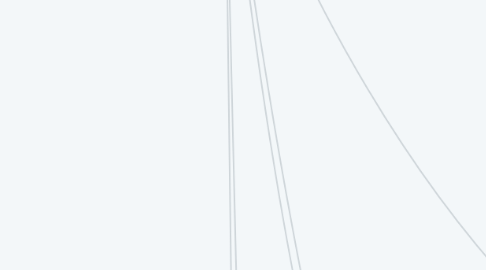
1. Computer Science knowledge
1.1. Algorithms
1.1.1. Sorting
1.1.2. Graph Theory
1.1.2.1. Trees
1.1.3. Strings
1.1.4. Greedy
1.1.5. Dynamic Programming
1.1.6. Bit Manipulation
1.1.7. Recursion
1.1.8. Game Theory
1.1.9. NP Complete
1.1.10. Big-O notation
1.2. Abstract Data Types
1.2.1. Stack
1.2.2. Array
1.2.3. List
1.2.4. Map
1.2.5. Multimap
1.2.6. Set
1.2.6.1. Multiset (Bag)
1.2.7. Graph
1.2.7.1. Tree
1.2.8. Queue
1.2.9. Double-ended queue
1.2.10. Priority Queue
1.2.11. Double-ended priority queue
1.3. System design
1.3.1. Problems
2. Retain cycles
3. Security
3.1. Keychain
3.2. Security Transforms API
4. Swift
4.1. Closures
4.2. Initializers
4.3. Generics
4.4. Protocols
4.5. Structs
4.6. Enums
4.7. Runtime
4.7.1. Method dispatch
5. Languages
5.1. Objective-C
5.1.1. Blocks
5.1.1.1. Memory Management
5.1.2. KVC
5.1.3. KVO
5.1.4. Toll-free bridging
5.1.5. Runtime
5.1.5.1. Swizzling
5.1.5.2. NSZombies and KVO implementation
5.1.5.3. Method messaging
5.2. Perform selector family
6. Factory
7. Memory management
7.1. Stack and Heap
7.2. Value vs Reference type
7.3. MRC
7.4. ARC
7.4.1. Shallow and deep copying
7.5. Garbage collection
7.6. Memory leaks
7.6.1. Weak references
7.7. Autorelease pool
8. Practical knowledge
8.1. First pet project ideas
8.2. GCD
8.3. Multithreading and concurency
8.3.1. POSIX and NSThreads
8.3.2. NSOperation(Queue)
8.3.3. Runloop
8.3.4. Synchronization
8.3.5. Problems
8.3.5.1. Race condition
8.3.5.2. Deadlock
8.3.5.3. Readers–writers problem
8.4. Cocoa Touch
8.4.1. UIKit
8.4.1.1. UIApplication
8.4.1.1.1. States
8.4.1.1.2. UIApplicationDelegate
8.4.1.2. UIViews
8.4.1.2.1. UITableViews
8.4.1.2.2. UICollectionViews
8.4.1.3. Layers
8.4.1.4. Layout
8.4.1.4.1. Frame-based
8.4.1.4.2. Autolayout
8.4.1.5. Animations
8.4.1.6. Transform
8.4.1.7. Navigation
8.4.1.8. UIViewController
8.4.1.8.1. Lifecycle
8.4.2. Core Motion
8.4.3. UserNotifications
8.4.4. Core Location
8.5. Foundation
8.5.1. Notifications vs Delegation vs Observing
8.5.2. Collections
8.5.3. Networking
8.5.4. Serialization
8.5.4.1. NSCoding
8.5.4.2. Codable
8.5.4.3. JSON
8.5.4.4. XML
8.5.4.5. Protobuf
8.6. Work in background mode
8.7. Software Architecture
8.7.1. Design Patterns
8.7.1.1. Cocoa
8.7.1.1.1. Abstract Factory
8.7.1.1.2. Adapter
8.7.1.1.3. Command Pattern
8.7.1.1.4. Chain of Responsibility
8.7.1.1.5. Decorator
8.7.1.1.6. Facade
8.7.1.1.7. Memento
8.7.1.1.8. Proxy
8.7.1.1.9. Receptionist
8.7.1.1.10. Singleton
8.7.1.1.11. Observer
8.7.1.1.12. Template Method
8.7.1.1.13. MVC
8.7.1.2. Architectural
8.7.1.2.1. MVC
8.7.1.2.2. MVVM
8.7.1.2.3. MVP
8.7.1.2.4. Clean architecture
8.7.1.2.5. Coordinators
8.7.1.3. Creational
8.7.1.3.1. Abstract Factory
8.7.1.3.2. Builder
8.7.1.3.3. Factory Method
8.7.1.3.4. Prototype
8.7.1.3.5. Object Pool
8.7.1.3.6. Singleton
8.7.1.4. Structural
8.7.1.4.1. Adapter
8.7.1.4.2. Bridge
8.7.1.4.3. Composite
8.7.1.4.4. Decorator
8.7.1.4.5. Facade
8.7.1.4.6. Flyweight
8.7.1.4.7. Proxy
8.7.1.5. Behavioural
8.7.1.5.1. Command
8.7.1.5.2. Chain of responsibility
8.7.1.5.3. Interpreter
8.7.1.5.4. Iterator
8.7.1.5.5. Mediator
8.7.1.5.6. Memento
8.7.1.5.7. Observer
8.7.1.5.8. State
8.7.1.5.9. Strategy
8.7.1.5.10. Visitor
8.7.1.6. Concurrency
8.7.1.6.1. Anti-pattern
8.7.2. Design Principles
8.7.2.1. SOLID
8.7.2.2. Inversion of Control
8.7.2.2.1. Dependency Injection
8.7.2.2.2. Service Locator
8.7.2.3. Protocol-Oriented Programming
8.7.3. Object-Oriented Programming
8.7.3.1. Focus interactions
8.7.4. Functional programming
8.7.4.1. Functional Reactive Programming Frameworks
8.7.4.1.1. React Native
8.7.4.1.2. RxSwift
8.7.4.1.3. RxRealm, RxDataSources
8.8. Dependencies management
8.8.1. Cocoapods
8.8.2. Carthage
8.8.3. Swift Package Manager
8.9. Project structure and File/Group organisation
8.10. Version Control Systems
8.10.1. Git
8.11. Debugging
8.11.1. Best practices
8.11.1.1. Checklists
8.11.2. Instruments
8.12. UX
8.13. Caching and Persistency
8.13.1. Core Data
8.13.2. Realm
8.13.3. YAPDatabase
8.14. Testing
8.14.1. Unit Tests
8.14.2. Snapshot Tests
8.14.3. Functional test
8.14.4. TDD
8.14.5. BDD
8.15. Performance optimization
8.15.1. Increase FPS
8.15.2. Decrease memory footprint
8.16. Code signing
8.17. Tools
8.17.1. IDE
8.17.1.1. Xcode
8.17.1.1.1. Interface Builder
8.17.2. Swiftlint
8.17.3. Sourcery
8.17.4. Fastlane
8.18. Continuous Integration
8.18.1. Jenkins
8.18.2. Xcode server