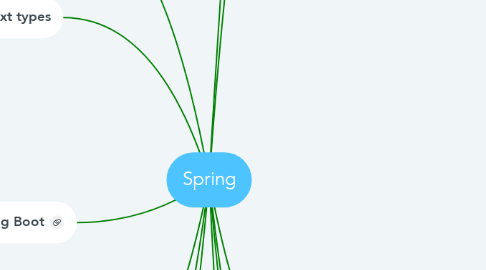
1. @ComponentScan
1.1. configures which packages to scan for classes with annotation configuration
1.2. @ComponentScan(basePackages="com.baeldung.annotations")
1.3. @ComponentScan(basePackageClasses=VehicleFactoryConfig.class)
1.4. <context:component-scan base-package="com.baeldung" />
2. Context types
3. Spring Boot
3.1. Автоконфигурирование компонентов
3.1.1. Spring Boot is basically an extension of the Spring framework which eliminated the boilerplate configurations required for setting up a Spring application.
3.2. Упрощение конфигурации
3.2.1. Opinionated ‘starter' dependencies to simplify build and application configuration
3.2.1.1. spring boot staters
3.2.2. Embedded server to avoid complexity in application deployment
3.2.3. Metrics, Health check, and externalized configuration
3.2.4. Automatic config for Spring functionality – whenever possible
3.3. Actuator
3.3.1. Предоставляет сервисные методы
3.3.2. Предоставляет endpoints для метрики
4. Scheduling
5. Spring Data
6. AOP
6.1. JDK Dynamic Proxy
6.1.1. Если есть интерфейсы
6.1.2. Создаёт прокси-объект
6.1.3. JDK dynamic proxy могут перекрывать только public методы
6.2. CGLib proxy
6.2.1. Если нет интерфейсов
6.2.2. Создаёт наследника
6.2.3. CGLib proxy – кроме public, также и protected методы и package-visible
6.3. Аннотации
6.3.1. @Aspect
6.3.2. @Around
6.3.3. @Pointcut
6.3.3.1. Advice
6.3.3.1.1. Before — перед вызовом метода
6.3.3.1.2. After — после вызова метода
6.3.3.1.3. After returning — после возврата значения из функции
6.3.3.1.4. After throwing — в случае exception
6.3.3.1.5. After finally — в случае выполнения блока finally
6.3.3.1.6. Around — можно сделать пред., пост., обработку перед вызовом метода, а также вообще обойти вызов метода.
7. Transactional
7.1. В Proxy-mode спринг не перехватывает вызовы методов внутри класса
7.2. В AspectJ вызовы внутри класса перехватываются
7.3. @EnableTransactionManagement
7.3.1. However, if we're using a Spring Boot project, and have a spring-data-* or spring-tx dependencies on the classpath, then transaction management will be enabled by default.
7.4. @Transactional
7.4.1. Над классом или над методом сервиса
7.4.2. Note that – by default, rollback happens for runtime, unchecked exceptions only. The checked exception does not trigger a rollback of the transaction. We can, of course, configure this behavior with the rollbackFor and noRollbackFor annotation parameters.
7.4.3. Параметры
7.4.3.1. value transactionManager
7.4.3.2. propagation
7.4.3.2.1. REQUIRED
7.4.3.2.2. REQUIRES_NEW
7.4.3.2.3. MANDATORY
7.4.3.2.4. SUPPORTS
7.4.3.2.5. NOT_SUPPORTED
7.4.3.2.6. NEVER
7.4.3.2.7. NESTED
7.4.3.3. isolation
7.4.3.3.1. DEFAULT
7.4.3.3.2. READ_UNCOMMITTED
7.4.3.3.3. READ_COMMITTED
7.4.3.3.4. REPEATABLE_READ
7.4.3.3.5. SERIALIZABLE
7.4.3.4. timeout
7.4.3.5. readOnly
7.4.3.5.1. Это только подсказка менеджеру транзакции для оптимизации. По-факту, не накладывает никаких ограничений на изменение данных.
7.4.3.6. rollbackFor
7.4.3.6.1. По-умолчанию откат происходит только для RuntimeException
7.4.3.7. noRollbackFor
7.4.3.7.1. Игнорирование указанных исключений
7.4.4. Спринг создаёт прокси для всего класса для вызовов методов
8. Bean definition
8.1. @Bean
8.1.1. @Component "Lite-mode"
8.1.1.1. Вызовы методов внутри класса происходят как обычно. Спринг не перехватывает вызов методов через CGLib-proxy
8.1.2. @Configuration mode
8.1.2.1. Создаётся CGLib-прокси объект. Методы класса вызывают другие методы через прокси-объект.
8.1.3. Additional annotations
8.1.3.1. @Qualifier
8.1.3.2. @Profile
8.1.3.3. @Scope
8.1.3.3.1. scopeName
8.1.3.3.2. proxyMode
8.1.3.4. @DependsOn
8.1.3.5. @Order
8.2. @Component
8.2.1. @Repository
8.2.1.1. @Repository’s job is to catch persistence specific exceptions and rethrow them as one of Spring’s unified unchecked exception.
8.2.1.2. PersistenceExceptionTranslationPostProcessor This bean post processor adds an advisor to any bean that’s annotated with @Repository.
8.2.1.3. @Bean public PersistenceExceptionTranslationPostProcessor exceptionTranslation() { return new PersistenceExceptionTranslationPostProcessor(); }
8.2.2. @Service
8.2.2.1. @Service indicates that it's holding the business logic. So there's no any other specialty except using it in the service layer.
8.2.3. @Controller
8.2.3.1. @RestController
8.2.3.1.1. It's a convenience annotation that combines @Controller and @ResponseBody
8.2.3.1.2. The controller is annotated with the @RestController annotation, therefore the @ResponseBody isn't required.
8.2.3.2. @RequestMapping
8.2.3.2.1. @GetMapping
8.2.3.2.2. @PostMapping
8.2.3.2.3. @PutMapping
8.2.3.2.4. @DeleteMapping
8.2.3.2.5. @PatchMapping
8.2.3.3. @ResponseBody
8.2.4. @Component class CarUtility { // ... }
8.3. @Configuration
8.3.1. @Import
8.4. @Lazy
9. Injection
9.1. @Autowired
9.1.1. @Qualifier
9.1.1.1. @Autowired @Qualifier("fooFormatter") private Formatter formatter;
9.1.2. Custom Qualifier
9.1.2.1. @Autowired @FormatterType("Foo") private Formatter formatter;
9.1.3. Autowiring by Name
9.1.3.1. @Autowired private Formatter fooFormatter;
9.2. @Resource
9.2.1. JSR-250
9.3. @Inject
9.3.1. JSR-330
9.3.2. <dependency> <groupId>javax.inject</groupId> <artifactId>javax.inject</artifactId> <version>1</version> </dependency>
10. Spring Web
11. Java
11.1. Object
11.1.1. Throwable (checked)
11.1.1.1. Error (unchecked)
11.1.1.2. Exception (checked)
11.1.1.2.1. RuntimeException (unchecked)
11.1.1.2.2. IOException (checked)
12. Context Loading
12.1. 1. Загрузка конфигурации
12.1.1. ApplicationContext
12.1.1.1. Generic
12.1.1.1.1. GenericGroovyApplicationContext
12.1.1.1.2. GenericXmlApplicationContext
12.1.1.1.3. StaticWebApplicationContext
12.1.1.2. WebApplicationContext
12.1.1.2.1. ConfigurableWebApplicationContext
12.1.1.2.2. GenericWebApplicationContext
12.1.1.2.3. AnnotationConfigWebApplicationContext
12.1.1.2.4. GroovyWebApplicationContext
12.1.1.2.5. StaticWebApplicationContext
12.1.1.2.6. StubWebApplicationContext
12.1.1.2.7. XmlWebApplicationContext
12.1.1.3. AnnotationConfigApplicationContext
12.1.1.3.1. @ComponentScan({"package.name"})
12.1.1.3.2. <context:component-scan base-package="package.name"/>
12.1.1.4. ClassPathXmlApplicationContext
12.1.1.5. FileSystemXmlApplicationContext
12.1.1.6. GroovyApplicationContext
12.1.1.7. StaticApplicationContext
12.1.1.8. ResourceAdapterApplicationContext
12.1.2. /** key is a bean name */ Map<String, BeanDefinition>
12.2. 2. Настройка BeanDefinitions - BeanFactoryPostProcessor
12.2.1. Настраивает определения бинов до их создания
12.2.2. PropertySourcesPlaceholderConfigurer
12.2.2.1. <context:property-placeholder location="property.properties" />
12.2.2.2. @Configuration @PropertySource("classpath:property.properties") public class DevConfig { @Bean public static PropertySourcesPlaceholderConfigurer configurer() { return new PropertySourcesPlaceholderConfigurer(); } }
12.3. 3. Создание кастомных - FactoryBean<T>
12.3.1. Управляет процессом создания бинов
12.4. 4. Создание бинов - FactoryBean
12.5. 5. Настройка созданных бинов - BeanPostProcessor
12.5.1. postProcessBeforeInitialization
12.5.2. postProcessAfterInitialization
12.5.2.1. прокси делать в этом методе, после инициализации бина
12.5.3. Оба метода в итоге должны вернуть бин
12.5.4. @Component