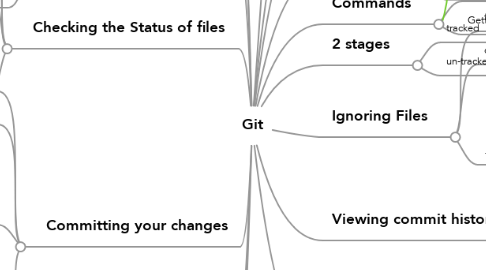
1. Everything in Git is checksummed before it is stored
1.1. And referred to by that checksum.
1.2. SHA-1 hash is the mechanism used for checksumming
1.2.1. 40 char string made of hex chars.
2. 3 Sections
2.1. 3 main sections of any Git project
2.2. Working directory
2.2.1. single checkout of one version of the project
2.3. Staging area
2.3.1. A simple file
2.3.2. generally inside Git directory
2.3.3. Stores information about what will go into your next commit
2.4. Git directory
2.4.1. Git stores meta data and object database for the project
3. First time Git Setup
3.1. Git comes with a tool called 'git config'
3.1.1. lets you set configuration variables
3.2. The config vars are stored in 3 different places
3.2.1. /etc/gitconfig
3.2.1.1. contains values for every user on the system and their respos
3.2.1.2. to read/write from this file
3.2.1.2.1. use 'git config --system ....'
3.2.2. ~/.gitconfig
3.2.2.1. specific to user
3.2.2.2. to read/write to this file
3.2.2.2.1. use 'git config --global ...'
3.2.3. .git/config
3.2.3.1. specific to single respository
4. Setting up a Repository
4.1. Add a project to a Git repo
4.1.1. initializing a repo in existing folder
4.1.1.1. $git init
4.1.1.1.1. creates a.git directory
4.1.1.1.2. nothing is tracked yet (until the first commit)
4.1.1.2. Adding files to git
4.1.1.2.1. $git add *.c
4.1.1.2.2. $git add README
4.1.1.2.3. $git commit -m 'initial project version'
4.2. Clone an existing Git repo(from other server)
4.2.1. $git clone git://github.com/akbar/proj.git
4.2.1.1. creates a directory /proj
4.2.1.2. Also checks out a working copy
5. Checking the Status of files
5.1. $git status
5.2. tracking new files
5.2.1. $git add FILENAME
5.3. staging changed files
5.4. Viewing staged and unstaged changes
5.4.1. $git status is too vague
5.4.2. use $git diff
5.4.3. To see whats changed but not staged
5.4.3.1. use $git diff
5.4.4. To see whats staged and going into commit
5.4.4.1. use $git diff --cached
5.4.4.2. or use (>1.6.1) $git diff --staged
6. Committing your changes
6.1. $git commit
6.2. Skipping staging area
6.2.1. $git commit -a
6.3. Removing files
6.3.1. $git rm FILENAME
6.3.1.1. removes it from Stage and working copy
6.3.2. $rm FILENAME
6.3.2.1. removes it in working dirctory
6.3.2.2. needs to be staged and committed
6.3.3. a scenario
6.3.3.1. If you make changes to a file and don't stage and want to delete
6.3.3.1.1. use $git rm FILENAME -f
6.3.4. Removing it only from stage
6.3.4.1. $git rm --cached FILENAME
6.4. Moving files
6.4.1. Git doesn't track file movement
6.4.2. If you rename a file
6.4.2.1. no meta data is stored in Git to capture that
6.4.3. command
6.4.3.1. $git mv file_from file_to
7. Undoing things
7.1. changing your last commit
7.1.1. $git commit --amend
7.1.2. takes staging area and used for commit
7.1.3. snapshot will look exactly the same
7.2. unstaging a staged file
7.2.1. $git reset HEAD filename
7.3. Unmodifying a modified file
7.3.1. $git checkout -- filename
7.3.2. be careful - you modified working copy changes would be lost
8. Tagging
8.1. Listing your tags
8.1.1. $git tag
8.1.2. $git tag -l v1.4.2 (searches)
8.2. Creating Tags
8.2.1. 2 types of tags
8.2.1.1. lightweight
8.2.1.1.1. like a branch that doesn't change
8.2.1.1.2. a pointer to a specific commit
8.2.1.2. annotated
8.2.1.2.1. stored as full objects in Git database
8.2.1.2.2. check-summed
8.2.1.2.3. contains tagger name
8.2.1.2.4. email
8.2.1.2.5. date
8.2.1.2.6. have a tagging message
8.2.1.2.7. can be signed with GPG
8.2.1.2.8. creating
8.3. $git show [tag-name]
9. A distributed version control system
10. 3 States
10.1. 3 main states that files can reside
10.2. modified
10.2.1. changed the file, but not committed
10.3. staged
10.3.1. marked a modified file in it's current version to go into next commit snapshot
10.4. committed
10.4.1. data is stored in the local database
11. Workflow
11.1. 1. Modify files in working directory
11.2. 2. Stage the files, adding snapshots of them to your staging area
11.3. 3. Do a commit - which takes the files as they are in staging area and stores that snapshot permanently in Git directory
12. Commands
12.1. git config --list
12.2. Getting help
12.2.1. git help <verb>
13. 2 stages
13.1. tracked
13.1.1. are files that were from last snapshot
13.2. un-tracked
14. Ignoring Files
14.1. files you don't want to add
14.2. or show as being untracked
14.3. .gitignore file
14.3.1. blank lines are ignored
14.3.2. lines with # are ignored
14.3.3. standard glob patterns work
14.3.4. patterns ending with / indicate folder
14.3.5. ! - to negate a pattern
15. Viewing commit history
15.1. $git log
16. Remote repositories
16.1. checking the remote for existing repo
16.1.1. $git remote -v
16.2. adding remote repos
16.2.1. $git remote add [shortname] [url of repo]
16.2.2. This gives a right to fetch the git repo into local repo
16.2.3. Ex: $git remote add pb git://github.com/akbar/myproj.git
16.2.3.1. $git fetch pb
16.3. Fetching and Pulling from remotes
16.3.1. $git fetch [remote-name]
16.3.2. fetch only pulls data to local repo - doesn't merge with working directory
16.3.3. $git pull
16.3.3.1. pull fetches the data and merges with working copy/branch
16.4. Pushing to remotes
16.4.1. $git push [remote-name] [branch-name]
16.5. Inspecting remotes
16.5.1. $git remote show [remote-name]
16.6. Removing and renaming remotes
16.6.1. $git remote renamte [old] [new]
16.6.2. $git remote rm [remote-name]