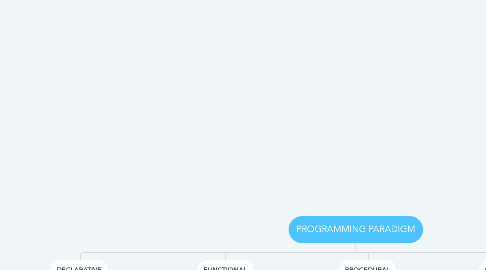
1. DECLARATIVE
1.1. While imperative programming uses instructions to tell the computer what to do, step by step, declarative pure functional programming uses expressions that can be evaluated by the computer in any order that respects mathematical rules like operator priority, associativity to the left or right, parenthesis, so forth. This liberates programming from the low level concern of micro managing cpu, memory, threads, and instead allows a higher level abstraction to programs. Because expressions can be evaluated in any order respecting math, a powerful choice is to evaluate every expression in a lazy manner i.e. evaluate it only when required (e.g. its value is needed to produce output directly or indirectly).
1.1.1. Taking I/O for instance, monads make it possible to do I/O either fully functionally lazy manner, either imperative style, either combining the benefits of functional lazy (composability) with those of imperative style (deterministic resource control), and from that combination results such patterns as Iteratees, conduits and pipes.
1.1.1.1. The side effects are contained in a type safe functional language, and do not have the contagious effect. They need to be explicit, first-class. The language is transparent to the side effects, and functions signature contains the side effects. The majority of functionality can be coded declaratively with pure functions and combinators that lift functions to abstract types. A minority of still pure functions deal with side effects in a contained manner that captures them in types monadically. The runtime system actually does side effects but in a much more sensible manner than in imperative platforms.
2. EVENT DRIVEN
2.1. Imagine that you’re building the interface for a photo editor. You want, for example, that every time a user clicks in the pencil tool, the pointer of the mouse changes to express that decision. You want that every time that the user clicks in canvas, the current shape of the pointer is drawn in the location of the canvas that was clicked. You want that every time that a user presses ctrl+z, the last action is undone. To do all that, you configure what is called events. The user clicks in a button? An event is fired. The user presses some keys? An event is fired. What you do is to program the reaction for those events. That’s called event driven programming. Although commonly used when building UIs, it can be present on any kind of program. For example, Node.JS is a framework built around the idea of event driven programming.
2.1.1. The advantage of event-driven programs is that they can process and control enormously complex clusters of events, that can be organized around some metaphor (such as the Windows “desktop”) so that human beings and other programs perceive a unified and simple interface that is also remarkably flexible and powerful.
2.1.1.1. The Disadvantages of event-driven program: -Fast moving, unstable API. -Smaller/newer ecosystem of libraries/frameworks. -Fewer hosting options. -Fewer learning resources (books, documentation, etc). -Fewer developers know it if you need to hire people.
3. OBJECT-ORIENTED
3.1. or OOP, is an approach to problem-solving where all computations are carried out using objects. An object is a component of a program that knows how to perform certain actions and how to interact with other elements of the program. Objects are the basic units of object-oriented programming. A method in object-oriented programming is like a procedure in procedural programming. The key difference here is that the method is part of an object. In object-oriented programming, you organize your code by creating objects, and then you can give those objects properties and you can make them do certain things.
3.1.1. The disadvantages of using object oriented programs is that they tend to require more memory and runtime efficiency.
4. PROCEDURAL
4.1. uses a list of instructions to tell the computer what to do step-by-step. Procedural programming relies on - you guessed it - procedures, also known as routines or subroutines. A procedure contains a series of computational steps to be carried out. Procedural programming is also referred to as imperative programming. Procedural programming languages are also known as top-down languages. If you want a computer to do something, you should provide step-by-step instructions on how to do it. It is, therefore, no surprise that most of the early programming languages are all procedural. Examples of procedural languages include Fortran, COBOL and C, which have been around since the 1960s and 70s.
4.1.1. Advantages of functional programming over OOP are: -Its relative simplicity, and ease of implementation of compilers and interpreters -The ability to re-use the same code at different places in the program without copying it. -An easier way to keep track of program flow. -The ability to be strongly modular or structured. -Needs only less memory.
4.1.1.1. These are balanced by several disadvantages: -Data is exposed to whole program, so no security for data. -Difficult to relate with real world objects. -Difficult to create new data types reduces extensibility. -Importance is given to the operation on data rather than the data.
5. FUNCTIONAL
5.1. a style of building the structure and elements of computer programs—that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It is a declarative programming paradigm in that programming is done with expressions or declarations instead of statements.
5.1.1. Benefits of functional programming in general Experienced functional programmers make the following claims about functional programming, regardless of the language they use: -Pure functions are easier to reason about -Testing is easier, and pure functions lend themselves well to techniques like property-based testing -Debugging is easier -Programs are more bulletproof -Programs are written at a higher level, and are therefore easier to comprehend -Function signatures are more meaningful -Parallel/concurrent programming is easier -Being able to (a) treat functions as values and (b) use anonymous functions makes code more concise, and still readable -Scala syntax generally makes function signatures easy to read -The Scala collections’ classes have a very functional API -Scala runs on the JVM, so you can still use the wealth of JVM-based libraries and tools with your Scala/FP applications
5.1.1.1. Drawbacks of functional programming in general: -Writing pure functions is easy, but combining them into a complete application is where things get hard. -The advanced math terminology (monad, monoid, functor, etc.) makes FP intimidating. -For many people, recursion doesn’t feel natural. -Because you can’t mutate existing data, you instead use a pattern that I call, “Update as you copy.” -Pure functions and I/O don’t really mix. -Using only immutable values and recursion can potentially lead to performance problems, including RAM use and speed. -You can mix FP and OOP styles. -Scala doesn’t have a standard FP library.