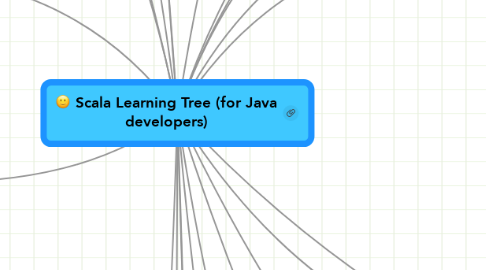
1. Scope
1.1. private[this]
1.2. private[package]
1.3. private[<whatever>]
2. vals/vars
2.1. 'name:Type' instead of 'Type name'
2.2. equivalent to Java's final
2.3. immutability encouraged by default
3. Type Basics
3.1. Inference
3.1.1. With recursive functions
3.2. Type Ascription
3.2.1. e.g. val b = 2 : Byte
3.3. Any/AnyRef/AnyVal vs Object
4. Specialist Topics
4.1. Testing
4.1.1. Specs
4.1.2. ScalaTest
4.1.3. SUnit
4.2. Java Interop
4.2.1. Collections
4.2.2. Array and GenericArray
4.2.3. Generics (erasure)
4.3. Concurrency
4.3.1. threads
4.3.2. Actors
4.3.2.1. react/reply
4.3.2.2. reactors
4.3.2.3. lift/akka/scalaz
4.3.3. fork/join
4.4. 3rd Party Libs
4.4.1. scaladb
4.4.2. scalaz
4.4.3. scalax
4.4.4. akka
4.4.5. liftweb
5. notes on this map
5.1. Try not to mention any feature unless it's defined in terms of stuff above it on the list
5.1.1. Unless under 'To Be Organised', where anything goes!
6. Oddities and FAQs
6.1. null vs Option[]
6.2. null/None/Nothing/Nil
6.3. Tooling support
6.3.1. Build systems
6.3.2. IDEs
6.3.3. Code Coverage
7. To Be Organised
8. Advanced Functions
8.1. higher-typed functions
8.1.1. call by name
8.2. PartialFunction
8.2.1. From a match block
8.2.2. isDefinedAt
8.3. closures
9. New node
10. Variance in generics
10.1. Declaration vs Use-Site
10.1.1. ±notation
10.2. Co/Con/In-variance
11. Methods (defs)
11.1. Don't *require* parenthesis
11.2. multiple argument lists
11.3. Unit instead of void
11.3.1. '()' syntactic sugar
11.4. ': Unit' vs. not using '='
11.5. If single statement, don't require { braces }
11.6. Named arguments
11.7. Default arguments
11.8. Varargs and the _* notation
11.9. override is a required keyword, not an annotation
11.10. Exception checking is not forced
11.10.1. the @throws annotation
11.11. 'Nothing' subclasses everything
11.12. Nested/inner methods
12. packages and imports
12.1. _ instead of *
12.2. import anywhere
12.3. multiple package statements and resolution
12.4. import from an instance
13. Uniform Access Principle
13.1. How getters/setters work
13.2. Overriding defs with vals/vars
13.3. @BeanPropery and @BeanInfo
14. Objects and Friends
14.1. classes
14.1.1. Body is the primary constructor
14.1.2. Secondary constructors
14.1.3. val & var on params
14.2. Singletons
14.3. Companions
14.3.1. Singleton is provided via companion object
14.4. Traits
14.4.1. mixins
14.5. Case Class Basics
14.5.1. hashcode/equals/canEqual
14.5.2. args as vals
14.5.3. Constructing without 'new'
14.5.3.1. Postpone explanation of how this is achieved
14.5.4. toString
14.5.5. Postpone usage in pattern matching until pattern matching is introduced
14.6. Package Objects
15. First-Class Functions
15.1. apply()
15.2. passing as an argument
15.2.1. higher-typed
15.3. functions vs methods
15.4. anonymous functions
15.4.1. underscore (_) as a placeholder
15.5. Partial Application
15.6. Currying
15.7. Singleton as a Function
15.7.1. Using apply on a companion object - factory pattern
15.8. Anonymous => syntactic sugar
16. For Comprehensions
16.1. Simple for (i <- 0 to 10) {println i}
16.2. Multiple Generators
16.3. Using yield to return a collection of the same type as the input
16.4. Desugaring to map/filter/flatmap/foreach
17. Exception Handling
18. Patterns
18.1. binding
18.2. simple usage - assigning a tuple to 2 vals
18.3. match blocks
18.4. Matching on Structure
18.5. Matching on Type
18.6. Matching on absolutes
18.7. and case classes
18.7.1. sealed cases and exhaustive matches
18.8. catch-all with an undescore
18.9. Use in for comprehension
18.10. Extractors
18.10.1. unapply
18.10.2. unapplySeq
19. Tuples / Pair
19.1. a -> b syntactic sugar
19.2. (a,b) syntactic sugar
20. Collections
20.1. Mutable vs Immutable
20.2. Seq vs List vs Iterable vs Traversable
20.3. Maps
20.3.1. A map is a function
20.4. Lists
20.4.1. cons
20.4.2. head
20.4.3. tail
20.4.4. Nil
21. Basic Generics
21.1. [] notation
22. Implicits
22.1. Conversions
22.1.1. View Bounds
22.1.2. Pimp-My-Library
22.2. Parameters
22.2.1. Final Parameter List may be implicit
22.2.2. e.g Ordering[T] passed to sort method
22.2.3. Context Bounds as syntaactic sugar: foo[T: Ordered](a: Any) === foo[T](a: Any)(implicit ev1$: Ordered[T])
22.2.4. Poor Mans Typeclasses: http://lampwww.epfl.ch/~odersky/talks/wg2.8-boston06.pdf
22.3. Implicit Scope
22.3.1. Local members (vals/vars/object/defs) and Imported members
22.3.1.1. (Shadowed by name!)
22.3.2. Companion object of parts of expected type
23. Reflection
23.1. classOf[]
23.2. Manifest and ClassManifest
23.2.1. Passed with a Context Bound