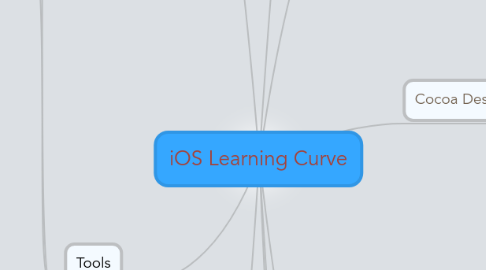
1. App Distribution
1.1. iOS Developer Program
1.1.1. iOS Developer Program
1.1.2. iOS Developer Enterprise Program
1.2. Certificates
1.2.1. Development Certificates
1.2.1.1. iOS App Development
1.2.1.2. Apple Push Notification service SSL (Sandbox)
1.2.2. Production Certificates
1.2.2.1. iOS App Distribution
1.2.2.1.1. Ad Hoc
1.2.2.1.2. In-House
1.2.2.1.3. App Store
1.2.2.2. Apple Push Notification service SSL (Production)
1.3. Identifiers
1.3.1. Registering an App ID
1.3.1.1. Explicit App ID
1.3.1.2. Wildcard App ID
1.4. Provisioning Profiles
1.4.1. Development Provisioning Profiles
1.4.2. Distribution Provisioning Profiles
1.4.2.1. Ad Hoc
1.4.2.2. In-House
1.4.2.3. App Store
1.5. Devices
1.5.1. Registering devices
2. Tools
2.1. Xcode 5
2.1.1. Getting familiar with the user interface
2.1.1.1. Navigators
2.1.1.2. Editors
2.1.1.2.1. Standard Editor
2.1.1.2.2. Assistant Editor
2.1.1.2.3. Version Editor
2.1.1.3. Utilities
2.1.1.3.1. Inspectors
2.1.1.3.2. Libraries
2.1.2. Interface Builder
2.1.3. Build Configurations
2.1.4. Schemes
2.1.5. Adding new frameworks
2.1.6. Archive
2.2. Instruments
2.2.1. Locating Memory Issues
2.2.2. Measuring I/O Activity
2.2.3. Measuring Graphics Performance
2.2.4. Analyzing CPU Usage
2.3. Git
2.3.1. Basic concepts
2.3.1.1. Distributed version control systems
2.3.1.2. Working directory
2.3.1.3. Staging area
2.3.2. Setting up a repository
2.3.2.1. Cloning an existing Git repository
2.3.2.2. Initializing a Git repository in an existing project
2.3.3. Ignoring Files
2.3.4. Committing changes
2.3.5. Viewing commit history
2.3.6. Remote repositories
2.3.6.1. Adding remote repos
2.3.6.2. Fetching and pulling from remotes
2.3.6.3. Pushing to remotes
2.3.6.4. Removing and renaming remotes
2.3.7. Tagging
2.3.8. Branching and merging
2.3.8.1. Creating a new branch
2.3.8.2. Renaming an existing branch
2.3.8.3. Merging a brach into current branch
2.3.8.4. Solving merge conflicts
2.3.8.5. Switching branches
2.3.9. Undoing things
2.3.9.1. Changing last commit
2.3.9.2. Un-staging staged files
2.3.9.3. Resetting modified files
2.3.10. git flow
2.4. CocoaPods
2.4.1. Adding a dependency
2.4.2. Updating outdated libraries
2.4.3. Custom Podspec
2.5. LLDB
2.5.1. Examining variables
2.5.2. Evaluating expressions
2.5.3. Working with breakpoints
2.6. Unix / Command Lines
3. Human Interface Design
3.1. Aesthetic integrity
3.2. Consistency
3.3. Direct manipulation
3.4. Feedback
3.5. Metaphors
3.6. User control
4. Objective-C
4.1. C
4.2. Object-oriented programming
4.2.1. Objects
4.2.2. Classes
4.2.3. Inheritance
4.2.4. Encapsulation
4.2.5. Polymorphism
4.3. Methods and Messaging
4.3.1. Instance Methods
4.3.2. Class Methods
4.4. Defining Classes
4.4.1. Interface
4.4.2. Implementation
4.4.3. Declared Properties and Access Methods
4.4.4. Class Clusters
4.5. Creating Objects
4.5.1. Object Allocation
4.5.2. Object Initialization
4.5.3. Class Factory Methods
4.6. Blocks
4.7. Dealing with Errors
4.8. Protocols
4.9. Categories and Extensions
4.10. Values and Collections
4.10.1. Fast Enumeration
4.11. Modern Objective-C
4.11.1. Method Ordering
4.11.2. Default Property Synthesis
4.11.3. NS_ENUM
4.11.4. Object Literals
4.11.4.1. NSNumber Literals
4.11.4.2. Boxed Expression Literals
4.11.4.3. Array Literals
4.11.4.4. Dictionary Literals
4.11.5. Object Subscripting
4.11.5.1. Array Subscripting
4.11.5.2. Dictionary Subscripting
4.11.5.3. Custom Objects Subscripting
4.12. Objective-C Runtime
4.12.1. Associative References
5. Algorithms
5.1. Algorithm Analysis
5.1.1. Big O notation
5.2. Algorithm Design
5.2.1. Divide-and-conquer algorithms
5.2.2. Dynamic programming
5.2.3. Greedy algorithms
5.3. Data Structures
5.3.1. Array vs Set
5.3.2. Linked List
5.3.3. Stacks vs Queues
5.3.4. Hash Tables
5.3.5. Priority queues
5.3.6. Trees
5.3.6.1. Binary Search Tree
5.3.6.2. B-Trees
5.3.7. Graphs
5.4. Sorting
5.4.1. Heapsort
5.4.2. Quicksort
5.4.3. Mergesort
5.5. Searching
5.5.1. Sequential Search
5.5.2. Binary Search
5.5.3. Hash-based Search
5.6. Graph Algorithms
5.6.1. Breadth-First Search
5.6.2. Depth-First Search
5.6.3. Minimum Spanning Trees
5.6.4. Single-Source Shortest Paths
6. Cocoa Design Patterns
6.1. Model-View-Controller
6.1.1. Models
6.1.2. Views
6.1.3. Controllers
6.1.3.1. Model controller
6.1.3.2. View controller
6.2. Delegation
6.2.1. Delegates
6.2.2. Data Sources
6.3. Protocols
6.4. Notifications
6.5. Key-Value Observing
6.6. Composite
6.7. Target-Action
6.8. Outlets
6.9. Introspection
6.9.1. isKindOfClass: vs isMemberOfClass:
6.9.2. respondsToSelector:
6.9.3. conformsToProtocol:
6.9.4. hash and isEqual:
6.10. Toll-Free Bridging
6.11. View hierarchy
6.12. Responder chain
6.13. Receptionist
6.14. Singleton
7. Frameworks
7.1. Foundation
7.1.1. NSString
7.1.2. NSNumber
7.1.3. Collections
7.1.3.1. NSArray and NSMutableArray
7.1.3.2. NSDictionary and NSMutableDictionary
7.1.3.3. NSSet and NSMutableSet
7.2. UIKit
7.2.1. UITableView
7.2.2. UIScrollView
7.2.3. UICollectionView
7.3. Core Data
7.4. Text Kit
7.5. GCD
7.6. NSOperationQueue
7.7. Database
7.8. Networking
8. Services
8.1. Local Notifications
8.2. Push Notifications
8.3. In-App Purchase
8.4. Map Kit
8.5. Location
8.6. Passbook
8.7. iCloud
8.8. Social Sharing
8.8.1. Facebook
8.8.2. Twitter
8.8.3. Weibo
8.9. Analytics
8.9.1. Flurry Analytics
8.9.2. Google Analytics
8.9.3. Localytics
8.10. Conversion Tracking
8.10.1. Facebook
8.10.2. Google