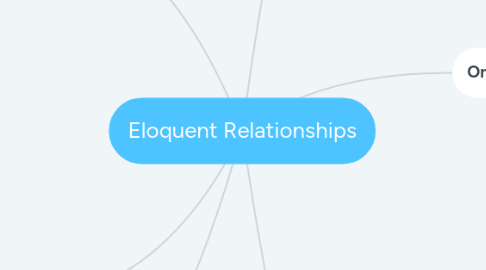
1. Many to Many
1.1. User has many contacts each Contacts related to many Users
1.2. belongsToMany() to define this relationship
1.3. pivot table to describe this relationship naming conventions: `users_contacts`
1.4. we must have `contact_id` and `user_id` in the pivot table
1.5. can pass id (either user_id or contact_id) to this relationship
1.6. using attach()/detach() to build relationship
1.7. updateExistingPivot() to update the pivot record
1.8. sync() : detach() all old relationship and attach() the new relationships
1.9. Getting data from Pivot Table
1.9.1. to store new fields to pivot table, define them at the relationship definitions: $this->belongsToMany()->withTimestamps() -> withPivot('field_1', 'field_2', ...)
1.9.2. access pivot record from : $user->contacts->each(function($contact){ $contact->pivot->created_at; });
2. Polymorphic
2.1. a single interface to objects of multiple types
2.2. for example: Star objects should define of which models it is relating to.
2.3. many to many polymorphic
2.3.1. example: Contacts, Events and Tags table we will have Taggables table to pivot these relationship Taggables has: tag_id, taggable_id, taggable_type (whether contacts or events)
2.3.2. morphToMany(Tag::class, 'taggable') morphedByMany(Event::class, 'taggable')
3. Child record update parent timestamps
3.1. if we want child record to update parent record, we should use this function
3.2. $touches = ['parentProperty']
4. One to One
4.1. define one to one relationships
4.2. ->hasOne( {Eloquent Class} , 'foreign_key')
4.3. ->belongsTo( {Eloquent Class'} , 'foreign_key')
4.4. we can inserting related item: $contact->phoneNumbers()->save($phoneInstance)
5. One to Many
5.1. define one to many relationships. take example : User has many contacts
5.2. ->hasMany(Contact::class, {user_id})
5.3. $user->contacts : return a collection
5.4. $user->contacts() : return a query builder
5.5. inverse relationship: belongsTo(User::class)
5.6. if we want to attach contacts to User: $contact->user()->associate(User::first()) then we can disassociate $contact->user()->disassociate()
5.7. Selecting records that have related item
5.7.1. can select only records with specific particular criteria
5.7.2. User with contacts : User::has('contacts')->get()
5.7.3. User with contacts > 5 User::has('contacts', '>', 5)->get()
5.7.4. Nesting, User has contacts with PhoneNumbers User::has('contacts.phoneNumbers')->get()
5.8. Has many though
5.8.1. pulling relationships of relationships
5.8.2. User has many contacts and each Contacts has many PhoneNumbers
5.8.3. we can define : public function phoneNumbers(){ return $this->hasManyThrough(PhoneNumber::class, Contact::class); }
5.8.4. customize the key of intermediate class (Contact in this case) as third parameters, and distant class (PhoneNumber in this case as Fourth parameters