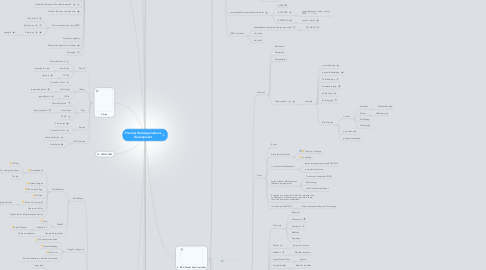
1. Preface
1.1. Greeting
1.1.1. Welcome!
1.1.2. Добре дошли!
1.1.3. Καλώς ήρθατε!
1.1.4. Hoşgeldiniz!
1.2. Who am I?
1.2.1. Stoyan Cheresharov :)
1.2.1.1. 15+ years in Web Applications Development
1.2.1.2. E-mail: [email protected]
1.2.1.3. Twitter: @wingman0070
1.2.1.4. Site: http://www.coolcsn.com/
1.3. Thoughts
1.3.1. We are on this planet to give love and be happy!
1.3.2. We are drops from the same ocean put in different vessels!
1.3.3. The world is inside of us. We are the creators of our life.
1.3.4. "Nothing makes sense, but it is very important to keep doing it!" Mahatma Gandhi
1.4. Live video streaming
1.4.1. On YouTube
1.4.1.1. 9:15 - 12:30 BG time (7:15 - 10:30 GMT) Every Friday Starting January 11, 2013 on Bulgarian 9:15-10:37:30 11:07:30 - 12:30
1.4.1.2. Links to be added later
1.4.1.3. My Channel
1.4.2. Using Google+
1.4.2.1. Hangout
1.4.2.1.1. 9 people online
1.5. Adventure
1.5.1. Web Development is a Beautiful Journey in a Magical Forest!
1.5.1.1. It changes as we go!
1.5.1.2. It becomes more friendly
1.6. Mind Maps
1.6.1. Link to this map
1.6.2. Tony Buzan
1.6.2.1. Answers How to learn and remember.
1.6.2.2. Mind Maps
1.6.2.3. Supermind
1.6.2.4. How to read fast and remember everything I want?
1.6.2.5. ...more
1.6.3. Web Based, Cloud
1.6.3.1. www.mind42.com
1.6.3.1.1. free
1.6.3.1.2. No presentations
1.6.3.1.3. No google drive integration
1.6.3.1.4. Adds
1.6.3.2. www.mindmeister.com
1.6.3.2.1. free for 3 maps max
1.6.3.2.2. Works with Google Drive
1.6.3.2.3. Your own pictures upload
1.6.3.2.4. Presentations
1.6.4. Popular Free Softwares
1.6.4.1. FreeMind
1.6.4.2. XMind
1.6.5. Tantek Çelik
1.6.5.1. IETF
1.6.6. Use it everywhere
1.6.6.1. Taking notes
1.6.6.2. Planing
1.6.6.3. Presenting
1.6.6.4. Brainstorming
1.6.6.5. ...more
1.6.7. Own cloud
1.7. Inovations
1.7.1. Web Based, Cloud Technologies
1.7.1.1. Mind Maps
1.7.1.2. Presentations
1.7.1.3. Live Streaming
1.7.1.4. Recording
1.7.1.5. Google+
1.7.1.6. Hangout
1.7.1.7. Cloud Based SDLC
1.7.2. University is to try the cutting edge tehnologies etc.
1.7.3. 21 century education
1.7.4. Everything in this series is new.
1.7.5. We will discover and learn things together.
1.8. Acknowledgments
1.8.1. доц. д-р Гъров
1.8.1.1. Gave me the opportunity
1.8.2. Тодор Величков
1.8.2.1. Inspired me
1.9. Copyrights
1.10. Organization
1.10.1. Introduction
1.10.2. Content
1.10.3. Summary
1.10.4. Q&A
1.11. Hangout on Air
2. 1. Zend Framework. Easy, Simple, Fast!
2.1. Introduction
2.1.1. LAMP
2.1.1.1. Linux
2.1.1.2. Apache web server
2.1.1.3. MySQL
2.1.1.4. PHP
2.1.2. Approaches
2.1.2.1. Computer
2.1.2.1.1. Installed
2.1.2.1.2. Portable
2.1.2.2. Cloud
2.1.2.2.1. Work from anywhere
2.1.2.2.2. Use any device
2.2. Let's get our hands dirty
2.2.1. Install XAMPP
2.2.1.1. Method A: Installation with the Installer
2.2.1.2. Method B: "Installation" without the Installer
2.2.1.2.1. 1. Download the zip file
2.2.1.2.2. 2. Unzip to the portable or local device (e.g. x:\xampp)
2.2.1.2.3. 3. Check if there is a service running on port 80
2.2.1.2.4. 4. Skype is usualy causing problems
2.2.1.2.5. 5. Go to the folder and run "apache_start.bat"
2.2.1.2.6. 6. Open the browser and type in the URL filed "localhost"
2.2.2. CGI
2.2.2.1. Pearl
2.2.2.2. PHP
2.2.2.3. BAT files
2.2.2.4. C
2.2.2.5. C++
2.2.2.6. Java + BAT files
2.2.2.6.1. bat
2.2.2.7. Fortran
2.2.2.8. ...more
2.2.3. ZF1
2.2.3.1. http://localhost
2.2.3.1.1. phpinfo
2.2.3.2. Download ZF1
2.2.3.3. Extract and copy Zend to H:\xampp\php\pear folder
2.2.3.4. Copy zf.bat in /xamp/php
2.2.3.5. Prepare the environment variables
2.2.3.6. create a project
2.2.3.7. create a virtual server
2.2.3.7.1. H:\xampp\apache\conf\extra\httpd-vhosts.conf
2.2.4. ZF2
2.2.4.1. Download ZF2
2.2.4.2. Extract and copy Zend to H:\xampp\php\pear\ZF2
2.2.4.3. Add to httpd.conf or H:\xampp\apache\conf\extra\httpd-vhosts.conf
2.2.4.3.1. alternative is to add it to.htaccess
2.2.4.4. ZendSkeletonApplication
2.2.4.4.1. Click on download as Zip
2.2.4.4.2. Unzip in the projects folder
2.2.4.5. Create a virtual host
2.2.4.5.1. H:\xampp\apache\conf\extra\httpd-vhosts.conf
2.2.4.6. Optional Read and follow Tutorial App
2.2.5. ZF2 and modules with composer
2.2.5.1. Install composer
2.2.5.1.1. SET PATH
2.2.5.1.2. Make sure SSL is enabled in php.ini
2.2.5.1.3. cd /xampp/php
2.2.5.1.4. Install composer
2.2.5.1.5. Create bath file
2.2.5.1.6. Make sure composer works
2.2.5.2. Install ZF2 skeleton App
2.2.5.2.1. Install
2.2.5.2.2. Alternative
2.2.5.3. Modules Installation
2.2.5.3.1. DoctrineModule
2.2.5.3.2. Doctrine ORM or ODM
2.2.5.3.3. Zfcuser
2.2.6. ZF2 with zftool
2.2.6.1. install zftool with composer
2.2.6.2. Manual zip from github
2.2.6.3. zftool.phar
2.2.7. phpcloud
2.2.7.1. dispaly_errors
2.2.7.1.1. index.php
2.2.7.1.2. module.config.php
2.3. Development Stacks
2.3.1. XAMPP
2.3.1.1. No xDebug installed in the latest versions
2.3.1.2. pros
2.3.1.2.1. Doesn't start with the sstartup
2.3.1.3. cons
2.3.1.3.1. no Xdebug
2.3.2. WAMP
2.3.2.1. Download
2.3.2.2. Microsoft Visual C++ 2010 SP1 Redistributable Package (x86)
2.3.2.3. WAMP server 2.4 not working on Virtual machine Win XP SP3 512 MB RAM
2.3.2.3.1. solution is to increase the RAM
2.3.2.4. config is in
2.3.2.4.1. C:\wamp\bin\apache\Apache2.4.4\conf
2.3.3. easyphp
2.3.4. Zend Server
2.3.4.1. Download
2.3.4.1.1. Zend Server (PHP 5.4)
2.3.4.1.2. Zend Server (PHP 5.4)
2.3.4.2. Zend Server Free Edition
2.3.4.3. Zend Server CE
2.3.4.4. Without License or Expired License is CE
2.3.5. If you are using PHP 5.4 or above - CLI server (cli-server)
2.3.5.1. php -S 0.0.0.0:8080 -t public/ public/index.php
2.3.6. Display Errors
2.3.6.1. Add to public/index.php
2.4. IDEs
2.4.1. Notepad++
2.4.1.1. Portable
2.4.1.2. Plugins
2.4.1.2.1. php script engine
2.4.1.2.2. Autocompletion for PHP
2.4.1.2.3. DBGP plugin
2.4.2. Eclipse PDT
2.4.2.1. Zend All-in-ones is Portable. Just unzip and use
2.4.2.2. PHP Development Tools
2.4.2.3. All-in-ones download
2.4.2.4. Zend All-in-ones PDT 3.2.0 w/Eclipse Indigo
2.4.2.5. Debuggers
2.4.2.5.1. Xdebug
2.4.2.5.2. Zend Debugger
2.4.2.6. Eclipse Downloads
2.4.2.7. pros
2.4.2.7.1. ZF2 project
2.4.2.7.2. integrates with Zend Server
2.4.2.7.3. Creates the sceleton app in the IDE
2.4.2.8. cons
2.4.2.8.1. for Git SVN we need to install plugins
2.4.2.9. EGit (on top of JGit) for Eclipse
2.4.2.9.1. WiKi
2.4.2.9.2. HowTo install it
2.4.2.9.3. Install
2.4.2.9.4. HoTo
2.4.2.9.5. But Not recommended and still need TortoiseGit for SSH
2.4.3. Dreamwaver
2.4.4. Zend Studio
2.4.5. NetBeans
2.4.5.1. PHP and HTML5 Learning Trail
2.4.5.2. NetBeans PHP
2.4.5.3. features
2.4.5.4. Download
2.4.5.5. You need JDK 6 or higher
2.4.5.6. Zend Tutorial App in NetBeans
2.4.5.7. pros
2.4.5.7.1. Has Git and SVN
2.4.5.8. cons
2.4.5.8.1. ZF2 is not supported yet (but could be done trough ZF.bat)
2.4.5.9. SSH git
2.4.5.10. Generating an SSH Key
2.4.5.10.1. Generating And Using RSA key on Windows
2.4.5.11. RentAFlat ZF1 tutorial app
2.4.5.11.1. There is a Readme.html in the root of the project
2.4.6. Microsoft Notepad
2.4.7. Apple TextMate
2.4.8. Linux vi
2.4.9. Sublimetext
2.4.10. Aptana Studio
2.4.11. Linux vim
2.4.12. phpDesigner
2.4.13. JetBrains PHPStorm
2.4.13.1. phpStorm
2.4.13.2. It's bit pricey though, unfortunately
2.4.14. ActiveState Komod
2.4.15. PHPEdit
2.4.16. RapidPHP
2.4.17. RadPHP (formerly Delphi for PHP)
2.4.18. OpenKomodo
2.4.19. Comparison
2.4.19.1. Seven great PHP IDEs compared
2.4.19.2. List in WiKi
2.5. Debuggers
2.5.1. Zend Debugger
2.5.1.1. proprietary protocol
2.5.2. XDebug
2.5.2.1. DBGP protocol
2.5.2.2. Remote Debugging
2.5.2.3. Profiling
2.5.2.3.1. Outputs cachegrind compatible file
2.5.2.3.2. Clients
2.5.2.4. Clients
2.5.2.5. Xdebug extensions for web browsers
2.5.2.6. Start a session
2.6. Repositories (SCM, VCS)
2.6.1. Distributed
2.6.1.1. Git
2.6.1.1.1. Linus Torvalds
2.6.1.1.2. Implementations
2.6.1.1.3. Version Control Hosting Services
2.6.1.1.4. RSA key pairs for git (Installing SSH keys on Windows)
2.6.1.1.5. Configuration (Setting up Git profile)
2.6.1.2. Mercurial
2.6.1.3. Bazaar
2.6.2. Central server
2.6.2.1. SVN (Subversion)
2.6.2.2. CVS
2.7. Cloud
2.7.1. Virtualization + web services
2.7.1.1. hypervisor
2.7.2. Categories by what they offer
2.7.2.1. IaaS
2.7.2.1.1. AWS
2.7.2.2. PaaS
2.7.2.2.1. phpcloud.com
2.7.2.2.2. CloudBees
2.7.2.2.3. CloudFoundry
2.7.2.2.4. Appfog
2.7.2.2.5. Heroku
2.7.2.2.6. OpenShift
2.7.2.2.7. Google App Engine
2.7.2.2.8. Elastic Beans Talk
2.7.2.2.9. Microsoft Azure
2.7.2.3. SaaS
2.7.2.3.1. Zoho
2.7.3. Categories by location
2.7.3.1. Private
2.7.3.2. Public
2.7.3.3. Hybrid
2.8. Cloud Approaches
2.8.1. IDE
2.8.1.1. Native apps
2.8.1.2. Web based (Cloud, HTML5)
2.8.1.2.1. Requrments
2.8.1.2.2. Will detach us from the device
2.8.2. VMware
2.8.2.1. Zend Image
2.8.2.1.1. We control it
2.8.3. VNC
2.8.3.1. VPN
2.8.3.1.1. I have to install native apps
2.8.4. Web Desktop, Web (Browser) OS
2.8.4.1. Lucid (Dojo)
2.8.4.2. ExtTop
2.8.5. HTML5
2.8.5.1. CSS3
2.8.5.2. HTML5
2.8.5.3. JS (ES5)
2.8.5.3.1. Alternatives???
2.9. Cloud Development Stacks
2.9.1. phpcloud.com
2.9.1.1. Managing your SSH keys
2.9.1.1.1. PEM RSA keypair
2.9.1.1.2. PPK RSA keypair
2.9.1.2. Connecting via Git
2.9.1.3. Connecting via SFTP
2.9.1.3.1. WinSCP
2.9.1.4. Setting up the Debugger
2.9.1.4.1. starting the debugger
2.9.1.5. Connecting to the Database
2.9.1.6. tools
2.9.1.6.1. OpenSSH
2.9.1.6.2. WinSCP
2.9.1.6.3. PuTTy
2.9.1.6.4. Git
2.9.1.6.5. MySQL
2.9.1.6.6. IDEs
2.9.1.6.7. Browser Plugins
2.9.1.6.8. XDebug
2.9.2. phpfog.com appfog.com
2.10. Cloud IDEs
2.10.1. Cloud 9
2.10.1.1. What it is according to Wikipedia?
2.10.1.2. Public code repositories
2.10.1.2.1. GitHub
2.10.1.2.2. BitBucket
2.10.1.3. Works with
2.10.1.3.1. Mercurial repositories
2.10.1.3.2. Git repositories
2.10.1.3.3. FTP servers
2.10.1.4. Support for deployment (PaaS)
2.10.1.4.1. Heroku
2.10.1.4.2. Joyent
2.10.1.4.3. Windows Azure
2.10.1.5. Features
2.10.1.6. OpenId
2.10.1.6.1. GitHub
2.10.1.6.2. BitBucket
2.10.1.7. Chrome app
2.10.1.7.1. Cloud 9
2.10.1.8. NOT FREE
2.10.1.9. Only 1 RSA key pair
2.10.1.10. Can not export the private key
2.10.1.10.1. you can not use PuTTy or WinSCP at the same time with Cloud 9
2.10.1.11. Can not import RSA key pair
2.10.1.12. HAS A TERMINAL WINDOW (console)
2.10.1.12.1. Big plus
2.10.2. Codenvy (former Exo IDE)
2.10.2.1. What it is according to Wikipedia?
2.10.2.1.1. No info on first search page
2.10.2.2. Public code repositories
2.10.2.2.1. GitHub
2.10.2.2.2. Google ???
2.10.2.3. Support for deployment (PaaS)
2.10.2.3.1. Amazon Web Services
2.10.2.3.2. appfog
2.10.2.3.3. CloudBees
2.10.2.3.4. CloudFoundry
2.10.2.3.5. Google App Engine
2.10.2.3.6. Heroku
2.10.2.3.7. OpenShift
2.10.2.4. OpenId
2.10.2.4.1. GitHub
2.10.2.4.2. Google
2.10.2.5. Mobile native Apps
2.10.2.5.1. iPad
2.10.2.5.2. iPhone
2.10.2.5.3. Android
2.10.2.6. Chrome app
2.10.2.6.1. Cloud IDE
2.10.2.7. Doesn't support
2.10.2.7.1. node.js
2.10.3. Installed on your own server
2.10.4. How to OSS
2.10.4.1. github
2.10.4.1.1. Exo IDE
2.10.4.1.2. Cloud9
2.10.4.2. bitbucket
2.11. Cloud free public repositories (hosting code)
2.11.1. http://sourceforge.net/
2.11.2. http://code.google.com/
2.11.3. https://github.com/
2.11.3.1. What is GitHub according to Wikipedia
2.11.3.2. Main Features
2.11.3.2.1. forking
2.11.3.2.2. pull request
2.11.3.2.3. merge
2.11.3.3. U can use it without web interface
2.11.3.4. It has
2.11.3.4.1. Issue Tracker
2.11.3.4.2. WiKi
2.11.4. https://bitbucket.org/
2.11.4.1. Issue Tracker
2.11.4.1.1. Jira
2.12. Summary
2.13. Q&A
2.14. Cloud Step By Step Tutorial
2.14.1. Exo IDE
2.14.1.1. Create New OSS project
2.14.1.1.1. 1. Create an account on GitHub
2.14.1.1.2. 2. (Optional) Create an account on Exo IDE
2.14.1.1.3. 3. Create an account on AppFog
2.14.1.1.4. 4. Login to Exo IDE
2.14.1.1.5. 5. Choose PaaS
2.14.1.1.6. 6. Create a Project
2.14.1.1.7. 7. (Optional) Delete the files and folders in the new project
2.14.1.1.8. 8. (Optional) Create your own files and folders sutable for the project
2.14.1.1.9. 9. Do "git init"
2.14.1.1.10. 10. Create a repository in GitHub
2.14.1.1.11. 11. Add Remote Repository (github)
2.14.1.1.12. 12. Generate RSA key pair in Exo IDE
2.14.1.1.13. 13. Add the generated public key to github
2.14.1.1.14. 14. In Exo IDE do "git add ."; "git commit -m "My first commit""; "git push <reponame> master"
2.14.1.2. Contribute to an OSS project
2.14.1.2.1. 1. Create an account on GitHub
2.14.1.2.2. 2. (Optional) Create an account on Exo IDE
2.14.1.2.3. 3. Create an account on AppFog
2.14.1.2.4. 4. Login to Exo IDE
2.14.1.2.5. 5. Choose PaaS
2.14.1.2.6. 6. Create a Project
2.14.1.2.7. 7. (Optional) Delete the files and folders in the new project
2.14.1.2.8. 8. (Optional) Create your own files and folders sutable for the project
2.14.1.2.9. 9. Do "git init"
2.14.1.2.10. 10. Add Remote Repository (github) To the project in Exo IDE
2.14.1.2.11. 11. Generate RSA key pair in Exo IDE
2.14.1.2.12. 12. Add the generated public key to github
2.14.1.2.13. 13. Fork the existing project
2.14.1.2.14. 14. In Exo IDE do "git pull"
2.15. git (DSCM, VCS)
2.15.1. git init
2.15.2. git clone ssh://[email protected]/gitprojects/fmi.git
2.15.3. git remote add zend ssh://[email protected]/gitprojects/fmi.git
2.15.4. git remote
2.15.5. git remote rm zend
2.15.6. git branch
2.15.6.1. git branch iss53
2.15.6.2. git checkout iss53
2.15.6.3. git commit -a -m 'added a new footer [issue 53]'
2.15.6.4. git branch -d hotfix
2.15.6.5. $ git branch -r
2.15.6.5.1. Remote tracking branches
2.15.7. git branch mybranch
2.15.8. git rm
2.15.9. git fetch zend
2.15.10. git merge zend/master
2.15.11. .gitignore
2.15.11.1. git rm --cached filename
2.15.12. git push origin master
2.15.13. git pull origin master
2.15.14. git checkout zend/mybranch
2.15.15. git add index.php (. to add all files)
2.15.16. git add <folder>/*
2.15.17. git commit -m "My first commit"
2.15.18. git --version
2.15.19. Zend help
2.15.19.1. git --version
2.15.19.2. git clone <git repo URL>
2.15.19.3. $ GIT_SSL_NO_VERIFY=1 git clone <git repo URL>
2.15.19.4. .git/configfile
2.15.19.4.1. [http] postBuffer = 524288000
2.15.19.5. $ git add index.php$ git commit -m "Adding index.php"
2.15.19.6. $ git push origin master
2.15.19.7. Pushing an Existing Git Project into Zend Developer Cloud Platform
2.15.19.7.1. $ git remote add zendcloud <git repository URL>
2.15.19.7.2. $ git pull zendcloud master
2.15.19.7.3. $ git push zendcloud master
2.15.20. Oficial Git tutorial
2.15.20.1. $ git config --global user.name "Your Name Comes Here"
2.15.20.2. $ git config --global user.email [email protected]
2.16. Cloud Design
2.16.1. wix.com
2.17. tools, frameworks, distribution systems (networks), repositories
2.17.1. PEAR
2.17.2. PECL
2.17.3. Pyrus
2.17.4. Composer
2.17.5. Phar extension
2.17.6. ZF2 Modules
2.17.7. github.com
2.18. Deployment to AppFog
2.18.1. .htaccess
2.18.1.1. root
2.18.1.2. public
2.18.1.3. all other folders
2.18.2. vendor
2.18.2.1. ZF2
2.18.2.1.1. library
2.18.3. .gitignore
2.18.3.1. public
2.18.3.2. vendor
2.18.3.3. first you have to remove the file
2.18.3.3.1. do git remove <filename>
2.18.3.4. After the removal the ignore will take place
2.18.3.5. Note: you can not ignore existing files without first deleting them
2.18.4. Services
2.18.4.1. MySQL
2.18.4.1.1. local.php
2.18.4.1.2. install phpMyAdmin
2.18.4.1.3. config.inc.php phpMyAdmin
2.18.4.1.4. Bind your PhpMyAdmin app to the MySQL service
2.18.4.1.5. PMA_PASSWORD
2.18.4.1.6. use your username for AppFog
2.18.4.1.7. configuration in the app
2.18.5. Custom DNS
2.18.5.1. Free public DNS
2.18.5.2. Free DNS hosting
2.18.5.3. Cloud DNS hosting
2.18.6. public/index.php
2.18.7. autoload file in vendor
2.19. phpunit
2.19.1. Installation
2.19.1.1. PEAR
2.19.1.2. Composer
2.19.1.3. PHP Archive (PHAR)
2.19.1.4. Optional packages
2.19.1.5. Upgrading
2.20. ZF2 cheatsheet
2.21. PSR-0 (Pretty Standard, Really)
2.22. Getting Started with composer packages
2.23. PHPspc
2.24. Behat
2.25. Red Mind
2.26. HTML5, CSS3, JS IDEs
2.27. Continuous integration
2.28. The best Server IDE combinations
2.28.1. Zend EclipsePDT
2.28.1.1. Zend Server (Zend Debugger)
2.28.1.2. EGit extra installed
2.28.1.2.1. SSH? trough PuTTY
2.28.2. NetBeans
2.28.2.1. XAMPP
2.28.2.1.1. Install Xdebug
2.28.2.2. WAMP
2.28.2.2.1. Comes with Xdebug
2.28.2.2.2. Why doesn't work
2.28.2.3. Comes with Git
2.28.2.3.1. SSH?
2.28.2.4. HoTo
2.28.2.4.1. 1 Download and install Git
2.28.2.4.2. 2* (Optional) Generate RSA key pairs
2.28.2.4.3. 3* (Optional) Enter the public key in your bitbucket account
2.28.2.4.4. 4 Download and install WAMP
2.28.2.4.5. 5 add to PATH
2.28.2.4.6. 6 Enable SSL in PHP manualy
2.28.2.4.7. 7 Install composer
2.28.2.4.8. 8 Virtual host
2.28.2.4.9. 9 NetBeans
2.28.3. Minimalistic approach
2.28.3.1. Git (MSYS)
2.28.3.1.1. ssh-keygen -t rsa
2.28.3.2. XAMPP, WAMP or Zend Server
2.28.3.2.1. Add to Path
2.28.3.2.2. vhost with ports
2.28.3.2.3. vhost subdomain
2.28.3.2.4. IMPORTANT NOTES for vhosts
2.28.3.3. composer
2.28.3.3.1. In the PATH
2.28.3.3.2. get composer.phar
2.28.3.3.3. create a bath file
2.28.3.3.4. IMPORTANT NOTES (espacialy for WAMP)
2.28.3.4. Notepad++
2.29. PPI framework Skeleton Application
2.30. ZfcUser, BjyAuthorize and Doctrine working together
2.30.1. How to start a project?
2.31. Naming coding standards
2.32. ZendTool
2.32.1. GitHub
2.32.2. separate from ZF2 project
2.32.3. Documentation
2.32.4. Installation
2.32.4.1. Composer
2.32.4.1.1. Move the folder to vendor
2.32.4.1.2. Sugested changes
2.32.4.2. Manual
2.32.4.3. Without installation, using the PHAR file
2.32.4.3.1. I have created zf.bat in the same folder as zftool.phar
2.32.5. Doesn't create composer.phar in the root of the project
3. 2. MVC. Model View Controller.
3.1. HTML5 kingdom
3.1.1. HTML5 the king and knight
3.1.1.1. HTML - HyperText Markup Language
3.1.1.1.1. History
3.1.1.2. History of HTML5
3.1.1.2.1. 2004
3.1.1.3. tags
3.1.1.3.1. block
3.1.1.3.2. inline
3.1.1.4. What is new in HTML5
3.1.1.4.1. The <canvas> element for 2D drawing
3.1.1.4.2. The <video> and <audio> elements for media playback
3.1.1.4.3. Support for local storage
3.1.1.4.4. New content-specific elements, like <article>, <footer>, <header>, <nav>, <section><aside>
3.1.1.4.5. New form controls, like calendar, date, time, email, url, search
3.1.1.5. Browser Support for HTML5
3.1.1.5.1. HTML5 is not yet an official standard
3.1.1.6. W3C
3.1.1.7. Structure
3.1.1.7.1. some structural HTML5 tags
3.1.1.7.2. drawing
3.1.2. CSS3 the tailor
3.1.2.1. structure and visualisation separation
3.1.2.2. W3C
3.1.2.3. Position
3.1.2.3.1. External
3.1.2.3.2. Internal
3.1.2.4. Selectors
3.1.2.5. Properties (style attributes )
3.1.2.6. Pseudo-classes
3.1.2.7. Pseudo elements
3.1.2.8. CSS for DHTML
3.1.2.8.1. position
3.1.2.8.2. top, left
3.1.2.8.3. bottom, right
3.1.2.8.4. width, height
3.1.2.8.5. z-index
3.1.2.8.6. display
3.1.2.8.7. visibility
3.1.2.8.8. clip
3.1.2.8.9. overflow
3.1.2.8.10. margin, border, padding
3.1.2.8.11. background
3.1.2.8.12. opacity
3.1.2.9. CSS Box model
3.1.2.9.1. Internet Explorer quirks
3.1.2.10. Our CSS
3.1.2.10.1. Main file
3.1.2.10.2. Layout
3.1.2.10.3. Typography
3.1.2.10.4. Navigation
3.1.3. JavaScript (ES5) the wizard
3.1.3.1. Embedding Scripts in HTML
3.1.3.1.1. The <script> Tag
3.1.3.1.2. Scripts in External Files
3.1.4. To conquer all natives
3.1.5. Progressive Enhancement vs Graceful Degradation
3.1.6. The all cool things in the web
3.1.7. ULITA
3.1.7.1. Usability
3.1.7.2. Localisation (l10n)
3.1.7.3. Internationalization (i18n)
3.1.7.4. Translation
3.1.7.5. Accessability
3.2. PHP
3.2.1. "Hello World"
3.2.2. ";" at the end of the statement
3.2.3. Variables start with "$"
3.2.3.1. a-z A-Z 0-9 _
3.2.3.2. don't start with number
3.2.4. Loosely Typed (dynamic)
3.2.4.1. vs
3.2.4.1.1. Stricktly Typed (Static)
3.2.5. Types
3.2.5.1. Numeric
3.2.5.1.1. float
3.2.5.1.2. int
3.2.5.2. Boolean
3.2.5.2.1. true
3.2.5.2.2. false
3.2.5.3. String
3.2.6. functions
3.2.7. PHP Arrays
3.2.8. PHP is from the syntax group of the C languages
3.2.9. Operators
3.2.10. Statements
3.2.10.1. if
3.2.10.2. if else
3.2.10.3. switch
3.2.11. while
3.2.12. do-while
3.2.13. for
3.2.14. $_GET
3.2.15. $_POST
3.2.16. constants
3.2.17. some magic constants
3.2.17.1. __FILE__
3.2.17.2. __DIR__
3.2.17.3. __METHOD__
3.2.17.4. __CLASS__
3.2.18. some predefined constants
3.2.18.1. DIRECTORY_SEPARATOR
3.2.18.2. PATH_SEPARATOR
3.2.19. some predefined superglobal variables
3.2.19.1. $_SERVER
3.2.19.2. $_COOKIE
3.2.19.2.1. setcookie('name', 'value', time() + 3600, "/", "", 0)
3.2.19.3. $_SESSION
3.2.19.3.1. session_start();
3.2.20. 1000+ functions
3.2.20.1. header($header, $replace, $response_code)
3.2.20.1.1. OSI Model
3.2.20.2. ob_start();
3.2.20.3. ob_flush()
3.3. OOP - Object Oriented Programing
3.3.1. Features
3.3.1.1. Inheritance
3.3.1.2. Abstraction
3.3.1.3. Encapsulation
3.3.1.4. Polymorphism
3.3.1.4.1. example
3.3.2. Goal
3.3.2.1. Reuse
3.3.2.2. Arhitecture (Structure)
3.3.2.2.1. The cost of change
3.3.2.2.2. vs hacking
3.3.2.3. 1 more level of abstraction
3.3.2.3.1. system programming usualy NO OOP
3.3.2.3.2. computer doesn care
3.3.2.4. Agile Software Development (Extreme programming)
3.3.2.4.1. Continuous Integration (OOP)
3.3.2.4.2. Refactoring
3.3.2.4.3. Test Driven Development
3.3.2.5. Everyone can write code which the computer can understand, but not everyone can write a code that othe human can understand.
3.3.2.6. Is it the Holy Grail? NO.
3.3.2.6.1. We continue searching and discovering
3.3.3. Topics
3.3.3.1. Class
3.3.3.1.1. Blueprint
3.3.3.1.2. Constructor
3.3.3.1.3. Destructor
3.3.3.1.4. Methods
3.3.3.1.5. Properties
3.3.3.2. Object
3.3.3.2.1. Instance of a class
3.3.3.3. Methods
3.3.3.3.1. Member functions
3.3.3.4. Access Parent Class
3.3.3.4.1. parent::
3.3.3.5. Properties
3.3.3.5.1. Member variables
3.3.3.6. Scope
3.3.3.6.1. private
3.3.3.6.2. protected
3.3.3.6.3. public
3.3.3.7. Constants
3.3.3.8. Static
3.3.3.8.1. Methods
3.3.3.8.2. Properties
3.3.3.8.3. for access ::
3.3.3.8.4. $this not available (use self)
3.3.3.8.5. has scope
3.3.3.9. Abstract Class
3.3.3.9.1. Must have at least 1 abstarct method
3.3.3.9.2. No direct instance
3.3.3.9.3. No abstract properties
3.3.3.10. Interface
3.3.3.10.1. Determen the methods
3.3.3.10.2. Can implement multiple interfaces
3.3.3.10.3. interface - keyword
3.3.3.10.4. implements - keyword
3.3.3.10.5. methods
3.3.3.10.6. makes sure there are well know methods comming from the interface
3.3.3.11. Overriding
3.3.3.12. Overloading
3.3.3.12.1. Magical methods
3.3.3.13. Autoload
3.3.3.13.1. Since PHP 5.2
3.3.3.13.2. spl_autoload_register()
3.3.3.14. Namespaces
3.3.3.14.1. Since PHP 5.3
3.3.3.14.2. basics
3.3.3.14.3. use
3.3.3.15. Anonymous (Lambda) functions and Closures
3.3.3.15.1. SInce 5.3
3.3.3.15.2. callback parameters
3.3.3.15.3. variable assignment
3.3.3.15.4. Closure
3.3.3.16. favor object composition over inheritance
3.3.3.16.1. inheritance
3.3.3.16.2. composition
3.3.3.16.3. Interfaces
3.3.4. Letter to a student (personal)
3.4. Design Patterns
3.4.1. Christopher Alexander
3.4.1.1. architect
3.4.1.2. first created the term
3.4.2. Gang Of Four - Design Patterns, Elements Of Reusable Object Oriented Software
3.4.2.1. Creational Patterns
3.4.2.1.1. Abstract Factory
3.4.2.1.2. Builder
3.4.2.1.3. Factory Method
3.4.2.1.4. Prototype
3.4.2.1.5. Singleton
3.4.2.2. Structural Patterns
3.4.2.2.1. Adapter
3.4.2.2.2. Bridge
3.4.2.2.3. Composite
3.4.2.2.4. Decorator
3.4.2.2.5. Façade
3.4.2.2.6. Flyweight
3.4.2.2.7. Proxy
3.4.2.3. Behavioral Patterns
3.4.2.3.1. Chain of Responsibility
3.4.2.3.2. Command
3.4.2.3.3. Interpreter
3.4.2.3.4. Iterator
3.4.2.3.5. Mediator
3.4.2.3.6. Memento
3.4.2.3.7. Observer
3.4.2.3.8. State
3.4.2.3.9. Strategy
3.4.2.3.10. Template Method
3.4.2.3.11. Visitor
3.4.3. Patterns of Enterprise Application Architecture
3.4.3.1. Domain Logic Patterns
3.4.3.1.1. Transaction Script
3.4.3.1.2. Domain Model
3.4.3.1.3. Table Module
3.4.3.1.4. Service Layer
3.4.3.2. Data Source Architectural Patterns
3.4.3.2.1. Table Data Gateway
3.4.3.2.2. Row Data Gateway
3.4.3.2.3. Active Record
3.4.3.2.4. Data Mapper
3.4.3.3. Object-Relational Behavioral Patterns
3.4.3.3.1. Unit of Work
3.4.3.3.2. Identity Map
3.4.3.3.3. Lazy Load
3.4.3.4. Object-Relational Structural Patterns
3.4.3.4.1. Identity Field
3.4.3.4.2. Foreign Key Mapping
3.4.3.4.3. Association Table Mapping
3.4.3.4.4. Dependent Mapping
3.4.3.4.5. Embedded Value
3.4.3.4.6. Serialized LOB
3.4.3.4.7. Single Table Inheritance
3.4.3.4.8. Class Table Inheritance
3.4.3.4.9. Concrete Table Inheritance
3.4.3.4.10. Inheritance Mappers
3.4.3.5. Object-Relational Metadata Mapping Patterns
3.4.3.5.1. Metadata Mapping
3.4.3.5.2. Query Object
3.4.3.5.3. Repository
3.4.3.6. Web Presentation Patterns
3.4.3.6.1. Model View Controller
3.4.3.6.2. Page Controller
3.4.3.6.3. Front Controller
3.4.3.6.4. Template View
3.4.3.6.5. Transform View
3.4.3.6.6. Two-Step View
3.4.3.6.7. Application Controller
3.4.3.7. Distribution Patterns
3.4.3.7.1. Remote Facade
3.4.3.7.2. Data Transfer Object
3.4.3.8. Offline Concurrency Patterns
3.4.3.8.1. Optimistic Offline Lock
3.4.3.8.2. Pessimistic Offline Lock
3.4.3.8.3. Coarse Grained Lock
3.4.3.8.4. Implicit Lock
3.4.3.9. Session State Patterns
3.4.3.9.1. Client Session State
3.4.3.9.2. Server Session State
3.4.3.9.3. Database Session State
3.4.3.10. Base Patterns
3.4.3.10.1. Gateway
3.4.3.10.2. Mapper
3.4.3.10.3. Layer Supertype
3.4.3.10.4. Separated Interface
3.4.3.10.5. Registry
3.4.3.10.6. Value Object
3.4.3.10.7. Money
3.4.3.10.8. Special Case
3.4.3.10.9. Plugin
3.4.3.10.10. Service Stub
3.4.3.10.11. Record Set
3.5. Zend Framework 2
3.5.1. Building a module
3.5.1.1. Modules
3.5.1.1.1. Setting up the Album module
3.5.1.1.2. Configuration
3.5.1.1.3. Informing the application about our new module
3.5.1.2. Routing and controllers
3.5.1.2.1. module.config.php
3.5.1.2.2. AlbumController.php
3.5.1.2.3. Initialise the view scripts
3.5.1.3. Database
3.5.1.3.1. connect
3.5.1.4. Models
3.5.1.4.1. model classes represent each entity in your application
3.5.1.4.2. ORM
3.5.1.5. Forms and actions
3.5.1.6. gitignore
3.6. We don't have to know the entire technology in details to start working
3.6.1. Know just enough to get the job done
3.6.2. Use google
4. 3. Forms.
4.1. ZF2 sources of knowledge
4.1.1. IRC: #zftalk (#zftalk.2 - closed) on Freenode Webchat
4.1.2. Maillist Subscribe Browse
4.1.2.1. Archive
4.1.3. Webinars
4.1.3.1. (old) Doctrine2 ZF1
4.1.4. Blogs
4.1.4.1. Enrico Zimuel
4.1.4.2. Matthew Weier O'Phinney
4.1.4.3. Rob Allen
4.1.4.4. Ralph Schindler
4.1.4.5. Ryan Mauger
4.1.4.5.1. github
4.1.4.6. Evan Coury
4.1.5. Official site
4.1.5.1. About
4.1.5.1.1. Overview
4.1.5.1.2. FAQ (ZF2 FAQ)
4.1.5.1.3. ZF1 FAQ
4.1.5.1.4. Security
4.1.5.1.5. Changelog
4.1.5.1.6. Blog
4.1.5.1.7. New BSD License
4.1.5.2. Learn
4.1.5.2.1. User Guide
4.1.5.2.2. Reference Guide
4.1.5.2.3. APIs
4.1.5.2.4. Training & Certification
4.1.5.2.5. Support & Consulting
4.1.5.3. Get Started
4.1.5.3.1. Downloads
4.1.5.3.2. Get the Skeleton App
4.1.5.3.3. Try on phpcloud.com
4.1.5.4. Participate
4.1.5.4.1. Overview
4.1.5.4.2. Contributors Guide
4.1.5.4.3. Blogs
4.1.5.5. Contact Us
4.1.5.5.1. form
4.1.5.5.2. mailing lists
4.1.5.5.3. IRC
4.1.6. Zend Framework 2
4.1.6.1. Getting started
4.1.6.2. Reference Guide
4.1.6.3. API
4.1.7. skeleton app
4.1.7.1. Welcome to Zend Framework 2
4.1.7.1.1. ZF2 Skeleton Application
4.1.7.1.2. Fork Zend Framework 2 on GitHub »
4.1.7.2. Follow Development
4.1.7.2.1. wiki
4.1.7.2.2. dev blog
4.1.7.2.3. issue tracker
4.1.7.2.4. ZF2 Development Portal
4.1.7.3. Discover Modules
4.1.7.3.1. on GitHub
4.1.7.3.2. Explore ZF2 Modules »(web site)
4.1.7.4. Help & Support
4.1.7.4.1. IRC: #zftalk (#zftalk.2 - closed) on Freenode
4.1.7.4.2. mailing lists
4.1.7.4.3. Ping us on IRC »
4.1.8. How to use ZF1 libraries in ZF2
4.1.9. Wiki
4.1.10. ? Thorsten Ruf's ZF1(zenddispatch_en.pdf) ?
4.1.11. ? Dispatch ZF2 Diagram ?
4.1.12. ? Front Controller Dispatch Process ?
4.1.13. Dispatch ZF2
4.1.13.1. correct link
4.1.14. Zend Framework 2 Cheat Sheet
4.1.15. ZF2 Patterns
4.1.16. Event Manager
4.1.16.1. Triggered: Zend Framework 2's EventManager
4.1.16.1.1. Event
4.1.16.1.2. Listener
4.1.16.1.3. Event Manager (Connection Bus)
4.1.16.1.4. Priority
4.1.16.1.5. how to trigger
4.1.16.1.6. Listeners
4.1.16.1.7. Custom Events
4.1.16.1.8. Recomendations
4.1.16.1.9. Global Events
4.1.16.1.10. some terms
4.1.16.1.11. other terms
4.1.17. Service Manager ("object manager” or “instance manager”)
4.1.18. The MVC architecture of ZF2
4.1.18.1. New architecture
4.1.18.1.1. MVC
4.1.18.1.2. Di
4.1.18.1.3. Events
4.1.18.1.4. Service
4.1.18.1.5. Module
4.1.18.2. PSR-2 compliant
4.1.18.3. packaging system
4.1.18.3.1. pyrus
4.1.18.3.2. composer
4.1.18.4. PHP 5.3.5
4.1.18.5. Performace Improvments
4.1.18.5.1. Lazy Loading
4.1.18.6. The new core
4.1.18.6.1. ZF1
4.1.18.6.2. ZF2
4.1.18.7. MVC
4.1.18.7.1. separation of concern
4.1.18.7.2. code reusability
4.1.18.8. Event Driven Architecture
4.1.18.8.1. bootstrap
4.1.18.8.2. root
4.1.18.8.3. dispatch
4.1.18.9. A common workflow
4.1.18.10. Default services
4.1.18.10.1. EventManager
4.1.18.10.2. ModuleManager
4.1.18.10.3. Request
4.1.18.10.4. Response
4.1.18.10.5. RouteListener
4.1.18.10.6. Router
4.1.18.10.7. DispatchListener
4.1.18.10.8. VIewManager
4.1.18.11. config/application.config.php
4.1.18.12. public/.htaccess
4.1.18.12.1. The front controller is index.php
4.1.18.13. public/index.php
4.1.18.14. Zend\ServiceManager
4.1.18.14.1. IS A SL (SERVICE LOCATOR) implementation
4.1.18.14.2. Well known object in which you can register object and retrieve them later
4.1.18.14.3. Driven by configuration
4.1.18.15. Type of services
4.1.18.15.1. Explicit
4.1.18.15.2. Invocables
4.1.18.15.3. Factories
4.1.18.15.4. Aliases
4.1.18.15.5. Abstract Factories
4.1.18.15.6. Scoped Containers
4.1.18.15.7. Shared
4.1.18.16. modules
4.1.18.16.1. inform the MVC about services and event listeners
4.1.18.16.2. subset of the app
4.1.18.16.3. Modules are simply
4.1.18.17. module/Application/Module.php
4.1.18.18. module/Application/config/module.config.php
4.1.18.19. module/Application/src/Application/Controller/IndexController.php
4.1.19. ZF 2, Doctrine 2
4.1.19.1. Using Doctrine 2 in Zend Framework 2
4.1.19.2. Install Doctrine 2 on ZF2
4.1.19.3. Doctrine 2 ORM Module for Zend Framework 2 github
4.1.19.4. Tutorial
4.1.19.4.1. github
4.1.20. Composer - Dependency Management for PHP
4.1.20.1. on github
4.1.20.2. Official site
4.1.20.3. Install
4.1.20.3.1. in php folder
4.1.20.3.2. Make sure SSL enabled in PHP
4.1.20.3.3. Make it global
4.1.20.4. use
4.1.20.4.1. Create Project
4.1.20.4.2. Require Module
4.1.20.4.3. Manualy Add a Module
4.1.20.4.4. Don't forget to add the modules to application.config.php
4.1.20.5. Autoloading
4.1.20.5.1. require 'vendor/autoload.php';
4.1.21. Do we need framework at all???
4.1.22. Some tips to write better Zend Framework 2 modules
4.1.22.1. On Russian
4.2. ZF2 Di Theory
4.2.1. Strong Cohesion
4.2.2. Loosly Coupling
4.2.2.1. Decoupling
4.2.3. Reuse
4.2.4. Cost of change
4.2.5. IoC Inversion of Control ( concep)
4.2.5.1. Inversion of Control Containers and the Dependency Injection pattern
4.2.5.1.1. Components and Services
4.2.5.1.2. A Naive Example
4.2.5.1.3. Inversion of Control
4.2.5.1.4. Forms of Dependency Injection
4.2.5.1.5. Using a Service Locator
4.2.5.1.6. Deciding which option to use
4.2.5.1.7. Some further issues
4.2.5.1.8. Concluding Thoughts
4.2.6. Di - Dependancy Injection (pattern)
4.2.6.1. Constructor Injection
4.2.6.1.1. pros
4.2.6.1.2. cons
4.2.6.2. Setter Injection
4.2.6.2.1. pros
4.2.6.2.2. cons
4.2.6.3. Interface injection
4.2.6.3.1. "Aware"
4.2.6.3.2. cons
4.2.6.4. DiC Dependancy Injection Container (tool)
4.2.6.5. sources of information
4.2.6.5.1. webinar
4.2.6.5.2. Documentation on the site
4.2.7. Service Locator (pattern)
4.2.7.1. alternative for Di
4.2.7.2. (SL) Service Locator !== (DiC) Dependancy injection Container
4.2.7.3. But DiC == SL
4.2.7.4. DiC can be the foundation of consumed for Service Location
4.2.7.5. cons
4.2.7.5.1. SL becomes a dependancy
4.2.7.6. pros
4.2.7.6.1. Not all code paths may use all dependancies
4.2.8. Zend\Di (DiC implementation)
4.2.8.1. Not a requirment
4.2.8.2. supprot for both
4.2.8.2.1. constructor
4.2.8.2.2. setter
4.2.8.3. Not "Hello World" friendly
4.2.9. Type-hints
4.3. Event Manager
4.3.1. Space Station Modules
4.3.1.1. Consists by independent modules
4.3.1.2. Each module is build from a group of smaller modules following the same pattern
4.3.1.2.1. The smaller modules are build from even smaller modules following the same pattern etc.
4.3.1.3. Each module can trigger an event
4.3.1.3.1. There are maybe modules listening on this channel (for this event) or maybe not
4.3.1.4. Each module can listen for an event
4.3.1.4.1. But there are maybe modules emitting (notifying) about this event or not
4.3.2. Electronic Circuits
4.3.2.1. Phisical Interfaces
4.3.2.1.1. Logical
4.3.2.1.2. Electrical
4.3.2.1.3. Phisical
4.3.3. Event
4.3.3.1. Something that does happen
4.3.4. Listener
4.3.4.1. a callback
4.3.4.1.1. responds to an event
4.3.5. Event Manager (how to attach a callback listener)
4.3.5.1. a collection of Listeners
4.3.5.2. triggers events
4.3.6. Priority
4.3.7. how to trigger
4.3.8. Listeners
4.3.8.1. Receive a single argument ($e)
4.3.8.2. Any PHP callback
4.3.9. Recomendations
4.3.9.1. __FUNCTION__
4.3.9.1.1. dot-notation
4.3.9.2. pass all input as a parameter
4.3.10. Global Events
4.3.11. some terms
4.3.11.1. trigger/attach
4.3.11.2. public/subscribe
4.3.11.3. notify/listen
4.3.12. more terms
4.3.12.1. target
4.3.12.2. context
4.3.13. associations
4.3.13.1. Environment for signals
4.3.13.2. Wires
4.3.13.3. Channels
4.3.13.4. Connection
4.3.13.5. Bus
4.4. MVC architecutre of ZF2
4.5. Bridge the domain Model and the View layer
4.5.1. spread across
4.5.1.1. Controller
4.5.1.2. Model
4.5.1.3. View
4.5.1.3.1. list = index
4.5.2. Terms
4.5.2.1. Hydration
4.5.2.2. Extraction
4.5.3. The bond
4.5.3.1. $form->bind($model)
4.5.3.1.1. Make the bond
4.5.3.1.2. Fly in the Clouds
4.5.4. Zend Hydrators
4.5.4.1. Zend\Stdlib\Hydrator\ArraySerializable
4.5.4.2. Zend\Stdlib\Hydrator\ClassMethods
4.5.4.3. Zend\Stdlib\Hydrator\ObjectProperty
4.5.4.4. HydratorInterface
4.5.5. Look the "Album" tutorial app
4.5.6. Annotation to unite
5. 4. ORM. Object Relational Mapping.
5.1. Doctrine 2
5.1.1. What is Doctrine?
5.1.2. What are Entities?
5.1.3. Doctrine 2 Working with Associations
5.1.4. Doctrine configuration
5.1.5. EntityManager
5.1.5.1. The main access point
5.1.6. Association Mapping
5.1.7. Getting Started
5.1.8. new Zend Form features explained
5.1.9. Hydrator
5.1.10. Notes on Doctrine 2
5.1.11. Doctrine ZF2 Lection
5.2. Propel
5.3. No SQL DB (ODM)
5.3.1. Mongo DB
5.3.2. Apache CouchDB
5.4. "What is first, the chicken or the egg?"
5.4.1. First build the DB as a result of the system analysis?
5.4.1.1. If you don't know the programming language framework etc.
5.4.1.2. The ORM has tools for reverse engineering to build the classes from DB.
5.4.2. First build the domain logic with classes (Entities) as a result of the system analysis.
5.4.2.1. If you are going to use OOP
5.4.2.2. If you are planning to use ORM
5.4.2.3. If the ORM has tools for creating DB
5.4.2.4. Always keep in mind the DB at the back of your head
5.4.2.5. If you don't know what DB are you going to use'
5.5. Composer is used for the modules (Doctrine)
5.5.1. Cloud 9 offers console but you have to pay
5.5.2. I would prefer to install development stack on a local machine
5.5.2.1. XAMPP
5.5.2.2. Composer
5.6. Local workflow
5.6.1. Start Notepad++
5.6.2. Start Git console
5.6.2.1. Clone the repo
5.6.3. /xampp/apache_start.bat
5.6.4. /xampp/mysql_start.bat
5.6.5. Console for MySQL
5.6.6. Start MongoDB
5.6.6.1. shutdown
5.6.6.2. The 32 bit version works on 46 bit WIn7
5.6.6.3. 64 bit allocates about 4GB disk space
5.6.7. Console for MongoDB
5.6.7.1. show databases = show dbs
5.6.7.2. tables = collections
5.6.7.3. rows = documents
5.6.8. Console for composer
5.6.8.1. Install ONLY ZF2
5.6.8.2. Install ONLY DoctrineORM
5.6.8.3. Install ONLY DoctrineODM
5.6.8.4. Install the Doctrine modules for ZF2
5.6.8.4.1. DoctrineModule
5.6.8.4.2. DoctrineORM Module
5.6.8.4.3. DoctrineODM Module
5.6.9. Allow the modules and setup the connections etc.
5.6.9.1. DoctrineORM
5.6.9.1.1. add DoctrineModule and DoctrineORMModule to your config/application.config.php
5.6.9.1.2. create directory data/DoctrineORMModule/Proxy
5.6.9.1.3. module.config.php or application.config.php
5.6.9.1.4. /config/autoload/
5.6.9.1.5. Registered Service names
5.6.9.1.6. Command Line
5.6.9.1.7. Service Locator
5.6.9.2. DoctrineODM
5.6.9.2.1. my/project/directory/configs/application.config.php
5.6.9.2.2. config/autoload/module.doctrine-mongo-odm.local.php
5.6.9.2.3. create directory my/project/directory/data/DoctrineMongoODMModule/Proxy
5.6.9.2.4. create directory my/project/directory/data/DoctrineMongoODMModule/Hydrator
5.6.9.2.5. Command Line
5.6.9.2.6. Service Locator
5.6.10. Console for Doctrine
5.6.10.1. DoctrineORM
5.6.10.1.1. Commands
5.6.10.1.2. you need cli-config.php and bootstrap
5.6.10.2. DoctrineODM
5.6.10.2.1. Commands
5.6.10.2.2. you need cli-config.php and bootstrap
5.6.10.3. Reverse engineer the DB
5.6.10.3.1. Create the bootstrap.php in your project
5.6.10.3.2. Create cli-config.php
5.6.10.3.3. Create the mappings
5.6.10.3.4. Generate the entities
5.6.10.4. CLI with the ZF2 module doctrine-module.bat
5.6.10.4.1. no bootsrap and cli-config neccessary
5.6.10.4.2. update
5.6.10.4.3. create
5.6.10.4.4. drop and create
5.6.11. Apache Virtual Host
5.6.11.1. .htaccess
5.6.11.2. local.php
5.6.12. links to the projects and initial installation
5.6.12.1. xampp
5.6.12.2. MongoDB
5.6.12.2.1. Download
5.6.12.2.2. Install and run on Windows
5.6.12.3. Composer
5.6.12.3.1. Getting Started
5.6.12.3.2. https://packagist.org/
5.6.12.3.3. Install
5.6.12.4. Doctrine
5.6.12.5. Zend Framework doc
5.6.12.6. Zend Modules
5.6.12.6.1. DoctrineModule on github
5.6.12.6.2. DoctrineORMModule on github
5.6.12.6.3. DoctrineMongoODMModule on github
5.6.12.7. php_intl
5.6.12.8. tinyMCE
5.6.12.8.1. in the view script
5.6.12.9. jQuery Date/Time Entry
5.6.12.9.1. in the view script
5.6.12.10. Dojo, dijit/form/DateTextBox
5.6.12.10.1. Themes and Theming
5.6.12.10.2. dijit/form/DateTextBox
5.6.12.10.3. in the view script
5.6.13. Module.php
5.6.13.1. module.config.php
6. 5. CRUD. Create Retrieve Update Delete.
6.1. WInSCP (native app) for the sensitive files
6.2. With Cloud 9
6.2.1. Only Git
6.2.2. No access to the private key
6.2.2.1. No other app can be used
6.2.3. Only Git tricks but very complex
6.2.3.1. Branch public
6.2.3.2. Branch production
6.2.3.3. Merging is a problem???
6.3. Codenvy
6.3.1. The solution for the sensitive files
6.4. Creating new Controller in Application
6.4.1. branch
6.4.1.1. exp1
6.4.2. module.config.php
6.4.2.1. controllers
6.4.2.1.1. invokables
6.4.3. view
6.4.3.1. optional
6.5. Set MIME type
6.5.1. application/php
6.6. branches
6.6.1. will be more dificult
6.7. approaches
6.7.1. MySQL, Oracle, PostgreSQL etc. API
6.7.1.1. MySQL Functions
6.7.1.1.1. mysql_connect
6.7.2. DBAL: ADOdb, PDO
6.7.2.1. PDO
6.7.2.1.1. PDO Drivers
6.7.2.2. ADOdb
6.7.2.3. ADOdb Active Record
6.7.2.3.1. Dealing with Collections
6.7.2.4. Zend\Db\Adapter
6.7.2.4.1. thin wrapper
6.7.2.5. Doctrine DBAL
6.7.2.5.1. thin wrapper of PDO
6.7.3. Zend\Adapter, Doctrine DBAL
6.7.3.1. Doctrine
6.7.3.1.1. DBAL over PDO
6.7.3.2. Zend
6.7.3.2.1. DBAL Zend\Db\Adapter
6.7.4. ORM technics
6.7.4.1. Design Patterns
6.7.4.1.1. Table Data Gateway
6.7.4.1.2. Row data Gateway
6.7.4.1.3. Active Record
6.7.4.1.4. Data Mapper
6.8. The progress in ZF2
6.8.1. Simple CRUD (with Table Data Gateway)
6.8.1.1. The Result Set is a Collection of Objects (each row is an object)
6.8.1.1.1. The ResultSet is Zend\Db\ResultSet\ResultSet. Each row is an Zend\Db\RowGateway\RowGateway Object. Zend\Db\TableGateway\Feature\RowGatewayFeature('usr_id') in Table Data Gateway
6.8.1.1.2. Return Custom Object
6.8.1.2. The Result Set is an Zend\Db\ResultSet\ResultSet of ArrayObjects. Each row is an ArrayObject.
6.8.1.2.1. The ArrayObject can be used as an ordinar array or as an object. $row->usr_id and $row['usr_id'] will both work
6.8.2. Integrate Doctrine wirh ZF2
6.8.2.1. Doctrine DBAL
6.8.2.1.1. almost the same like Zend\Db\Adapter\Adapter
6.8.2.2. Doctrine Entity Manager
6.8.2.2.1. With separate classes and files for forms and DQL in the controller
6.8.2.2.2. We use annotations to generate forms from the entities
6.8.2.2.3. Entity Repositories
6.9. The evolution
6.9.1. 1) native DB API
6.9.1.1. set of functions and variables
6.9.1.2. difficult to replace
6.9.2. 2) Abstract the connection to DB
6.9.2.1. DBAL OOP. We delegate the communication to DB to an object.
6.9.2.2. We create the SQL statments
6.9.2.3. Easy to replace the RDBMS by changing the Object
6.9.2.4. But we still deal with concrete tables (we have to know the names structure etc.) and we use SQL statements
6.9.3. 3) Abstract the tables - objects in the DB
6.9.3.1. We can change the tables and still be OK
6.9.3.2. Table Data Gateway
6.9.3.3. but we deal with functions which have nothing to do with our domain logic CRUD and we have to know the structure of the DB
6.9.4. 4) Abstract the rows (they better represent the real entities of our domain logic)
6.9.4.1. Row Data Gateway
6.9.5. 5) Add domain logic to these Row Objects. Think for them as Domain Objects. (e.g. teach Object User to tell his name. add tellYourName(), greating etc..)
6.9.5.1. We still think about technical details. We are not detached from the concrete implementation. We have our domain logic in the DB not in the App
6.9.6. 6) Forget about the DB. Create a Domain model using Entities. Network of objects - virtual DB. Data mapper. Persisting is not a concern for this object. We don't need methods like save delete etc. We have ONLY the properties-characteristics of the object and accessory methods to set and get values to the properties.
6.9.6.1. In the perfect world we will not need to save anything to persistent layer. The SSD drives will become the RAM of the computer. Even if you turn your computer off the running programs will keep their state until we decide to exit or close the program.
6.9.7. 7) Forget about the DB. Use a dedicated Data Mapper like Doctrine.
6.9.7.1. We don't have to create the datamapper objects anymore for each table
6.9.7.2. There is Doctirne Entity Manager (ORM) or Doctrine DOcumentManager (ODM)
6.9.7.3. Network of objects. Virtual Data Base
7. 6. JS basics.
7.1. sources of knowledge
7.1.1. David Flanagan
7.1.1.1. JavaScript: The Definitive Guide, Sixth Edition
7.1.1.2. jQuery Pocket Reference
7.1.1.3. JavaScript Pocket Reference, 3rd Edition
7.1.2. Douglas Crockford
7.1.2.1. JavaScript: The Good Parts
7.1.3. Nicholas C. Zakas
7.1.3.1. High Performance JavaScript (Build Faster Web Application Interfaces)
7.1.4. Stoyan Stefanov
7.1.4.1. JavaScript Patterns
7.1.4.2. Object-Oriented Java Script
7.1.5. John Resig
7.1.5.1. Manning: Secrets of the JavaScript Ninja
7.1.6. YUI Theater
7.2. Development
7.2.1. Prototype based
7.2.2. Classless
7.2.3. Object Oriented
7.2.4. Event Driven
7.3. Getting Started Example
7.4. Functions
7.4.1. First Class Objects
7.4.1.1. A function is an instance of the Object type
7.4.1.2. A function can have properties and has a link back to its constructor method
7.4.1.3. You can store the function in a variable
7.4.1.4. You can pass the function as a parameter to another function
7.4.1.5. You can return the function from a function
7.4.2. Example
7.4.3. definitions
7.4.3.1. functions defined with the function statement
7.4.3.2. function defined with literal expression
7.4.3.3. With the Function class
7.4.4. Universal Properties and Methods
7.4.4.1. Properties
7.4.4.1.1. myNewClass.length
7.4.4.1.2. myNewClass.prototype
7.4.4.2. Methods
7.4.4.2.1. myNewClass.call({}, 'arg1', 'arg2')
7.4.4.2.2. myNewClass.apply({}, ['arg1', 'arg2'])
7.4.5. Operators
7.4.5.1. typeof myNewClass
7.4.5.2. myNewClass instanceof Function
7.4.6. Functions methods and Constructors
7.4.6.1. Function Constructor
7.4.6.2. Method
7.5. Objects
7.5.1. Create
7.5.1.1. Created as Literal (Expression)
7.5.1.2. Created with one of the Standart Classes "Object Class"
7.5.1.3. Created with a Constructor that defines a Class of Objects (custom class)
7.5.2. Universal Properties and Methods
7.5.2.1. Properties
7.5.2.1.1. myObject.constructor
7.5.2.2. Methods
7.5.2.2.1. myObject.toString()
7.5.2.2.2. myObject.toLocaleString()
7.5.2.2.3. myObject.valueOf()
7.5.2.2.4. myObject.hasOwnProperty('prop1')
7.5.2.2.5. myObject.propertyIsEnumerable('prop1')
7.5.2.2.6. Object.isPrototypeOf( myObject )
7.5.3. Operators
7.5.3.1. typeof myObject
7.5.3.2. myObject instanceof myClass
7.6. Programming Styles
7.6.1. Pseudoclasical technique
7.6.2. Prototypal style
7.6.3. Functional style
7.7. Ajax
7.7.1. DOM Manipulation
7.7.2. Remouting
7.7.3. Dynamic Behaviour
7.8. Example 1
7.9. Example 2
7.10. Architecture of an app
7.10.1. Leave if you don't like it
7.10.1.1. I am always amazed by the negative people!
7.10.1.1.1. You have to appreciate at least the effort.
7.10.1.1.2. If you have to say something bad, better don't say anything
7.10.1.2. Create somethign yourself.
7.10.1.3. Don't criticise!
7.10.1.4. We live in a free country! Everybody can be stupid and ugly as he likes! :)
7.10.1.5. I have a great lifestyle!
7.10.1.5.1. I am happy and thankful!
7.10.1.6. The fact that somebody doesn't like the ideas, is not going to change the fact I enjoy my life and what I am doing!
7.10.1.7. These are my original ideas
7.10.1.8. Maybe somebody else came up with the same ideas
7.10.1.8.1. Usually the same ideas come to more than one person when the moment is suitable!
7.10.1.8.2. The truth and the ideas are always there the people are just the conductors.
7.10.1.9. I am not asking anybody to watch the videos. If I am the only viewer I still will be happy. they will help me to recall some ideas and concepts.
7.10.1.10. I am doing these videos for myself, my friends, colleagues and students.
7.10.2. lets mimic the real objects and systems
7.10.2.1. Nature
7.10.2.1.1. Plant
7.10.2.1.2. Human
7.10.2.2. Technical systems (They to mimic the nature)
7.10.2.2.1. Space Station
7.10.2.2.2. Motherboard
7.10.3. Everythign starts from a seed
7.10.3.1. Config is the DNA
7.10.3.2. Events (communication object) gives the senses
7.10.3.3. Bootstrap is not really a paradox
7.10.3.3.1. What is first? The chick or the egg?
7.10.3.3.2. What is first the program or the computer? Is it a real paradox?
7.10.3.3.3. it would be a paradox if it is like Baron von Munchausen pulling himself from the swamp.
7.10.3.3.4. Bootstrapping
7.10.3.4. Wizard
7.10.3.4.1. In the case of JS the wizard is (HTML tag)
7.10.3.4.2. The MVC comes later
8. 7. JS toolkits. (frameworks???)
8.1. Dojo Toolkit
8.1.1. Dijit
8.1.2. Getting Dojo
8.1.2.1. Downloading an official release
8.1.2.2. Downloading from Subversion
8.1.2.3. CDN
8.1.3. Hello Dojo
8.1.4. Modern Dojo
8.1.4.1. Migration Guide
8.1.4.2. require()
8.1.4.3. define()
8.1.4.4. module IDs (MID)
8.1.4.5. AMD
8.1.5. Dojo Base and Core
8.1.6. Events and Advice
8.1.7. DOM Manipulation
8.1.8. Waiting for the DOM
8.1.9. Adding Visual Effects
8.1.10. Dijit and Widgets
8.1.11. XHR
8.1.12. Ajax
8.2. jQuery
8.2.1. fluent interfaces and method chaining
8.2.2. An expressive syntax (CSS selectors) for referring to elements in the document
8.2.3. intro
8.2.4. jQuery Basics
8.2.4.1. The jQuery library defines a single global function named jQuery().
8.2.4.1.1. $ as a shortcut for it
8.2.4.2. This single global function with two names is the central query function for jQuery.
8.2.4.3. var divs = $("div");
8.2.4.4. jQuery() is a factory function rather than a constructor: it returns a newly created object but is not used with the new keyword.
8.2.4.5. $("p.details").css("background-color", "yellow").show("fast");
8.2.4.6. method chaining idiom is common in jQuery programming
8.2.4.7. $(".clicktohide").click(function() { $(this).slideUp("slow"); });
8.2.4.8. Obtaining jQuery
8.2.4.8.1. download
8.2.4.8.2. CDN (Content Distribution Network)
8.2.4.9. The jQuery() Function
8.2.4.9.1. $() is to pass a CSS selector
8.2.4.9.2. The second way to invoke $() is to pass it an Element or Document or Window object
8.2.4.9.3. The third way to invoke $() is to pass it a string of HTML text
8.2.4.9.4. Finally, the fourth way to invoke $() is to pass a function to it. If you do this, the function you pass will be invoked when the document has been loaded and the DOM is ready to be manipulated.
8.2.4.9.5. The jQuery library also uses the jQuery() function as its namespace
8.2.4.10. Queries and Query Results
8.2.4.10.1. $("body").length
8.2.5. jQuery Getters and Setters
8.2.5.1. Getting and Setting HTML Attributes
8.2.5.1.1. $("#icon").attr("src", "icon.gif"); set
8.2.5.1.2. $("form").attr("action"); get
8.2.5.2. Getting and Setting CSS Attributes
8.2.5.2.1. $("h1").css("font-weight"); get
8.2.5.2.2. $("div.note").css("border", compound styles "solid black 2px"); set
8.2.5.3. Getting and Setting CSS Classes
8.2.5.3.1. $("h1").addClass("hilite");
8.2.5.4. Getting and Setting HTML Form Values
8.2.5.4.1. $("#surname").val(); get
8.2.5.4.2. $("#email").val("Invalid email address"); set
8.2.5.5. Getting and Setting Element Content
8.2.5.5.1. text()
8.2.5.5.2. html()
8.2.5.6. Getting and Setting Element Geometry
8.2.5.7. Getting and Setting Element Data
8.2.6. Altering Document Structure
8.2.6.1. Inserting and Replacing Elements
8.2.6.2. Copying Elements
8.2.6.3. Wrapping Elements
8.2.6.4. Deleting Elements
8.2.6.4.1. empty() removes all children (including text nodes)
8.2.6.4.2. The remove() method removes any event handlers
8.2.6.4.3. the unwrap()
8.2.7. Handling Events with jQuery
8.2.7.1. Simple Event Handler Registration
8.2.7.2. jQuery Event Handlers
8.2.7.3. The jQuery Event Object
8.2.7.4. Advanced Event Handler Registration
8.2.7.4.1. $('p').bind('click', f);
8.2.7.5. Deregistering Event Handlers
8.2.7.5.1. unbind()
8.2.7.6. Triggering Events
8.2.7.7. Custom Events
8.2.7.8. Live Events
8.2.8. Animated Effects
8.2.8.1. Simple Effects
8.2.8.1.1. fadeIn(), fadeOut(), fadeTo()
8.2.8.1.2. show(), hide(), toggle()
8.2.8.1.3. slideDown(), slideUp(), slideToggle()
8.2.8.2. Custom Animations
8.2.9. Ajax with jQuery
8.2.9.1. The load() Method
8.2.10. Utility Functions
8.2.11. Extending jQuery with Plug-ins
8.2.12. The jQuery UI Library
8.2.12.1. widgets
8.3. Prototype
8.3.1. http://script.aculo.us/
8.4. MooTools
8.5. YUI Library
8.6. Ext JS
8.6.1. Very Good Documentation
8.6.2. JSDuck
8.7. Backbone.js
8.8. Server Side JS
8.8.1. node.js
8.9. AngularJS by Google
8.9.1. on GitHub
8.9.2. MVW
8.10. Twitter Bootstrap
8.10.1. on GitHub
9. 8. JS Zend integration.
9.1. Create a Layout
9.1.1. get the links right
9.1.2. add to your module
9.1.3. Use the View Helpers
9.1.3.1. Doctype Helper
9.1.3.2. HeadLink Helper
9.1.3.3. HeadMeta Helper
9.1.3.4. HeadScript Helper
9.1.3.5. HeadStyle Helper
9.1.3.6. HeadTitle Helper
9.1.3.7. HTML Object Helpers
9.1.3.8. InlineScript Helper
9.1.4. Use existing template example
9.1.5. layout.phtml
9.1.6. change.phtml
9.2. Switch the Layout in the actions
9.3. Load different JS files in the view
9.4. Use widgets (Dojo, jQuery)
9.4.1. jQuery
9.4.2. Dijit
9.4.2.1. js file
9.5. View Helper Json
9.6. Disabling the layout in Zend Framework 2
9.7. Disable the layout and the view
9.8. Communicate to the server (ZF2)
9.8.1. Only with JS
9.8.2. jQuery way
9.8.3. Dojo way
9.8.3.1. example
9.9. Deploy to AppFog
9.10. Change the layout for a module
9.11. index.php
10. 9. Ajax.
10.1. Pure JS
10.1.1. Controller Action
10.1.2. View Script
10.1.2.1. js-ajax.phtml
10.1.3. JS File
10.1.3.1. jsAjax.js
10.2. jQuery
10.2.1. Controller Action
10.2.2. View Script
10.2.2.1. jquery-ajax.phtml
10.2.3. JS File
10.2.3.1. jqueryAjax.js
10.3. Dojo
10.3.1. Controller Action
10.3.2. View Script
10.3.2.1. dojo-ajax.phtml
10.3.3. JS File
10.4. Service
10.4.1. View Script
10.4.2. Controller Action
10.5. JSON service
10.5.1. Controller Action
10.5.2. View Script
11. 10. HTML5 CSS3.
12. Introduction
12.1. What is a Web Development?
12.2. What is the Web Application Development?
12.3. Web Application
12.4. Software engineering
12.4.1. Could be the best job in the world!
12.4.1.1. No boss!
12.4.1.2. No working hours.
12.4.1.3. Full creative freedom!
12.4.1.4. You are an ... alien!
12.4.2. Why some people think "The cariera in IT sucks?"
12.4.2.1. Average salary in the developed countries.
12.4.2.2. A lot of work
12.4.2.3. Age and sex discrimination
12.4.2.4. There are no "old experience professionals"
12.4.2.4.1. Everyone is a newbie
12.4.2.4.2. How can be "old experience professional" in a 6 months old technology
12.4.2.5. You never stop reading and learning
12.4.3. We have to raise the prestige of the profession.
12.4.4. Nothing can stop you if you have a real passion and fun!
12.5. The topics
12.5.1. Server-side (The first 5 Fridays)
12.5.1.1. PHP
12.5.1.1.1. ZF
12.5.2. Client-side (The last 5 Firdays)
12.5.2.1. Front End Engineering
12.5.2.1.1. JavaScript
12.5.2.1.2. HTML
12.5.2.1.3. CSS
12.6. I hold the rights to change the topics as we go.
12.7. Used tools
12.7.1. Web Based, Cloud
12.7.2. The students can change the devices
13. Lessons Learned
13.1. MInd Maps
13.1.1. MindMesiter
13.1.1.1. Presentations
13.1.1.1.1. Difficult
13.1.1.1.2. Do I realy need them
13.1.1.1.3. Tip
13.1.1.2. Upload Images
13.1.1.3. The Android App
13.1.1.4. Not free
13.1.1.5. Notes are very small
13.1.1.5.1. Try bigger font size
13.1.1.6. Use zoom In Out
13.1.1.7. Right Click to collapse expand nodes
13.1.2. MInd42
13.1.2.1. Free
13.1.2.2. Android 2.2
13.1.2.2.1. Stock Browser
13.1.3. Google Presentations
13.1.3.1. Try the combination
13.2. Google+ Hangout
13.2.1. No background noise
13.2.2. Sound Breaking
13.2.3. No zoom
13.2.4. Ask Somebody to watch the live stream
13.3. Speach
13.3.1. Don't use often
13.3.1.1. "In general"
13.3.1.2. "Общо взето..."
13.3.1.3. "etc."
13.3.1.4. "така нататък"
13.3.2. use
13.3.2.1. acurate words
13.3.2.2. pay attention to the details
13.3.2.3. the right words and terms
13.3.3. replace
13.3.3.1. "I don't know"
13.3.3.1.1. with "We will check"
13.4. Other technologies for recording
13.4.1. Try Adobe Flash Media Live Encoder
13.4.2. screencast-o-matic
13.4.2.1. Doesn't even start in FF and Chrome
13.4.2.1.1. Java updates done
13.5. YouTube
13.5.1. Create playlists
13.6. Use HD, at least 720p
13.7. Use screen resolution of 1280x720p for YouTbe HD
13.7.1. Use 1280x1024 top and render at the same resolution in Camtasia.
13.7.1.1. YouTube is not cutting anything 1280x1024 is also OK
13.7.1.2. YouTube is filling the right and left with black
13.7.1.3. Renderin at 1280x1024 is much slower compare to 1280x720
13.7.2. Camtasia cuts top and the bottom to fit in 1280x720
13.7.3. Resolution 1280x720 doesn't work on my screen 4:3. Everythign is distorted.
13.8. codenvy was giving me [ERROR] on git push zend but no changes have been made
13.9. Codenvy
13.9.1. consol - very limited
13.9.2. I can upload RAS private key
13.9.3. No SFTP client
13.10. git
13.10.1. problems the way we use it
13.10.2. idea
13.10.2.1. git rm .
13.10.2.1.1. still doesn't work
13.10.2.2. clone in codenvy instead of doing init
13.10.2.3. use patch and diff at the bottom ofthe pull requests
13.10.2.4. try to use clone in the IDE instead of new project
13.10.2.5. merge manualy with patches (no fetch merge)
13.10.2.5.1. Don't use the original for anything else
13.10.3. check the diffs and the changes before merging
13.11. Cloud 9
13.11.1. NO way to upload RSA private key
13.11.2. Very nice consol
13.11.3. You can run your pages there
13.11.4. No SFTP client
13.12. Maybe we don't need AppFog for the students
13.13. pay atention
13.13.1. <?php echo $this->basePath(); ?>/
13.13.2. css, js, images in public
13.13.3. ActionNames
13.13.3.1. mmLayoutAction
13.13.3.1.1. mm-layout.phtml
13.14. workflow (idea)
13.14.1. fork (in github)
13.14.1.1. clone (no fetch merge) in the IDE
13.14.1.1.1. PullRequest
13.15. some students don't use the original repo to create their modules
13.16. some students keep css, js, images in the folder module
13.17. some students don't add $this->basePath() to the URLs in the layout
13.18. some students use <? echo $this->basePath(); ?>
13.19. correct the paths in css files also
13.20. codenvy approaches
13.20.1. create new project
13.20.1.1. use PaaS
13.20.1.1.1. git init
13.20.2. clone repo or import from github
13.20.2.1. NO PaaS
13.20.2.1.1. clone guthub
13.20.2.1.2. clone zend
13.20.2.2. Projects -> New -> Import from github
13.20.2.2.1. NO PaaS
13.20.2.3. Git -> Clone
13.20.2.3.1. NO PaaS
13.21. frequent mistakes
13.21.1. Add ...Controller.php at the end of the controller files
13.21.2. Add ..phtml as an extension for the view files
13.21.3. redirect
14. praktiki.mon.bg
14.1. 12 weeks
14.1.1. 240 hours per student
14.1.2. 8 hours per mentor
14.1.3. Every Monday 10:00 BG time untill 12:00 - lection
14.1.3.1. for 4 weeks 8 hours are over
14.1.3.2. But I will continue
14.1.4. Every working day consultations from 13:00 untill 17:00 15 min per student
14.1.4.1. In 6-7 weeks the 8 hours will be over
14.1.4.2. But I will continue
14.1.4.3. 1:15 per student per week
14.2. 5 projects
14.2.1. 3 students per project
14.2.1.1. designer
14.2.1.2. server site (back end)
14.2.1.3. front end
14.2.2. projects
14.2.2.1. BTK
14.2.2.2. MGS
14.2.2.3. GRD
14.2.2.4. STO
14.2.2.5. NIK
14.3. Open source project
14.3.1. Regrouping
14.4. communication
14.4.1. Google+ for the lections
14.4.1.1. Google Hangout on Air
14.4.2. Skype for the consultations
14.4.2.1. shared audio files in google drive
14.4.3. Everythign recorded
14.5. Documents shared in Google Drive
14.5.1. Schedule
14.5.2. Students
14.6. Virtualisation
14.6.1. Oracle VM Virtual Box
14.6.1.1. free
14.6.2. VMware
14.6.2.1. free
14.7. Design
14.7.1. Responsive Design
14.8. JS toolkits
14.8.1. jQuery
14.8.2. Dojo
14.9. cheatsheet
14.10. coding naming standards
14.11. Self project vs Zfc
14.11.1. self
14.11.1.1. pros
14.11.1.1.1. better learning
14.11.1.2. cons
14.11.1.2.1. low quality in the beginning
14.11.2. Zf-commons
14.11.2.1. pros
14.11.2.1.1. popular
14.11.2.1.2. somebody else has done the job
14.11.2.1.3. high quality?
14.11.2.2. cons
14.11.2.2.1. integration in our project
14.12. Shell we use modules vs building them from scratch?
14.12.1. common opinion is to use already build modules
14.12.1.1. Zf-commons
14.13. Git HowTo
14.13.1. Centralized Workflow
14.13.2. How It Works
14.13.2.1. Managing Conflicts
14.13.3. Example
14.13.3.1. Someone initializes the central repository
14.13.3.2. Everybody clones the central repository
14.13.3.2.1. git clone ssh://user@host/path/to/repo.git
14.13.3.3. John works on his feature
14.13.3.4. Mary works on her feature
14.13.3.5. John publishes his feature
14.13.3.5.1. git push origin master
14.13.3.6. Mary tries to publish her feature
14.13.3.6.1. git push origin master
14.13.3.7. Mary rebases on top of John’s commit(s)
14.13.3.7.1. git pull --rebase origin master
14.13.3.8. Mary resolves a merge conflict
14.13.3.8.1. git status
14.13.3.8.2. solve the problem
14.13.3.8.3. git rebase --abort
14.13.3.9. Mary successfully publishes her feature
14.13.3.10. Mary successfully publishes her feature
14.13.3.10.1. git push origin master
14.13.4. How to merge PR on GitHub?
14.14. Skeleton App how to start?
14.14.1. composer
14.14.2. git
14.14.2.1. composer
14.14.3. ZFTool
14.14.3.1. How to Install ZFTool
14.14.3.1.1. Installation using Composer¶
14.14.3.1.2. Manual installation
14.14.3.1.3. Without installation, using the PHAR file
14.14.3.2. Usage
14.14.3.2.1. Basic information
14.14.3.2.2. Project creation
14.14.3.2.3. Module creation
14.14.3.2.4. Classmap generator
14.14.3.2.5. ZF library installation
14.14.3.2.6. Compile the PHAR file
14.14.3.2.7. What works for me
14.15. tasks
14.15.1. ss
14.15.1.1. install
14.15.1.1.1. install DoctrineModule
14.15.1.1.2. ZfcUser
14.15.1.1.3. BjyAuthorize
14.15.1.1.4. Doctrine ZfcUser ORM
14.15.1.1.5. SamUser
14.15.1.1.6. cdli/CdliTwoStageSignup
14.15.1.1.7. How To Extend ZfcUser (and other modules) to match my needs?
14.15.2. designers
14.15.2.1. viewhelpers in the layout
14.15.3. Ajax in the scripts
14.16. NetBeans (very good IDE)
14.17. CMS
14.17.1. libracms
14.17.1.1. github
14.17.2. GotCms
14.17.2.1. github
14.17.3. DotsCms
14.18. ideas for name
14.18.1. DB naming standards
14.18.2. SPDX OpenSource Registry
14.19. Lessons Learnd
14.19.1. Using modules
14.19.1.1. CMS
14.19.1.1.1. Monolyte system
14.19.1.1.2. They almost don't use other modules
14.19.1.1.3. Like Joomla, Drupal, WordPress
14.19.1.1.4. No way to register user
14.19.1.1.5. Only Admin can login
14.19.1.1.6. You can not have ordinary users
14.19.1.1.7. Not easy to learn and extend
14.19.1.1.8. Specific Installations
14.19.1.1.9. Not separate modules applications
14.19.1.1.10. Black Box
14.19.1.1.11. Very simple sites with the available CMS
14.20. DB Naming convensions
14.20.1. DB design for mere mortals
14.20.1.1. Table types
14.20.1.1.1. Data
14.20.1.1.2. Linking
14.20.1.1.3. Subset
14.20.1.1.4. Validation
14.20.1.2. Table Names
14.20.1.3. Fields
14.20.1.3.1. Resolving Multipart Fields
14.20.1.3.2. Resolving Multivalued Fields
14.21. ZF2
14.21.1. Form
14.21.1.1. Binding
14.21.2. ZF tool
14.21.3. Tutorial App
14.21.4. Controller Plugins
14.21.5. Zend/Mvc
14.21.5.1. on top of
14.21.5.1.1. Zend\ServiceManager
14.21.5.1.2. Zend\EventManager
14.21.5.1.3. Zend\Http
14.21.5.1.4. Zend\Stdlib\DispatchableInterface
14.21.5.2. sub-components are exposed
14.21.5.2.1. Zend\Mvc\Router
14.21.5.2.2. Zend\Http\PhpEnvironment
14.21.5.2.3. Zend\Mvc\Controller
14.21.5.2.4. Zend\Mvc\Service
14.21.5.2.5. Zend\Mvc\View
14.21.5.3. The gateway to the MVC
14.21.5.3.1. Zend\Mvc\Application
14.21.5.4. Bootstrapping an Application
14.22. mysqladmin change password
14.23. Book
14.24. Authorization
14.24.1. How to get Zend\Authentication\AuthenticationService
14.24.1.1. new instance every time
14.24.1.2. alias invocables in the config
14.24.1.2.1. use Controller Plugin
14.24.2. simple approach with direct use
14.24.2.1. cons
14.24.2.1.1. Problem with more complex requirments.
14.24.2.1.2. No central point
14.24.2.1.3. No separation of concern.
14.24.2.1.4. We don't follow DRY
14.24.2.2. pros
14.24.2.2.1. very intuitive
14.25. Authentication with Doctrine
14.25.1. Comparison with and without Doctrine
14.25.2. AuthenticationService has 2 Drivers
14.25.2.1. Authentication
14.25.2.2. Storage
14.25.3. ViewHelper
14.25.4. ControllerPlugin
14.26. RememberMe
14.27. Registration with Doctrine
14.27.1. Form Class vs Annotation
14.28. Forgotten Password with Doctrine
14.29. navigation
14.29.1. 2 types of pages
14.29.1.1. MVC
14.29.1.2. URI
14.29.2. navigation.global.php
14.29.2.1. navigation
14.29.2.2. service_manager
14.29.2.2.1. factories
14.29.3. layout/layout
14.29.3.1. ViewHelper
14.29.4. the guard for the controllers and navigation use different mechanisms
14.29.4.1. navigation view helper
14.29.4.2. event listener
14.29.4.3. These 2 controllers access and navigation are independent systems. We have to sync them
14.30. pagination
14.30.1. Zend Paginator
14.30.1.1. module.config.php
14.30.1.1.1. new rout
14.30.1.2. Controller Index
14.30.1.3. view script
14.30.1.3.1. add the view helper
14.30.1.3.2. create the partial
14.30.2. Doctrine Paginator
14.31. phpUnit
14.32. phpDocumentor
14.32.1. Install
14.32.1.1. PEAR
14.32.1.1.1. $ pear channel-discover pear.phpdoc.org
14.32.1.1.2. $ pear install phpdoc/phpDocumentor
14.32.1.2. composer
14.32.1.2.1. $ php composer.phar require phpdocumentor/phpdocumentor WHEN asked dev-develop
14.32.1.2.2. call it
14.32.1.3. phar
14.32.1.3.1. Download the phar file
14.32.2. Getting Started
14.32.2.1. vendor\bin\phpdoc.php.bat --help
14.32.2.2. vendor\bin\phpdoc.php.bat -d <folder to parse> -t <target>
14.32.2.3. $ phpdoc -d [SOURCE_PATH] -t [TARGET_PATH]
14.32.3. phpdoc.xml
14.32.4. What is a docblock
14.32.5. Which structural elements can have a DocBlock
14.32.5.1. namespace
14.32.5.2. require(_once)
14.32.5.3. include(_once)
14.32.5.4. class
14.32.5.5. interface
14.32.5.6. trait
14.32.5.7. function (including methods)
14.32.5.8. property
14.32.5.9. constant
14.32.5.10. variables, both local and global scope.
14.32.6. A DocBlock roughly exists of 3 sections:
14.32.6.1. Short Description; a one-liner
14.32.6.2. Long Description;
14.32.6.3. Tags;
14.32.7. Inline tag reference
14.32.7.1. inline
14.32.7.1.1. {@example [location] [<start-line> [<number-of-lines>] ] [<description>]}
14.32.7.2. tag
14.32.7.2.1. @example [location] [<start-line> [<number-of-lines>] ] [<description>]
14.32.8. Definition of a ‘Type’
14.32.8.1. string
14.32.8.2. integer or int
14.32.8.3. boolean or bool
14.32.8.4. float or double
14.32.8.5. object
14.32.8.6. mixed
14.32.8.7. array
14.32.8.8. resource
14.32.8.9. void
14.33. php-cs-fixer
14.33.1. GitHub
14.33.2. Install
14.33.2.1. Download php-cs-fixer.phar
14.33.3. Run
14.33.4. Update
14.33.5. Usage
14.33.5.1. On my system
14.33.5.2. Dry run
14.33.5.3. One file only
14.34. php-CodeSniffer
14.34.1. GitHub
14.34.2. Documentation
14.34.3. composer installation
14.34.4. blog
14.34.5. Documentation
14.35. less popular CS
14.35.1. PHPCP
14.35.2. Flitch
14.36. PSR-0, PSR-1, PSR-2
14.36.1. PSR0