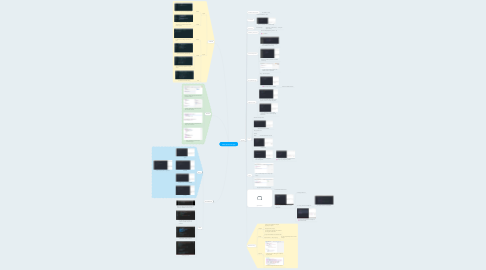
1. Classes
1.1. Declaring classes and properties within the constructor method
1.2. methods added with es6 function syntax and OO bock structure.
1.3. extends works under the requirement of using super constructor
1.4. static methods as any other object oriented language
2. modules
2.1. export
2.1.1. default
2.1.1.1. every file can only have one defautlt export
2.1.2. named
2.1.2.1. you can export functions with names associated or constants using the arrow function syntax..
2.2. import
2.2.1. default
2.2.1.1. as default you can assign any name you want
2.2.2. named
2.2.2.1. import named using destructuring
2.2.2.2. import all named properties in a super object
2.2.3. both
2.2.3.1. first default, then destructuring
3. New Methods
3.1. String
3.1.1. repeat
3.1.2. startsWIth
3.1.2.1. it also accepts a position argument.
3.1.3. endsWith which works as startsWith
3.1.4. includes
3.2. fetch
3.2.1. fetch is not es6 but a browser mehtod that makes ajax callbacks much more easier
3.2.2. use response.json to get the Json response and add a new promise resolution
3.2.3. add method, headers, body etc
3.2.4. checking for errors
4. Syntax
4.1. Variable Devlaration
4.1.1. let varName = value
4.2. constants
4.2.1. const constantName = value
4.2.2. can be redeclared within a smaller scope
4.3. functions
4.3.1. like coffeescript
4.3.1.1. (parameter1, paramter2)=>{ .... body with implicit return }
4.4. defatult paramters
4.4.1. function example(param1, param2 = 10)
4.4.2. excellent
4.5. spread Operator
4.5.1. ...array to explode the elements so they can be added to another array
4.5.2. also can be used to paramters in functions from arrays.
4.5.3. you can used to handle functions with variable number of paramters
4.6. templateStrings
4.6.1. using `` instead of quotes
4.6.2. embed expresions or variables
4.6.2.1. makes concatenation easiee
4.7. ObjectLiterals
4.7.1. you can use variable name as key to set a property withe the corresponding value.
4.7.2. function declaration are more object oriented so you don't need to explicitly define them.
4.8. sets
4.8.1. collection of unique items
4.8.2. .add method can be chained
4.8.3. .size() prints the size
4.8.4. .delete()
4.8.5. .clear()
4.8.5.1. removes all elements in the set.
4.8.6. .has(element(
4.8.7. you can create a set from an array so it removes duplicates
4.8.7.1. You can also create an array again from the set by using the spread operator
4.9. maps
4.9.1. you can create a map from an array or just empty
4.9.2. with get and set to manage content
4.10. generators
4.10.1. Controlled executed functions
4.10.2. once you call them the first time it returns the iterator
4.10.2.1. on next() you advance
4.10.2.2. you can put yield as the breakpoints
4.10.2.2.1. you can also store the result ASYNC
4.10.2.3. you can pass values to the breakponts execution WOW
4.11. Destructuring
4.11.1. Objects
4.11.1.1. create a new variable from a keyed property in an object
4.11.1.2. let {key:newVar} = object;
4.11.1.3. you can skip new Var and it will create the var using the variable name
4.11.2. Arrays
4.11.2.1. you put the variables for the element index
4.11.2.2. let {first,second, ...rest} = myArray;
4.11.2.2.1. you can use spread operator to collect multiple
4.11.3. Imports
4.11.3.1. when you import a library you can specify the properties.
4.11.3.2. this is the comparission between es5 es6