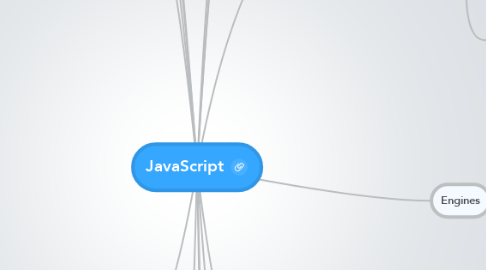
1. Objects
1.1. Window
1.1.1. Methods
1.1.1.1. onload
1.1.1.2. onresize
1.1.1.3. onkeyup
1.1.1.4. onerror
1.1.2. Global scope (browser-based JS)
1.1.3. Implemented as JS object
1.1.4. Fields
1.1.4.1. parent
1.1.4.2. screen
1.1.4.3. window
1.1.4.3.1. current window
1.2. Document
1.2.1. Reference to HTML DOM els
1.2.2. Not JS
1.2.3. Fields
1.2.3.1. cookie
1.2.3.1.1. Usage: window.document.cookie
1.2.3.2. activeElement
1.2.3.2.1. focused element
1.2.3.3. anchors
1.2.3.4. body
1.2.3.5. defaultCharset
1.2.3.6. documentElement
1.2.3.6.1. link to itself
1.2.3.7. location
1.2.3.7.1. changing forces refresh
1.2.3.8. URL
1.2.3.8.1. read-only
1.2.3.9. parentWindow
1.2.3.10. referrer
1.2.3.11. scripts
1.2.3.12. width
1.2.4. One instance for web page
1.2.5. Methods
1.2.5.1. ready
1.2.5.1.1. DeferredBinding analog
1.3. Hierarchy
1.3.1. Navigator
1.3.1.1. MimeType
1.3.1.2. Window
1.3.1.2.1. Frame
1.3.1.2.2. History
1.3.1.2.3. Location
1.3.1.2.4. Document
1.3.1.3. Plugin
1.4. Functions
1.4.1. Function closure
1.4.1.1. Acts as anonymous class in java
2. Language notes
2.1. No {} scope like in other langs
2.2. OOP
2.2.1. Instead of class/instance - Object
2.2.2. Instead of super: __proto__
2.3. Functions have an attribute called prototype
2.3.1. Immutable
2.4. All variables are function-local. Even in loops!
2.5. Also has gc
2.6. Functions are classes
3. Browser compatibility
3.1. Events
3.1.1. http://www.quirksmode.org/dom/events/index.html
3.2. Autohead
3.2.1. Automatical creating 'head' element even if it doesn't exist
3.2.2. Disabled in android, iPhome, Opera 8
3.2.2.1. That's why adding script to head from code may fail here
3.3. When you modify body from script which isn't a part of it - you get 'Operation aborted' in IE
3.4. Correct insertion of script
3.4.1. var first = document.getElementsByTagName('script')[0]; first.parentNode.insertBefore(js, first);
3.5. IE
3.5.1. GET caching in IE
3.5.1.1. Usage of hashes in links
3.5.1.2. set expire flag
3.5.2. Operation aborted
3.5.2.1. Change parent while it hasn't been constructed yet
3.5.3. Doesn't have capturing
3.5.4. Doesn't have w3c event registration mechanism (addEventListener)
4. Debug
4.1. Chrome should be started with debug option
5. Patterns
5.1. See JavaScript MVC
6. Tools
6.1. yui compressor
6.1.1. Obfuscates and compressed
6.1.1.1. short names
6.1.1.2. css compression
7. AJAX
7.1. Pure implementation
7.1.1. XMLHttpRequest
7.1.1.1. Methods
7.1.1.1.1. abort()
7.1.1.1.2. getAllResponseHeaders()
7.1.1.1.3. getResponseHeader(headerName)
7.1.1.1.4. open(method, URL, async, userName, password)
7.1.1.1.5. send(content)
7.1.1.1.6. setRequestHeader(type, value)
7.1.1.2. Fields
7.1.1.2.1. readystate
7.1.1.2.2. responseText
7.1.1.2.3. responseXML
7.1.1.2.4. status
7.2. Frameworks
7.2.1. GWT
7.2.2. Dojo
7.2.3. jQuery
8. History handling
8.1. Native
8.1.1. History object
8.1.1.1. Fields
8.1.1.1.1. current
8.1.1.1.2. next
8.1.1.1.3. prev
8.1.1.1.4. length
8.1.1.2. Methods
8.1.1.2.1. forward()
8.1.1.2.2. back()
8.1.1.2.3. go(link)
8.1.2. Managing
8.1.2.1. In jQuery its used using iframe and timert
9. DOM
9.1. See native support
10. Inside
10.1. Browser events
10.1.1. Types
10.1.1.1. DOM events
10.1.1.1.1. onclick
10.1.1.1.2. mouseover
10.1.1.2. Window events
10.1.1.2.1. resize
10.1.1.3. Other events
10.1.1.3.1. load(handler)
10.1.1.3.2. readystatechange
10.1.2. Working with
10.1.2.1. addEventListener
10.1.2.2. removeEventListener
10.1.2.3. detachEvent
10.1.2.4. Use event as a parameter in fuction
10.1.3. Event bubbling
10.1.3.1. Parents handle child events
10.1.3.2. Slows down gwt code and huge widget hierarchies
10.1.4. Methods
10.1.4.1. stopPropagation
10.1.4.1.1. to protect from handle bubbling
10.1.4.2. preventDefault
10.1.4.2.1. prevent default action handling (example: anchor click not redirects to URI)
10.1.5. DOM standart
10.1.5.1. First - capture event
10.1.5.2. Then - bubble
10.1.5.3. ! not all the browsers use this standart
10.1.6. Prevent unnecessary bubbling
10.1.6.1. if (event.target == this) {}
10.1.6.2. event.stopPropagation()
10.1.7. Removal
10.1.7.1. unbind('name')
10.1.7.1.1. Example: unbind('click')
10.1.8. Rebind
10.1.8.1. bind('name', handlerFunction)
10.1.9. Simulating user actions
10.1.9.1. trigger(eventName)
10.1.9.1.1. Example: trigger('click')
10.1.10. Hierarchy
10.1.10.1. 4 Model registration models
10.1.10.1.1. inline
10.1.10.1.2. traditional
10.1.10.1.3. w3c
10.1.10.1.4. Microsoft
10.1.10.2. Event access
10.1.10.2.1. function(event)
10.1.10.2.2. Cross-browser
10.1.10.3. 2 Event order models
10.1.10.3.1. Event capturing
10.1.10.3.2. Even bubbling
10.2. Execution context
10.2.1. call() and apply() methods
10.2.1.1. Example: someFunction().call(objectB)
10.2.1.1.1. 'this' will now link to objectB instance
10.3. Event loop
10.3.1. Thread by browser checking event states
10.3.2. Executed using event queue
11. Server side
11.1. Node.js
11.1.1. Socket.io library
11.1.2. Event-driven
11.1.3. Non-blocking
11.1.4. Being used
11.1.4.1. eBay
11.1.4.2. Microsoft
11.1.5. May be used
11.1.5.1. Creating web servers
11.1.5.1.1. HTTP servers are very easy
11.1.6. Execution
11.1.6.1. node program.js
11.1.7. Objects
11.1.7.1. global
11.1.7.1.1. process
11.1.7.1.2. console
11.1.8. STDIO
11.1.8.1. using console object
11.1.9. Other features
11.1.9.1. Buffers
11.1.9.2. DNS work
11.1.9.3. HTTP utils
11.1.9.4. FS
11.1.9.5. SSL
11.1.9.6. ...
12. Other notes
12.1. window.onload may be called when DOM hasn't been constructed
12.1.1. Use document.ready
12.2. To prevent from default event handling use return false;
12.2.1. Cases like a href="bla" and $(a).click(function() {bla2})
13. jQuery
14. JavaScriptMVC
14.1. MVC mechanism
14.1.1. jQueryMX
14.1.1.1. $.Model
14.1.1.1.1. encapsulates app model
14.1.1.2. $.View
14.1.1.3. $.Controller
14.2. Dependency management
14.2.1. StealJS
14.2.1.1. Loads several modules, prevents from reloading
14.2.1.2. Single load point
14.2.1.3. Usage
14.2.1.3.1. steal('widgets/tabs.js', './style.css', function(){ $('#tabs ).tabs(); });
14.3. Functional, unit testing
14.3.1. FuncUnit
14.3.1.1. Asserts
14.3.1.1.1. test("counter", function() { ok(Conctacts.first().name, "there is a name property"); equal(Contacts.counter(), 5, "there are 5 contacts"); });
14.3.1.2. Methods
14.3.1.2.1. setup
14.3.1.2.2. test
14.3.1.2.3. teardown
15. Engines
15.1. Google v8
15.1.1. Used in Chrome
15.2. Main features
15.2.1. Memory allocation
15.2.2. Compile
15.2.3. GC