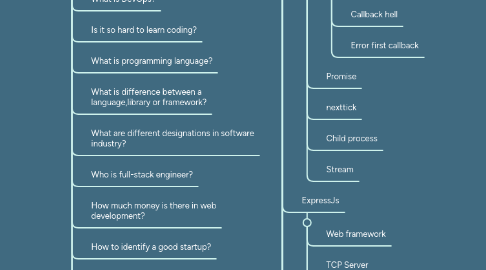
1. Mobile
1.1. Webview
1.1.1. Ionic
1.2. Native
1.2.1. React Native
1.3. Which one to choose?
2. Basic
2.1. JavaScript
2.1.1. Context/This
2.1.2. Closure/Scope
2.1.3. Global object
2.1.4. Hoisting
2.1.5. Reference
2.1.5.1. Immutability
2.1.5.2. Pass by reference
2.1.6. Class
2.1.6.1. Constructor
2.1.6.2. Chaining
2.1.6.3. Inheritance
2.1.6.4. Getters/Setters
2.1.7. Object
2.1.8. Events
2.1.8.1. Event listener
2.1.8.2. Event bubbling
2.1.9. Truthy/Falsy
2.1.10. Prototypes
2.1.11. Promise
2.1.11.1. Promise chaining
2.1.11.2. Async/Await
2.1.11.3. Microtask
2.1.11.4. Promise All
2.1.11.4.1. When to avoid it?
2.1.11.4.2. Handling errors
2.1.11.5. Promisify
2.1.12. IFFE
2.1.13. Currying
2.1.14. Spread operator
2.1.15. Destructuring
2.1.16. Garbage collection
2.1.17. Functions
2.1.17.1. Function declaration
2.1.17.2. Function Invocation
2.1.17.3. Pure Function
2.1.17.3.1. Side effect
2.2. TypeScript
2.2.1. Transpiler
2.2.2. Interface
2.2.3. Type
2.2.4. Generics
2.2.5. Enum
2.2.6. Is it even required?
2.2.7. Decorators
2.2.8. Partial
2.3. Linux
2.3.1. Why linux?
2.3.2. Tops Distributions
2.3.2.1. Ubuntu
2.3.2.1.1. Why ubuntu?
2.3.2.1.2. Difference between server and desktop?
2.3.2.2. Fedora
2.3.2.3. Redhat
2.3.2.4. OpenSUSE
2.3.3. CLI
2.3.3.1. Why CLI?
2.3.3.2. How to make your CLI look more beautiful ?
2.3.3.3. BASH/ZSH
2.3.4. File Structure
2.3.5. whoami
2.3.6. Root user
2.4. HTML
2.5. DOM
2.6. CSS
2.7. HTTP/HTTPS
2.7.1. Status code
2.7.2. Request header
2.7.3. Response header
2.7.4. CORS
2.7.4.1. What is CORS?
2.7.4.2. When it occurs?
2.7.4.3. How to fix CORS problem?
2.7.5. Host
2.7.6. Port
2.7.7. Query params
2.7.8. Methods
2.7.8.1. Get
2.7.8.2. Post
2.7.8.3. Put
2.7.8.4. Patch
2.7.8.5. Delete
2.7.8.6. Head
2.7.8.7. Options
2.7.9. TLS/SSL
2.7.9.1. Why is it necessary
2.7.9.2. How to generate one for free?
2.7.9.3. How it works?
2.8. DNS
2.9. Client - Server
2.10. Web Application
2.11. AJAX
2.12. APIs
2.12.1. What exactly is an API
2.12.2. API Reference
2.12.3. Web APIs
2.12.4. REST API
2.12.4.1. What is Specification?
2.12.4.2. What is protocal?
2.12.4.3. How to design better REST APIs
2.12.4.4. REST APIs Constraint
2.12.5. GraphQL
2.12.5.1. Why GraphQL?
2.12.5.2. What problem it solves?
2.12.6. Difference between REST and GraphQL
2.12.7. Which one to choose?
2.13. GIT
2.13.1. What exactly is GIT?
2.13.2. What is version control
2.13.3. Branch
2.13.4. What is local?
2.13.5. What is remote?
2.13.6. What is pull request or merge request?
2.13.7. What is fork?
2.13.8. What is branch tracking?
2.13.9. How to fix merge conflicts?
2.13.10. What is stashing?
2.13.11. Writing a commit message?
2.13.12. What is cherry picking?
2.13.13. What are git hooks?
2.13.14. What happens when you run "git push -u origin master"
2.13.15. What is difference between http and ssh
2.13.16. How to handle mutliple github accounts on same machine?
2.13.17. What is origin and upstream?
2.13.18. .gitignore
2.13.19. .gitattributes
2.14. Microservice/Monolith
2.15. Serverless
2.16. Package Manager
2.17. Linting
2.17.1. eslint
2.18. JSON
2.18.1. Difference between JSON and JavaScript Object
2.18.2. JSON parse/stringify
2.18.3. GeoJSON
2.19. Style Guide/Best Practices
2.19.1. Design patterns
2.19.2. Is it even required?
2.19.3. 12 factor app
2.19.4. How and where to find out resources for this?
2.20. Finding Answer
2.20.1. Stack overflow
2.20.2. Github issues
2.20.3. Awesome
2.20.3.1. JavaScript
2.20.3.2. Node
2.20.3.3. React
2.20.3.4. Express
3. Product And Architecture
3.1. Requirement
3.2. Planning
3.3. Product Design
3.3.1. UI
3.3.2. UX
3.4. System design
3.4.1. Identifying stack
3.4.2. Thinking in terms of data
3.5. Execution
4. General Questions
4.1. What is machine and it's building blocks?
4.1.1. Disk
4.1.2. Processor
4.1.3. RAM
4.2. What is internet and how it works
4.3. What happens behind the scene when you type google.com in your browser?
4.4. What is programming?
4.5. Is there a difference between programming and coding?
4.6. What is web development?
4.7. What is DevOps?
4.8. Is it so hard to learn coding?
4.9. What is programming language?
4.10. What is difference between a language,library or framework?
4.11. What are different designations in software industry?
4.12. Who is full-stack engineer?
4.13. How much money is there in web development?
4.14. How to identify a good startup?
4.15. Why companies are prefering someone who has worked in startup?
4.16. I am stuck in maintenance job, how to get out of it?
4.17. How to become front-end engineer?
4.18. How to become backend engineer?
4.19. How to stay motivated?
4.20. I spend too much of time but still not able to deliver. How do i improve myself?
5. Backend
5.1. Web server
5.2. NodeJs
5.2.1. Runtime
5.2.2. Eventloop
5.2.2.1. epoll
5.2.2.2. Phases in eventloop
5.2.2.3. Events
5.2.3. Callback
5.2.3.1. What exactly is callback?
5.2.3.2. How to write callback?
5.2.3.3. Callback hell
5.2.3.4. Error first callback
5.2.4. Promise
5.2.5. nexttick
5.2.6. Child process
5.2.7. Stream
5.3. ExpressJs
5.3.1. Web framework
5.3.2. TCP Server
5.3.3. Middleware
5.3.3.1. Next()
5.4. Apollo GraphQL
5.4.1. Apollo Server
5.4.2. Resolver
5.4.3. Context
5.4.4. Apollo Federation
5.4.5. API Gateway
5.4.6. N+1 Problem
5.5. Process
5.5.1. What exactly is process
5.5.2. What is node process?
5.5.3. Daemons
5.6. Thread
5.6.1. Thread pool
5.6.2. Is it even required?
5.6.3. Multithreading
5.7. Memory Management
5.7.1. Default memory
5.7.2. How to manage it?
5.8. IO
5.8.1. What exactly is IO
5.8.2. Types of IO
5.9. Encryption
5.10. Encoding
5.11. Blocking/Non-blocking
5.11.1. What exactly get blocked?
5.11.2. How to avoid blocking code?
5.12. Synchronous/Asynchronous
5.12.1. What all things are synchronous and asynchronous
5.12.2. How to make a code asynchronous
5.13. API Gateway
5.14. Authentication/Authorization
5.14.1. Basic Auth
5.14.2. JWT
5.14.2.1. Symmetric
5.14.2.2. Asymmetric
5.14.2.3. Payload
5.14.2.4. Header
5.14.2.4.1. JOSE
5.14.2.5. Algorithms
5.14.3. JWK
5.14.4. JWS
5.14.5. JWE
5.14.6. SSO
5.14.7. Third party authenticaion
5.14.8. OAuth
5.14.8.1. What exactly is Oauth
5.14.8.2. OpenId connect
5.14.8.3. Identiry token
5.14.8.4. Access token
5.14.8.5. Refresh token
5.14.8.6. Grant types
5.14.8.6.1. Explicit
5.14.8.6.2. Implicit
5.14.9. Firebase/Auth0/Cognito
5.15. Session Management
5.15.1. Cookie
5.15.2. Token
5.16. Message Queue
5.16.1. Push based
5.16.1.1. RabbitMQ
5.16.2. Pull based
5.16.2.1. Kafka
5.16.3. What exactly is message queue
5.16.4. When is it required?
5.16.5. Pub/Sub
5.16.6. Producer/Consumer
5.17. Websockets
5.17.1. Socket.io
5.18. Validations
5.18.1. JOI
5.18.2. ValidatorJs
5.19. Error Handling
5.19.1. Global
5.19.1.1. unhandled promise rejection
5.19.1.2. unhandledRejection
5.19.2. Custom Error
5.20. Logging
5.20.1. Winston
5.20.2. Morgan
5.21. Inter Service Communication
5.21.1. When is it required?
5.21.2. Synchronous
5.21.2.1. http
5.21.2.2. grpc
5.21.3. Asynchronous
5.21.3.1. pub/sub
5.22. Template engine
5.23. Environment Variables
5.23.1. Why environment variable?
5.23.2. OS level env
5.23.3. Process level env
5.24. ACL
5.25. Testing
5.25.1. Mocha
5.25.2. Chai/Should
5.26. Security
5.26.1. Validation/Sanitization
5.26.2. Headers
5.26.3. Reverse Proxy
5.26.4. NPM Audit
5.26.5. Rate limiting
5.27. Regex
5.28. CRON
6. Deployment/DevOps
6.1. Datacenter
6.1.1. What all the things are there in datacenter?
6.1.2. Availability Zone
6.2. Networking
6.2.1. DNS
6.2.2. IP
6.2.2.1. CIDR
6.2.3. Private/Public IP
6.2.4. Firewall
6.2.5. Network
6.2.6. VPC/VPN
6.3. Cloud
6.3.1. IaaS
6.3.2. PaaS
6.3.2.1. Heroku
6.3.3. SaaS
6.3.4. Private/Public Cloud
6.4. Containerisation
6.4.1. Docker
6.4.1.1. Docker engine
6.4.1.2. Docker image
6.4.1.3. Docker container
6.4.1.4. Networking
6.4.1.4.1. Modes
6.4.1.4.2. Port mapping
6.4.1.5. Volume
6.4.2. Docker compose
6.5. Orchestration
6.5.1. Kubernetes
6.6. Virtualisation
6.6.1. VM
6.7. Build
6.7.1. Webpack
6.7.2. Grunt
6.7.3. Gulp
6.8. Scaling
6.8.1. Virtical
6.8.2. Horizontal
6.9. Process Manager
6.10. Task Manager
6.11. Monitoring
6.12. SSH
6.12.1. Private vs Public Key
6.12.2. Handling multiple keys
6.12.3. How SSH Works?
6.13. SCP
6.14. AWS
6.14.1. EC2
6.14.2. VPC
6.14.3. Security Groups
6.14.4. Load Balancer
6.14.5. Subnets
6.14.5.1. Private
6.14.5.1.1. NAT
6.14.5.2. Public
6.14.6. ECS
6.14.7. EKS
6.14.8. ECR
6.14.9. Amplify
6.14.10. Cognito
6.14.11. IAM
6.14.12. KMS
6.14.13. SES
6.14.14. SNS
6.14.15. S3
6.14.16. Lambda
6.14.17. Cloudfront
6.14.18. Route 53
6.14.18.1. Hosted Zones
6.14.19. Cloudwatch
6.14.20. Cloudformation
6.14.21. Secret Manager
6.14.22. Console
6.14.23. CLI
6.15. GCP
6.15.1. Compute Engine
6.16. Infrastructure as code
6.16.1. Terraform
6.16.2. CDK
7. Database
7.1. Database server
7.2. MongoDB
7.2.1. Mongoose
7.2.2. ODM
7.2.3. Aggregation
7.2.4. Map-reduce
7.2.5. Search
7.3. SQL/NoSQL
7.3.1. What the difference SQL/NoSQL?
7.3.2. Which one is faster?
7.3.3. Which to choose when?
7.4. Redis
7.4.1. Data storage
7.4.2. Caching
7.5. MySQL
7.5.1. ORM
7.6. Indexing
7.7. Data Modelling
7.7.1. Transactional Data
7.7.2. Operational Data
7.8. Replication
7.9. Connection pool
7.10. Connection retry
7.11. Sharding
7.12. Migration
7.13. ACID
7.14. Transaction
7.15. Scaling
7.16. N+1 Problem
7.17. Table Partitioning
7.18. Data Type
7.18.1. String
7.18.1.1. ISO
7.18.2. Number
7.18.3. Array
7.19. Can we have multiple database in an app?
7.20. Administration
7.20.1. Reporting
7.20.2. Backup
7.20.3. Logging and Monitoring
7.21. Security
7.21.1. RBAC
7.21.2. Authentication
7.21.3. TLS/SSL
7.21.4. Encryption
8. Frontend
8.1. Framework/Library
8.1.1. ReactJs
8.1.2. VueJs
8.1.3. Anugular
8.1.4. JQuery
8.2. Static/Dynamic Sites
8.2.1. Static content
8.2.2. GatbyJs
8.3. ReactJs
8.3.1. Virtual DOM
8.3.2. Diff Algorithm
8.3.3. Components
8.3.3.1. Class
8.3.3.2. Component lifecycle
8.3.3.3. Functional
8.3.4. State and Props
8.3.5. Hooks
8.3.5.1. Lifecycle
8.3.5.2. Custom hooks
8.3.6. Render props
8.3.7. State management
8.3.8. Error boundaries
8.3.9. Styled Components
8.4. Rendering
8.4.1. What exactly is rendering
8.4.2. Server side rendering
8.4.2.1. NextJs
8.4.3. Client side rendering
8.5. Routing
8.5.1. What exactly is routing
8.5.2. Static routing
8.5.3. Dynamic routing
8.5.4. Client side routing
8.5.5. Server sider routing
8.5.6. Query/Query params
8.5.7. next/forward
8.5.8. Private/Protected routes
8.6. Refs
8.7. State management
8.7.1. Context
8.7.1.1. hooks
8.7.1.2. Provider
8.7.1.3. Consumer
8.7.2. Redux
8.7.2.1. Redux architecture
8.7.2.2. State/Store
8.7.2.3. Action
8.7.2.4. Action creator
8.7.2.5. Reducer
8.7.2.6. Redux persist
8.7.2.7. Middlewares
8.7.2.7.1. thunk
8.7.2.7.2. dev tool
8.7.2.8. Async operations
8.7.2.9. hooks