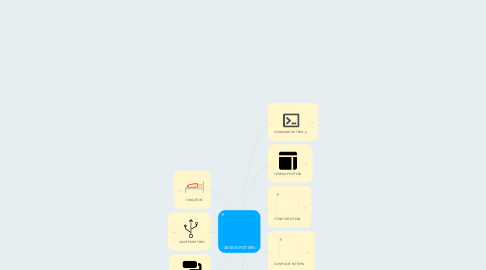
1. SINGLETON
1.1. definition
1.1.1. one instance for entire application
1.2. example
1.2.1. dialog box, thread pools, object used for loging,...
1.3. key implement
1.3.1. private static variable(s)
1.3.2. public static method to get it (or new it) if we have not init it
1.3.3. protect from multi thread problem
1.3.3.1. synchronize method if needed
1.3.3.1.1. public static synchronize void methodNameA()
1.3.3.2. to not using synchronize option, we can new instance first, then no need to worry about multi thread problem
1.3.3.3. only use synchronize method on init code part
1.3.3.3.1. private volatile static myClass onlyInstance
1.3.3.3.2. if(onlyInstance == null) { synchronized(myClass.class) { if(onlyInstance==null) // new instance
2. COMMAND PATTERN
2.1. definition
2.1.1. seeing command as object to execute without knowledge about what gonna execute
2.2. example
2.2.1. control home devices using a remoteControl, send command to devices.
2.3. key implement
2.3.1. having a interface Command to change command to object
2.3.1.1. public interface Command { public void execute() }
2.3.1.2. you can add more function such as
2.3.1.2.1. public void undo()
2.3.2. having some class (concrete or not) implement Command interface. Write content for execute() function
2.3.2.1. public lightOnCommand implement Command { execute() { // do something }}
2.3.3. implement RemoteControl with content series of command
2.3.3.1. in constructor: can use NoCommand class for null execute
2.3.3.2. having method setCommand(slot, commandTypeA, commandTypeB)
2.3.3.3. some others method for execute request from client, for ex
2.3.3.3.1. onButtonClicked(int slot) { onCommand[slot].execute()
2.3.3.3.2. offButtonClicked(int slot) { offCommand[slot].execute()
2.3.4. using main() to add command to list of command in remoteControl
2.3.4.1. ex: lightOnCommand livingRoomLightOn = new ...
2.3.4.2. lightOffCommand livingRoomLightOff = new ...
2.3.4.3. add arguments to remoteControl
2.3.4.3.1. remote.setCommand(1, lightOffCommand, lightOnCommand)
2.3.4.4. check with button using function: remote.onButtonPressed(slot)
2.4. by changing from method to object, we can execute them whenever we want, it like we have a library of command with using only one method execute() to do lots type of command.
3. ADAPTER PATTERN
3.1. definition
3.1.1. convert the interface of a class into another interface the clients expect. Adapter lets classes work together that couldn't otherwise because of incompatible interfaces
3.2. example
3.2.1. client have shorted on duck, and we decided to use some turkeys instead of ducks for client. So we implement a TurkeyAdapter implement Duck.
3.2.1.1. public class TurkeyAdapter implements Ducks { Turkey turkey; public TurkeyAdapter(Turkey turkey) { this.turkey = turkey; } public void quack() { turkey.gobble();}
3.3. Key implement
3.3.1. has one or more adaptees in the adapter class to support client interface.
4. FACADE PATTERN
4.1. this is a simple idea pattern
4.1.1. has all needed class in it and simple some actions for client without affect old code, class.
4.2. example
4.2.1. in facade class have one method name: playMusic
4.2.1.1. inside it we have: turnOnTv, turnOnCd, playCd, setVolumn(int a),...
5. TEMPLATE PATTERN
5.1. definition
5.1.1. as the name of this pattern say: template
5.1.1.1. functions
5.2. example
5.2.1. make survey template include questions, type of question,... developer can add more type but adding type must have somethings which ruled in the template
5.3. key implement
5.3.1. using composition and inheritance.
5.3.1.1. extends or interface
5.3.2. and key word "final" to avoid overide
5.3.2.1. final method for make Crazy Cake: public final void makeCrazyCake() { a(); b(); if(c()) // do something }
5.3.3. use abstract method for diverse implementation
5.3.3.1. public abstract void a();
5.3.4. using concrete function for overiding option
5.3.4.1. public boolean c() { return true; }
6. ITERATOR PATTERN
6.1. Definition
6.1.1. share same loop interface.
6.2. Example
6.2.1. when you have two or more different types of data ( for ex: array list, array, ...) and you want to use one loop to go through all of these type of data. Use this pattern
6.3. Key implement
6.3.1. create a interface Iterator which has two basic method (you can add more later): next() and hasNext()
6.3.2. implement concrete classes implement this interface and its method.
6.3.2.1. variables
6.3.2.1.1. appropriate client concrete class
6.3.2.1.2. position to hold current pos
6.3.2.2. for next method:
6.3.2.2.1. using its own variables
6.3.2.3. for hasNext
6.3.2.3.1. check by using current pos and null
6.3.3. modify client classes: add createIterator() and return appropriate Iterator class
6.4. Design summary
6.4.1. image
7. COMPOSITE PATTERN
7.1. Definition
7.1.1. allows
7.1.1.1. you to compose objects into tree structures
7.1.1.1.1. to represent part-whole hierachies
7.1.1.2. clients treat individual objects and composition of objects uniformly
7.2. example
7.2.1. Meet these kind of problems a lot in real life.
7.2.1.1. create a survey and you want a sub question inside main question
7.2.2. Menu in a restaurant
7.3. Key implement
7.3.1. general class view
7.3.2. implement
7.3.2.1. create abstract or interface: Component with method of both leaf and composite
7.3.2.1.1. ex
7.3.2.2. leaf class extend or implement from Component
7.3.2.3. composite class
7.3.2.3.1. extend or implement from Component
7.3.2.3.2. contain list of Component inside
8. STATE PATTERN
8.1. Definition
8.1.1. allows an object to alter its behavior when its internal state changes
8.1.1.1. The pattern encapsulates state into separate classes and delegates to the object representing the current state, we know that behavior changes along wit the internal state
8.1.2. The object will appear to change its class
8.2. Example
8.2.1. Meet lots in real life
8.2.1.1. you have trouble when using too much conditional check for which state the machine, object, user in
8.3. Class diagram
8.3.1. state pattern diagram
8.4. Key implement
8.4.1. View each state as a separate class extend or implement State class
8.4.2. view all action to go from this state to that state as method inside state class
8.4.3. implement action for each method inside state class