.5. Arithmetic
6.4.6. Type comparison
6.5. Numbers and String
6.5.1. Number
6.5.2. String
6.6. Exceptions
6.6.1. Try Catch
6.6.2. Finally block
6.6.3. Throws
7. Structures
7.1. Suggested
7.1.1. Documentation Section
7.2. Optional
7.2.1. Package Statement
7.2.2. Import Statement
7.2.3. Interface Statement
7.2.4. Class Definition
7.3. Essential
7.3.1. Main Method Definition
8. Network
8.1. IP Address
8.2. Protocol
8.2.1. TCP
8.2.2. FTP
8.2.3. Telnet
8.2.4. SMTP
8.2.5. POP etc.
8.3. Port Number
8.4. MAC Address
8.5. Connection- oriented and connection less protocol
9. Applications
9.1. Desktop GUI Applications
9.2. Enterprise Applications
9.3. Distributed Applications
9.4. Scientific Applications
9.5. Trading Applications
9.6. Web-based Applications
9.7. Cloud-based Applications
9.8. Web Servers and Applications Servers
9.9. Big Data Technologies
9.10. Gaming and Animation
9.11. Software Tools
9.12. Mobile Applications Development
10. Operators
10.1. Arithmetic Operators
10.1.1. Multiplication
10.1.1.1. *
10.1.2. Addition
10.1.2.1. +
10.1.3. Subtraction
10.1.3.1. -
10.1.4. Division
10.1.4.1. /
10.1.5. Modulus
10.1.5.1. %
10.2. Logical Operators
10.2.1. AND
10.2.1.1. &&
10.2.2. OR
10.2.2.1. II
10.3. Unary Operators
10.3.1. Postfix
10.3.1.1. a++ , a--
10.3.2. Prefix
10.3.2.1. II
10.3.2.2. ++a , --a
10.4. Ternary Operators
10.5. Shift Operators
10.6. Assignment Operators
10.6.1. Bitwise AND
10.6.2. Bitwise exclusive OR
10.6.3. Bitwise inclusive OR
10.7. Relation Operators
10.7.1. Comparison
10.7.2. equality
11. Data Types
11.1. Boolean Type
11.1.1. Boolean
11.2. Numeric Type
11.2.1. Character Value
11.2.1.1. float
11.2.1.2. double
11.2.2. integral value
11.2.2.1. Integer
11.2.2.1.1. byte
11.2.2.1.2. short
11.2.2.1.3. int
11.2.2.1.4. long
11.2.2.2. C
11.2.2.2.1. float
11.2.2.2.2. double
12. Core API
12.1. Annotations
12.1.1. Retention
12.1.1.1. Source
12.1.1.2. Runtime
12.1.1.3. Class
12.1.2. Target
12.1.3. Inherited
12.2. IO
12.2.1. IO streams
12.2.1.1. Byte streams
12.2.1.2. Buffered stream
12.2.1.3. Data stream
12.2.2. File
12.2.2.1. Random Access File
12.3. NIO
12.3.1. Buffer
12.3.2. Channel
12.3.3. Selector
12.4. Reflection
12.5. Concurrency
12.5.1. Process
12.5.2. Thread
12.5.2.1. Creating
12.5.2.2. Lifecycle
12.5.2.3. Join
12.5.2.4. Interrupt
12.5.2.5. Lock
12.5.2.6. Executors
12.5.2.7. Fork Join
12.5.2.8. Atomic types
12.6. Utill
12.6.1. collection
12.6.2. Random Access
12.6.3. Map
12.6.4. String Joiner
12.6.5. Optional
12.6.6. Objects
12.7. Lang
12.7.1. Marker interfaces
12.7.2. Number
12.7.3. String
12.7.3.1. String Builder
12.7.3.2. String Buffer
12.7.4. System
12.7.5. Process
12.7.5.1. Process Builder
12.7.6. Runtime
12.7.7. Thread
12.7.8. Class Loader
12.7.9. Throwable
12.7.9.1. Exception
12.7.9.1.1. Runtime Exception
12.7.9.2. Error
12.8. XML
12.8.1. DOM
12.8.2. SAX
12.8.3. STAX
12.8.4. JAXP
12.9. Date
12.10. Networking
12.10.1. Socket
12.10.2. Datagram
12.11. Security
12.11.1. Signature
12.11.2. Certificate
12.11.3. Key
12.11.4. Message Digest