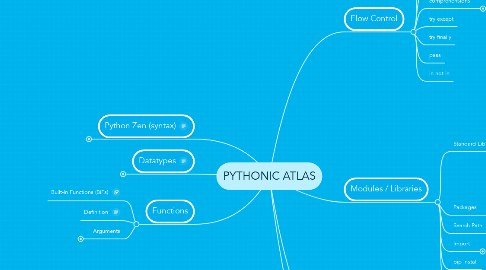
1. Python Zen (syntax)
1.1. Case-sensitive
1.1.1. a = 1 A = 2 print(a == A) # Evals to False
1.2. # Commenting
1.3. \t Code blocks
1.3.1. Are defined by their indentation
1.3.2. 1 tab or 4 spaces per indentation level
1.4. PEP-8 and Beyond
2. Datatypes
2.1. Strings
2.1.1. s1 = ‘:dog:\n’ s2 = "Dogge's home" s3 = """ Hello, Dogge! """ print(type(s1)) # <class 'str'> print("%s, %s, %s" % (s1, s2, s3)) # :dog: # , Dogge's home, # Hello, # Dogge!
2.1.2. Length
2.1.2.1. print(len(s1)) # 2
2.1.3. Slicing
2.1.3.1. s = 'study and practice' print('{0}:{1}'.format(s[:5], s[-8:])) # study:practice
2.1.4. Operator
2.1.4.1. +
2.1.4.2. print("abc" + "." + "xyz") # "abc.xyz"
2.1.5. Casting
2.1.5.1. print(str.__doc__)
2.1.5.1.1. """ str(object='') -> str str(bytes_or_buffer[, encoding[, errors]]) -> str """
2.1.5.2. print(str(3.14)) # "3.14" print(str(3)) # "3" print(str([1,2,3])) # "[1,2,3]" print(str((1,2,3))) # "(1,2,3)" print(str({1,2,3})) # "{1,2,3}" print(str({'python': '*.py', 'javascript': '*.js'})) "{'python': '*.py', 'javascript': '*.js'}"
2.2. Numbers
2.2.1. integer
2.2.1.1. a = 1 b = 0x10 # 16 print(type(a)) # <class 'int'>
2.2.2. float
2.2.2.1. c = 1.2 d = .5 # 0.5 g = .314e1 # 3.14 print(type(g)) # <class 'float'>
2.2.3. complex
2.2.3.1. e = 1+2j f = complex(1, 2) print(type(e)) # <class 'complex'> print(f == e) # True
2.2.4. Operators
2.2.4.1. + - * / % **
2.2.4.2. ## Operators: + - * / ** // % print(1 + 1) # 2 print(2 - 2) # 0 print(3 * 3) # 9 print(5 / 4) # 1.25 print(2 ** 10) # 1024 print(5 // 4) # 1 print(5 % 4) # 1
2.2.5. Casting
2.2.5.1. # Integer/String -> Float
2.2.5.1.1. print(float.__doc__)
2.2.5.1.2. print(float(3)) # 3.0 print(3 / 1) # 3.0 print(float("3.14")) # 3.14
2.2.5.2. # Float/String -> Integer
2.2.5.2.1. print(int.__doc__)
2.2.5.2.2. print(int(3.14)) # 3 print(int("3", base = 10)) # 3 print(int("1010", base = 2)) # 10 print(int("0b1010", base = 0)) # 10
2.3. Constants
2.3.1. True/False (bool)
2.3.1.1. True
2.3.1.2. False
2.3.1.3. print(type(True)) # <class 'bool'>
2.3.2. None
2.3.2.1. print(None is None) # True
2.3.2.2. print(type(None)) # <class 'NoneType'>
2.3.3. Ellipsis
2.3.3.1. The same as ... Special value used mostly in conjunction with extended slicing syntax for user-defined container data types.
2.3.4. NotImplemented
2.3.4.1. Special value which can be returned by the “rich comparison” special methods (__eq__(), __lt__(), and friends), to indicate that the comparison is not implemented with respect to the other type.
2.4. Iterables
2.4.1. String = ""
2.4.1.1. s1 = ‘:dog:\n’ s2 = "Dogge's home" s3 = """ Hello, Dogge! """ print(type(s1)) # <class 'str'> print("%s, %s, %s" % (s1, s2, s3)) # :dog: # , Dogge's home, # Hello, # Dogge!
2.4.1.2. Length
2.4.1.2.1. print(len(s1)) # 2
2.4.1.3. Slicing
2.4.1.3.1. s = 'study and practice' print('{0}:{1}'.format(s[:5], s[-8:])) # study:practice
2.4.1.4. Operator
2.4.1.4.1. +
2.4.1.4.2. print("abc" + "." + "xyz") # "abc.xyz"
2.4.1.5. Casting
2.4.1.5.1. print(str.__doc__)
2.4.1.5.2. print(str(3.14)) # "3.14" print(str(3)) # "3" print(str([1,2,3])) # "[1,2,3]" print(str((1,2,3))) # "(1,2,3)" print(str({1,2,3})) # "{1,2,3}" print(str({'python': '*.py', 'javascript': '*.js'})) "{'python': '*.py', 'javascript': '*.js'}"
2.4.2. List = [ ]
2.4.2.1. l = ['python', 3, 'in', 'one'] print(type(l)) # <class 'list'>
2.4.2.2. Length
2.4.2.2.1. print(len(l)) # 4
2.4.2.3. Slicing
2.4.2.3.1. print(l[0]) # 'python' print(l[-1]) # 'one' print(l[1:-1]) # [3, 'in']
2.4.2.4. Alter
2.4.2.4.1. l.append('pic') # None # l == ['python', 3, 'in', 'one', 'pic'] l.insert(2, '.4.1') # None # l == ['python', 3, '.4.1', 'in', 'one', 'pic'] l.extend(['!', '!']) # l == ['python', 3, '.4.1', 'in', 'one', 'pic', '!', '!'] print(l.pop()) # '!' # l == ['python', 3, '.4.1', 'in', 'one', 'pic', '!'] print(l.pop(2)) # '.4.1' # l == ['python', 3, 'in', 'one', 'pic', '!'] l.remove("in") # l == ['python', 3, 'one', 'pic', '!'] del l[2] # l == ['python', 3, 'pic', '!']
2.4.2.5. Index
2.4.2.5.1. print(l.index('pic')) # 2
2.4.3. Tuple = ( )
2.4.3.1. Immutable list
2.4.3.2. tp = (1, 2, 3, [4, 5]) print(type(tp)) # <class 'tuple'> ## Length print(len(tp)) # 4 print(tp[2]) # 3 tp[3][1] = 6 print(tp) # (1, 2, 3, [4, 6]) ## Single element tp = (1, ) # Not tp = (1)
2.4.3.3. assign multiple values
2.4.3.3.1. v = (3, 2, 'a') (c, b, a) = v print(a, b, c) # a 2 3
2.4.4. Dict = { }
2.4.4.1. dic = {} print(type(dic)) # <class 'dict'> dic = {'k1': 'v1', 'k2': 'v2'} ## Length print(len(dic)) # 2
2.4.4.2. print(dic['k2']) # 'v2' print(dic.get('k1')) # 'v1' print(dic.get('k3', 'v0')) # 'v0' dic['k2'] = 'v3' print(dic) # {'k1': 'v1', 'k2': 'v3'} print('k2' in dic) # True print('v1' in dic) # False
2.4.5. Set
2.4.5.1. st = {'s', 'e', 'T'} print(type(st)) # <class 'set'> ## Length print(len(st)) # 3 ## Empty st = set() print(len(st)) # 0 st = {} print(type(st)) # <class 'dict'>
2.4.5.2. Alter
2.4.5.2.1. st = set(['s', 'e', 'T']) st.add('t') # st == {'s', 'e', 't', 'T'} st.add('t') # st == {'s', 'e', 't', 'T'} st.update(['!', '!']) # st == {'s', 'e', 't', 'T', '!'} st.discard('t') # st == {'T', '!', 's', 'e'} # No Error st.remove('T') # st == {'s', 'e', '!'} # KeyError st.pop() # 's' # st == {'e'} st.clear() # st == set()
2.5. Bytes
2.5.1. list of ascii character
2.5.2. # 0-255/x00-xff
2.5.2.1. byt = b'abc' print(type(byt)) # <class 'bytes'> print(byt[0] == 'a')# False print(byt[0] == 97) # True
2.5.3. Length
2.5.3.1. print(len(byt)) # 3
2.6. Conversion
3. Functions
3.1. Built-In Functions (BiFs)
3.2. Definition
3.3. Arguments
3.3.1. Decorator
3.3.1.1. def log(f): def wrapper(): print("Hey log~") f() print("Bye log~") return wrapper @log def fa(): print("This is fa!") # Equal to... def fb(): print("This is fb!") fb = log(fb) fa() print("*"*10) fb()
3.3.1.1.1. # Hey log~ # This is fa! # Bye log~ # ********** # Hey log~ # This is fb! # Bye log~
3.3.2. Lambda
3.3.2.1. pairs = [(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')] pairs.sort(key=lambda pair: pair[1]) print(pairs) "[(4, 'four'), (1, 'one'), (3, 'three'), (2, 'two')]" pairs.sort(key=lambda pair: pair[0]) print(pairs) "[(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')]"
3.3.3. arbitrary arguments
3.3.3.1. def f(*args, **kargs): print("args ", args) print("kargs ", kargs) print("FP: {} & Scripts: {}".format(kargs.get("fp"), "/".join(args))) f("Python", "Javascript", ms = "C++", fp = "Haskell") # args ('Python', ‘Javascript’) # kargs {'ms': 'C++', 'fp': 'Haskell'} # FP: Haskell and Scripts: Python/Javascript
3.3.3.2. def f(*args, con = " & "): print(isinstance(args, tuple)) print("Hello", con.join(args)) f("Python", "C", "C++", con = "/") # True # "Hello Python/C/C++"
3.3.4. keyword arguments
3.3.4.1. def f(v, l = "Python"): """return '$v, $l'""" return "{}, {}!".format(v, l) print(f("Hello")) # "Hello, Python!" print(f("Bye", "C/C++")) # "Bye, C/C++!"
3.3.5. default arguments
3.3.5.1. def f(name = "World"): """return 'Hello, $name'""" return "Hello, {}!".format(name) print(f()) # 'Hello, World!' print(f("Python")) # 'Hello, Python!'
4. Flow Control
4.1. loops
4.1.1. while
4.1.1.1. prod = 1 i = 1 while i < 10: prod = prod * i i += 1 print(prod)
4.1.2. for
4.1.2.1. for i in "Hello": print(i)
4.1.3. break / continue
4.1.3.1. for n in range(2, 10): if n % 2 == 0: print("Found an even number ", n) continue if n > 5: print("GT 5!") break
4.1.4. Iterators / Generators
4.1.4.1. def reverse(data): for index in range(len(data)-1, -1, -1): yield data[index] nohtyp = reverse("Python") print(nohtyp) # <generator object reverse at 0x1029539e8> for i in nohtyp: print(i) # n # o # h # t # y # P
4.1.4.2. python = iter("Python") print(python) # <str_iterator object at 0x10293f8d0> for i in python: print(i) # P # y # t # h # o # n
4.2. if elif else
4.2.1. import sys if sys.version_info.major < 3: print("Version 2.X") elif sys.version_info.major > 3: print("Future") else: print("Version 3.X")
4.3. comprehensions
4.3.1. Dict
4.3.1.1. ls = {s: len(s) for s in ["Python", "Javascript", "Golang"]} print(ls) # {'Python': 6, 'Javascript': 10, 'Golang': 6} sl = {v: k for k, v in ls.items()} print(sl) # {10: 'Javascript', 6: 'Golang'}
4.3.2. Set
4.3.2.1. s = {2 * x for x in range(10) if x ** 2 > 3} print(s) # {4, 6, 8, 10, 12, 14, 16, 18} pairs = set([(x, y) for x in range(2) for y in range(2)]) print(pairs) # {(0, 1), (1, 0), (0, 0), (1, 1)}
4.3.3. List
4.3.3.1. pairs = [(x, y) for x in range(2) for y in range(2)] print(pairs) # [(0, 0), (0, 1), (1, 0), (1, 1)]
4.3.3.2. s = [2 * x for x in range(10) if x ** 2 > 3] print(s) #[4, 6, 8, 10, 12, 14, 16, 18]
4.4. try except
4.5. try finally
4.6. pass
4.7. in not in
5. Modules / Libraries
5.1. Standard Libraries
5.1.1. math
5.1.2. fractions
5.1.3. decimal
5.1.4. os
5.1.5. sys
5.1.6. random
5.2. Packages
5.2.1. """ MyModule/ |--SubModuleOne/ |--__init__.py |--smo.py # smo.py def run(): print("Running MyModule.SubModuleOne.smo!") """ from MyModule.SubModule import smo smo.run() # Running MyModule.SubModuleOne.smo!
5.3. Search Path
5.3.1. 1. current directory 2. echo $PYTHONPATH 3. sys.path
5.4. Import
5.4.1. import os print(os.name) # posix from sys import version_info as PY_VERSION print("VERSON: {}.{}".format(PY_VERSION.major, PY_VERSION.minor)) # VERSON: 3.5 from math import * print(pi) # 3.141592653589793
5.5. pip install
5.6. wheels
6. Debugging
6.1. error
6.1.1. EnvironmentError
6.1.2. EOFError
6.1.3. IOError
6.1.4. ImportError
6.1.5. IndexError
6.1.6. KeyError
6.1.7. MemoryError
6.1.8. NameError
6.1.9. NotImplementedError
6.1.10. OSError
6.1.11. OverflowError
6.1.12. ReferenceError
6.1.13. RuntimeError
6.1.14. StopIteration
6.1.15. SyntaxError
6.1.16. SystemError
6.1.17. TypeError
6.1.18. UnboundLocalError
6.1.19. UnicodeError
6.1.20. UnicodeEncodeError
6.1.21. UnicodeDecodeError
6.1.22. UnicodeTranslateError
6.1.23. ValueError
6.1.24. WindowsError
6.1.25. ZeroDivisionError
6.2. exception
6.2.1. Exception
6.2.2. BaseException
6.2.3. StandardException
6.2.4. ArithmeticException
6.2.5. LookupException
6.2.6. AssertionException
6.2.7. AttributeException
6.2.8. FloatingException
6.3. warning
6.3.1. Warning
6.3.2. UserWarning
6.3.3. DeprecatationWarning
6.3.4. PendingDeprecatationWarning
6.3.5. SyntaxWarning
6.3.6. RuntimeWarning
6.3.7. FutureWarning
6.3.8. ImportWarning
6.3.9. UnicodeWarning
6.4. other
6.4.1. GenerateExit
6.4.2. SystemExit
6.4.3. KeyboardInterrupt
7. rarely useful
7.1. Class (OOP)
7.1.1. Override
7.1.1.1. class Animal: """This is an Animal""" def __init__(self, can_fly = False): self.can_fly = can_fly def fly(self): if self.can_fly: print("I CAN fly!") else: print("I can not fly!") class Bird: """This is a Bird""" def fly(self): print("I'm flying high!") bird = Bird() bird.fly() # I'm flying high!
7.1.2. Inheritance
7.1.2.1. class Animal: """This is an Animal""" def __init__(self, can_fly = False): self.can_fly = can_fly def fly(self): if self.can_fly: print("I CAN fly!") else: print("I can not fly!") class Dog(Animal): """This is a Dog""" def bark(self): print("Woof!") d = Dog() d.fly() # I can not fly! d.bark() # Woof!
7.1.3. Instance
7.1.3.1. class Animal: pass class Human: pass a = Animal() h = Human() print(isinstance(a, Animal)) # True print(isinstance(h, Animal)) # False
7.1.4. __init__ & self
7.1.4.1. class Animal: """This is an Animal""" def __init__(self, can_fly = False): print("Calling __init__() when instantiation!") self.can_fly = can_fly def fly(self): if self.can_fly: print("I CAN fly!") else: print("I can not fly!") a = Animal() # Calling __init__() when instantiation! a.fly() # I can not fly! b = Animal(can_fly = True) # Calling __init__() when instantiation! b.fly() # I CAN fly!
7.1.5. Class
7.1.5.1. class Animal: """This is an Animal""" def fly(_): print("I can fly!") a = Animal() a.fly() # I can fly! print(a.__doc__) # This is an Animal