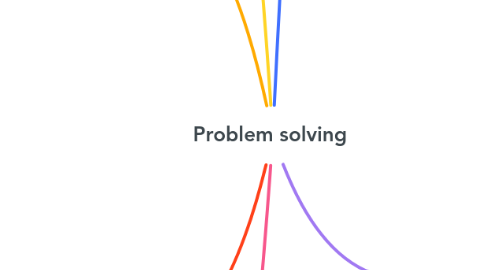
1. Compitational thinking
1.1. Computational thinking is the process of approaching a problem in a systematic manner and creating and expressing a solution such that it can be carried out by a computer.
1.1.1. Thinking procedurally: Procedural thinking is a disciplined method of thinking in sequence, in order and logically. Procedural thinking can be reflected in a flow chart. For example, following a recipe.
1.1.2. Thinking logically: Logical thinking is the act of analyzing a situation and coming up with a sensible solution. Similar to critical thinking, logical thinking requires the use of reasoning skills to study a problem objectively, which will allow you to make a rational conclusion about how to proceed.
1.1.3. Thinking ahead: to prepare for a future event or situation by thinking about what might happen.
2. Pseudocode
2.1. Pseudocode is a combination of two words: Pseudo and Code. “Pseudo” means imitation and “code” refers to instruction written in the programming language. Pseudo code is not a real programming code. It is the generic (informal) way of describing an algorithm without using any specific programming language-related notations.
2.2. Characteristics
2.2.1. • Named variables represent data and identifiers denote higher level functions. • Composed of a sequence of statements or steps. • Statements are often numbered sequentially. • Operational (Imperative) statements include assignment, input, and output. • Control structures provide iterative and conditional execution. • Indentations used for grouping blocks of statements. • There is no fixed syntax. Clarity of expression, rather than rigidity, is the essence of pseudocode.
3. Programming language
3.1. A programming language is a computer language programmers use to develop software programs, scripts, or other sets of instructions for computers to execute.
3.1.1. Low-level programming language and example: A low-level language is a type of programming language that contains basic instructions recognized by a computer. It is often cryptic and not human-readable.
3.1.1.1. Examples: assembly language and machine language.
3.1.2. High- level programming language and example: A high-level language is a programming language designed to simplify computer programming. It is "high-level" since it is several steps removed from the actual code run on a computer's processor. High-level source code contains easy-to-read syntax that is later converted into a low-level language, which can be recognized and run by a specific CPU.
3.1.2.1. Examples: Java, Phyton
4. Sources
4.1. https://www.coursera.org/learn/computational-thinking-problem-solving https://computersciencewiki.org/index.php/Procedural_thinking#:~:text=Procedural%20thinking%20is%20a%20disciplined,follow%20the%20steps%20in%20order https://www.indeed.com/career-advice/career-development/strengthen-logical-thinking-skills#:~:text=Logical%20thinking%20is%20the%20act,conclusion%20about%20how%20to%20proceed https://www.merriam-webster.com/dictionary/think%20ahead#:~:text=%3A%20to%20prepare%20for%20a%20future,ahead%20and%20brought%20an%20umbrella . https://www.unf.edu/~broggio/cop2221/2221pseu.htm#:~:text=Pseudocode%20is%20an%20artificial%20and,%2C%20for%2C%20if%2C%20switch . https://launchschool.com/books/ruby/read/variables https://techterms.com/definition/algorithm https://launchschool.com/books/ruby/read/arrays#whatisanarray https://kullabs.com/class-12/computer-science-2/algorithm-and-flowchart-2/pseudocode-and-algorithm https://launchschool.com/books/ruby/read/loops_iterators#simpleloop https://www.lucidchart.com/pages/what-is-a-flowchart-tutorial https://www.bbc.co.uk/bitesize/guides/zwmbgk7/revision/5 https://courses.cs.vt.edu/~cs1104/Algorithms/algorithm_6.html https://www.computerscience.gcse.guru/theory/pseudocodehttps://techterms.com/definition/process https://www.computerhope.com/jargon/p/programming-language.htm https://www.smartdraw.com/flowchart/flowchart-symbols.htm#:~:text=Flowcharts%20use%20special%20shapes%20to,are%20known%20as%20flowchart%20symbols . https://www.visual-paradigm.com/tutorials/flowchart-tutorial/ https://techterms.com/definition/low-level_language https://www.bbc.co.uk/bitesize/guides/zc6s4wx/revision/5 https://techterms.com/definition/high-level_language https://study.com/academy/lesson/what-is-an-algorithm-definition-examples.html#:~:text=One%20of%20the%20most%20obvious,the%20back%20of%20the%20box .
5. Flowchart
5.1. A flowchart is a diagram that depicts a process, system or computer algorithm. They are widely used in multiple fields to document, study, plan, improve and communicate often complex processes in clear, easy-to-understand diagrams.
5.1.1. Flowchart shapes: Flowcharts, sometimes spelled as flow charts, use rectangles, ovals, diamonds and potentially numerous other shapes to define the type of step, along with connecting arrows to define flow and sequence.
5.1.1.1. Oval: Represents a start or end point.
5.1.1.2. Arrows: Connector which shows relationships between the representative shapes.
5.1.1.3. Parallelogram: Represents input or output
5.1.1.4. Rectangle: Represents a process
5.1.1.5. Diamond: Represents a decision
6. Algorithm
6.1. They are created as functions that serve as small programs that can be referenced by a larger program.
6.1.1. Input: Programs are written to solve problems. To solve a problem, a program needs data input and data, or information, output. Data can be input in different ways: Written directly into the program. This is called hard coding. By the user when the program is running. From a file or other source when the program is running.
6.1.2. Output: Once data has been processed, programs often need to output the data they have generated. In Python, the ‘print’ statement is used to output data. This program uses the ’print’ statement to:
6.1.2.1. Display a message explaining what information is being output. Text is placed within quotes.
6.1.2.2. Output the contents of the variable ‘perimeter’. Variables are not placed within quotes.
6.1.3. Process: A process is a program that is running on your computer. This can be anything from a small background task, such as a spell-checker or system events handler to a full-blown application like Internet Explorer or Microsoft Word.
6.1.4. Variable: Variables are used to store information to be referenced and manipulated in a computer program. They also provide a way of labeling data with a descriptive name, so our programs can be understood more clearly by the reader and ourselves.
6.1.5. Constant: Data values that stay the same every time a program is executed are known as constants. Constants are not expected to change.
6.1.6. Literal constants are actual values fixed into the source code. An example of this might be the character string "hello world". The data value "hello world" has been fixed into the code.
6.1.7. Named constants are values where a name is defined to be used instead of a literal constant. An example of this might be stating that the 'starting level' of a game is always referred to as 1.
6.1.8. Operators: An operator is a character or characters that determine the action that is to be performed or considered. There are three types of operators that programmers use: arithmetic operators. relational operators. logical operators.
6.1.9. Loops: A loop is the repetitive execution of a piece of code for a given amount of repetitions or until a certain condition is met.
6.1.10. Arrays: Arrays are what we call indexed lists. That means that each slot in an array is numbered. You can reference any element by its index number. The syntax to do this is typing the array name with the index in brackets [] directly following.
6.2. Examples:
6.2.1. One of the most obvious examples of an algorithm is a recipe. It's a finite list of instructions used to perform a task. For example, if you were to follow the algorithm to create brownies from a box mix, you would follow the three to five step process written on the back of the box.
6.2.2. One of the most common uses for algorithms is in computer science. Computers can't do anything without being told what to do. Algorithms allow us to give computers step-by-step instructions in order to solve a problem or perform a task.
6.2.3. When we get dressed Since getting dressed involves putting on various items of clothing, we'll need a subroutine put_on, that takes as input a variable of type clothing. Variables of type clothing may be any particular item of clothing available to you -- in other words, what's in your closet.