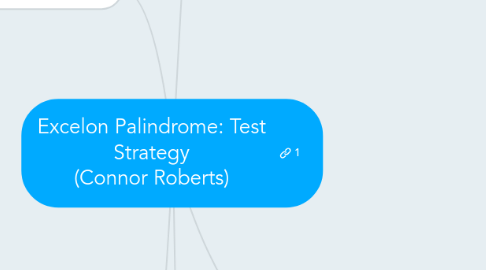
1. Consistency
1.1. Familiarity
1.2. Explainability
1.3. World
1.4. History
1.5. Image
1.6. Comparable Products
1.7. Claims
1.8. Users’ Desires
1.9. Product
1.10. Purpose
1.11. Statutes and Standards
2. Product Elements
2.1. Structure
2.2. Function
2.3. Data
2.4. Interfaces
2.5. Platform
2.6. Operations
2.7. Time
3. Other Questions/Concerns
3.1. External Resources
3.1.1. Do we have access to the development team that last worked on this/originally made it?
4. Test Techniques
4.1. Functional Testing
4.2. Domain Testing
4.2.1. Data input concerns?
4.2.1.1. Alpha only
4.2.1.2. Alpha-Numeric
4.2.1.3. Special Characters
4.2.1.3.1. !@#$%^ etc.
4.2.1.3.2. Spaces
4.2.1.3.3. UTF-8 compat? (e.g. pictorial language support)
4.2.1.4. Casing
4.2.1.4.1. Upper
4.2.1.4.2. Lower
4.2.1.4.3. Mixed
4.2.1.5. Length
4.2.1.5.1. Minimum
4.2.1.5.2. Maximum
4.2.1.6. Numeric only
4.2.1.7. What happens when I submit the same answer multiple times?
4.2.1.8. What if left blank and submitted?
4.3. Stress Testing
4.4. Flow Testing
4.5. Scenario Testing
4.6. Claims Testing
4.7. User Testing
4.8. Risk Testing
4.9. Automatic Checking
4.9.1. Find a list/txt file of existing pailindromes. Use a tool to iterate through them all, auto-refreshing the page and grabbing user message to determine which are/aren't successful.
5. Quality Criteria
5.1. Capability
5.1.1. Data storage
5.1.1.1. Is this all being done client side, or are we storing answers in a DB/file on the server?
5.1.1.2. Are we using cookies? caching? etc.
5.1.1.3. Does refreshing the page lose currently input data or is that preserved?
5.2. Reliability
5.3. Usability
5.3.1. UX
5.3.1.1. What visual notifications/responses are given to the user?
5.3.1.1.1. When a palindrome is entered?
5.3.1.1.2. When a non-palindrome is entered?
5.3.1.2. After answer entry, does the form reset or does the user have to reset it?
5.3.1.3. Can I type in the field?
5.3.1.4. As I type, am I supposed to get any kind of live feedback? (autocomplete, form validation, etc.)
5.3.1.5. Should the submit button be disabled until I type in the minimum characters?
5.4. Charisma
5.5. Security
5.5.1. Security
5.5.1.1. What kind of transmission is used between the form and the server?
5.5.1.1.1. Does this need to be secure?
5.5.1.2. SSL/HTTPS?
5.5.1.3. Certificates?
5.6. Scalability
5.6.1. What other functions/abilities does this Palindrome feature need to do in the future? How easy will it be for those teams/devs to add on to it?
5.7. Compatibility
5.7.1. What other products need to digest data output by this program?
5.7.2. External
5.7.2.1. Multi-Browser
5.7.2.1.1. Mobile, Tablet, etc.
5.7.2.1.2. Desktop
5.8. Performance
5.8.1. Timing
5.8.1.1. Submit typical response at 11:59:00
5.8.1.2. Submit typical response at 12:00:00
5.8.1.3. Submit typical response at 23:59:59
5.8.1.4. Submit typical response at 00:00:00
5.8.2. How many asserts does the site need to serve at once (1/min, 1000/s, etc)?
5.9. Installability
5.10. Development
5.10.1. Analytics
5.10.1.1. What data do we have on current usage?
5.10.1.1.1. i.e. trends to drive the establishment of User Personas to better guide future testing
5.10.1.1.2. For example, if 80% of our users are consistently only ever putting in alpha-numeric characters only, then maybe the large portion of our testing should focus on that.
5.10.1.2. What bugs have been found/reported recently by other groups?
5.10.2. Code
5.10.2.1. Use RCRCRC model to address regression concerns (what areas of the code have been modified in these areas that we need to be concerned with)
5.10.2.1.1. Recent
5.10.2.1.2. Core
5.10.2.1.3. Risky
5.10.2.1.4. Chronic
5.10.2.1.5. Repaired
5.10.2.1.6. Configuration
5.10.2.2. What language is this written in?
5.10.2.2.1. Any known risks/limitations using this lang to write past similar functions?
5.10.2.3. Look at the page source code to see if there are any obvious logic flaws or scenarios that would immediately fail (this snippet may include more than just the palindrome code)
5.10.2.3.1. //Authors: // Paul Harju (harjup13@gmail.com) // Justin Rohrman function isPalindrome() { var original original = document.getElementById("originalWord").value; var palindrome = original.split("").reverse().join(""); if ( original === palindrome) { document.getElementById("palindromeResult").innerHTML = "Yes! " + original + " reversed is " + palindrome; } else { document.getElementById("palindromeResult").innerHTML = "No! " + original + " reserved is " + palindrome; } } function shuffle(o){ //v1.0 for(var j, x, i = o.length; i; j = Math.floor(Math.random() * i), x = o[--i], o[i] = o[j], o[j] = x); return o; }; function makeAnagram() { var original original = document.getElementById("anagramWord").value; var anagramList = ""; document.getElementById("anagramResult").innerHTML = "5 potential anagrams:</br>"; for (var i = 0; i < 5; i++) { var anagram = shuffle(original.split("")).join(""); document.getElementById("anagramResult").innerHTML += anagram + "</br>"; } }