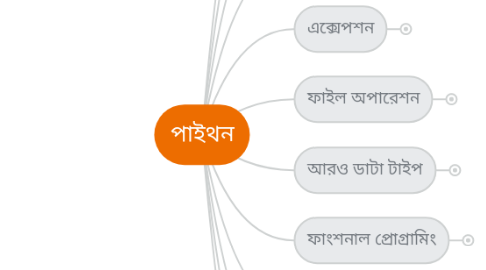
1. ব্যাসিক
1.1. পরিচিতি
1.1.1. হাই লেভেল, জেনারেল পারপাস প্রোগ্রামিং ল্যাঙ্গুয়েজ
1.1.2. ওয়েব, মোবাইল, আর্টিফিশিয়াল ইন্টেলিজেন্স, ডাটা সায়েন্স সহ অনেক ক্ষেত্রে ব্যবহার করা যায়
1.1.3. রানটাইমে পাইথন প্রসেসড হয়, কম্পাইল করার দরকার নাই
1.1.4. ৩টি প্রধান ভার্সন 1.x, 2.x, 3.x
1.1.5. আরও জানতে
1.2. কনসোল
1.2.1. পাইথন ইন্সটলেশনের সাথে থাকে IDLE
1.2.2. Mac, Linux এ বিল্ট ইন পাইথন থাকায় টার্মিনাল থেকে interpreter চালু করা যায়
1.2.3. একে REPL (a read-eval-print loop) -ও বলা হয়
1.2.4. কনসোল/REPL বন্ধ করতে exit() বা quit() লিখে এন্টার চাপতে হয়
1.3. সিম্পল অপারেশন
1.3.1. >>> 5+2 7 >>> 5 - 2 3 >>> 2 * (3 + 4) 14 >>> 10 / 3 3.33333...
1.4. Float
1.4.1. যে সংখ্যা গুলো Integer বা পূর্ণ সংখ্যা নয় তাদের প্রকাশ করতে float ব্যবহার হয়
1.4.2. >>> a = 10.5 >>> a 10.5 >>> type(a) <class 'float'> >>> 50/5 10.0 >>> pi = .314e1 >>> pi 3.14
1.5. আরও কিছু নিউমেরিক অপারেশন
1.5.1. >>> 2 ** 5 #exponentiation 32 >>> 9 ** (1/2) 3.0
1.5.2. >>> 20 // 6 #quotient 3 >>> 10 % 3 #remainder 1
1.6. String
1.6.1. >>> "Python is fun!" 'Python is fun!' >>> 'Always look on the bright side of life' 'Always look on the bright side of life'
1.6.2. >>> 'I\'m Nuhil' "I'm Nuhil"
1.6.3. >>> """Name: Nuhil ... age: 28""" 'Name: Nuhil\nage: 28'
1.7. ইনপুট - আউটপুট
1.7.1. >>> print(1 + 1) 2 >>> print("Hello\nWorld!") Hello World!
1.7.2. >>> input("Enter something please: ") Enter something please: This is what\nthe user enters! 'This is what\\nthe user enters!'
1.8. String অপারেশন
1.8.1. >>> "Spam" + 'eggs' 'Spameggs' >>> print("First string" + ", " + "second string") First string, second string
1.8.2. >>> "2" + "2" '22' >>> 1 + '2' + 3 + '4' Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: unsupported operand type(s) for +: 'int' and 'str'
1.8.3. >>> print("spam" * 3) spamspamspam >>> 4 * '2' '2222' >>> '17' * '87' TypeError: can't multiply sequence by non-int of type 'str'
1.9. টাইপ কনভার্সন
1.9.1. >>> "2" + "3" '23' >>> int("2") + int("3") 5
1.10. ভ্যারিয়েবল
1.10.1. >>> x = 7 >>> print(x) 7 >>> print(x + 3) 10 >>> print(x) 7
1.10.2. >>> x = 123.456 >>> print(x) 123.456 >>> x = "This is a string" >>> print(x + "!") This is a string!
1.10.3. >>> this_is_a_normal_name = 7 >>> 123abc = 7 SyntaxError: invalid syntax >>> spaces are not allowed SyntaxError: invalid syntax
1.10.4. >>> foo 'a string' >>> del foo >>> foo NameError: name 'foo' is not defined
1.11. ইনপ্লেস অপারেটর
1.11.1. >>> x = 2 >>> print(x) 2 >>> x += 3 #x = x + 3 >>> print(x) 5
2. কন্ট্রোল স্ট্রাকচার
2.1. বুলিয়ান ও তুলনা
2.1.1. >>> my_boolean = True >>> my_boolean True >>> 2 == 3 False >>> "hello" == "hello" True
2.1.2. >>> 1 != 1 False >>> "eleven" != "seven" True >>> 2 != 10 True
2.1.3. >>> 7 > 5 True >>> 10 < 10 False
2.1.4. >>> 7 <= 8 True >>> 9 >= 9.0 True
2.1.5. >>> a = 10 >>> b = 20 >>> a is not b True >>> a is b False
2.2. if
2.2.1. >>> if 10 > 5: print("10 is greater than 5") #Output 10 is greater than 5
2.2.2. num = 12 if num > 5: print("Bigger than 5") if num <=47: print("Between 5 and 47") #Output Bigger than 5 Between 5 and 47
2.3. else
2.3.1. x = 4 if x == 5: print("Yes") else: print("No") #Output No
2.3.2. num = 7 if num == 5: print("Number is 5") elif num == 11: print("Number is 11") elif num == 7: print("Number is 7") else: print("Number isn't 5, 11 or 7") -------------------- #Output Number is 7
2.4. বুলিয়ান লজিক
2.4.1. >>> 1 == 1 and 2 == 2 True >>> 1 == 1 and 2 == 3 False >>> 1 != 1 and 2 == 2 False >>> 2 < 1 and 3 > 6 False
2.4.2. >>> 1 == 1 or 2 == 2 True >>> 1 == 1 or 2 == 3 True >>> 1 != 1 or 2 == 2 True >>> 2 < 1 or 3 > 6 False
2.4.3. >>> not 1 == 1 False >>> not 1 > 7 True
2.5. অপারেটর প্রাধান্য
2.5.1. >>> False == False or True True >>> False == (False or True) False >>> (False == False) or True True
2.5.2. ** ~+- * / % // +- >><< & ^| <= < > >= <> == != = %= /= //= -= += *= **= is is not in not in not or and
2.6. while লুপ
2.6.1. i = 1 while i <= 5: print(i) i = i + 1 print("Finished!") --------------- #Output 1 2 3 4 5 Finished!
2.6.2. break
2.6.2.1. i = 0 while i < 10: print(i) i += 1 if i == 5: break --------- #Output 0 1 2 3 4
2.6.3. continue
2.6.3.1. i = 0 while True: i = i +1 if i == 2: print("Skipping 2") continue if i == 5: print("Breaking") break print(i) print("Finished") #Output 1 Skipping 2 3 4 Breaking Finished
2.7. লিস্ট
2.7.1. words = ["Hello", "world", "!"] print(words[0]) print(words[1]) print(words[2]) -------------------------------------- #Output Hello world !
2.7.2. number = 3 things = ["string", 0, [1, 2, number], 4.56] print(things[1]) print(things[2]) print(things[2][2]) -------------------------------------- #Output 0 [1, 2, 3] 3
2.7.3. str = "Hello world!" print(str[6]) ---------------------- #Output w
2.8. list অপারেশন
2.8.1. nums = [7, 7, 7, 7, 7] nums[2] = 5 print(nums) ---------------- #Output [7, 7, 5, 7, 7]
2.8.2. nums = [1, 2, 3] print(nums + [4, 5, 6]) print(nums * 3) ------------------------ #Output [1, 2, 3, 4, 5, 6] [1, 2, 3, 1, 2, 3, 1, 2, 3]
2.8.3. words = ["spam", "egg", "spam", "sausage"] print("spam" in words) print("egg" in words) print("tomato" in words) ------------------------------------- #Output True True False
2.8.4. nums = [1, 2, 3] print(not 4 in nums) print(4 not in nums) print(not 3 in nums) print(3 not in nums) ----------------------- #Output True True False False
2.8.5. numbers = [1,2,3,4,5,6,7,8,9,10] last_number = numbers[-1] print(last_number) first_five_numbers = numbers[0:5] print(first_five_numbers) last_five_numbers = numbers[-6:-1] print(last_five_numbers) ------------------------------------- #Output 10 [1, 2, 3, 4, 5] [5, 6, 7, 8, 9]
2.9. list ফাংশন সমূহ
2.9.1. nums = [1, 2, 3] nums.append(4) print(nums) --------------- #Output [1, 2, 3, 4]
2.9.2. nums = [1, 3, 5, 2, 4] print(len(nums)) --------------- #Output 5
2.9.3. words = ["Python", "fun"] words.insert(1, "is") print(words) -------------------------- #Output ['Python', 'is', 'fun']
2.9.4. letters = ['p', 'q', 'r', 's', 'p', 'u'] print(letters.index('r')) print(letters.index('p')) print(letters.index('z')) ---------------------------- #Output 2 0 ValueError: 'z' is not in list
2.10. Range রেঞ্জ
2.10.1. numbers = list(range(10)) print(numbers) --------------- #Output [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
2.10.2. numbers = list(range(3, 8)) print(numbers) ---------------- #Output [3, 4, 5, 6, 7]
2.10.3. numbers = list(range(5, 20, 2)) print(numbers) ------------------------- #Output [5, 7, 9, 11, 13, 15, 17, 19]
2.11. for লুপ
2.11.1. for i in range(5): print("hello!") ---------- #Output hello! hello! hello! hello! hello!
2.11.2. for letter in 'Python': print(letter) ------------------------------ #Output P y t h o n
2.11.3. words = ["hello", "world", "spam", "eggs"] for word in words: print(word + "!") ------------------------------ #Output hello! world! spam! eggs!
3. ফাংশন ও মডিউল
3.1. কোডের পুনব্যবহার
3.1.1. DRY - Do not Repeat Yourself
3.1.2. ফাংশন ও মডিউল ব্যবহার করে DRY মেইন্টেইন করা যায়
3.1.3. WET - Write Everything Twice
3.2. ফাংশন
3.2.1. def my_func(): print("spam") my_func() ------------------ #Output spam
3.2.2. hello() def hello(): print("Hello world!") ------------------------- #Output NameError: name 'hello' is not defined
3.3. আর্গুমেন্ট
3.3.1. def print_with_exclamation(word): print(word + "!") print_with_exclamation("spam") --------------------------- #Output spam!
3.3.2. def print_sum_twice(x, y): print(x + y) print(x + y) print_sum_twice(5, 8) --------------------------------- #Output 13 13
3.3.3. def function(variable): variable += 1 print(variable) function(7) print(variable) --------------------------- #Output 8 NameError: name 'variable' is not defined
3.4. রিটার্ন
3.4.1. def give_double(x): return x * 2 my_double = give_double(10) print(my_double) -------------------------------- #Output 20
3.4.2. def add_numbers(x, y): total = x + y return total print("This won't be printed") print(add_numbers(4, 5)) -------------------------------- #Output 9
3.5. কমেন্ট ও ডক স্ট্রিং
3.5.1. x = 365 y = 7 # this is a comment print(x % y) # find the remainder # print (x // y) # another comment --------------------------- #Output 1
3.5.2. def shout(word): """ Print a word with an exclamation mark following it. """ print(word + "!") shout("spam") print(shout.__doc__) #To print the doc string --------------------------- #Output spam! Print a word with an exclamation mark following it.
3.6. অবজেক্ট হিসেবে ফাংশন
3.6.1. def multiply(x, y): return x * y a = 4 b = 7 operation = multiply print(operation(a, b)) ---------------- #Output 28
3.6.2. def add(x, y): return x + y def do_twice(func, x, y): return func(func(x, y), func(x, y)) a = 5 b = 10 print(do_twice(add, a, b)) ---------------------------------------- #Output 30
3.7. মডিউল
3.7.1. import random for i in range(5): value = random.randint(1, 6) print(value) ------------------------------ #Output 2 3 6 5 4
3.7.2. from math import pi print(pi) ----------------- #Output 3.141592653589793
3.7.3. from math import sqrt as square_root print(square_root(100)) -------------------- #Output 10.0
3.8. pip ও স্ট্যান্ডার্ড লাইব্রেরী
3.8.1. string, re, datetime, math, random, os, multiprocessing, subprocess, socket, email, json, doctest, unittest, pdb
3.8.2. অনেকের ডেভেলপ করা মডিউল জমা থাকে Python Package Index (PyPi) তে
3.8.3. pip নামের টুল/প্রোগ্রাম ব্যবহার করে এগুলো ইন্সটল করে নেয়া যায়
3.8.4. আরও জানতে
4. এক্সেপশন
4.1. এক্সেপশন কি
4.1.1. ভুল কোন কিছু ঘটে গেলে এটি তৈরি হয়
4.1.2. num1 = 7 num2 = 0 print(num1/num2) ------------------------- #Output ZeroDivisionError: division by zero
4.1.3. ImportError: an import fails; IndexError: a list is indexed with an out-of-range number; NameError: an unknown variable is used; SyntaxError: the code can't be parsed properly; TypeError: a function is called on a value of an inappropriate type; ValueError: a function is called on a value of the correct type, but with an inappropriate value.
4.2. এক্সেপশন হ্যান্ডলিং
4.2.1. try: num1 = 7 num2 = 0 print (num1 / num2) print("Done calculation") except ZeroDivisionError: print("An error occurred") print("due to zero division") ----------------------- #Output An error occurred due to zero division
4.2.2. try: variable = 10 print(variable + "hello") print(variable / 2) except ZeroDivisionError: print("Divided by zero") except (ValueError, TypeError): print("Error occurred") ----------------------------------- #Output Error occurred
4.2.3. try: word = "spam" print(word / 0) except: print("An error occurred") ----------------------- #Output An error occurred
4.3. ফাইনালি
4.3.1. try: print("Hello") print(1 / 0) except ZeroDivisionError: print("Divided by zero") finally: print("This code will run no matter what") ----------------------------------- #Output Hello Divided by zero This code will run no matter what
4.4. এক্সেপশন রেইজ করা
4.4.1. print(1) raise ValueError print(2) ------------- #Output 1 ValueError
4.4.2. name = "123" raise NameError("Invalid name!") --------------------------------- #Output NameError: Invalid name!
4.4.3. try: num = 5 / 0 except: print("An error occurred") raise -------------------------- #Output An error occurred ZeroDivisionError: division by zero
4.5. Assertion
4.5.1. print(1) assert 2 + 2 == 4 print(2) assert 1 + 1 == 3 print(3) ----------------- #Output 1 2 AssertionError
4.5.2. temp = -10 assert (temp >= 0), "Colder than absolute zero!" --------------------------------------------- #Output AssertionError: Colder than absolute zero!
5. ফাইল অপারেশন
5.1. ফাইল খোলা
5.1.1. myfile = open("filename.txt")
5.1.2. # write mode open("filename.txt", "w") # read mode open("filename.txt", "r") # append mode open("filename.txt", "a") # binary write mode open("filename.txt", "wb")
5.1.3. file = open("filename.txt", "w") # do stuff to the file file.close()
5.2. ফাইল থেকে পড়া
5.2.1. file = open("filename.txt", "r") cont = file.read() print(cont) file.close() ------------------------ #Output will be content of that file
5.2.2. file = open("filename.txt", "r") print(file.read(16)) ------------------------------------ #Output will be first 16 byte of the file
5.2.3. file = open("filename.txt", "r") print(file.readlines()) file.close() --------------------------- #Output ['Line 1 text \n', 'Line 2 text \n', 'Line 3 text']
5.2.4. file = open("filename.txt", "r") for line in file: print(line) file.close() --------------------------- #Output Line 1 text Line 2 text Line 3 text
5.3. ফাইলে লেখা
5.3.1. file = open("newfile.txt", "w") file.write("This has been written to a file") file.close() file = open("newfile.txt", "r") print(file.read()) file.close()
5.3.2. file = open("newfile.txt", "r") print(file.read()) Hello file.close() file = open("newfile.txt", "a") file.write(" World!") 7 file.close() file = open("newfile.txt", "r") file.read() 'Hello World!' file.close()
5.4. ফাইল নিয়ে কাজের টিপস
5.4.1. try: f = open("filename.txt") print(f.read()) finally: f.close()
5.4.2. with open("filename.txt") as f: print(f.read())
6. ব্যবহার বিধি
6.1. (ব্যবহার বিধি) থেকে (ক্রেডিট) পর্যন্ত ক্রম অনুযায়ী দেখুন
6.2. যেখানে এই চিহ্ন দেখবেন সেখানে বর্ণনা মূলক নোট আছে
6.3. এই চিহ্ন এখানে একটি রেফারেন্স লিঙ্ককে নির্দেশিত করে
6.4. সবুজ রঙের চেক মার্ক মানে হচ্ছে এই কোড ব্লকটি এখনি টেস্ট করা যাবে, লিঙ্ক চিহ্নে ক্লিক করে
7. ক্রেডিট
7.1. ইন্টারনেটের বিভিন্ন সোর্স থেকে নেয়া
7.2. শীঘ্রই নির্দিষ্ট করে লিঙ্ক গুলো যুক্ত করে দেয়া হবে
7.3. সমন্বয়ক ও যোগাযোগ - Nuhil Mehdy -
8. আরও ডাটা টাইপ
8.1. None
8.1.1. >>> None == None True >>> None >>> print(None) None >>>
8.1.2. def some_func(): print("Hi!") var = some_func() print(var) ----------------- #Output Hi! None
8.2. ডিকশনারি
8.2.1. ages = {"Dave": 24, "Mary": 42, "John": 58} print(ages["Dave"]) print(ages["Mary"]) ------------------------------ #Output 24 42
8.2.2. primary = { "red": [255, 0, 0], "green": [0, 255, 0], "blue": [0, 0, 255], } print(primary["red"]) print(primary["yellow"]) ------------------ #Output [255, 0, 0] KeyError: 'yellow'
8.3. ডিকশনারি ফাংশন সমূহ
8.3.1. squares = {1: 1, 2: 4, 3: "error", 4: 16,} squares[8] = 64 squares[3] = 9 print(squares) --------------------------- #Output {8: 64, 1: 1, 2: 4, 3: 9, 4: 16}
8.3.2. nums = { 1: "one", 2: "two", 3: "three", } print(1 in nums) print("three" in nums) print(4 not in nums) ------------------ #Output True False True
8.3.3. pairs = {1: "apple", "orange": [2, 3, 4], True: False, None: "True", } print(pairs.get("orange")) print(pairs.get(7)) print(pairs.get(12345, "not in dictionary")) ------------------------------------ #Output [2, 3, 4] None not in dictionary
8.4. টাপল
8.4.1. words = ("spam", "eggs", "sausages",) print(words[0]) --------------------------- #Output spam
8.4.2. words = ("spam", "eggs", "sausages",) words[1] = "cheese" --------------------------------- #Output Traceback (most recent call last): File "", line 1, in TypeError: 'tuple' object does not support item assignment
8.4.3. my_tuple = "one", "two", "three" print(my_tuple[0]) ----------------------- #Output one
8.5. লিস্ট স্লাইস
8.5.1. squares = [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] print(squares[2:6]) print(squares[3:8]) print(squares[0:1]) -------------------------------------- #Output [4, 9, 16, 25] [9, 16, 25, 36, 49] [0]
8.5.2. squares = [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] print(squares[:7]) print(squares[7:]) --------------------------------- #Output [0, 1, 4, 9, 16, 25, 36] [49, 64, 81]
8.5.3. squares = [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] print(squares[::2]) print(squares[2:8:3]) --------------------------------- #Output [0, 4, 16, 36, 64] [4, 25]
8.5.4. squares = [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] print(squares[1:-1]) --------------------------- #Output [1, 4, 9, 16, 25, 36, 49, 64]
8.5.5. squares = [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] print(squares[::-1]) ------------------------------------ #Output [81, 64, 49, 36, 25, 16, 9, 4, 1, 0]
8.6. লিস্ট কম্প্রিহেনশন
8.6.1. cubes = [i**3 for i in range(5)] print(cubes) ----------------------- #Output [0, 1, 8, 27, 64]
8.6.2. evens=[i**2 for i in range(10) if i**2 % 2 == 0] print(evens) ------------------------------- #Output [0, 4, 16, 36, 64]
8.7. স্ট্রিং ফরম্যাটিং
8.7.1. nums = [4, 5, 6] msg = "Numbers: {0} {1} {2}". format(nums[0], nums[1], nums[2]) print(msg) ----------------------------------- #Output Numbers: 4 5 6
8.7.2. a = "{x}, {y}".format(x=5, y=12) print(a) -------------------- #Output 5, 12
8.8. কিছু উপকারী ফাংশন
8.8.1. string
8.8.1.1. print(", ".join(["spam", "eggs", "ham"])) #prints "spam, eggs, ham" print("Hello ME".replace("ME", "world")) #prints "Hello world" print("This is a sentence.".startswith("This")) # prints "True" print("This is a sentence.".endswith("sentence.")) # prints "True" print("This is a sentence.".upper()) # prints "THIS IS A SENTENCE." print("AN ALL CAPS SENTENCE".lower()) #prints "an all caps sentence" print("spam, eggs, ham".split(", ")) #prints "['spam', 'eggs', 'ham']"
8.8.2. numeric
8.8.2.1. print(min(1, 2, 3, 4, 0, 2, 1)) print(max([1, 4, 9, 2, 5, 6, 8])) print(abs(-99)) print(abs(42)) print(sum([1, 2, 3, 4, 5])) ------------------- #Output 0 9 99 42 15
8.8.3. list
8.8.3.1. nums = [55, 44, 33, 22, 11] if all([i > 5 for i in nums]): print("All larger than 5") if any([i % 2 == 0 for i in nums]): print("At least one is even") for v in enumerate(nums): print(v) ------------------------ #Output All larger than 5 At least one is even (0, 55) (1, 44) (2, 33) (3, 22) (4, 11)
9. ফাংশনাল প্রোগ্রামিং
9.1. ভূমিকা
9.1.1. প্রোগ্রামিং স্টাইল যেটা বিশেষত নির্ভর করে ফাংশনের উপর
9.1.2. higher-order-function গুলো এই ধারার মুল জিনিষ
9.1.2.1. def make_twice(func, arg): return func(func(arg)) def add_five(x): return x + 5 print(make_twice(add_five, 10)) -------------------- #Output 20
9.1.3. পিওর ফাংশন
9.1.3.1. def my_pure_function(a,b): c = (2 * a) + (2 * b) return c my_pure_function(5,10) ------------------------ #Output 30
9.1.4. ইম্পিওর ফাংশন
9.1.4.1. my_list = [] def my_impure_function(arg): my_list.append(arg) my_impure_function(10) print(my_list) ------------------------ #Output [10]
9.2. ল্যাম্বডা
9.2.1. def my_function(func, arg): return func(arg) print(my_function(lambda x: 2 * x, 5)) ----------------------- #Output 10
9.2.2. print((lambda x,y: x + 2 * y)(2,3)) ----------------------- #Output 8
9.3. ম্যাপ ও ফিল্টার
9.3.1. def make_double(x): return x * 2 my_marks = [10, 12, 20, 30] result = map(make_double, my_marks) print(list(result)) ------------------------ #Output [20, 24, 40, 60]
9.3.2. def is_even(x): return x % 2 == 0 my_numbers = [1,2,3,4,5,6] result = filter(is_even, my_numbers) print(list(result)) --------------------------- #Output [2, 4, 6]
9.4. জেনারেটর
9.4.1. def my_iterable(): i = 5 while i > 0: yield i i -= 1 for i in my_iterable(): print(i) --------------------------- #Output 5 4 3 2 1
9.4.2. def even_numbers(x): for i in range(x): if i % 2 == 0: yield i even_nums_list = list(even_numbers(10)) print(even_nums_list) ------------------------------- #Output [0, 2, 4, 6, 8]
9.5. ডেকোরেটর
9.5.1. def my_decorator(func): def decorate(): print("****************") func() print("****************") return decorate def print_raw(): print("Clear Text") decorated_function = my_decorator(print_raw) decorated_function() --------------------------------------------- #Output **************** Clear Text ****************
9.5.2. def my_decorator(func): def decorate(): print("****************") func() print("****************") return decorate @my_decorator def print_text(): print("Hello World!") --------------------------------------------- #Output **************** Clear Text ****************
9.6. রিকারসন
9.6.1. def factorial(x): if x == 1: return 1 else: return x * factorial(x-1) print(factorial(5)) --------------------------------- #Output 120
9.6.2. def is_even(x): if x == 0: return True else: return is_odd(x-1) def is_odd(x): return not is_even(x) print(is_odd(17)) print(is_even(23)) ------------------------ #Output True False
9.7. সেট
9.7.1. num_set = {1, 2, 3, 4, 5} word_set = set(["spam", "eggs", "sausage"]) print(3 in num_set) print("spam" not in word_set) ---------------------------- #Output True False
9.7.2. সেট এর ভ্যালু গুলো অর্ডারড (ক্রম অনুসারে সাজানো) নয়
9.7.3. nums = {1, 2, 1, 3, 1, 4, 5, 6} print(nums) -------------------- #Output {1, 2, 3, 4, 5, 6}
9.7.4. অপারেশন
9.7.4.1. first = {1, 2, 3, 4, 5, 6} second = {4, 5, 6, 7, 8, 9} print(first | second) print(first & second) print(first - second) print(second - first) print(first ^ second) -------------------- #Output {1, 2, 3, 4, 5, 6, 7, 8, 9} {4, 5, 6} {1, 2, 3} {8, 9, 7} {1, 2, 3, 7, 8, 9}
9.8. itertools
9.8.1. এটা একটা স্ট্যান্ডার্ড লাইব্রেরী যার মধ্যে বেশ কিছু গুরুত্বপূর্ণ ফাংশন আছে যেগুলো ফাংশনাল প্রোগ্রামিং এর জন্য উপকারী
9.8.2. from itertools import count for i in count(3): print(i) if i >=11: break --------------------- #Output 3 4 5 6 7 8 9 10 11
9.8.3. from itertools import accumulate, takewhile nums = list(accumulate(range(8))) print(nums) print(list(takewhile(lambda x: x<= 6, nums))) -------------------------------------------- #Output [0, 1, 3, 6, 10, 15, 21, 28] [0, 1, 3, 6]
10. অবজেক্ট ওরিয়েন্টেড
10.1. ক্লাস
10.1.1. class Cat: def __init__(self, color, legs): self.color = color self.legs = legs felix = Cat("ginger", 4) print(felix.color) -------------------------- #Output ginger
10.1.2. class Dog: def __init__(self, name, color): self.name = name self.color = color def bark(self): print("Woof!") fido = Dog("Fido", "brown") print(fido.name) fido.bark() ------------------------------- #Output Fido Woof!
10.1.3. class Dog: legs = 4 def __init__(self, name, color): self.name = name self.color = color fido = Dog("Fido", "brown") print(fido.legs) print(Dog.legs) ----------------------------- #Output 4 4
10.2. ইনহেরিট্যান্স
10.2.1. class Animal: def __init__(self, name, color): self.name = name self.color = color class Cat(Animal): def purr(self): print("Purr...") class Dog(Animal): def bark(self): print("Woof!") fido = Dog("Fido", "brown") print(fido.color) fido.bark() --------------------- #Output brown Woof!
10.2.2. class Wolf: def __init__(self, name, color): self.name = name self.color = color def bark(self): print("Grr...") class Dog(Wolf): def bark(self): print("Woof") husky = Dog("Max", "grey") husky.bark() ----------------------- #Output Woof
10.2.3. class A: def method(self): print("A method") class B(A): def another_method(self): print("B method") class C(B): def third_method(self): print("C method") c = C() c.method() c.another_method() c.third_method() ------------------------- #Output A method B method C method
10.2.4. class A: def spam(self): print(1) class B(A): def spam(self): print(2) super().spam() B().spam() ---------------- #Output 2 1
10.3. ম্যাজিক মেথড ও অপারেটর অভারলোডিং
10.3.1. class Vector2D: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): return Vector2D(self.x + other.x, self.y + other.y) first = Vector2D(5, 7) second = Vector2D(3, 9) result = first + second print(result.x) print(result.y) ------------------------------- #Output 8 16
10.3.2. __sub__ for - __mul__ for * __truediv__ for / __floordiv__ for // __mod__ for % __pow__ for ** __and__ for & __xor__ for ^ __or__ for |
10.3.3. __lt__ for < __le__ for <= __eq__ for == __ne__ for != __gt__ for > __ge__ for >=
10.3.4. __len__ for len() __getitem__ for indexing __setitem__ for assigning to indexed values __delitem__ for deleting indexed values __iter__ for iteration over objects (e.g., in for loops) __contains__ for in
10.3.4.1. import random class VagueList: def __init__(self, cont): self.cont = cont def __getitem__(self, index): return self.cont[index + random.randint(-1, 1)] def __len__(self): return random.randint(0, len(self.cont)*2) vague_list = VagueList(["A", "B", "C", "D", "E"]) print(len(vague_list)) print(len(vague_list)) print(vague_list[2]) print(vague_list[2]) -------------------------------------------------- #Output 6 7 D C
10.4. অবজেক্টের জীবনকাল
10.4.1. creation, manipulation, destruction
10.4.2. একটি অবজেক্টের Destruction ঘটে যখন এর reference কাউন্ট শূন্য হয়ে যায়
10.4.2.1. del স্টেটমেন্ট দিয়ে রেফারেন্স কাউন্ট এর মান কমানো যায়
10.4.3. অপ্রয়োজনীয় তথা শূন্য রেফারেন্স কাউন্ট ওয়ালা অবজেক্টদের ডিলিট করার কাজকেই গারবেজ কালেকশন বলা হয়ে থাকে
10.4.4. a = 42 # Create object <42> b = a # Increase ref. count of <42> c = [a] # Increase ref. count of <42> del a # Decrease ref. count of <42> b = 100 # Decrease ref. count of <42> c[0] = -1 # Decrease ref. count of <42>
10.5. ডাটা হাইডিং
10.5.1. encapsulation
10.5.1.1. পাইথনে সরাসরি এই ধারনা নাই
10.5.2. "we are all consenting adults here"
10.5.3. বরং ইউজারকে ক্লাসের কোন ইমপ্লিমেন্টেশন ডিটেইলকে অ্যাক্সেস করতে নিরুৎসাহিত করা হয় অন্য ভাবে
10.5.4. weakly private
10.5.4.1. এরকম মেথড ও অ্যাট্রিবিউট গুলোর সামনে সিঙ্গেল আন্ডার স্কোর দিয়ে নির্দেশিত করা হয়
10.5.4.2. যদিও কড়াকড়ি ভাবে এটা অন্যদের কাছ থেকে অ্যাক্সেস বন্ধ করে না কিন্তু নিরুৎসাহিত করার জন্য প্রকাশ করে
10.5.4.3. কিন্তু
10.5.4.3.1. from module_name import * এভাবে ইম্পোরট করা ক্লাসের সেই "সিঙ্গেল আন্ডার স্কোর" ওয়ালা এলিমেন্ট গুলো ইম্পোরট হবে না
10.5.4.4. class Queue: def __init__(self, contents): self._hiddenlist = list(contents) def push(self, value): self._hiddenlist.insert(0, value) def pop(self): return self._hiddenlist.pop(-1) def __repr__(self): return "Queue({})".format(self._hiddenlist) queue = Queue([1, 2, 3]) print(queue) queue.push(0) print(queue) queue.pop() print(queue) print(queue._hiddenlist) ------------------------------------- #Output Queue([1, 2, 3]) Queue([0, 1, 2, 3]) Queue([0, 1, 2]) [0, 1, 2]
10.5.5. strongly private
10.5.5.1. এলিমেন্ট এর সামনে ডাবল আন্ডারস্কোর দিয়ে স্ট্রং প্রাইভেসি প্রকাশ করা হয়
10.5.5.2. class Spam: __egg = 7 def print_egg(self): print(self.__egg) s = Spam() s.print_egg() print(s._Spam__egg) print(s.__egg) --------------- #Output 7 7 AttributeError: 'Spam' object has no attribute '__egg'
10.6. ক্লাস ও স্ট্যাটিক মেথড
10.6.1. ক্লাস মেথড
10.6.1.1. class Rectangle: def __init__(self, width, height): self.width = width self.height = height def calculate_area(self): return self.width * self.height @classmethod def new_square(cls, side_length): return cls(side_length, side_length) #instantiation done correctly even with one argument square = Rectangle.new_square(5) #So, it can now call class's regular method print(square.calculate_area()) --------------------------------------------- #Output 25
10.6.2. স্ট্যাটিক মেথড
10.6.2.1. class Pizza: def __init__(self, toppings): self.toppings = toppings @staticmethod def validate_topping(topping): if topping == "pineapple": raise ValueError("No pineapples!") else: return True ingredients = ["cheese", "onions", "spam"] if all(Pizza.validate_topping(i) for i in ingredients): pizza = Pizza(ingredients) print(pizza) --------------------------------- #Output <__main__.Pizza object at 0x1057a2908>
10.7. প্রোপার্টি
10.7.1. class Pizza: def __init__(self, toppings): self.toppings = toppings @property def pineapple_allowed(self): return False pizza = Pizza(["cheese", "tomato"]) print(pizza.pineapple_allowed) pizza.pineapple_allowed = True ---------------------- #Output False AttributeError: can't set attribute
10.7.2. class Pizza: def __init__(self, toppings): self.toppings = toppings self._pineapple_allowed = False @property def pineapple_allowed(self): return self._pineapple_allowed @pineapple_allowed.setter def pineapple_allowed(self, value): if value: password = input("Enter the password: ") if password == "Sw0rdf1sh!": self._pineapple_allowed = value else: raise ValueError("Alert! Intruder!") --------------------------- #Output False Enter the password to permit pineapple: Sw0rdf1sh! True
11. পাইথনিকনেস ও প্যাকেজিং
11.1. Zen of Python
11.1.1. The Zen of Python, by Tim Peters Beautiful is better than ugly. Explicit is better than implicit. Simple is better than complex. Complex is better than complicated. Flat is better than nested. Sparse is better than dense. Readability counts. Special cases aren't special enough to break the rules. Although practicality beats purity. Errors should never pass silently. Unless explicitly silenced. In the face of ambiguity, refuse the temptation to guess. There should be one-- and preferably only one --obvious way to do it. Although that way may not be obvious at first unless you're Dutch. Now is better than never. Although never is often better than *right* now. If the implementation is hard to explain, it's a bad idea. If the implementation is easy to explain, it may be a good idea. Namespaces are one honking great idea -- let's do more of those!
11.2. PEP
11.2.1. Python Enhancement Proposals
11.2.2. অভিজ্ঞ পাইথন প্রোগ্রামারগণ এই ল্যাঙ্গুয়েজের উন্নয়নের জন্য প্রস্তাবনা রাখেন
11.2.3. যেমন
11.2.3.1. একটি লাইন 80 ক্যারেক্টার এর বেশি লম্বা হওয়া উচিৎ নয়
11.2.3.2. from module import * ব্যবহার উচিৎ নয়
11.2.3.3. এক লাইনে একটিই স্টেটমেন্ট থাকা উচিৎ
11.2.3.4. ইত্যাদি...
11.3. ফাংশন আর্গুমেন্ট (বর্ধিত)
11.3.1. def function(named_arg, *args): print(named_arg) print(args) function(1, 2, 3, 4, 5) ------------------------------ #Output 1 (2, 3, 4, 5)
11.3.2. def function(x, y, food="spam"): print(food) function(1, 2) function(3, 4, "egg") ----------------- #Output spam egg
11.3.3. def my(x, y=7, *args, **kwargs): print(x) print(y) print(args) print(kwargs) my(2,3,4,5,6,a=7,b=8) ------------------------ #Output 2 3 (4, 5, 6) {'a': 7, 'b': 8}
11.4. টাপল আনপ্যাকিং
11.4.1. numbers = (1, 2, 3) a, b, c = numbers print(a) print(b) print(c) ---------- #Output 1 2 3
11.4.2. a, b, *c, d = [1, 2, 3, 4, 5, 6, 7, 8, 9] print(a) print(b) print(c) print(d) ----------------------------------- #Output 1 2 [3, 4, 5, 6, 7, 8] 9
11.5. টারনারি অপারেটর
11.5.1. a = 7 b = 1 if a >= 5 else 42 print(b) ------------ #Output 1
11.6. else স্টেটমেন্ট (বর্ধিত)
11.6.1. for i in range(10): if i == 999: break else: print("Unbroken 1") ----------------- #Output Unbroken 1
11.6.2. try: print(1) except ZeroDivisionError: print(2) else: print(3) try: print(1/0) except ZeroDivisionError: print(4) else: print(5) -------------------- #Output 1 3 4
11.7. __main__
11.7.1. def function(): print("This is a module function") if __name__=="__main__": print("This is a script") ------------------ #Output This is a script
11.8. জরুরি কিছু লাইব্রেরী
11.8.1. Web
11.8.1.1. Django
11.8.1.2. Flask
11.8.1.3. CherryPy
11.8.2. Data Scraping
11.8.2.1. BeautifulSoup
11.8.3. Math & Science
11.8.3.1. matplotlib
11.8.3.2. NumPy
11.8.3.3. SciPy
11.8.4. Game
11.8.4.1. Panda3D
11.8.4.2. pygame
11.9. প্যাকেজ
11.9.1. MyPackage/ LICENSE.txt README.txt setup.py mypackage/ __init__.py sololearn.py sololearn2.py
11.9.1.1. __init__.py
11.9.1.2. setup.py
11.9.1.2.1. from distutils.core import setup setup( name='MyPackage', version='0.1dev', packages=['mypackage',], license='MIT', long_description=open('README.txt').read(), )
11.10. ইউজারের জন্য প্যাকেজিং
11.10.1. windows
11.10.1.1. py2exe
11.10.2. PyInstaller
11.10.3. cx_Freeze
11.10.4. OSX
11.10.4.1. py2app
11.10.4.2. PyInstaller
11.10.4.3. cx_Freeze